· Charlotte Will · webscraping · 6 min read
How to Automate Web Scraping with Python and AsyncIO
Discover how to automate web scraping using Python and AsyncIO. Learn best practices, error handling, rate limiting, and more to create efficient async web scrapers. Ideal for beginners to intermediate users aiming to extract data from websites quickly and effectively.
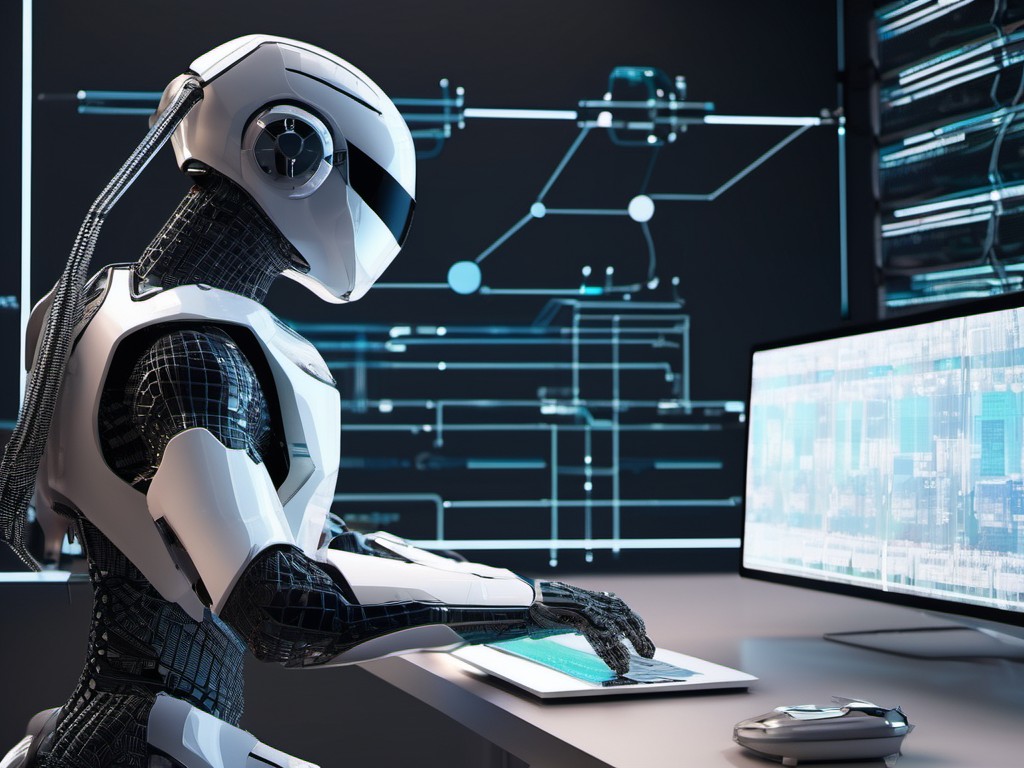
Web scraping has become an essential skill in the data science and development communities, enabling professionals to extract valuable information from websites efficiently. Traditional web scraping methods can be slow and resource-intensive when dealing with large datasets or numerous pages. However, by leveraging Python’s asyncio
library, you can significantly enhance the performance of your web scraping tasks.
This comprehensive guide will walk you through the process of automating web scraping using Python and AsyncIO. We’ll cover the basics of web scraping, introduce AsyncIO, provide step-by-step instructions for creating an async web scraper, and discuss best practices to ensure efficient and ethical data extraction.
What is Web Scraping?
Web scraping involves extracting data from websites using automated scripts or programs. This data can then be used for various purposes such as market research, price monitoring, content aggregation, and more. Python is a popular choice for web scraping due to its robust libraries like BeautifulSoup, Scrapy, and Requests.
Introduction to AsyncIO
AsyncIO is a Python library that allows you to write single-threaded concurrent code using the async
and await
syntax. It is particularly useful for I/O-bound tasks such as web scraping, where the majority of time is spent waiting for responses from servers rather than processing data. By using AsyncIO, you can perform multiple requests simultaneously, significantly speeding up your web scraping process.
Setting Up Your Environment
Before diving into the code, ensure you have a Python environment set up with the necessary libraries:
pip install requests beautifulsoup4 asyncio
Creating an Async Web Scraper
Let’s walk through the steps to create an async web scraper using Python and AsyncIO. We will use the aiohttp
library for making asynchronous HTTP requests.
Step 1: Import Libraries
First, import the necessary libraries:
import aiohttp
import asyncio
from bs4 import BeautifulSoup
Step 2: Define Asynchronous Functions
Define an asynchronous function to fetch the HTML content of a webpage. This function will use aiohttp
to make a GET request and return the page’s HTML.
async def fetch_html(session, url):
async with session.get(url) as response:
return await response.text()
Step 3: Parse HTML Content
Next, define a function to parse the HTML content and extract the desired data using BeautifulSoup.
def parse_html(html):
soup = BeautifulSoup(html, 'html.parser')
# Replace this with your actual parsing logic
data = [item.text for item in soup.select('your-selector')]
return data
Step 4: Main Function to Coordinate Tasks
Now, define the main function that will coordinate the tasks of fetching HTML and parsing it.
async def main(urls):
async with aiohttp.ClientSession() as session:
tasks = []
for url in urls:
task = asyncio.ensure_future(fetch_html(session, url))
tasks.append(task)
responses = await asyncio.gather(*tasks)
for response in responses:
data = parse_html(response)
print(data) # Replace with your desired data handling logic
Step 5: Run the Asynchronous Function
Finally, run the main function and pass a list of URLs you want to scrape.
if __name__ == '__main__':
urls = ['http://example.com/page1', 'http://example.com/page2'] # Replace with actual URLs
loop = asyncio.get_event_loop()
loop.run_until_complete(main(urls))
Best Practices for Async Web Scraping
Respect Robots.txt
Before scraping any website, always check the site’s robots.txt
file to ensure you are compliant with its rules regarding web crawling and indexing.
Rate Limiting
To avoid overwhelming a server with too many requests in a short period, implement rate limiting in your async scraper. You can use libraries like aiohttp
’s built-in semaphore to control the number of concurrent connections.
async def fetch_html(session, url, sem):
async with sem:
async with session.get(url) as response:
return await response.text()
Error Handling
Add error handling to manage exceptions and retries gracefully. This ensures that your scraper can continue running even if it encounters issues with specific URLs or servers.
async def fetch_html(session, url):
try:
async with session.get(url) as response:
return await response.text()
except Exception as e:
print(f"Error fetching {url}: {e}")
# Implement retry logic if needed
Alternative Methods for Web Scraping
While AsyncIO is a powerful tool for web scraping, there are alternative methods and tools you might consider depending on your specific needs:
Selenium
For websites that heavily rely on JavaScript to render content, Selenium can be a better choice. It allows you to control a web browser programmatically and extract data from dynamically loaded pages.
Learn more about automating web scraping with Python and Selenium: How to Automate Web Scraping with Python and Selenium.
Scrapy
Scrapy is a popular open-source web scraping framework that handles many aspects of web scraping, including request scheduling, concurrency control, and data extraction. It is particularly useful for larger projects requiring more advanced features.
FAQs
Q: How do I handle captchas when web scraping?
A: Handling captchas can be challenging because they are designed to prevent automated access. One approach is to use services that solve captchas manually or through machine learning models. Another option is to look for websites that offer APIs providing the data you need without requiring a manual captcha solution.
Q: What is the difference between synchronous and asynchronous web scraping?
A: Synchronous web scraping processes one request at a time in sequence, while asynchronous web scraping allows multiple requests to be handled concurrently. This can significantly speed up data extraction for large datasets or numerous pages.
Q: Is web scraping legal?
A: The legality of web scraping depends on the terms of service of the website you are scraping and local laws. Always ensure you comply with the site’s robots.txt
file and terms of use, and consider contacting the website owner if unsure.
Q: How can I avoid getting my IP blocked when web scraping?
A: To minimize the risk of being blocked, implement rate limiting to control the frequency of your requests. Use proxies or rotating IP addresses to distribute the load across multiple servers. Also, ensure you handle errors and retries gracefully to avoid overwhelming a server with repeated failed attempts.
Q: What are some best practices for storing scraped data?
A: Store scraped data in a structured format like CSV, JSON, or databases such as SQLite or PostgreSQL for easy access and analysis. Regularly back up your data and consider using version control systems to track changes and ensure data integrity.
Conclusion
Automating web scraping with Python and AsyncIO can significantly enhance the efficiency and speed of your data extraction tasks. By following best practices like respecting robots.txt
, implementing rate limiting, handling errors gracefully, and exploring alternative methods when necessary, you can create powerful and robust web scrapers tailored to your needs.
Embrace the potential of async web scraping and unlock the valuable insights hidden within the vast amount of data available on the web. Happy coding!