· Charlotte Will · webscraping · 5 min read
What is Web Scraping Using Puppeteer?
Learn how to use Puppeteer for efficient and practical web scraping. This comprehensive guide covers the basics, advanced techniques, and best practices to help you extract data from websites using a headless browser. Ideal for beginners and experienced users alike, this article offers actionable advice on handling dynamic content, navigating pages, intercepting network requests, and more.
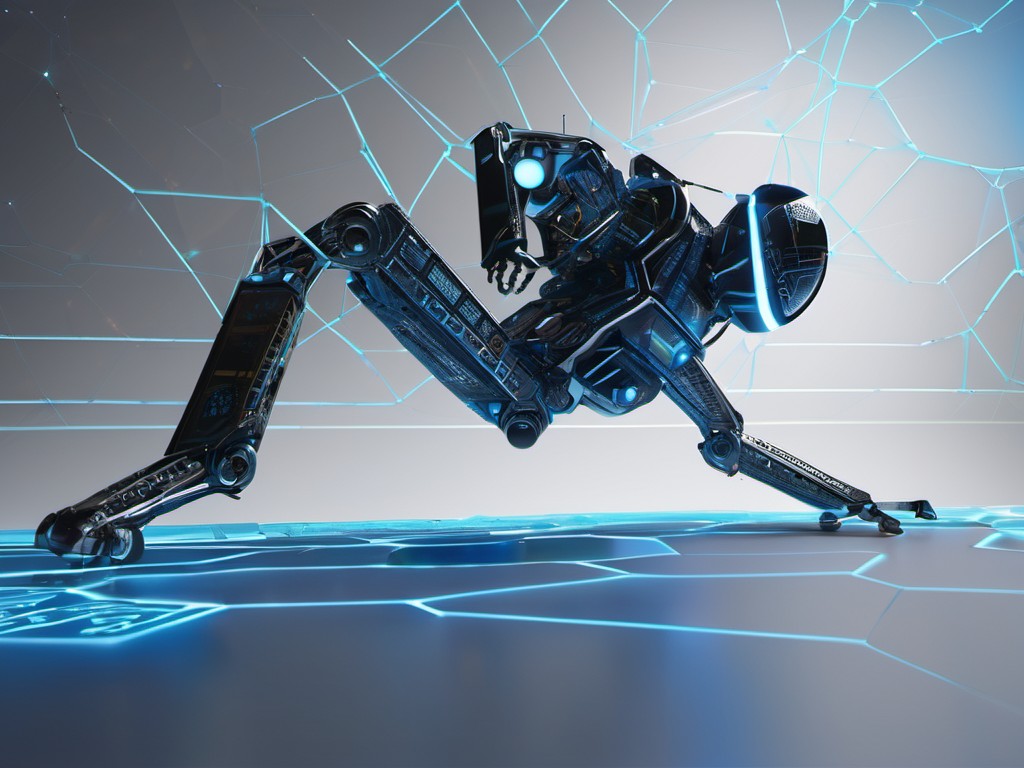
Introduction to Web Scraping Using Puppeteer
Web scraping has become an essential tool for data extraction, automation, and various other purposes in today’s digital landscape. Among the many tools available for web scraping, Puppeteer stands out as a powerful and flexible option. In this comprehensive guide, we will explore what Puppeteer is, how it can be used for web scraping, and provide practical examples to get you started.
What is Web Scraping?
Web scraping refers to the process of extracting data from websites. This data can then be used for various purposes such as market research, price monitoring, or even building databases. With the rise of big data and the increasing need for automation, web scraping has become more important than ever before.
What is Puppeteer?
Puppeteer is a Node library developed by the Chrome team at Google. It provides a high-level API to control headless Chrome or Chromium over the DevTools Protocol. Headless browsers are crucial for web scraping because they allow you to interact with web pages in the same way a human user would, but without a graphical interface.
Why Use Puppeteer for Web Scraping?
Puppeteer offers several advantages that make it an excellent choice for web scraping:
- Headless Browser: Allows you to automate interactions with web pages without opening a browser window.
- JavaScript Execution: Enables you to run JavaScript on the page, which is essential for handling dynamic content.
- Network Interception: Allows you to intercept network requests and responses, useful for data extraction.
- Screenshots and PDFs: Capable of taking screenshots and generating PDFs of web pages.
Getting Started with Puppeteer
Before diving into web scraping with Puppeteer, let’s set up a basic environment.
Installation
First, you need to have Node.js installed on your machine. You can download it from here. Once Node.js is installed, open your terminal and run the following commands:
npm install puppeteer
Basic Usage
Let’s start with a simple example to understand how Puppeteer works. Create a new file named scrape.js
and add the following code:
const puppeteer = require('puppeteer');
(async () => {
const browser = await puppeteer.launch();
const page = await browser.newPage();
await page.goto('https://example.com');
const title = await page.title();
console.log(`Title of the page: ${title}`);
await browser.close();
})();
This script launches a headless browser, navigates to https://example.com
, and logs the title of the page.
Advanced Web Scraping with Puppeteer
Now that we have a basic understanding of how Puppeteer works, let’s dive into more advanced web scraping techniques.
Handling Dynamic Content
Many modern websites use JavaScript to load content dynamically. To extract this data, you need to wait for the JavaScript to execute. Puppeteer makes this easy with its waitForSelector
and evaluate
methods.
const puppeteer = require('puppeteer');
(async () => {
const browser = await puppeteer.launch();
const page = await browser.newPage();
await page.goto('https://example.com/dynamic-content');
// Wait for the dynamic content to load
await page.waitForSelector('.dynamic-content');
// Extract the text of the dynamic content
const dynamicContent = await page.evaluate(() => {
return document.querySelector('.dynamic-content').innerText;
});
console.log(`Dynamic Content: ${dynamicContent}`);
await browser.close();
})();
Navigating and Clicking
Often, the data you need is hidden behind multiple clicks or navigation steps. Puppeteer allows you to simulate user interactions like clicking buttons and navigating through pages.
const puppeteer = require('puppeteer');
(async () => {
const browser = await puppeteer.launch();
const page = await browser.newPage();
await page.goto('https://example.com/home');
// Click a button to navigate to another page
await page.click('#navigate-button');
// Wait for the new page to load
await page.waitForSelector('.target-content');
const targetContent = await page.evaluate(() => {
return document.querySelector('.target-content').innerText;
});
console.log(`Target Content: ${targetContent}`);
await browser.close();
})();
Intercepting Network Requests
Sometimes, the data you need is loaded via network requests rather than rendered on the page. Puppeteer allows you to intercept these requests and extract the necessary information.
const puppeteer = require('puppeteer');
(async () => {
const browser = await puppeteer.launch();
const page = await browser.newPage();
await page.goto('https://example.com/network-request');
// Intercept network requests
await page.setRequestInterception(true);
page.on('request', request => {
if (request.resourceType() === 'document') {
request.continue();
} else if (request.url().includes('api')) {
request.respond({
status: 200,
contentType: 'application/json',
body: JSON.stringify({ message: 'Intercepted API response' }),
});
} else {
request.continue();
}
});
// Extract data from the intercepted response
await page.evaluate(() => {
fetch('/api/data')
.then(response => response.json())
.then(data => console.log(`Intercepted Data: ${JSON.stringify(data)}`));
});
await browser.close();
})();
Best Practices for Web Scraping with Puppeteer
While Puppeteer is a powerful tool, it’s essential to use it responsibly and ethically. Here are some best practices:
Respect Robots.txt
Before scraping any website, check its robots.txt
file to ensure you comply with the site’s rules regarding web crawlers and bots.
Rate Limiting
Implement rate limiting to prevent overwhelming the server with too many requests in a short period. This helps to avoid getting your IP address blocked.
Error Handling
Include proper error handling to manage network errors, timeouts, and other issues that may arise during the scraping process.
Legal Considerations
Ensure you have the legal right to scrape the data from the website. Some websites explicitly prohibit web scraping in their terms of service.
Conclusion
Web scraping using Puppeteer is a powerful way to automate data extraction from web pages. Whether you’re dealing with static content or dynamic JavaScript-driven sites, Puppeteer provides the tools needed to get the job done efficiently and effectively. By following best practices and respecting the target website’s rules, you can harness the full potential of web scraping for a wide range of applications.
FAQ
Q: How do I handle CAPTCHAs with Puppeteer? A: Handling CAPTCHAs is complex and often involves using third-party services or manual intervention. It’s best to avoid websites that use CAPTCHAs for web scraping.
Q: Can Puppeteer handle AJAX requests? A: Yes, Puppeteer can handle AJAX requests by intercepting network responses and extracting the necessary data.
Q: Is web scraping with Puppeteer legal? A: The legality of web scraping depends on various factors, including the target website’s terms of service and local laws. Always ensure you have permission to scrape a site before proceeding.
Q: How can I avoid getting my IP address blocked during web scraping? A: Implement rate limiting, rotate proxies, and use headers that mimic human browsers to reduce the likelihood of being blocked.
Q: Can Puppeteer be used for automated testing? A: Yes, Puppeteer is commonly used for end-to-end testing due to its ability to simulate user interactions with web pages.