· Charlotte Will · webscraping · 5 min read
How to Use BeautifulSoup for Web Scraping with Python
Discover how to use BeautifulSoup for web scraping with Python in this comprehensive guide. Learn step-by-step techniques, best practices, and ethical considerations for extracting data from websites efficiently. Perfect for both beginners and experienced coders looking to master Python web scraping.
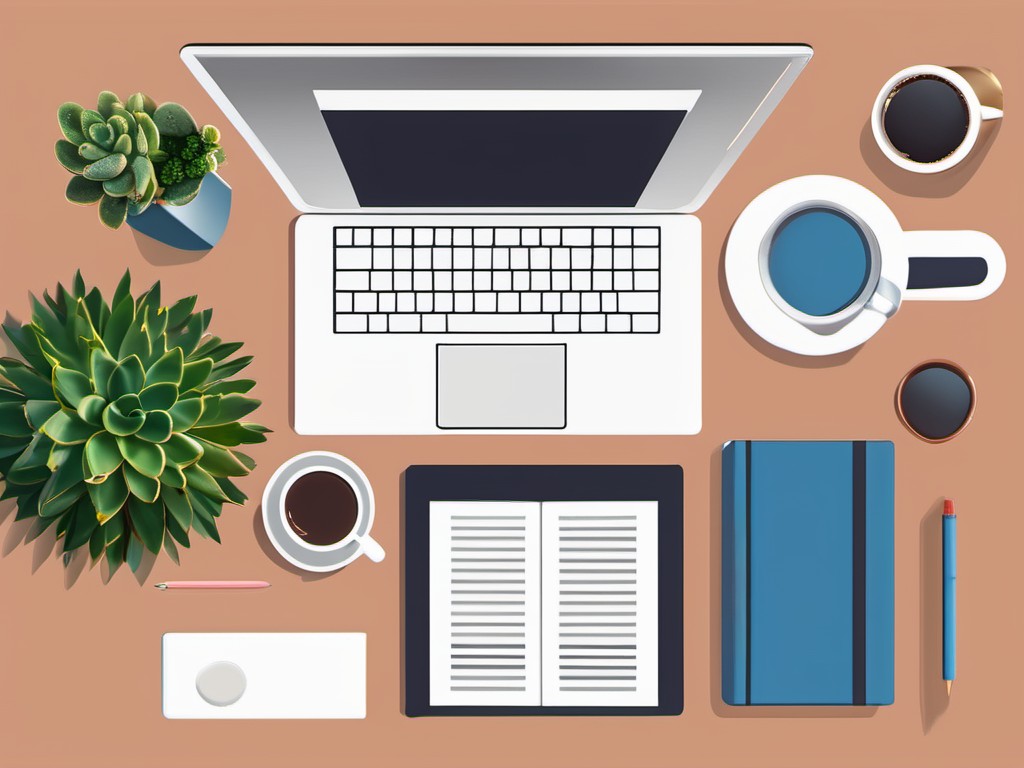
Are you looking to extract data from websites but don’t know where to start? Look no further than this comprehensive guide on how to use BeautifulSoup for web scraping with Python. Whether you’re a beginner or have some coding experience, we’ll walk you through the process step-by-step, ensuring you leave as an expert in Python web scraping!
Introduction to Web Scraping and BeautifulSoup
Web scraping is the automated extraction of data from websites. It’s a powerful technique for gathering information that might otherwise be time-consuming or impossible to collect manually. Python, with its rich set of libraries, makes web scraping straightforward and efficient. One such library is BeautifulSoup, designed for parsing HTML and XML documents.
Why Choose BeautifulSoup?
BeautifulSoup stands out among other web scraping tools due to several reasons:
- Ease of Use: BeautifulSoup provides a simple, Pythonic way to navigate, search, and modify the parse tree.
- Robustness: It handles even poorly designed HTML gracefully.
- Flexibility: Compatible with various parsers (e.g., lxml, html5lib) for different needs.
Setting Up Your Environment
Before diving into coding, let’s set up our environment:
- Install Python: Ensure you have the latest version of Python installed on your machine.
- Install BeautifulSoup and requests libraries: Open your terminal or command prompt and type:
pip install beautifulsoup4 requests
Your First Web Scraping Project
Step 1: Import Libraries
Start by importing the necessary libraries in your Python script.
import requests
from bs4 import BeautifulSoup
Step 2: Make an HTTP Request
Use requests
to fetch the webpage content.
url = 'https://example.com'
response = requests.get(url)
Check if the request was successful by printing the response status code.
if response.status_code == 200:
print("Request successful!")
else:
print("Failed to retrieve content.")
Step 3: Parse HTML Content
Create a BeautifulSoup object to parse the HTML content of the page.
soup = BeautifulSoup(response.content, 'html.parser')
Navigating the Parse Tree
BeautifulSoup allows you to navigate and search through the HTML document with ease. Here are some common operations:
Accessing Tags
You can access specific tags by calling soup.tag_name
.
title = soup.title # Accesses the <title> tag
print(title.string) # Prints the text within the title tag
Extracting Data from Tags
Use methods like find()
, find_all()
, and attribute filters to extract specific data.
Using find() Method
first_paragraph = soup.find('p') # Finds the first <p> tag
print(first_paragraph.text) # Prints the text within the paragraph
Using find_all() Method
all_paragraphs = soup.find_all('p') # Finds all <p> tags
for para in all_paragraphs:
print(para.text) # Prints text of each paragraph
Filtering by Attributes
You can filter tags based on their attributes.
By Class Name
class_example = soup.find(class_='example-class')
print(class_example) # Prints the tag with class 'example-class'
By ID
id_example = soup.find(id='example-id')
print(id_example) # Prints the tag with id 'example-id'
Advanced BeautifulSoup Techniques
Navigating Siblings and Parents
You can navigate between siblings (previous/next tags) or access parent elements.
Sibling Navigation
first_tag = soup.find('p') # Find the first paragraph
next_sibling = first_tag.find_next_sibling()
print(next_sibling) # Prints the next sibling tag
Parent Navigation
first_tag = soup.find('p') # Find the first paragraph
parent = first_tag.find_parent()
print(parent) # Prints the parent of the paragraph
Using CSS Selectors
BeautifulSoup supports CSS selectors for more complex queries.
css_selector = soup.select('.example-class') # Finds all elements with class 'example-class'
for element in css_selector:
print(element)
Handling Dynamic Websites
Some websites load content dynamically using JavaScript, making traditional scraping methods insufficient. Tools like Selenium
can be used in conjunction with BeautifulSoup to handle such cases.
Using Selenium with BeautifulSoup
- Install Selenium:
pip install selenium webdriver-manager
- Import and set up the WebDriver.
from selenium import webdriver
from webdriver_manager.chrome import ChromeDriverManager
driver = webdriver.Chrome(ChromeDriverManager().install())
url = 'https://example.com'
driver.get(url)
- Parse the page source with BeautifulSoup after waiting for dynamic content to load.
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
WebDriverWait(driver, 10).until(EC.presence_of_element_located((By.CLASS_NAME, 'example-class')))
page_source = driver.page_source
soup = BeautifulSoup(page_source, 'html.parser')
Ethical Considerations and Best Practices
Respecting Robots.txt
Always check a website’s robots.txt
file to see which pages are allowed for scraping.
import requests
from bs4 import BeautifulSoup
url = 'https://example.com/robots.txt'
response = requests.get(url)
if response.status_code == 200:
print(response.text)
else:
print("Failed to retrieve robots.txt")
Rate Limiting
Avoid overwhelming the server with too many requests in a short period. Implement rate limiting using libraries like time
.
import time
for i in range(10):
response = requests.get('https://example.com')
print(response.status_code)
time.sleep(2) # Waits for 2 seconds before the next request
Avoiding Legal Issues
Ensure you comply with the website’s terms of service and copyright laws.
Conclusion
Web scraping with BeautifulSoup in Python is a powerful technique that opens up a world of data extraction possibilities. By following this guide, you now have the tools to start your own web scraping projects efficiently. Whether it’s for market research, content aggregation, or data analysis, BeautifulSoup offers a robust and user-friendly way to parse HTML documents.
FAQs
What is BeautifulSoup?
- BeautifulSoup is a Python library used for parsing HTML and XML documents. It creates parse trees that are helpful for extracting data from web pages.
Why use BeautifulSoup for web scraping?
- BeautifulSoup is easy to use, handles poorly designed HTML gracefully, and offers flexibility with different parsers like lxml and html5lib.
How do I install BeautifulSoup?
- You can install BeautifulSoup using pip:
pip install beautifulsoup4
. Additionally, you might need to install a parser likelxml
orhtml5lib
.
- You can install BeautifulSoup using pip:
Can BeautifulSoup handle dynamic content?
- BeautifulSoup itself cannot handle JavaScript-loaded content, but it can be used in conjunction with tools like Selenium to scrape dynamically loaded pages.
What are some ethical considerations when web scraping?
- Always respect a website’s
robots.txt
file, implement rate limiting, and ensure you comply with the website’s terms of service and copyright laws.
- Always respect a website’s