· Charlotte Will · Amazon API · 5 min read
How to Set Up Serverless Functions for Web Scraping with Amazon API Gateway
Discover how to set up serverless functions using Amazon API Gateway and AWS Lambda for efficient web scraping tasks. Learn best practices, handle rate limits, and deploy your function seamlessly.
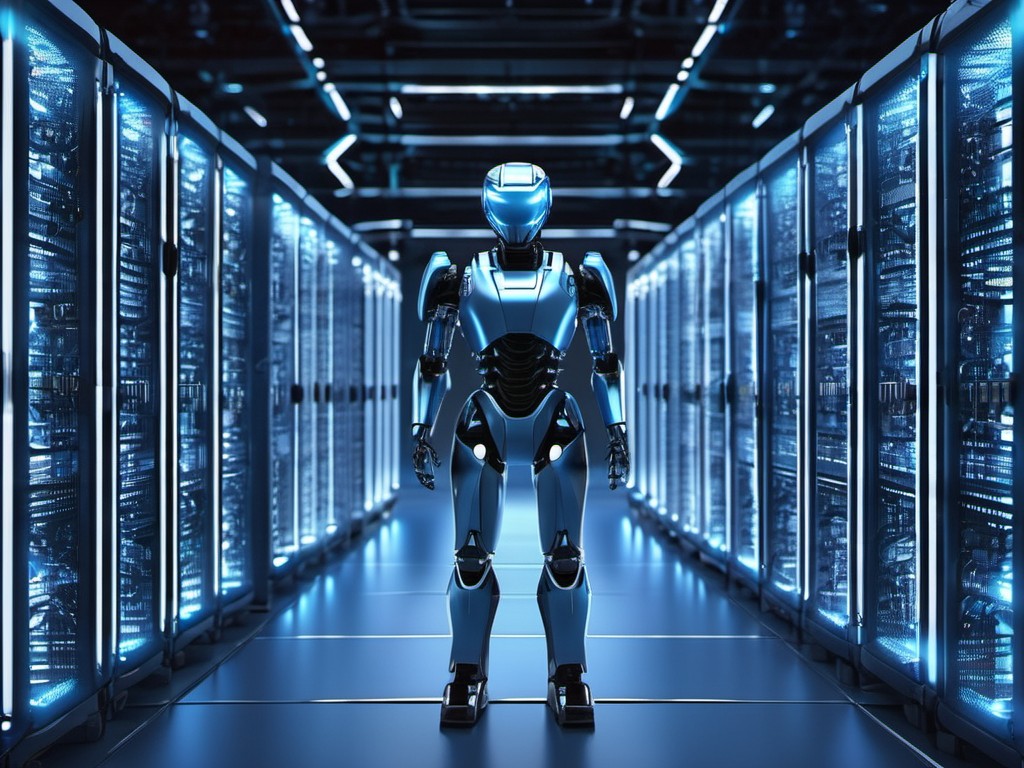
Web scraping has become an essential tool for extracting valuable data from websites. However, managing the infrastructure required for web scraping can be complex and time-consuming. This is where serverless functions come in handy. By using serverless architecture, you can focus more on writing code and less on maintaining servers. Amazon API Gateway, combined with AWS Lambda, offers a powerful platform to build and deploy serverless functions for web scraping efficiently.
Introduction
Serverless functions allow developers to run code without provisioning or managing servers. They are event-driven and automatically scale based on the demand. When it comes to web scraping, serverless functions provide an ideal environment for running scraping tasks that can be triggered by various events such as HTTP requests.
Amazon API Gateway acts as a front door for applications, enabling you to create RESTful APIs. By integrating Amazon API Gateway with AWS Lambda, you can build serverless web scraping solutions that are scalable, cost-effective, and easy to manage.
Setting Up AWS Lambda Functions
Before diving into the integration part, let’s set up an AWS Lambda function for our web scraping task. Here’s a step-by-step guide:
Step 1: Creating a New Lambda Function
- Sign in to your AWS Management Console and navigate to the AWS Lambda service.
- Click on “Create function.”
- Choose “Author from scratch” and configure the basic settings like function name, runtime (select Python), and permissions.
- Click “Create function” to proceed.
Step 2: Configuring the Lambda Function
Function Code: Replace the default code with your web scraping script written in Python. Make sure you include necessary libraries like
requests
orbeautifulsoup4
.import requests from bs4 import BeautifulSoup def lambda_handler(event, context): url = event['url'] response = requests.get(url) soup = BeautifulSoup(response.text, 'html.parser') # Extract data here... return { 'statusCode': 200, 'body': 'Data extracted successfully' }
Environment Variables: Add any environment variables your function might need.
IAM Role: Ensure that the Lambda function has the necessary IAM roles and permissions to access other AWS services if required.
Memory and Timeout: Adjust memory allocation and timeout settings based on your scraping task’s requirements.
Save Changes.
Integrating Amazon API Gateway
Now, let’s create an API in Amazon API Gateway that will act as a front door for our Lambda function:
Step 1: Creating an API
- Navigate to the Amazon API Gateway service in your AWS Management Console.
- Click on “Create API” and choose “REST API.”
- Configure the basic settings like API name, description, and protocol (HTTP).
- Click “Create API.”
Step 2: Linking the API to Lambda Function
- Create a Resource: Add a new resource (e.g.,
/scrape
). - Add Method: Select
POST
method for the resource and configure it to trigger the Lambda function created earlier. - Deploy API: Once the configuration is done, deploy your API to a stage (e.g.,
dev
).
Writing Web Scraping Code
Let’s dive into writing an efficient web scraping code. Here’s an example using Python:
Example Code Snippets
import requests
from bs4 import BeautifulSoup
def lambda_handler(event, context):
url = event['url']
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
# Example: Extracting title of the webpage
title = soup.title.string if soup.title else "No title found"
return {
'statusCode': 200,
'body': f'Title: {title}'
}
Best Practices for Efficient and Reliable Web Scraping
- Respect Robots.txt: Always check the
robots.txt
file of the website you intend to scrape. - Handle Rate Limits: Use appropriate headers and delay between requests to avoid getting blocked.
- Error Handling: Implement robust error handling to manage network errors and unexpected responses.
- Data Storage: Decide where to store the extracted data—could be a database, S3 bucket, or another service.
- Scalability: Design your scraping script to handle multiple requests efficiently.
Handling Rate Limits and Errors
When making API calls, it’s crucial to manage rate limits effectively:
Strategies for Handling Rate Limits
- Exponential Backoff: Increase the delay between retries exponentially to avoid overwhelming the server.
- Rotating Proxies or IPs: Use different proxies or IP addresses to distribute the load and reduce the chances of being blocked.
- Respect
robots.txt
: Always respect the website’s rules for crawling.
Implementing Error Handling and Retry Logic
import time
import requests
from bs4 import BeautifulSoup
def scrape(url):
retry_attempts = 3
delay = 5
while retry_attempts > 0:
try:
response = requests.get(url)
response.raise_for_status()
return BeautifulSoup(response.text, 'html.parser')
except requests.exceptions.RequestException as e:
print(f"Error occurred: {e}")
retry_attempts -= 1
time.sleep(delay)
raise Exception("Failed to scrape the URL after multiple attempts")
Testing and Deployment
Before deploying your serverless function, it’s essential to test it locally:
Step 1: Local Testing
- Test Lambda Function: Use AWS SAM or local development tools like PyCharm or VSCode to test your Lambda function locally.
- Mock API Gateway: Utilize tools like Postman or cURL to simulate API Gateway requests and verify your function’s behavior.
Step 2: Deployment
- Deploy Lambda Function: Once you’re satisfied with the local testing, deploy the Lambda function using AWS CLI or directly from the AWS Management Console.
- Update API Gateway: Ensure that any changes in your Lambda function are correctly reflected in the integrated API Gateway.
- Monitor Performance: Use AWS CloudWatch to monitor the performance and logs of your Lambda function.
FAQ Section
Common Questions About Setting Up Serverless Functions for Web Scraping
1. What is serverless architecture, and why should I use it for web scraping? Serverless architecture allows you to run code without managing servers. It’s ideal for web scraping because it scales automatically and reduces infrastructure management overhead.
2. How do I handle rate limits when web scraping with AWS Lambda? Use techniques like exponential backoff, rotating proxies or IP addresses, and respect the website’s robots.txt
file to manage rate limits effectively.
3. Can I use other programming languages for serverless functions besides Python? Yes, AWS Lambda supports multiple programming languages, including Node.js, Java, Go, and .NET Core. Choose the one that best fits your project requirements.
4. How can I ensure my web scraping code is efficient and reliable? Follow best practices such as handling rate limits, implementing robust error handling, respecting robots.txt
, and designing your script to handle multiple requests efficiently.
5. What should I do if the website blocks my IP after a few requests? If your IP gets blocked, consider using rotating proxies or different IP addresses. Ensure that you are respecting the website’s robots.txt
file and not making too many requests in a short period.