· Charlotte Will · 4 min read
How to Integrate APIs into Your Web Scraping Project Using Python
Learn how to integrate APIs into your web scraping projects using Python for more reliable, efficient, and compliant data extraction. This guide covers setting up your environment, making API requests, parsing responses, handling errors, and best practices for successful API integration in web scraping.
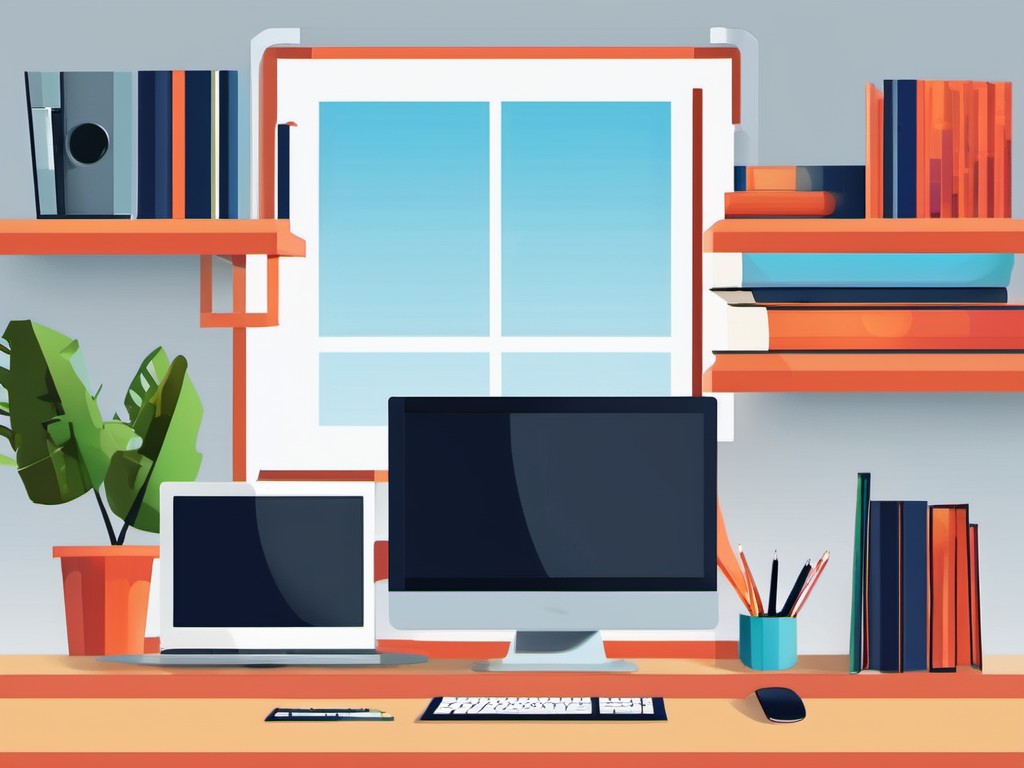
Web scraping is an essential skill in the toolkit of any modern developer or data scientist, enabling you to extract valuable information from web pages. However, scraping can become complex and unreliable due to ever-changing website structures and legal concerns. This is where APIs come into play. By integrating APIs into your web scraping projects, you can gain more reliable access to data while complying with terms of service. Let’s dive deep into how you can achieve this using Python.
Understanding Web Scraping and APIs
What is Web Scraping?
Web scraping involves extracting data from websites by sending HTTP requests to fetch the HTML content and then parsing it to retrieve relevant information. Tools like BeautifulSoup and libraries such as Requests in Python are commonly used for this purpose.
What are APIs?
APIs (Application Programming Interfaces) provide a structured way to access data from web services. They return data in formats like JSON or XML, making it easier to parse and integrate into your projects compared to raw HTML scraping.
Why Integrate APIs into Your Web Scraping Project?
Integrating APIs offers several advantages:
- Reliability: API responses are more stable and predictable than web page structures.
- Compliance: Many websites prefer you use their API over scraping, adhering to their terms of service.
- Efficiency: APIs often provide the exact data you need without parsing unnecessary HTML.
- Performance: APIs can handle large-scale data extraction more efficiently than traditional web scraping methods.
Setting Up Your Python Environment
Before diving into integration, ensure your environment is set up properly.
Installing Necessary Libraries (Requests, BeautifulSoup)
You’ll need two main libraries: requests
for making HTTP requests and BeautifulSoup
from the bs4
package for parsing HTML. Install them using pip:
pip install requests beautifulsoup4
Step-by-Step Guide to API Integration in Web Scraping
Making API Requests
First, you need to make a request to the API endpoint. Here’s an example using the requests
library:
import requests
url = "https://api.example.com/data"
response = requests.get(url)
data = response.json() # Assuming JSON response
Parsing API Responses
Once you have the data, parse it as needed:
for item in data['items']:
print(item['name'])
Handling Errors and Edge Cases
Always handle potential errors and edge cases to make your scraper robust:
try:
response = requests.get(url)
response.raise_for_status() # Raise an HTTPError for bad responses
except requests.exceptions.HTTPError as http_err:
print(f"HTTP error occurred: {http_err}")
except Exception as err:
print(f"Other error occurred: {err}")
Best Practices for API Integration in Web Scraping
- Rate Limiting: Respect the API’s rate limits to avoid getting blocked.
- Error Handling: Implement comprehensive error handling to manage different types of failures.
- Data Storage: Efficiently store extracted data using databases like SQLite or cloud storage services.
- Documentation: Always refer to the API documentation for the most accurate and up-to-date information.
- Ethical Considerations: Ensure your scraping activities comply with legal and ethical guidelines.
Example Project: Building a Simple API-Integrated Web Scraper
Let’s create a simple web scraper that fetches data from an API and extracts specific information:
import requests
def fetch_api_data(url):
try:
response = requests.get(url)
response.raise_for_status()
return response.json()
except requests.exceptions.HTTPError as http_err:
print(f"HTTP error occurred: {http_err}")
except Exception as err:
print(f"Other error occurred: {err}")
return None
def extract_data(api_data):
for item in api_data['items']:
print(item['name'])
url = "https://api.example.com/data"
data = fetch_api_data(url)
extract_data(data)
Conclusion
Integrating APIs into your web scraping projects can significantly enhance the reliability, efficiency, and compliance of your data extraction efforts. By leveraging Python libraries like requests
and BeautifulSoup
, you can efficiently parse API responses and extract the data you need. Always remember to adhere to best practices and ethical considerations for sustainable web scraping.
FAQ
What are some common mistakes when integrating APIs into web scraping projects?
Common mistakes include ignoring rate limits, not handling errors properly, and failing to refer to API documentation. Always ensure your code is robust and ethical.
How do I handle rate limits in API requests?
Implement logic to throttle your requests according to the API’s rate limit. Use time.sleep()
or more advanced techniques like token buckets to manage request rates effectively.
What libraries are essential for web scraping and API integration in Python?
The core libraries are requests
for making HTTP requests, BeautifulSoup
from the bs4
package for parsing HTML, and optionally pandas
for data manipulation and storage.
Can I use this technique for large-scale data extraction?
Yes, API integration is particularly useful for large-scale data extraction due to its reliability and efficiency. However, ensure you handle rate limits and potential server responses effectively.
Is it legal to scrape websites using APIs?
Legality depends on the website’s terms of service. Many sites prefer API usage over web scraping. Always check the site’s robots.txt
file and terms of service before proceeding.