· Charlotte Will · webscraping · 4 min read
How to Handle Cookies and Sessions in Python Web Scraping
Discover how to handle cookies and sessions in Python web scraping. Learn practical techniques for managing cookies, bypassing cookie consent pop-ups, and maintaining effective session management. Enhance your web scraping skills with actionable advice tailored for both beginners and experienced developers.
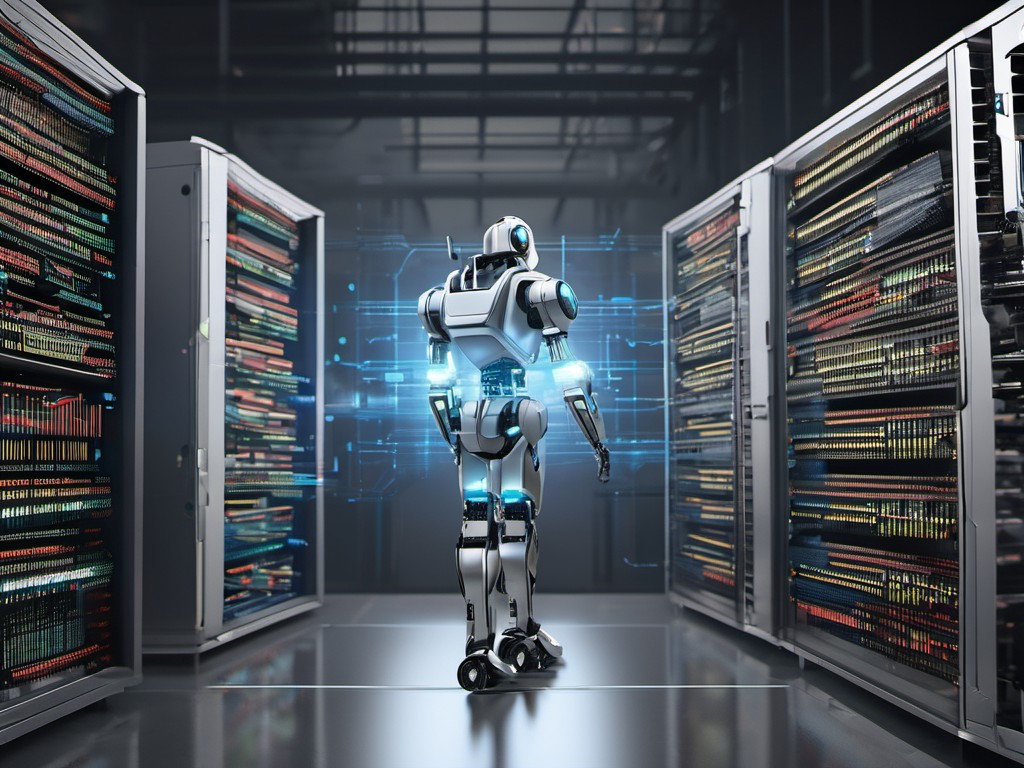
Title: How to Handle Cookies and Sessions in Python Web Scraping
Introduction
Web scraping is a powerful technique for extracting information from websites, but it often involves handling cookies and sessions to ensure smooth data retrieval. Properly managing these elements can make the difference between successful web scraping and failure. In this comprehensive guide, we’ll explore how to handle cookies and sessions in Python web scraping, providing practical tips and actionable advice for both beginners and experienced developers.
Understanding Cookies and Sessions in Web Scraping
Before diving into the technical aspects, it’s crucial to understand what cookies and sessions are. Cookies are small data files stored on a user’s browser by websites to remember information about that user. Sessions, on the other hand, are server-side storage mechanisms used to keep track of user interactions across multiple requests.
Why Handle Cookies in Web Scraping?
Handling cookies is essential for several reasons:
- Persistent Logins: Websites often require users to log in before accessing certain content. Handling cookies allows you to maintain a persistent login session.
- Personalized Content: Some websites serve personalized content based on user preferences stored in cookies. Managing these can help you scrape relevant data.
- Bypassing Rate Limits: Properly managing cookies can help you distribute requests more evenly, thus bypassing rate limits imposed by websites.
How to Handle Cookies in Python Web Scraping
Handling cookies involves creating, updating, and sending them with your requests. Here’s how you can do it using libraries like requests
and BeautifulSoup
.
Handling Cookies with Requests Library
The requests
library makes it easy to handle cookies:
import requests
# Create a session object
session = requests.Session()
# Log in to the website
login_data = {'username': 'your_username', 'password': 'your_password'}
session.post('https://example.com/login', data=login_data)
# Access a page that requires login
response = session.get('https://example.com/protected-page')
print(response.text)
In this example, we create a requests.Session()
, use it to send a login request, and then use the same session object to access protected pages.
Handling Cookie Consent Pop-ups
Many websites show cookie consent pop-ups that block web scraping. You can bypass these using headers or specific cookies:
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36'
}
cookies = {'cookie_consent': 'true'}
response = session.get('https://example.com', headers=headers, cookies=cookies)
print(response.text)
Managing Sessions in Python Web Scraping
Sessions are crucial for maintaining the state between requests. Here’s how you can manage them effectively:
Using Selenium for Session Management
For more complex scenarios, using Selenium
can be beneficial:
from selenium import webdriver
# Initialize the browser driver
driver = webdriver.Chrome()
# Open a website and perform actions
driver.get('https://example.com')
driver.find_element_by_name('username').send_keys('your_username')
driver.find_element_by_name('password').send_keys('your_password')
driver.find_element_by_name('submit').click()
# Extract data from the page
source = driver.page_source
driver.quit()
print(source)
Selenium
can handle JavaScript-rendered content and sessions more effectively than simple HTTP requests.
Handling CAPTCHAs and Anti-Bot Mechanisms
While handling cookies and sessions, you may encounter CAPTCHAs or other anti-bot mechanisms. Here are some tips:
- Rotate Proxies: Use rotating proxies to distribute requests across different IP addresses.
- Implement Delays: Add random delays between requests to mimic human behavior.
- User-Agent Rotation: Change User-Agent headers frequently to avoid detection.
Advanced Session Management Techniques
For more advanced scenarios, consider using libraries like Scrapy
:
import scrapy
class MySpider(scrapy.Spider):
name = 'my_spider'
start_urls = ['https://example.com']
def parse(self, response):
# Your parsing logic here
pass
Scrapy
offers built-in session management and can handle complex scraping tasks more efficiently.
FAQs
What is the difference between handling cookies and sessions?
- Cookies are client-side storage, while sessions are server-side. Handling both is crucial for maintaining a consistent state during web scraping.
How do I manage multiple cookies in Python web scraping?
- Use the
cookies
parameter of therequests
library to send multiple cookies with your requests:cookies = { 'cookie1': 'value1', 'cookie2': 'value2' } response = session.get('https://example.com', cookies=cookies)
- Use the
Can I use headers to manage sessions?
- While headers can contain useful information, they are not used for session management. Sessions and cookies are the primary methods for maintaining state.
How do I handle cookie consent pop-ups in web scraping?
- You can bypass these using specific headers or cookies that indicate consent:
headers = { 'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36' } cookies = {'cookie_consent': 'true'} response = session.get('https://example.com', headers=headers, cookies=cookies)
- You can bypass these using specific headers or cookies that indicate consent:
What are some best practices for managing sessions in Python web scraping?
- Use libraries like
requests
orSelenium
for managing sessions. Implement random delays and User-Agent rotation to avoid detection. Consider using rotating proxies for larger projects.
- Use libraries like
Conclusion
Handling cookies and sessions is crucial for effective web scraping in Python. By understanding the basics and applying practical techniques, you can extract data more efficiently and overcome common challenges like cookie consent pop-ups and anti-bot mechanisms. Whether you’re a beginner or an experienced developer, mastering these skills will greatly enhance your web scraping capabilities.
For further reading on related topics, check out our other articles: