· Charlotte Will · webscraping · 5 min read
How to Use Headless Browsers for Web Scraping
Discover how to use headless browsers like Puppeteer and Selenium for effective web scraping, including practical tips and best practices for handling dynamic content, CAPTCHAs, and more. Optimize your data extraction workflows and stay ahead of the competition with our comprehensive guide.
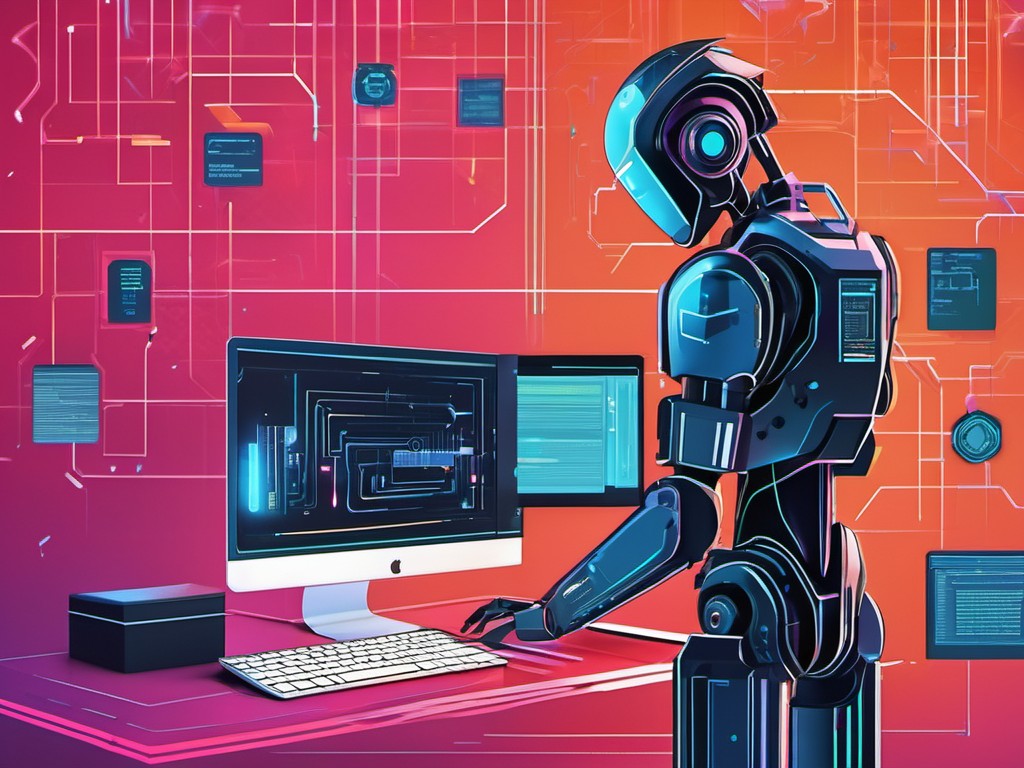
Web scraping has become an essential tool for extracting data from websites, enabling businesses to gather valuable insights and stay competitive. Traditional web scraping methods often use tools like BeautifulSoup and Requests, which work well with static content. However, as websites evolve to include more dynamic elements such as JavaScript, these traditional methods can fall short. Enter headless browsers—they offer a powerful solution for scraping modern, interactive web pages.
What is a Headless Browser?
A headless browser is essentially a web browser without a graphical user interface (GUI). It operates in the background and executes JavaScript code, rendering web pages similarly to how a standard browser like Chrome or Firefox would. This makes it an ideal tool for scraping dynamic content. Headless browsers are particularly useful for tasks that require interaction with web elements, such as filling out forms, clicking buttons, and navigating through multiple pages.
Popular Headless Browsers
Several headless browsers are available, each with its own set of features and advantages:
- Puppeteer: Developed by the Chrome team, Puppeteer is a Node.js library that provides a high-level API to control headless Chrome or Chromium over the DevTools Protocol.
- Selenium: While not strictly a headless browser, Selenium offers capabilities for running browsers in headless mode. It supports multiple browsers and languages like Python, Java, C#, etc.
- Playwright: Developed by Microsoft, Playwright is a Node.js library that allows you to automate Chromium, Firefox, and WebKit with a single API.
- PhantomJS: An older option, PhantomJS is a headless WebKit scriptable with a JavaScript API. It’s less commonly used today due to the advent of more modern tools like Puppeteer and Playwright.
Setting Up Headless Browsers
Puppeteer Setup
To get started with Puppeteer, you need Node.js installed on your machine. Then, install Puppeteer using npm:
npm install puppeteer
You can now write scripts to control a headless browser instance. Here’s a basic example:
const puppeteer = require('puppeteer');
(async () => {
const browser = await puppeteer.launch();
const page = await browser.newPage();
await page.goto('https://example.com');
const content = await page.content();
console.log(content);
await browser.close();
})();
Selenium Setup
For Selenium, you need to install the WebDriver and a browser driver like ChromeDriver:
pip install selenium
Download the appropriate driver for your browser from the official website. Here’s an example script in Python:
from selenium import webdriver
from selenium.webdriver.chrome.service import Service
from selenium.webdriver.chrome.options import Options
chrome_options = Options()
chrome_options.add_argument("--headless")
driver = webdriver.Chrome(service=Service('/path/to/chromedriver'), options=chrome_options)
driver.get('https://example.com')
content = driver.page_source
print(content)
driver.quit()
Playwright Setup
To use Playwright, install it via npm:
npm install playwright
Here’s a simple script to get you started:
const { chromium } = require('playwright');
(async () => {
const browser = await chromium.launch();
const page = await browser.newPage();
await page.goto('https://example.com');
const content = await page.content();
console.log(content);
await browser.close();
})();
Best Practices for Web Scraping with Headless Browsers
Respect Robots.txt and Terms of Service
Always check the robots.txt
file of the website you intend to scrape. This file outlines which parts of the site can be crawled and indexed by bots. Additionally, review the website’s terms of service to ensure your actions comply with their policies.
Use Proxies and Rotating User Agents
To avoid getting blocked, use proxies and rotate user agents frequently. This makes it seem like your requests are coming from different users and locations, reducing the risk of being detected as a bot.
Handle CAPTCHAs
Dynamic websites often employ CAPTCHA challenges to prevent automated access. While headless browsers can’t solve CAPTCHAs directly, you can use third-party services like 2Captcha or Anti-Captcha to handle them.
Optimize Resource Usage
Headless browsers can consume significant system resources, especially when handling multiple tabs and pages simultaneously. Optimize your scripts to close pages and free up memory as soon as they’re no longer needed.
Advanced Techniques in Headless Browser Web Scraping
Interacting with Forms
Headless browsers excel at interacting with forms, allowing you to fill out fields and submit data programmatically. Here’s an example using Puppeteer:
const puppeteer = require('puppeteer');
(async () => {
const browser = await puppeteer.launch();
const page = await browser.newPage();
await page.goto('https://example.com/form-page');
// Fill out the form
await page.type('#name', 'John Doe');
await page.type('#email', '[email protected]');
await page.click('#submit');
const content = await page.content();
console.log(content);
await browser.close();
})();
Handling AJAX Requests
Websites using AJAX to load data dynamically can be challenging to scrape with traditional methods. Headless browsers, however, render JavaScript and handle AJAX requests natively:
from selenium import webdriver
from selenium.webdriver.chrome.service import Service
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
chrome_options = Options()
chrome_options.add_argument("--headless")
driver = webdriver.Chrome(service=Service('/path/to/chromedriver'), options=chrome_options)
driver.get('https://example.com')
# Wait for AJAX content to load
WebDriverWait(driver, 10).until(EC.presence_of_element_located((By.ID, 'ajax-content')))
content = driver.find_element(By.ID, 'ajax-content').text
print(content)
driver.quit()
Scraping Infinite Scroll Pages
Pages with infinite scrolling can also be effectively scraped using headless browsers:
const puppeteer = require('puppeteer');
(async () => {
const browser = await puppeteer.launch();
const page = await browser.newPage();
await page.goto('https://example.com/infinite-scroll-page');
// Scroll down to load more content
for (let i = 0; i < 10; i++) {
await page.evaluate(() => window.scrollBy(0, window.innerHeight));
await new Promise(resolve => setTimeout(resolve, 2000)); // Wait for content to load
}
const content = await page.content();
console.log(content);
await browser.close();
})();
Conclusion
Headless browsers have revolutionized web scraping by enabling the extraction of dynamic content that was previously inaccessible to traditional methods. By using tools like Puppeteer, Selenium, and Playwright, you can automate complex interactions with websites, extract valuable data efficiently, and stay compliant with legal and ethical standards.
FAQs
Why use headless browsers for web scraping? Headless browsers render JavaScript and handle dynamic content, making them ideal for modern web scraping tasks that involve interactivity.
Which headless browser should I choose? The choice depends on your specific needs and familiarity with the tool. Puppeteer is great for Chrome/Chromium, while Selenium supports multiple browsers. Playwright offers a unified API for Chromium, Firefox, and WebKit.
How do I handle CAPTCHAs in headless browsers? Use third-party services like 2Captcha or Anti-Captcha to solve CAPTCHA challenges programmatically.
Can headless browsers be used for automated testing? Yes, headless browsers are frequently used for automated testing as they can simulate user interactions and render pages similarly to a standard browser.
What are some common pitfalls when using headless browsers? Common issues include getting blocked by websites, handling CAPTCHAs, optimizing resource usage, and dealing with complex dynamic content. Following best practices can help mitigate these challenges.