· Charlotte Will · webscraping · 6 min read
What is Headless Browser Web Scraping?
Discover how headless browser web scraping can enhance your data extraction efforts. Learn about its benefits, best practices, and practical examples in this comprehensive guide designed for both beginners and intermediate users.
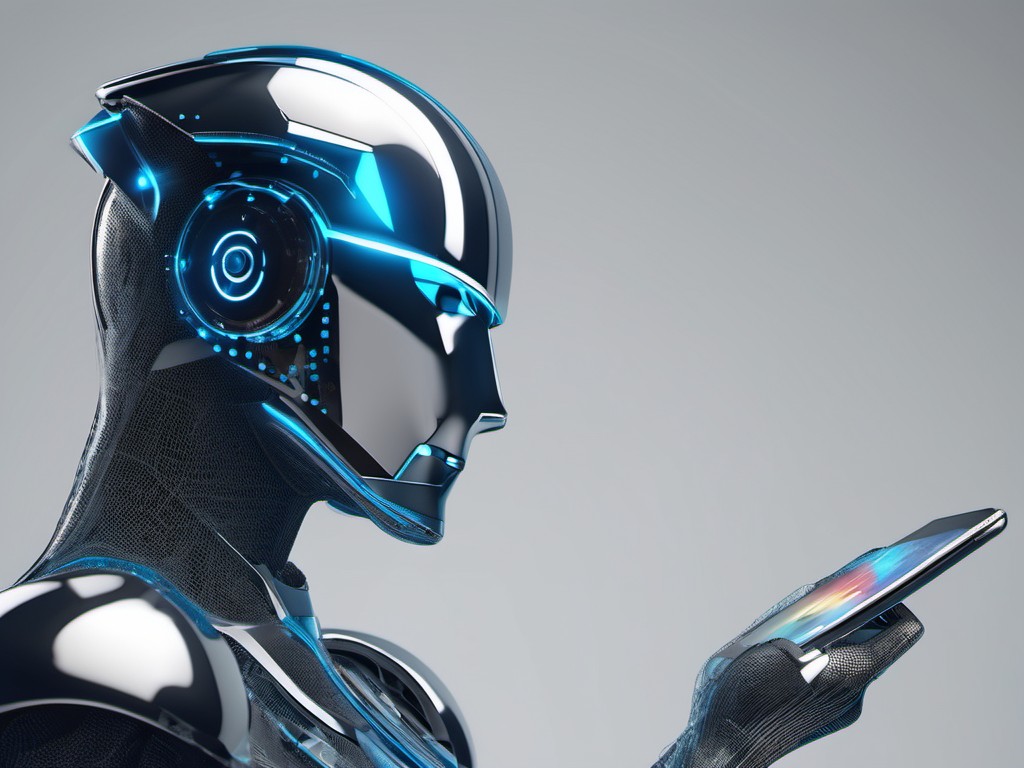
Introduction
Headless browser web scraping is a powerful technique that allows developers to extract data from websites without rendering the user interface. This approach offers numerous advantages, such as improved performance, reduced resource usage, and increased flexibility in automating web interactions. In this comprehensive guide, we will explore what headless browsers are, their benefits, best practices for implementing them, and practical examples to help you get started with headless browser web scraping.
Understanding Headless Browsers
Definition of Headless Browsers
A headless browser is a web browser without a graphical user interface (GUI). It operates in the background, allowing automation scripts to interact with web pages as if they were being viewed by a human user. This functionality makes headless browsers ideal for tasks such as automated testing, web scraping, and rendering JavaScript-heavy websites.
How They Differ from Traditional Browsers
Unlike traditional browsers like Google Chrome or Mozilla Firefox, headless browsers do not display a visual interface. Instead, they run in the background, making them faster and more resource-efficient. This difference is crucial for web scraping, where speed and performance are critical factors.
Popular Headless Browsers and Tools
Several tools and libraries support headless browser functionality:
- Puppeteer: A Node.js library developed by the Chrome team that provides a high-level API to control Chrome or Chromium over the DevTools Protocol.
- Selenium: A widely-used browser automation tool that supports multiple programming languages and can run in headless mode.
- Playwright: An end-to-end testing framework developed by Microsoft that supports various browsers, including headless modes for Chromium, Firefox, and WebKit.
Advantages of Headless Browser Web Scraping
Speed and Performance Benefits
Headless browsers operate without the overhead of rendering a user interface, making them significantly faster than traditional browsers. This speed advantage is particularly beneficial for web scraping tasks that involve processing large volumes of data or navigating through numerous web pages.
Resource Efficiency (CPU, Memory)
Because headless browsers do not require resources to render graphical elements, they consume less CPU and memory. This efficiency makes them more suitable for running on servers with limited resources, such as cloud instances, allowing you to handle more concurrent scraping sessions without incurring high costs.
Flexibility in Automating Web Interactions
Headless browsers can simulate user interactions like clicking buttons, filling out forms, and navigating through pages. This flexibility enables automation of complex workflows that would be challenging to achieve with traditional web scraping tools.
Handling JavaScript-Rendered Content
Many modern websites use JavaScript to dynamically load content as the user interacts with the page. Headless browsers can execute this JavaScript, allowing you to extract data that would otherwise be inaccessible using simple HTTP requests.
Best Practices for Headless Browser Web Scraping
Setting Up a Headless Browser Environment
Setting up a headless browser environment involves installing the necessary tools and dependencies. For example, to use Puppeteer with Node.js, you would need to run:
npm install puppeteer
Once installed, you can create a script to launch the headless browser:
const puppeteer = require('puppeteer');
(async () => {
const browser = await puppeteer.launch({ headless: true });
const page = await browser.newPage();
await page.goto('https://example.com');
// Perform scraping actions here
await browser.close();
})();
Configuring and Optimizing Performance Settings
To optimize performance, you can configure settings such as reducing the timeouts and adjusting resource usage limits:
const browser = await puppeteer.launch({
headless: true,
args: ['--no-sandbox', '--disable-setuid-sandbox'],
});
Handling Common Issues like CAPTCHAs and Rate Limits
Web scraping often involves dealing with anti-bot mechanisms like CAPTCHAs. Headless browsers can help bypass simple CAPTCHAs using techniques such as solving them programmatically or employing third-party services. To handle rate limits, consider implementing delays between requests and rotating proxies to distribute the load.
Implementing Error Handling and Retries
Error handling is crucial for maintaining the robustness of your scraping script. You can implement retries with exponential backoff to handle transient errors:
const maxRetries = 3;
for (let attempt = 0; attempt < maxRetries; attempt++) {
try {
await page.goto('https://example.com');
break; // Exit the loop if successful
} catch (error) {
console.error(`Attempt ${attempt + 1} failed:`, error);
await new Promise(resolve => setTimeout(resolve, Math.pow(2, attempt) * 1000));
}
}
Practical Examples and Use Cases
Scraping Dynamic Websites with JavaScript Rendering
Headless browsers excel at scraping dynamic websites that rely on JavaScript to render content. For instance, you can use Puppeteer to extract data from a website like this:
const puppeteer = require('puppeteer');
(async () => {
const browser = await puppeteer.launch({ headless: true });
const page = await browser.newPage();
await page.goto('https://example.com/dynamic-content');
const data = await page.$eval('#data', element => element.textContent);
console.log(data);
await browser.close();
})();
Extracting Data from Single-Page Applications (SPA)
Single-page applications pose challenges for traditional scraping methods due to their heavy reliance on JavaScript. With headless browsers, you can interact with these applications as if you were a user:
const puppeteer = require('puppeteer');
(async () => {
const browser = await puppeteer.launch({ headless: true });
const page = await browser.newPage();
await page.goto('https://example.com/spa-app');
// Interact with the SPA, e.g., click buttons, fill forms
await browser.close();
})();
Integrating Headless Browsers into Web Scraping Projects
Integrate headless browsers into your existing web scraping workflows to enhance data extraction capabilities. You can use libraries like Scrapy with middleware that supports Selenium or Puppeteer:
# settings.py
DOWNLOADER_MIDDLEWARES = {
'scrapy_selenium.SeleniumMiddleware': 800,
}
SELENIUM_DRIVER_NAME = 'firefox'
SELENIUM_DRIVER_EXECUTABLE_PATH = '/usr/local/bin/geckodriver'
SELENIUM_DRIVER_ARGUMENTS = ['-headless']
FAQ Section
What are the benefits of using headless browsers for scraping data?
Headless browsers offer several benefits, including improved performance, resource efficiency, flexibility in automating web interactions, and the ability to handle JavaScript-rendered content.
How do I set up a headless browser environment?
Setting up a headless browser environment involves installing tools like Puppeteer or Selenium and configuring them to run in headless mode. This can be done using command-line instructions or scripted configurations.
Can headless browsers handle CAPTCHAs?
While headless browsers can help bypass simple CAPTCHAs, more complex ones may require additional techniques like solving them programmatically or employing third-party services.
What is the best practice for handling rate limits when using headless browsers?
The best practices for handling rate limits include implementing delays between requests and rotating proxies to distribute the load, ensuring that your scraping activities remain within acceptable usage policies of the target websites.
How can I optimize the performance of headless browser web scraping?
To optimize performance, configure settings such as reducing timeouts and adjusting resource usage limits. Additionally, implement error handling and retries to manage transient errors effectively.
For more in-depth information on these topics, you can refer to the following articles: