· Charlotte Will · 12 min read
How to Set Up a Web Scraping Project Using BeautifulSoup and Requests
Learn how to set up a web scraping project using BeautifulSoup and Requests. Discover step-by-step guides, best practices, and advanced techniques for efficient data extraction from websites.
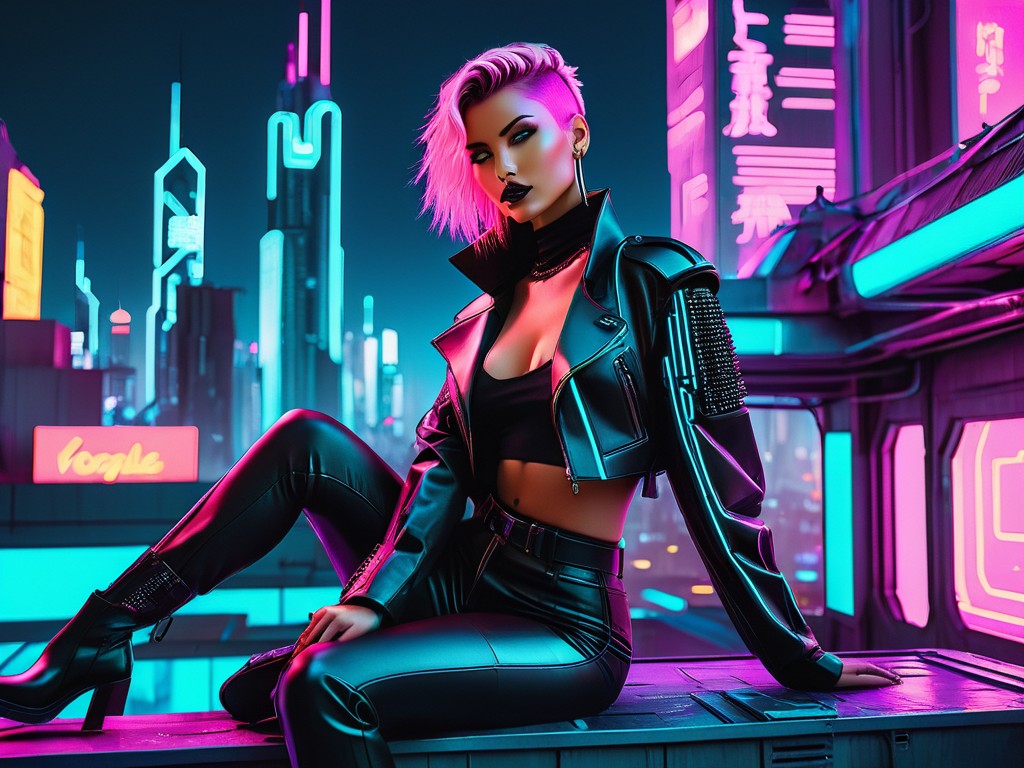
Imagine you have a mountain of data scattered across various websites that you need to gather for analysis, but manually extracting it would take days. Web scraping comes to the rescue! With Python’s powerful libraries like BeautifulSoup and Requests, you can automate this process, saving time and effort. In this article, we’ll guide you through setting up a web scraping project that makes data extraction seamless and efficient. Whether you’re a beginner or looking to expand your web scraping skills, we’ve got you covered.
We’ll start with the basics of what web scraping is and why BeautifulSoup and Requests are your best friends in this journey. We’ll then walk you through setting up your Python environment, understanding HTML and HTTP requests, and writing your first web scraper. Additionally, we’ll cover best practices, troubleshooting common issues, and even touch on handling dynamic content and scaling your project. By the end of this article, you’ll be equipped to scrape data from websites efficiently and legally.
Let’s dive into the world of web scraping and unlock a treasure trove of data!
Introduction to Web Scraping
Web scraping is the process of extracting data from websites automatically, often using software tools. This technique allows you to gather large amounts of information quickly and efficiently, making it ideal for tasks like market research, data analysis, and content aggregation.
What is Web Scraping?
Web scraping involves extracting data from web pages, often structured in HTML or XML format. The extracted data can then be stored, analyzed, or used to populate databases. This process is particularly useful for automating repetitive tasks that would otherwise be time-consuming and tedious.
Benefits of Web Scraping
Web scraping offers several benefits. For instance, it helps you gather real-time data from various sources, which can be invaluable for businesses looking to stay competitive. It also allows you to maintain a database of information that can be updated automatically, saving countless hours of manual work.
For more in-depth insights and examples on web scraping techniques, check out our article on How to Use BeautifulSoup and Requests for Effective Web Scraping. This guide offers practical tips and case studies that can help you understand the broader applications of web scraping.
Why Use BeautifulSoup and Requests?
When it comes to web scraping, Python’s BeautifulSoup library is a popular choice due to its ease of use and powerful features. Combined with the Requests library, you can easily fetch and parse web content, making your scraping project more efficient.
Introduction to BeautifulSoup
BeautifulSoup is a Python library designed for parsing HTML and XML documents. It provides simple methods to navigate, search, and modify the parse tree. BeautifulSoup makes it easy to extract data from web pages by providing an intuitive API for traversing and searching the parse tree.
Introduction to Requests Library
The Requests library is another essential tool for web scraping. It simplifies the process of making HTTP requests in Python, allowing you to fetch content from web pages easily. With Requests, you can handle various HTTP methods like GET and POST, manage cookies, sessions, and headers, making it a versatile choice for web scraping tasks.
For detailed steps on integrating APIs into your web scraping project, see our guide on How to Integrate APIs into Your Web Scraping Project Using Python. This article can help you extend the functionality of your scraping project by leveraging APIs.
Setting Up Your Python Environment
Before you start scraping, it’s crucial to set up your environment correctly. This includes installing the necessary libraries and setting up a clean workspace.
Installing Necessary Libraries
To get started, you need to install the BeautifulSoup and Requests libraries. You can do this using pip, Python’s package installer.
pip install beautifulsoup4 requests
Once installed, you can import these libraries in your Python script to begin scraping data. For instance:
import requests
from bs4 import BeautifulSoup
url = "https://example.com"
response = requests.get(url)
soup = BeautifulSoup(response.content, 'html.parser')
print(soup.prettify())
This code fetches the content of a web page and parses it using BeautifulSoup, allowing you to extract data easily.
For more advanced techniques on handling web scraping projects, check out our guide on Automated Pricing Strategies Using Machine Learning and Web Scraping. This article offers a unique perspective on how web scraping can be combined with machine learning to create sophisticated pricing strategies.
Basic Concepts of Web Scraping
Understanding the basic concepts of web scraping is crucial before diving into more complex tasks. This includes knowing how to parse HTML and handle HTTP requests, which form the foundation of web scraping.
Understanding HTML and HTTP Requests
Web pages are structured using HTML, a markup language that defines the structure of web documents. When you make an HTTP request to fetch a webpage, the server responds with HTML content.
For example, if you want to extract data from an online store:
import requests
from bs4 import BeautifulSoup
url = "https://example-store.com"
response = requests.get(url)
soup = BeautifulSoup(response.content, 'html.parser')
products = soup.find_all('div', class_='product')
for product in products:
print(product.text)
This script fetches the content of a web page and extracts all product details, which can then be processed further.
Handling Web Pages with BeautifulSoup
BeautifulSoup provides various methods to navigate and search the HTML parse tree. You can use find
, find_all
, and other methods to extract specific elements:
title = soup.find('h1')
print(title.text)
For more advanced scraping techniques and handling complex sites, see our guide on What is Web Scraping Using Selenium for Complex Sites?. This article explores how to use Selenium, another powerful tool for web scraping, especially when dealing with dynamic content.
Step-by-Step Guide to Setting Up a Web Scraping Project
Now that you have the basics down, let’s walk through setting up your web scraping project step by step.
Writing Your First Web Scraper
Start by identifying the data you need and the website from which you’ll extract it. Once you have your target, follow these steps:
- Fetch the content: Use Requests to fetch the webpage.
- Parse the HTML: Use BeautifulSoup to parse and analyze the HTML content.
- Extract data: Utilize BeautifulSoup’s methods to extract specific elements.
For example, if you want to scrape product details from an e-commerce site:
import requests
from bs4 import BeautifulSoup
url = "https://example-ecommerce.com"
response = requests.get(url)
soup = BeautifulSoup(response.content, 'html.parser')
products = soup.find_all('div', class_='product')
for product in products:
name = product.find('h2').text
price = product.find('span', class_='price').text
print(name, price)
This script extracts product names and prices from an e-commerce site.
Debugging Common Issues
Web scraping isn’t always smooth sailing. You may encounter errors like HTTP 403 Forbidden or timeout issues. Debugging these requires understanding common pitfalls:
- Check HTTP status codes: Ensure the request was successful.
- Handle exceptions: Use try-except blocks to manage errors gracefully.
For more tips on handling errors and improving your scraping efficiency, see our guide on How to Make an API Call for Web Scraping Using Python. This article provides practical advice on making API calls and handling data effectively.
Best Practices for Web Scraping
To ensure your web scraping project is both effective and legal, follow these best practices:
Ethical Considerations and Legalities
Always respect the website’s terms of service and robots.txt file. Avoid overloading servers with too many requests, and use rate limiting to ensure your scraping doesn’t disrupt the website’s functionality.
For more insights on ethical web scraping and legal considerations, check out our article on How to Extract Data Using Web Scraping APIs. This guide offers a comprehensive look at ethical scraping practices and the legal implications.
Advanced Techniques in Web Scraping
Web scraping can get complex, especially when dealing with dynamic content and large-scale data extraction.
Handling Dynamic Content with BeautifulSoup and Requests
Some websites use JavaScript to load content dynamically, making it challenging for traditional web scraping methods. However, you can still extract this data by waiting for the JavaScript to load and then parsing it:
import requests
from bs4 import BeautifulSoup
url = "https://example-site.com"
response = requests.get(url)
soup = BeautifulSoup(response.content, 'html.parser')
# Wait for JavaScript to load and then parse
For advanced techniques on handling complex sites, see our guide on What is Web Scraping Using Puppeteer?. This article explores how to use Puppeteer, a headless browser, for web scraping.
Scaling Your Web Scraping Project
As your project grows, consider scaling up to handle larger datasets and more frequent updates.
Optimizing Performance and Scalability
Optimize your scraping project by using techniques like multithreading, caching responses, and handling rate limits effectively. For instance, you can set up serverless functions to manage web scraping tasks:
import boto3
client = boto3.client('lambda')
response = client.invoke(
FunctionName='web_scraper',
InvocationType='RequestResponse'
)
For more on setting up serverless functions for web scraping, see our guide on How to Set Up Serverless Functions for Web Scraping with Amazon API Gateway.
Troubleshooting and Common Errors
Web scraping can be tricky, and you might encounter various issues along the way.
Handling HTTP Errors and Timeouts
Common errors include 403 Forbidden, timeouts, and network issues. To handle these:
- Check and adjust headers: Sometimes adding or changing headers can resolve 403 errors.
- Implement retries and timeouts: Use libraries like
retry
to handle transient network issues.
Dealing with Anti-Scraping Techniques
Websites often implement anti-scraping techniques to deter scraping. To avoid detection:
- Use rotating proxies: Rotating through different IP addresses can help evade detection.
- Scrape at a reasonable rate: Avoid overloading the server with too many requests.
Real-World Applications of Web Scraping
Web scraping has numerous real-world applications, from market research to content aggregation.
Case Studies and Examples
For instance, automated pricing strategies often rely on web scraping to gather competitor prices. Another example is aggregating product reviews from various websites for a comparative analysis.
For more on automated pricing strategies using web scraping, check out our guide on Automated Pricing Strategies Using Machine Learning and Web Scraping.
Conclusion
By now, you should have a good grasp of how to set up and manage a web scraping project using BeautifulSoup and Requests. From setting up your environment to handling complex content, we’ve covered a broad range of topics.
Recap of Key Points
- Setting up your environment with BeautifulSoup and Requests
- Understanding HTML and HTTP requests
- Writing and debugging your first web scraper
Next Steps and Further Learning
To deepen your understanding, explore more advanced topics like handling dynamic content or setting up serverless functions. For more resources and in-depth guides, check out our series on web scraping techniques.
Quick Takeaways
- Install Necessary Libraries: Ensure you have BeautifulSoup and Requests installed to fetch and parse web content.
- Understand HTML and HTTP: Familiarize yourself with how to make HTTP requests and parse HTML using BeautifulSoup.
- Write Your First Web Scraper: Use examples like extracting product details from an e-commerce site to get started.
- Handle Common Issues: Debug common errors such as HTTP 403 Forbidden and timeouts by checking headers, implementing retries, and managing rate limits.
- Ethical Considerations: Always respect the website’s terms of service and robots.txt file to avoid legal issues.
- Advanced Techniques: Learn how to handle dynamic content using headless browsers like Puppeteer and scale your project with serverless functions.
- Real-World Applications: Explore practical applications like automated pricing strategies and market research to see the true value of web scraping.
FAQs
What are some common mistakes to avoid when setting up a web scraping project?
- Common mistakes include overloading servers with too many requests, ignoring the website’s terms of service and robots.txt file, and not handling errors gracefully. To avoid these issues, use rate limiting, respect legal guidelines, and implement proper error handling in your code. For more tips on best practices, check out our guide on How to Extract Data Using Web Scraping APIs.
Can I use BeautifulSoup and Requests for large-scale data extraction projects?
- Yes, BeautifulSoup and Requests are well-suited for large-scale data extraction projects. However, for better performance and scalability, consider using techniques like multithreading or setting up serverless functions. Our article on How to Set Up Serverless Functions for Web Scraping with Amazon API Gateway provides detailed steps to help you scale your project effectively.
How do I handle dynamic content on websites with BeautifulSoup and Requests?
- Handling dynamic content can be challenging since traditional web scraping methods might not work. To scrape dynamically loaded data, you can use headless browsers like Puppeteer or Selenium. These tools render the JavaScript on the page, allowing you to extract dynamic content effectively. For more information, see our guide on What is Web Scraping Using Puppeteer?.
What are some alternatives to BeautifulSoup and Requests for web scraping?
- While BeautifulSoup and Requests are excellent choices, you can also consider alternatives like Scrapy for a more robust solution. Libraries such as Selenium are great for handling JavaScript-heavy websites, and tools like Scrapy-Redis can help manage large-scale web scraping projects. For a comprehensive guide on handling complex sites, check out our article on What is Web Scraping Using Selenium for Complex Sites?.
How can I ensure my web scraping project complies with legal standards?
- To ensure compliance, always check the website’s terms of service and robots.txt file to avoid legal issues. Respect rate limits, use proxies to mask your IP address, and handle errors gracefully to minimize server load. For more detailed guidance on ethical web scraping practices, see our article on How to Extract Data Using Web Scraping APIs.
Feedback and Social Shares
We hope this guide has provided you with valuable insights into setting up a web scraping project using BeautifulSoup and Requests. Your feedback is incredibly important to us as it helps us improve and create more helpful content in the future.
Please take a moment to share your thoughts: Did this guide help you get started with web scraping? Do you have any tips or tricks to share?
We’d also love it if you could help spread the word by sharing this article on your social media platforms. Your support is greatly appreciated!
Lastly, we’d love to hear from you—what other web scraping topics would you like us to cover in future articles? Leave your suggestions in the comments below!