· Charlotte Will · 12 min read
How to Use Python for Web Scraping: Step-by-Step Tutorial
Learn how to use Python for web scraping with this step-by-step tutorial. Master tools like Beautiful Soup, requests, and Selenium to extract data efficiently while adhering to ethical guidelines.
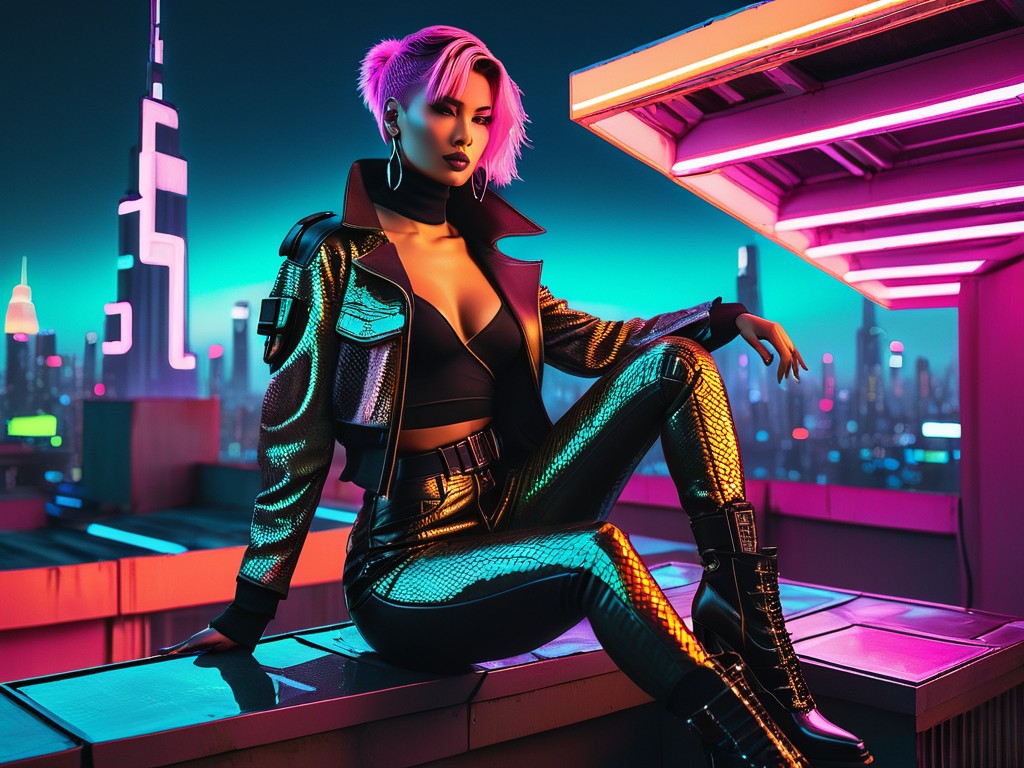
Web scraping is a powerful tool that allows you to automate the process of extracting data from websites. Whether you’re gathering market research, analyzing trends, or just curious about how data is structured online, Python makes it incredibly easy to get started. In this step-by-step tutorial, we’ll walk you through everything from setting up your environment to advanced techniques like handling cookies and using proxies. You’ll learn how to use popular libraries such as Beautiful Soup, requests
, and Selenium to scrape data effectively. By the end of this tutorial, you’ll have a solid understanding of how to use Python for web scraping and be ready to tackle your own projects. So, let’s dive into the world of web scraping and unlock the power of data extraction!
Getting Started with Web Scraping in Python
Setting Up Your Environment
Before we dive into the technical details, let’s make sure you have everything set up to start web scraping with Python. This section will guide you through setting up your environment and installing the necessary libraries.
To get started, you’ll need to have Python installed on your system. If you haven’t already, head over to the official Python website and download the latest version. Once installed, you can verify your installation by running python --version
in your command line or terminal.
Next, you’ll want to set up a virtual environment. This will keep your dependencies organized and prevent conflicts with other projects.
python -m venv mywebscrapingproject
source mywebscrapingproject/bin/activate # On Windows, use `mywebscrapingproject\Scripts\activate`
Now that you have a virtual environment, it’s time to install the libraries you’ll need for web scraping. The most popular ones are Beautiful Soup, requests
, and Selenium
.
pip install beautifulsoup4 requests selenium
To ensure everything is working, you can create a simple script to test your setup. Here’s an example using Beautiful Soup:
import requests
from bs4 import BeautifulSoup
url = "https://example.com"
response = requests.get(url)
soup = BeautifulSoup(response.content, 'html.parser')
print(soup.title.string)
This script sends an HTTP request to the provided URL, parses the HTML content using Beautiful Soup, and prints out the title of the page. If you see the title printed in your terminal, congratulations! You’re ready to start web scraping.
For a more detailed guide on using Beautiful Soup for web scraping, check out How to Use BeautifulSoup for Web Scraping with Python.
Understanding the Basics of Web Scraping
To be effective at web scraping, it’s important to understand both the HTML structure of web pages and how to interact with websites programmatically.
What is HTML?
HTML, or Hypertext Markup Language, is the standard markup language for creating web pages. Understanding HTML tags and elements will help you navigate and extract data effectively.
For example, let’s say you want to scrape product information from an e-commerce site. The products are likely listed within div
elements, each containing various details like the product name, price, and image URL. You can use Beautiful Soup to find these elements by their tags or attributes.
# Example: Finding product divs
products = soup.find_all('div', class_='product')
for product in products:
name = product.find('h2').text
price = product.find('span', class_='price').text
print(f"Name: {name}, Price: {price}")
This script finds all div
elements with the class “product”, then extracts and prints the product name and price. Understanding how to navigate HTML structures is crucial for effective web scraping.
If you need more insights into parsing HTML with Beautiful Soup, check out What is Python Web Scraping and How to Use BeautifulSoup.
Sending HTTP Requests
To interact with websites, you’ll often need to send HTTP requests. The requests
library in Python makes this easy.
import requests
url = "https://example.com"
response = requests.get(url)
if response.status_code == 200:
print("Success!")
else:
print("Failed to retrieve data.")
This script sends a GET request to the specified URL and checks if the response status code is 200, indicating a successful request. If you encounter issues like rate limiting or blocked requests, consider using proxies to maintain anonymity.
For more advanced techniques on handling HTTP requests, such as interacting with APIs, see A Step-by-Step Guide to Making API Calls for Efficient Web Scraping.
Parsing HTML Content
Now that you’ve got a basic understanding of setting up your environment and sending HTTP requests, let’s dive into parsing HTML content with Beautiful Soup.
Introduction to Beautiful Soup
Beautiful Soup is a Python library that makes it easy to pull data out of HTML and XML files. You can install it using pip, as mentioned earlier.
from bs4 import BeautifulSoup
# Example: Parsing HTML content
url = "https://example.com"
response = requests.get(url)
soup = BeautifulSoup(response.content, 'html.parser')
Beautiful Soup provides various methods to navigate and search the parse tree. For instance, you can use find()
or find_all()
to locate elements based on their tags or attributes.
# Example: Finding all paragraphs
paragraphs = soup.find_all('p')
for p in paragraphs:
print(p.text)
This script finds all <p>
elements and prints their text. You can also use CSS selectors to find elements, which can be more powerful for complex HTML structures.
If you want a deeper dive into using Beautiful Soup, check out How to Use BeautifulSoup for Web Scraping with Python.
Extracting Data
Once you’ve parsed the HTML content, extracting specific data becomes straightforward. Let’s say you want to scrape a list of articles from a news site.
# Example: Extracting article titles and links
articles = soup.find_all('article')
for article in articles:
title = article.find('h1').text
link = article.find('a')['href']
print(f"Title: {title}, Link: {link}")
This script finds all article
elements, extracts the title and link from each one, and prints them. By understanding how to extract data effectively, you can gather valuable information from any website.
For more advanced techniques on extracting and saving scraped data, see How to Use Python for Automated Data Collection with Web Scraping.
Advanced Techniques in Web Scraping
Handling Cookies and Sessions
Web scraping often involves managing cookies and sessions to mimic human interactions. You can use the requests
library to handle these seamlessly.
import requests
session = requests.Session()
response = session.get(url)
# Handling cookies
cookies = response.cookies
print(cookies)
For more advanced session management, see How to Automate Web Scraping with Python and AsyncIO.
Using Proxies for Anonymity
To avoid detection by websites, you can use proxies. This is especially useful in large-scale scraping projects.
proxies = {
'http': 'http://10.10.1.10:3128',
'https': 'http://10.10.1.10:1080',
}
response = requests.get(url, proxies=proxies)
For more details on using proxies effectively, see How to Use Proxies for Large-Scale Web Scraping Projects.
Parsing Data from APIs
Web scraping isn’t just about HTML. Many websites offer APIs that can provide structured data directly.
import requests
api_url = "https://api.example.com/data"
response = requests.get(api_url)
data = response.json()
print(data)
For more on making efficient API calls, see A Step-by-Step Guide to Making API Calls for Efficient Web Scraping.
Ethical and Legal Considerations
Understanding Terms of Service
Always check a website’s terms of service and robots.txt file to ensure you’re not violating any rules. Respect privacy policies and avoid scraping personal data without consent.
For more detailed guidance on legal boundaries, see Ethical and Legal Considerations in Web Scraping.
Protecting Personal Data
Privacy concerns are paramount in web scraping. Always ensure you’re handling personal data responsibly and legally.
For more on protecting personal data, see Ethical Considerations in Web Scraping.
Troubleshooting Common Issues
Handling Errors
Common errors in web scraping include connection timeouts, HTTP status codes, and parsing issues. Debugging techniques can help resolve these.
try:
response = requests.get(url)
except requests.RequestException as e:
print(f"Error: {e}")
For more on handling errors, see Troubleshooting Web Scraping Errors.
Optimizing Performance
Optimizing performance is crucial for efficient web scraping. Techniques include parallel processing, using headless browsers, and rate limiting.
For more on performance optimization, see Optimizing Web Scraping Performance.
Best Practices
Rotating IP Addresses
Rotating IP addresses helps avoid detection by websites. Use services like proxies to manage this effectively.
For more on rotating IP addresses, see How to Use Proxies for Large-Scale Web Scraping Projects.
Respecting Website Robots.txt
Always check and respect the robots.txt
file of a website to avoid scraping disallowed content.
For more on respecting robots.txt
, see Respecting Robots.txt in Web Scraping.
Rate Limiting
Rate limiting helps you scrape websites without overwhelming their servers. Use techniques to control the frequency of requests.
For more on rate limiting, see Rate Limiting in Web Scraping.
Real-world Applications
Data Analysis
Web scraping can provide valuable data for analysis. For instance, tracking market trends or sentiment analysis of social media posts.
For more on data analysis applications, see Data Analysis with Web Scraping.
Market Research
E-commerce companies often scrape competitor pricing to adjust their own prices dynamically. This can provide a competitive edge.
For more on market research, see Market Research with Web Scraping.
Automated Data Collection
Automating data collection can save time and effort. Integrate web scraping with other tools for more efficient workflows.
For more on automated data collection, see Automated Data Collection with Web Scraping.
Resources and Tools
Documentation Links
Official documentation for libraries like Beautiful Soup, requests
, and Selenium
can provide essential guidance.
For more on documentation, see Official Documentation Links.
Recommended Books and Courses
Books and courses can provide in-depth knowledge on web scraping techniques.
For more recommendations, see Recommended Books and Courses.
Community Forums and Support
Participate in forums to get help, share insights, and contribute to the web scraping community.
For more on community support, see Community Forums for Web Scraping.
Quick Takeaways
- Environment Setup: Ensure Python and necessary libraries like Beautiful Soup,
requests
, andSelenium
are installed in a virtual environment to avoid dependency conflicts. - HTML Basics: Understanding HTML tags and structure is crucial for navigating and extracting data effectively from web pages.
- HTTP Requests: Use the
requests
library to send HTTP requests and handle responses, checking status codes for successful retrieval. - Beautiful Soup: Master the basics of Beautiful Soup to parse and navigate HTML content, using methods like
find()
andfind_all()
. - Data Extraction: Extract specific data elements using tags, classes, or CSS selectors and save the results to a file for further analysis.
- Advanced Techniques: Incorporate advanced techniques such as handling cookies, using proxies, and parsing data from APIs for more sophisticated scraping tasks.
- Ethical Considerations: Always respect website terms of service, legal boundaries, and privacy concerns when web scraping to avoid potential issues.
These key points summarize the essential steps and best practices for using Python for web scraping, ensuring you have a solid foundation to start your projects.
Conclusion
In this tutorial, we’ve covered a comprehensive range of topics to help you get started with web scraping using Python. From setting up your environment and understanding HTML basics to advanced techniques like handling cookies and proxies, you now have the tools to extract valuable data from websites efficiently.
Whether you’re a software engineer, software developer, or project manager, mastering web scraping can significantly enhance your data collection and analysis capabilities. Web scraping allows you to automate repetitive tasks, gather market intelligence, or create rich datasets for data science projects. By leveraging libraries like Beautiful Soup and Selenium
, you can unlock a world of possibilities in data extraction.
Remember to always adhere to ethical guidelines and legal considerations when web scraping. Respect website terms of service, use proxies judiciously to avoid detection, and handle personal data responsibly.
If you want to dive deeper into specific areas like making API calls or using headless browsers, be sure to check out other articles in our series:
- How to Use Python for Automated Data Collection with Web Scraping
- How to Automate Web Scraping with Python and Selenium
Start experimenting with Python and web scraping today, and you’ll be well on your way to becoming proficient in this valuable skill. Happy coding!
FAQs
What are the legal risks of web scraping?
- Web scraping comes with several legal considerations. Always check a website’s terms of service and robots.txt file to ensure you’re not violating any rules. Common legal issues include copyright infringement, breach of contract, and unauthorized access. To stay compliant, respect privacy policies and avoid scraping personal data without consent. For more detailed guidance on legal boundaries, see Ethical and Legal Considerations in Web Scraping.
How can I avoid getting blocked by websites while scraping?
- To avoid detection and blocking, use techniques like rotating proxies, setting user-agent headers to mimic human browsers, and implementing rate limiting. Rotate your IP addresses periodically using services like How to Use Proxies for Large-Scale Web Scraping Projects. Additionally, spread out your requests over time to mimic human behavior and reduce the likelihood of triggering automated detection systems.
What are some popular use cases for web scraping?
- Web scraping is widely used in various applications, including market research, price tracking, sentiment analysis, and data aggregation for AI models. For instance, e-commerce companies scrape competitor pricing to adjust their own prices dynamically. Data analysts use web scraping for sentiment analysis of social media posts or customer reviews to gauge public opinion on products. Detailed examples can be found in Real-world Applications of Web Scraping.
Are there any free proxies available for web scraping?
- Yes, some free proxy services are available online, but they can be unreliable and may lack the features needed for large-scale scraping projects. For more robust solutions, consider using paid proxy services that offer higher reliability and better performance. Explore options like How to Use Proxies for Large-Scale Web Scraping Projects for more information on selecting and managing proxies effectively.
How can I parse complex HTML structures effectively?
- Parsing complex HTML structures requires a good understanding of CSS selectors and DOM traversal methods. Use Beautiful Soup’s advanced selection techniques like
select()
to target elements with specific attributes or classes. For more complex scenarios, consider using headless browsers likeSelenium
to handle JavaScript-rendered content dynamically. Learn more about advanced parsing techniques in How to Automate Web Scraping with Python and Selenium.
- Parsing complex HTML structures requires a good understanding of CSS selectors and DOM traversal methods. Use Beautiful Soup’s advanced selection techniques like
Your Feedback Matters!
We hope this tutorial has provided you with valuable insights into using Python for web scraping. Your feedback is incredibly important to us! Please take a moment to share your thoughts, experiences, or any additional tips you have on the topic. Your input helps us improve and create more useful content for our community.
Also, if you found this article helpful, we would greatly appreciate it if you could share it on your social media platforms. Your shares help us reach more readers who might benefit from these tips.
Lastly, we’d love to hear from you! Have you encountered any specific challenges while web scraping? What projects are you working on that could benefit from these techniques? Share your stories and questions in the comments below!
Thank you for reading, and we look forward to hearing from you!