· Charlotte Will · webscraping · 4 min read
Scrapy Middleware: Enhancing Your Web Scraping Projects
Discover how Scrapy middleware can enhance your web scraping projects with practical advice on custom plugins, proxy management, error handling, and more. Learn to optimize your scraping tasks for better performance, reliability, and adaptability.
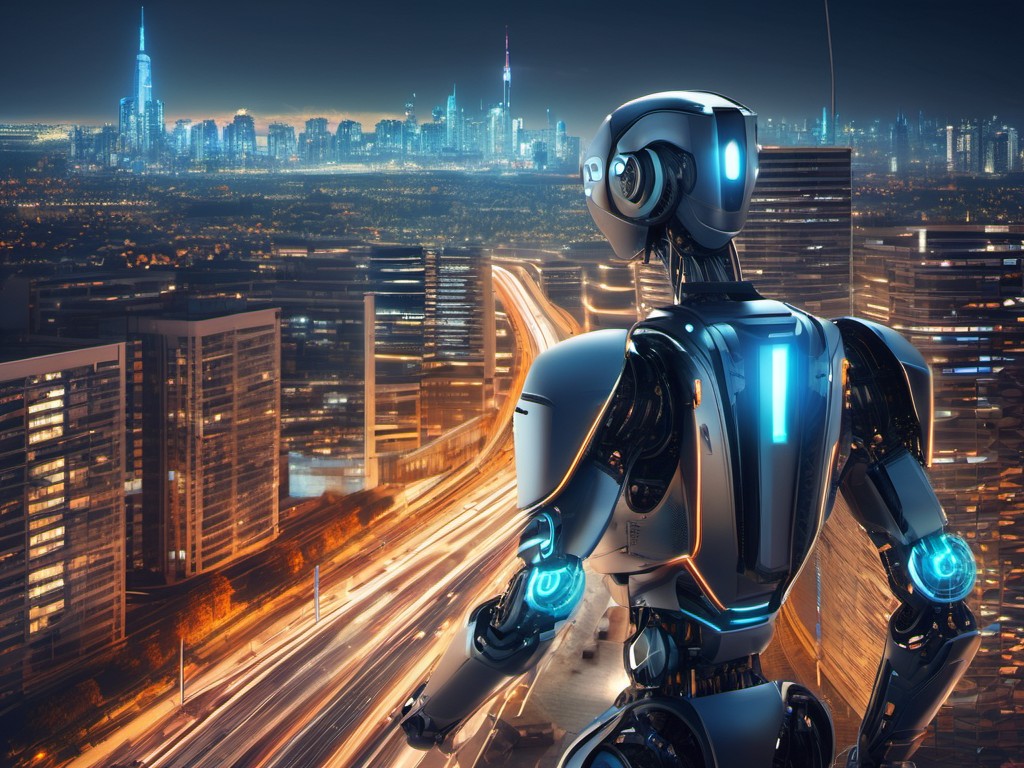
In the dynamic world of web scraping, efficiency, reliability, and adaptability are key. Scrapy, an open-source and collaborative web crawling framework for Python, is a powerful tool that enhances these aspects. One of its most potent features is middleware, which acts as custom plugins to process requests and responses. In this article, we’ll delve into Scrapy Middleware and how it can transform your web scraping projects.
Understanding Scrapy Middleware
Middleware in Scrapy operates between the core components—the engine, spiders, items, pipelines, and extensions. It allows you to intercept requests and responses, enabling custom processing that enhances functionality. This is particularly useful for tasks like managing proxies, handling errors, and rotating logs.
Scrapy Settings: The Foundation
Before diving into middleware, it’s essential to understand Scrapy settings. These configuration files define how your project behaves. You can enable or disable middlewares through the DOWNLOADER_MIDDLEWARES
and SPIDER_MIDDLEWARES
settings in settings.py
.
DOWNLOADER_MIDDLEWARES = {
'scrapy.downloadermiddlewares.useragent.UserAgentMiddleware': None,
'myproject.middlewares.CustomDownloaderMiddleware': 543,
}
SPIDER_MIDDLEWARES = {
'scrapy.spidermiddlewares.httperror.HttpErrorMiddleware': None,
'myproject.middlewares.CustomSpiderMiddleware': 543,
}
Types of Scrapy Middleware
Downloader Middleware
This middleware processes requests and responses before they reach the spiders or after leaving them. Common use cases include:
- Proxy management
- User-agent spoofing
- Request throttling
Custom Downloader Middleware Example:
class CustomDownloaderMiddleware:
def process_request(self, request, spider):
# Add your custom processing logic here
pass
def process_response(self, request, response, spider):
# Process the response before it reaches the spider
pass
Spider Middleware
This middleware operates at the spider level. It’s useful for tasks like:
- Error handling
- Log rotation
- Data extraction enhancements
Custom Spider Middleware Example:
class CustomSpiderMiddleware:
def process_spider_input(self, response, spider):
# Process the input before it reaches the spider
pass
def process_spider_output(self, response, result, spider):
# Process the output after it leaves the spider
pass
Practical Applications of Middleware
Proxy Management with Scrapy HTTP Proxies
Using middleware for proxy management can help bypass geo-restrictions and IP blocking. You can create a custom downloader middleware to rotate proxies automatically.
class ProxyMiddleware:
def process_request(self, request, spider):
request.meta['proxy'] = self.get_proxy()
def get_proxy(self):
# Fetch a proxy from your pool
pass
Error Handling with Middleware
Middleware can intercept HTTP errors and take appropriate actions, such as retrying or logging the error for further inspection.
class ErrorHandlingMiddleware:
def process_exception(self, request, exception, spider):
# Handle exceptions here
pass
Log Rotation in Scrapy
Log rotation helps manage disk space and ensures logs don’t grow indefinitely. You can configure log rotation using middleware and the logging
library.
import logging
from scrapy.utils.log import configure_logging
configure_logging({'LOG_FORMAT': '%(levelname)s: %(message)s', 'LOG_DATEFORMAT': '%Y-%m-%d %H:%M:%S'})
Advanced Topics in Scrapy Middleware
User-Agent Spoofing
Rotating user agents helps mimic human behavior and reduces the risk of getting blocked. You can use middleware to achieve this.
class UserAgentMiddleware:
def process_request(self, request, spider):
request.headers['User-Agent'] = self.get_user_agent()
def get_user_agent(self):
# Return a random user agent from your pool
pass
Request and Response Processing
Middleware allows you to modify requests and responses. This can be useful for adding headers, cookies, or even stripping unwanted data.
class RequestResponseMiddleware:
def process_request(self, request, spider):
# Modify the request here
pass
def process_response(self, request, response, spider):
# Modify the response here
pass
Optimizing Scrapy Projects with Middleware
Performance Tuning
Middleware can help optimize performance by managing download delays and concurrent requests.
Data Extraction Enhancements
By intercepting responses, you can pre-process data to extract only what’s necessary, reducing the load on your pipelines.
Custom Middleware Development
For those looking to delve deeper into custom middleware, our article on Scrapy Middleware: Enhancing Web Scraping Projects with Custom Plugins provides a comprehensive guide. Additionally, understanding the basics of web scraping can be crucial before diving into advanced topics like middleware. Check out our What is Scrapy Web Scraping Tutorial? for foundational knowledge.
Conclusion
Scrapy middleware is a powerful tool that can significantly enhance your web scraping projects. Whether you’re managing proxies, handling errors, or optimizing performance, middleware provides the flexibility to tailor Scrapy to your specific needs. By understanding and leveraging this feature, you can create more efficient, reliable, and adaptable web scraping solutions.
FAQs
What is the difference between downloader middleware and spider middleware? Downloader middleware processes requests and responses, while spider middleware operates at the spider level, handling inputs and outputs.
How do I enable custom middleware in Scrapy? You can enable custom middleware by adding it to the
DOWNLOADER_MIDDLEWARES
orSPIDER_MIDDLEWARES
settings in your project’ssettings.py
.Can I use middleware for user-agent spoofing? Yes, middleware can be used to rotate user agents and mimic human behavior.
What are some practical uses of Scrapy middleware? Middleware is useful for tasks like proxy management, error handling, log rotation, data extraction enhancements, and performance tuning.
How can I develop custom middleware for my Scrapy project? You can develop custom middleware by creating a new Python class that inherits from
scrapy.middlewares.base.BaseMiddleware
or the appropriate middleware base class, and then implementing the required methods likeprocess_request
,process_response
, etc.