· Charlotte Will · 5 min read
How to Make an API Call for Web Scraping Using Python
Learn how to make API calls for web scraping using Python, a powerful method for extracting structured data efficiently. This comprehensive guide covers the basics of HTTP requests, handling different response formats, and implementing best practices for reliable web scraping solutions. Ideal for both beginners and intermediate developers.
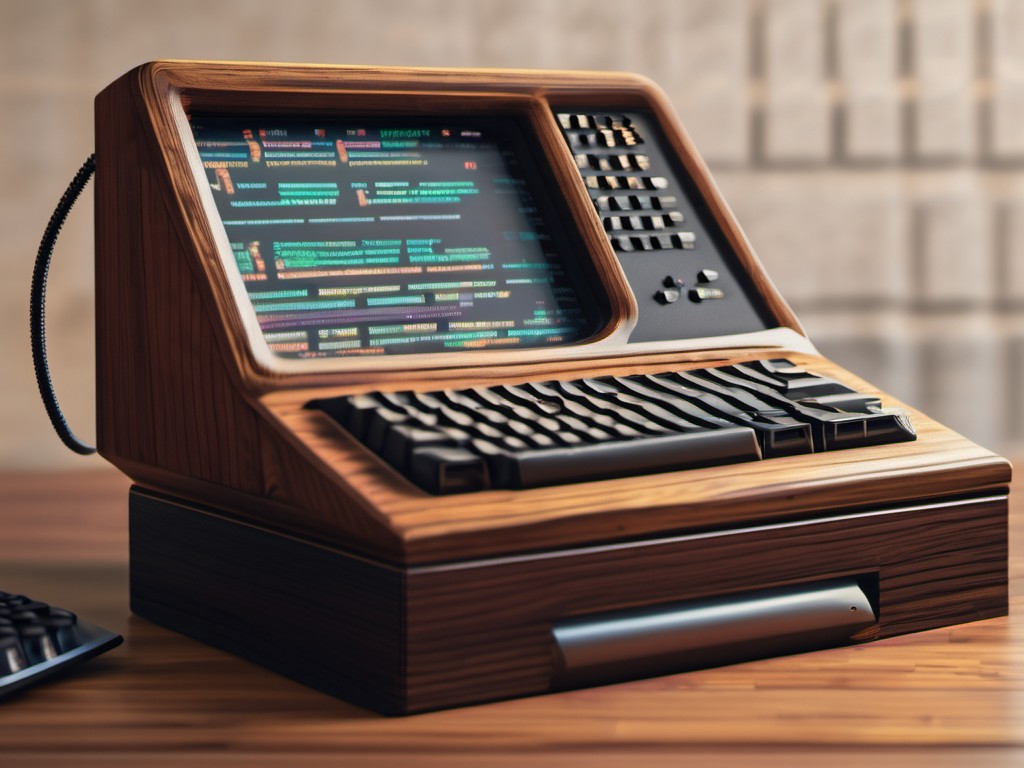
Web scraping has become an essential skill in data extraction, analysis, and automation. While traditional web scraping methods involve parsing HTML directly from websites, using APIs can be more efficient and less error-prone. In this guide, we’ll walk you through making API calls for web scraping using Python, one of the most popular programming languages.
Understanding Web Scraping with APIs
Web scraping with APIs involves making HTTP requests to a server that returns data in a structured format like JSON or XML. Unlike traditional web scraping, which may require dealing with ever-changing HTML structures and potential legal issues, API-based scraping is often more stable and compliant.
Why Use APIs for Web Scraping?
- Structured Data: APIs return data in a consistent format, making it easier to parse.
- Less Error-Prone: Changes in website layouts don’t affect API responses.
- Compliance: Using an API is often within the terms of service of many websites.
- Rate Limiting: APIs usually come with rate limits, preventing you from overwhelming a server.
Setting Up Your Environment
Before diving into code, ensure you have the necessary tools and libraries installed:
Required Libraries
requests
: For making HTTP requests.json
: For parsing JSON responses.
You can install these using pip:
pip install requests
Making Your First API Call in Python
Let’s start with a simple example to demonstrate how to make an API call and parse the response data. We’ll use the JSONPlaceholder API, which is perfect for beginners.
Step-by-Step Guide
1. Import Libraries
First, import the required libraries:
import requests
2. Make an HTTP GET Request
Use the requests.get()
method to make a request to the API endpoint:
url = "https://jsonplaceholder.typicode.com/posts"
response = requests.get(url)
3. Check the Response Status Code
Ensure the request was successful by checking the status code:
if response.status_code == 200:
print("Success!")
else:
print(f"Failed with status code {response.status_code}")
4. Parse the JSON Response
If the request was successful, parse the JSON data:
data = response.json()
print(data)
Complete Example Code
Here’s the complete code snippet for making an API call and parsing the response:
import requests
url = "https://jsonplaceholder.typicode.com/posts"
response = requests.get(url)
if response.status_code == 200:
data = response.json()
print(data)
else:
print(f"Failed with status code {response.status_code}")
Handling API Keys and Headers
Many APIs require authentication using an API key or tokens. You can include these in the request headers.
Example with API Key
url = "https://api.example.com/data"
headers = {
"Authorization": "Bearer YOUR_API_KEY"
}
response = requests.get(url, headers=headers)
Posting Data to an API
Sometimes you may need to send data to an API. This can be done using the requests.post()
method.
Example with JSON Payload
url = "https://jsonplaceholder.typicode.com/posts"
data = {
"title": "foo",
"body": "bar",
"userId": 1
}
headers = {"Content-Type": "application/json"}
response = requests.post(url, json=data, headers=headers)
Error Handling
Robust error handling is crucial when making API calls. Use try-except blocks to catch and handle exceptions.
Example with Error Handling
import requests
from requests.exceptions import HTTPError, ConnectionError, Timeout, RequestException
url = "https://jsonplaceholder.typicode.com/posts"
try:
response = requests.get(url)
response.raise_for_status()
data = response.json()
print(data)
except HTTPError as http_err:
print(f"HTTP error occurred: {http_err}")
except ConnectionError as conn_err:
print(f"Connection error occurred: {conn_err}")
except Timeout as timeout_err:
print(f"Timeout error occurred: {timeout_err}")
except RequestException as req_err:
print(f"An error occurred: {req_err}")
Working with Different API Response Formats
APIs often return data in different formats like JSON, XML, or even plain text. Parse the response based on its format.
JSON Response Parsing
data = response.json()
XML Response Parsing
import xml.etree.ElementTree as ET
tree = ET.ElementTree(ET.fromstring(response.content))
root = tree.getroot()
# Parse XML data
Best Practices for API-Based Web Scraping
- Respect Rate Limits: Always honor the rate limits specified by APIs to avoid being blocked.
- Error Handling: Implement comprehensive error handling to manage network issues and API changes gracefully.
- Caching Responses: Cache responses where appropriate to reduce the number of requests made to an API.
- Logging: Keep logs of your API interactions for debugging and monitoring purposes.
- Environment Variables: Store sensitive information like API keys in environment variables or configuration files, not directly in your code.
Advanced Techniques
Using Asynchronous Requests with aiohttp
For more efficient data retrieval, especially when dealing with multiple endpoints, consider using asynchronous requests:
import aiohttp
import asyncio
async def fetch(session, url):
async with session.get(url) as response:
return await response.json()
async def main():
url = "https://jsonplaceholder.typicode.com/posts"
async with aiohttp.ClientSession() as session:
html = await fetch(session, url)
print(html)
asyncio.run(main())
Conclusion
Making API calls for web scraping using Python is an effective and efficient method to extract data. By understanding the basics of HTTP requests, handling different response formats, and implementing best practices, you can build robust and reliable web scraping solutions. Whether you’re a beginner or an intermediate developer, mastering API-based web scraping will open up numerous opportunities for data extraction and automation.
FAQs
1. What is the difference between traditional web scraping and using APIs?
Traditional web scraping involves parsing HTML directly from websites, while using APIs involves making HTTP requests to a server that returns structured data (like JSON). API-based scraping is often more stable and compliant with terms of service.
2. How can I handle authentication when making API calls?
Include your API key or token in the request headers using the Authorization
field:
headers = {
"Authorization": "Bearer YOUR_API_KEY"
}
response = requests.get(url, headers=headers)
3. How do I handle errors when making API calls?
Use try-except blocks to catch and handle exceptions like HTTPError, ConnectionError, Timeout, and RequestException:
try:
response = requests.get(url)
response.raise_for_status()
data = response.json()
except Exception as e:
print(f"An error occurred: {e}")
4. Why is it important to respect rate limits?
Respecting rate limits helps you avoid being blocked by the API provider and ensures fair usage of their resources. It also prevents your own system from becoming overwhelmed with too many requests.
5. Can I cache API responses to reduce the number of requests?
Yes, caching responses can significantly reduce the number of requests made to an API. You can use libraries like cachetools
or even a simple file-based caching mechanism to store and retrieve cached data.