· Charlotte Will · webscraping · 5 min read
How to Handle JavaScript Rendered Content in Python Web Scraping
Discover how to handle JavaScript rendered content in Python web scraping with practical tools and techniques. Optimize your scraping projects to extract dynamic data effectively.
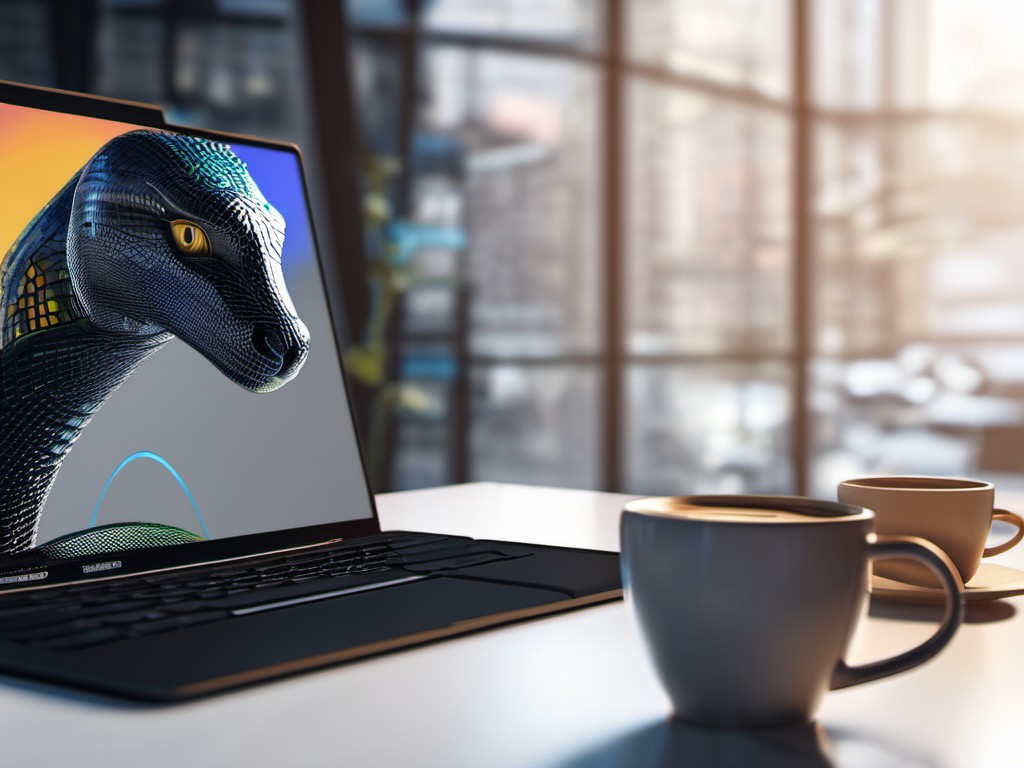
Introduction
Web scraping has become an essential skill for data extraction and analysis. However, modern websites often use JavaScript to render content dynamically, making it challenging to extract data using traditional web scraping techniques. In this article, we will explore how to handle JavaScript rendered content in Python web scraping effectively.
Understanding JavaScript Rendered Content
JavaScript rendered content refers to the dynamic loading of website elements after the initial HTML page has been loaded. This process is commonly used on websites to enhance user experience by delivering interactive and real-time updates without requiring a page reload. For web scrapers, this means that simply parsing the static HTML won’t be sufficient—you’ll need additional tools and techniques to extract the dynamic content.
Why Handle JavaScript Rendered Content?
Handling JavaScript rendered content is crucial for several reasons:
- Comprehensive Data Extraction: Dynamic websites often contain valuable data that is only available after JavaScript execution. Ignoring this content can lead to incomplete and potentially misleading datasets.
- Accurate Analysis: To perform accurate analysis, you need all relevant data. Handling dynamic content ensures that your analyses are based on complete information.
- Competitive Advantage: Many businesses rely on web scraping for competitive intelligence. Being able to extract dynamically rendered content gives you an edge over those who cannot.
Challenges in Handling JavaScript Rendered Content
Before diving into solutions, let’s understand the challenges associated with handling JavaScript rendered content:
- Dynamic Loading: The content changes as you interact with the page, making it difficult to scrape all necessary data in one go.
- AJAX Requests: Websites often use AJAX (Asynchronous JavaScript and XML) to fetch data from servers without reloading the page. This requires additional handling.
- JavaScript Execution: Traditional web scraping tools do not execute JavaScript, so you need specialized libraries or headless browsers that can run JavaScript.
Tools for Handling JavaScript Rendered Content
Selenium
Selenium is a powerful tool for automating browser interactions. It allows you to control a web browser via scripts and can execute JavaScript, making it ideal for scraping dynamic content.
from selenium import webdriver
# Set up the Selenium WebDriver
driver = webdriver.Chrome()
# Open the website
driver.get('https://example.com')
# Extract content after JavaScript execution
content = driver.page_source
# Close the browser
driver.quit()
BeautifulSoup with Selenium
BeautifulSoup is a popular library for parsing HTML and XML documents. When combined with Selenium, it can handle both static and dynamic content effectively.
from selenium import webdriver
from bs4 import BeautifulSoup
import time
# Set up the Selenium WebDriver
driver = webdriver.Chrome()
# Open the website
driver.get('https://example.com')
# Wait for JavaScript to render the content
time.sleep(5)
# Extract content using BeautifulSoup
soup = BeautifulSoup(driver.page_source, 'html.parser')
content = soup.find_all('div', class_='some-class')
# Close the browser
driver.quit()
Pyppeteer
Pyppeteer is a Python port of Puppeteer, which provides a high-level API to control headless Chrome or Chromium browsers. It’s particularly useful for handling complex JavaScript interactions.
import asyncio
from pyppeteer import launch
async def main():
# Launch the browser
browser = await launch()
page = await browser.newPage()
await page.goto('https://example.com')
# Extract content after JavaScript execution
content = await page.content()
# Close the browser
await browser.close()
# Run the async function
asyncio.get_event_loop().run_until_complete(main())
Scrapy with Splash
Scrapy is a popular web scraping framework, and when combined with Splash (a lightweight browser for JavaScript rendering), it can handle dynamic content seamlessly.
import scrapy
from scrapy_splash import SplashRequest
class MySpider(scrapy.Spider):
name = 'my_spider'
start_urls = ['https://example.com']
def start_requests(self):
for url in self.start_urls:
yield SplashRequest(url, self.parse, args={'wait': 5})
def parse(self, response):
# Extract content using BeautifulSoup or Scrapy selectors
content = response.css('div.some-class::text').getall()
Best Practices for Handling JavaScript Rendered Content
- Wait for Content to Load: Use appropriate wait times or conditions to ensure that the dynamic content is fully loaded before extracting it.
- Avoid Overhead: Minimize the use of headless browsers as they can be resource-intensive. Only use them when necessary.
- Respect Robots.txt and Terms of Service: Always check the website’s
robots.txt
file and terms of service to ensure you are not violating any rules. - Handle Rate Limits: Implement rate limits and delays in your scraping scripts to avoid overwhelming the server.
- Use Proxies: Rotate IP addresses using proxies to prevent getting blocked by the target website.
Internal Linking Section
When dealing with JavaScript rendered content, it’s important to understand how to handle AJAX requests and API rate limits, as covered in How to Handle AJAX Requests in Python Web Scraping and How to Handle API Rate Limits for Efficient Web Scraping with Python. Additionally, mastering cookies and sessions can greatly enhance your scraping capabilities, as discussed in How to Handle Cookies and Sessions in Python Web Scraping and How to Handle Cookie Consent Pop-Ups in Web Scraping Automation.
Conclusion
Handling JavaScript rendered content is crucial for comprehensive web scraping. By using tools like Selenium, BeautifulSoup, Pyppeteer, and Scrapy with Splash, you can effectively extract dynamic content from modern websites. Always remember to follow best practices and respect the target website’s rules and limitations.
FAQs
What is JavaScript rendered content? JavaScript rendered content refers to web page elements that are loaded or updated dynamically using JavaScript after the initial HTML page has been loaded.
Why is it important to handle JavaScript rendered content in web scraping? Handling JavaScript rendered content ensures that you extract complete and accurate data, which is essential for thorough analysis and competitive intelligence.
What tools can I use to handle JavaScript rendered content in Python? Common tools include Selenium, BeautifulSoup with Selenium, Pyppeteer, and Scrapy with Splash. Each tool has its strengths and can be used depending on the complexity of the dynamic content.
How do I wait for JavaScript rendered content to load? You can use explicit waits (e.g.,
time.sleep()
in Python) or implicit conditions (e.g., checking for specific elements) to ensure that the dynamic content is fully loaded before extracting it.What best practices should I follow when handling JavaScript rendered content? Best practices include waiting for content to load, minimizing resource usage, respecting website rules, handling rate limits, and using proxies to rotate IP addresses.