· Charlotte Will · 5 min read
How to Use BeautifulSoup and Requests for Effective Web Scraping
Learn how to use BeautifulSoup and Requests for efficient web scraping in Python with our comprehensive guide. Discover practical techniques, step-by-step instructions, and code examples to extract valuable data from websites. Ideal for beginners and intermediate users alike!
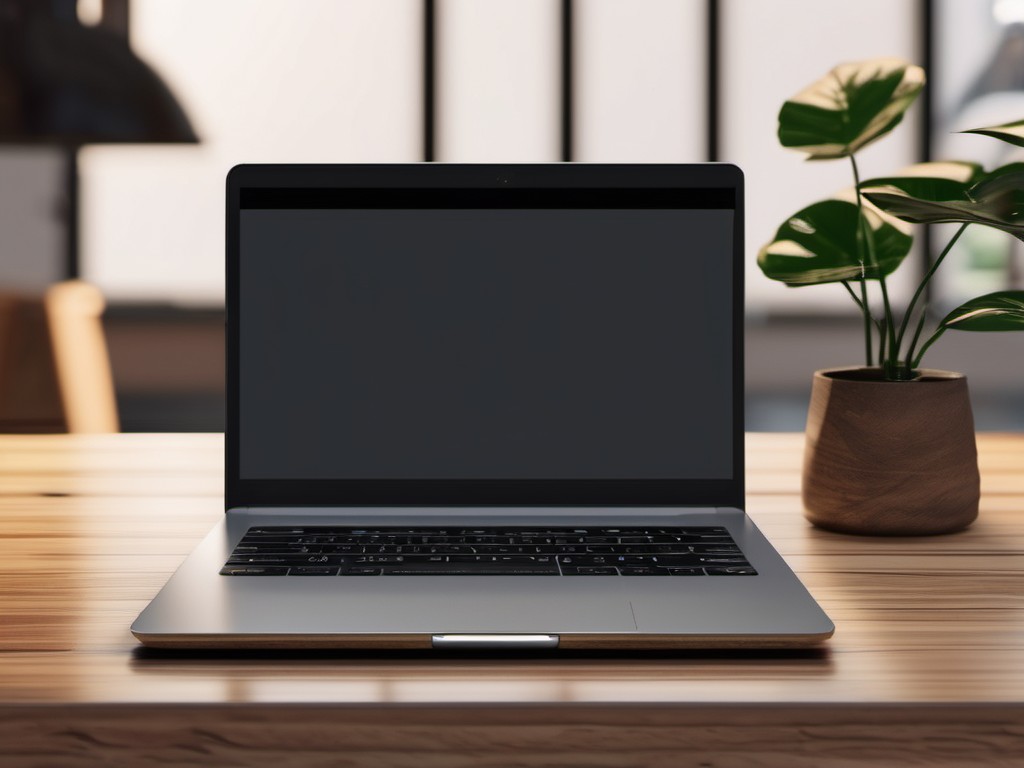
Welcome to our comprehensive guide on using BeautifulSoup and Requests for effective web scraping! If you’re new to web scraping, don’t worry—we’ll walk you through everything step by step. By the end of this article, you’ll be well-equipped to extract valuable data from websites using Python.
Introduction to Web Scraping
Web scraping is a technique used to extract data from websites. It can be incredibly useful for tasks like collecting market research, monitoring prices, or even gathering news articles. With the right tools, anyone can become proficient at web scraping—and today, we’ll focus on two powerful libraries: BeautifulSoup and Requests.
Setting Up Your Environment
Before diving into the code, let’s make sure you have a proper environment set up. You’ll need Python installed on your computer. If not, download it from python.org.
Next, create a virtual environment to manage your dependencies:
python -m venv webscraping_env
source webscraping_env/bin/activate # On Windows use `webscraping_env\Scripts\activate`
Now, install the required libraries using pip:
pip install requests beautifulsoup4
Making HTTP Requests
The first step in web scraping is to fetch the HTML content of a webpage. We’ll use the requests
library for this purpose. Here’s how you can make an HTTP request:
Basic GET Request
import requests
url = 'https://example.com'
response = requests.get(url)
print(response.text)
This code sends a GET request to the specified URL and prints out the HTML content of the webpage.
Parsing HTML with BeautifulSoup
Now that we have the HTML content, we need to parse it to extract the relevant data. This is where BeautifulSoup
comes in handy. Let’s see how to use it:
Basic Parsing
from bs4 import BeautifulSoup
import requests
url = 'https://example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
print(soup.prettify()) # Prints a nicely formatted HTML
The BeautifulSoup
object is created by passing the HTML content and specifying the parser. Here, we use 'html.parser'
, but you can also use other parsers like lxml
.
Extracting Data
Let’s extract some data from a webpage. Suppose we want to scrape all the links on a page:
Extracting Links
links = soup.find_all('a')
for link in links:
print(link.get('href'))
The find_all
method searches for all tags of the specified type (in this case, <a>
tags) and returns a list of them. The get
method retrieves the value of the href
attribute for each link.
Navigating the DOM
Understanding how to navigate the Document Object Model (DOM) is crucial for effective web scraping:
Parent, Sibling, and Child Relationships
- Parent: The tag that contains another tag.
- Child: A tag contained within another tag.
- Sibling: Tags that share the same parent.
Example
parent = soup.find('div', class_='container')
children = parent.find_all('p') # Find all <p> tags inside the <div>
for child in children:
print(child.text)
This code finds a <div>
with the class 'container'
and then extracts all its child paragraph (<p>
) tags, printing their text content.
Handling Dynamic Content
Many modern websites load content dynamically using JavaScript. To handle such cases, you might need to use a tool like Selenium along with BeautifulSoup:
Using Selenium for Dynamic Content
from selenium import webdriver
from bs4 import BeautifulSoup
driver = webdriver.Chrome() # Make sure you have ChromeDriver installed and in your PATH
driver.get('https://example.com')
html = driver.page_source
soup = BeautifulSoup(html, 'html.parser')
driver.quit()
This code uses Selenium to load a webpage and then extracts its HTML content using BeautifulSoup.
Respecting Robots.txt
Always remember to respect the robots.txt
file of a website, which specifies which parts of the site can be crawled by bots:
Checking robots.txt
import requests
robots_url = 'https://example.com/robots.txt'
response = requests.get(robots_url)
print(response.text)
This code fetches and prints the robots.txt
file of a website, so you can see which parts are allowed to be scraped.
Handling Exceptions
When web scraping, it’s important to handle exceptions gracefully:
Exception Handling Example
try:
response = requests.get(url)
response.raise_for_status() # Raises an HTTPError for bad responses (4xx and 5xx)
except requests.exceptions.RequestException as e:
print(f"An error occurred: {e}")
This code handles common exceptions that might occur during web scraping, such as connection errors or invalid URLs.
Conclusion
Congratulations! You now have a solid foundation in using BeautifulSoup and Requests for effective web scraping. With practice, you’ll become proficient at extracting valuable data from websites.
Remember to always respect the terms of service of any website you scrape and adhere to ethical guidelines. Happy scraping!
FAQs
1. Can I use BeautifulSoup without Requests?
Yes, you can parse HTML content that is already stored in a variable or file using BeautifulSoup alone. However, Requests is commonly used for fetching the HTML from a website.
2. How do I handle paginated data?
For paginated data, you typically need to send multiple requests and iterate through the pages. You can often find the next page link using BeautifulSoup and then repeat the scraping process.
3. What should I do if a website blocks my IP?
If your IP gets blocked, consider using proxies or rotating user agents to avoid detection. Always respect the website’s policies and avoid excessive requests that could cause performance issues.
4. How can I improve the speed of my web scraper?
Using asynchronous requests with libraries like aiohttp
and asyncio
can significantly improve your scraping speed by making multiple requests concurrently. Additionally, optimizing your code and minimizing network delays are crucial for efficiency.
5. Are there any legal considerations I should be aware of?
Yes, web scraping can have legal implications. Always check the website’s terms of service and comply with copyright laws. Be mindful of sensitive data and privacy concerns, and consider obtaining permission if you plan to scrape a site extensively or for commercial purposes.