· Charlotte Will · 5 min read
A Step-by-Step Guide to Making API Calls for Efficient Web Scraping
Learn how to make efficient API calls for web scraping with this step-by-step guide. Discover best practices, error handling, and common mistakes to avoid. Optimize your data extraction process today!
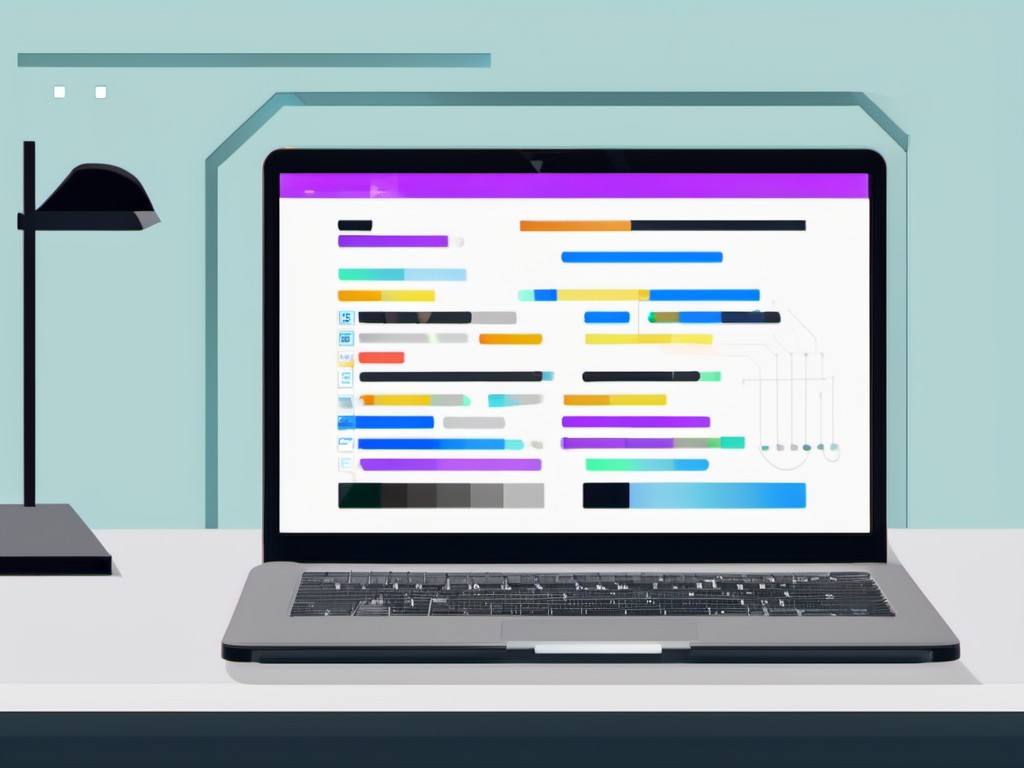
Welcome to your comprehensive guide on making API calls for efficient web scraping! If you’re new to web scraping or looking to enhance your existing skills, this guide is tailored just for you. Let’s dive into the world of APIs and see how they can streamline your web scraping processes.
Introduction to Web Scraping and APIs
Web scraping is the process of extracting data from websites. While traditional methods involve manually copying data or using automated tools, making API calls can be a more efficient alternative. APIs (Application Programming Interfaces) allow different software applications to communicate with each other, enabling seamless data extraction without the need for manual intervention.
Why Use API Calls for Web Scraping?
Using APIs for web scraping offers several advantages:
- Efficiency: APIs provide structured data that is easier and faster to process compared to manually scraped content.
- Legal Compliance: Many websites offer public APIs, which means you’re less likely to run into legal issues compared to traditional scraping methods.
- Reliability: API calls are more reliable as the data structure is consistent, reducing the risk of errors.
Setting Up Your Environment
Before diving into making API calls, let’s set up your environment. You’ll need a few tools and languages to get started:
Tools Needed
- Programming Language: Python is widely used for web scraping due to its simplicity and powerful libraries like
requests
andBeautifulSoup
. - API Client Library: Libraries such as
requests
in Python make it easy to send HTTP requests to APIs. - Integrated Development Environment (IDE): Tools like VS Code or PyCharm can enhance your coding experience.
- API Documentation: Always keep the API documentation of the service you’re using handy.
Step-by-Step Setup Guide
- Install Python: Download and install Python from python.org.
- Set Up a Virtual Environment: This helps in managing dependencies for your project.
python -m venv myenv source myenv/bin/activate # On Windows, use `myenv\Scripts\activate`
- Install Required Libraries:
pip install requests
Making API Calls: A Practical Guide
Now that your environment is set up, let’s make our first API call. We’ll use the GitHub API as an example to fetch user data.
Sending a GET Request
Import Libraries:
import requests
Send a GET Request:
response = requests.get('https://api.github.com/users/octocat') data = response.json() print(data)
Handling API Response
Handling the response correctly is crucial. Here’s how you can handle different HTTP status codes:
if response.status_code == 200:
data = response.json()
print("Success:", data)
elif response.status_code == 404:
print("Error:", response.status_code, "User not found")
else:
print("Error:", response.status_code, response.text)
Working with Headers and Parameters
APIs often require headers or parameters for authentication and filtering data:
headers = {
'Authorization': 'token YOUR_ACCESS_TOKEN',
'Accept': 'application/vnd.github.v3+json'
}
params = {
'since': '2015-01-01'
}
response = requests.get('https://api.github.com/search/repositories', headers=headers, params=params)
data = response.json()
print(data)
Best Practices for Efficient Web Scraping with APIs
Rate Limiting and Throttling
APIs often have rate limits to prevent abuse. Be sure to respect these limits:
- Use headers like
Retry-After
to understand when you can make the next request. - Implement exponential backoff strategies for retries.
Error Handling
Implement robust error handling to manage network issues, API changes, or unexpected responses:
try:
response = requests.get('https://api.github.com/users/octocat')
data = response.json()
except requests.exceptions.RequestException as e:
print("An error occurred:", e)
Caching Responses
Caching responses can reduce the number of API calls and improve efficiency:
import time
from datetime import datetime, timedelta
cache = {}
def fetch_data(url):
if url in cache and datetime.now() - cache[url]['timestamp'] < timedelta(minutes=5):
return cache[url]['data']
else:
response = requests.get(url)
data = response.json()
cache[url] = {'data': data, 'timestamp': datetime.now()}
return data
Documentation and Version Control
Always keep your code documented and use version control systems like Git to track changes:
def main():
"""
Main function to fetch user data from GitHub API.
"""
url = 'https://api.github.com/users/octocat'
data = fetch_data(url)
print(data)
if __name__ == "__main__":
main()
Common Mistakes to Avoid
Ignoring Rate Limits
Ignoring rate limits can lead to your API key being blocked or throttled. Always check and respect the rate limits of the APIs you are using.
Not Handling Errors Properly
Not handling errors properly can lead to unexpected behavior in your application. Implement comprehensive error handling to manage various edge cases.
Neglecting Security
Never hardcode API keys or sensitive information in your code. Use environment variables or secure vaults to store and access this data safely.
Conclusion
Making API calls for web scraping can significantly enhance the efficiency and reliability of your data extraction processes. By following best practices and using the right tools, you can harness the power of APIs to streamline your workflow. Whether you’re a beginner or an experienced developer, integrating APIs into your web scraping projects is a valuable skill that will pay dividends in the long run.
FAQs
1. What are some popular public APIs for web scraping?
Some popular public APIs include GitHub API, Twitter API, Reddit API, and OpenWeatherMap API. Always check the documentation of these services to understand their usage policies.
2. How do I handle paginated responses from an API?
To handle paginated responses, you can loop through the pages by following the “next” or “prev” links provided in the API response headers or body. Implementing a while-loop based on these links is a common approach.
3. What should I do if an API doesn’t provide all the data I need?
If an API does not provide all the necessary data, you might need to combine it with traditional web scraping techniques or use additional APIs that complement each other. Always ensure that your methods comply with the terms of service of the websites you are working with.
4. How can I monitor and optimize my API usage?
Monitor your API usage by logging requests, responses, and errors. Use tools like Prometheus or Grafana to visualize this data and identify bottlenecks. Optimizing your code and implementing caching strategies can also help in reducing unnecessary API calls.
5. What are some alternatives to using APIs for web scraping?
Alternatives to using APIs include traditional web scraping techniques with tools like BeautifulSoup or Scrapy, headless browsers like Puppeteer or Selenium, and cloud-based web scraping services that offer API access. Each method has its pros and cons, so choose the one that best fits your needs.