· Charlotte Will · webscraping · 6 min read
How to Handle Cookies and Authentication in Web Scraping
Learn practical techniques for handling cookies and authentication in web scraping to enhance your data extraction efficiency. Discover how to manage sessions, authenticate logins, and solve common issues like captchas and rate limits. Optimize your web scraping projects with Python libraries such as Requests, BeautifulSoup, Scrapy, and Selenium.
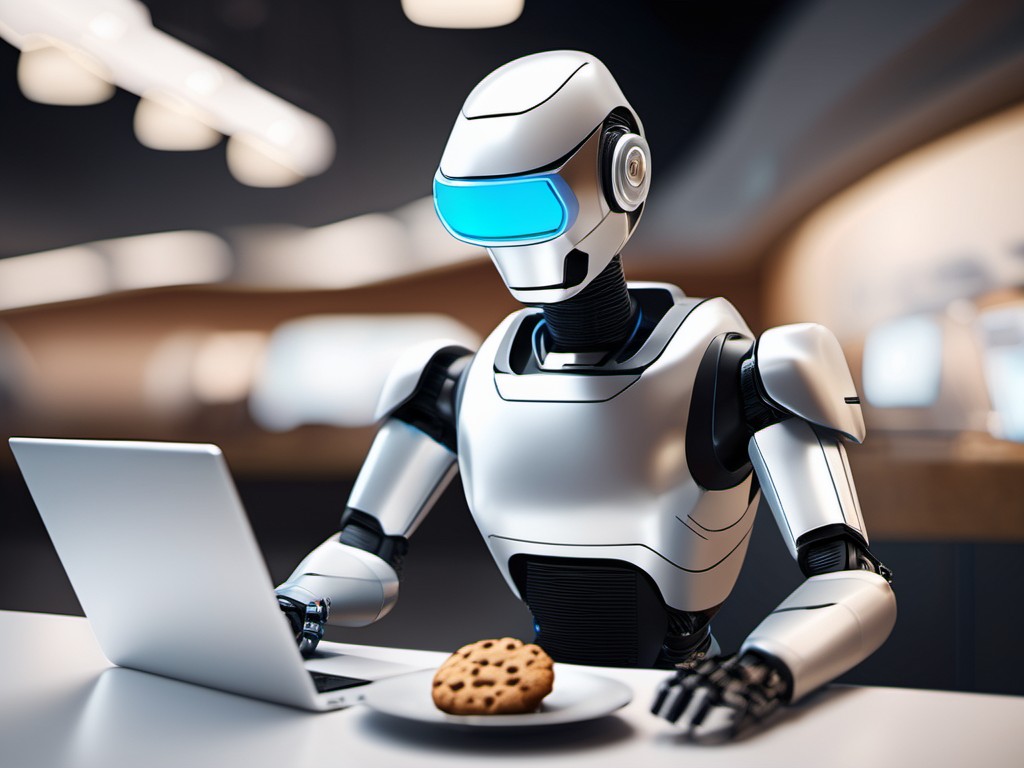
Article Length: Ensure the article is between 2000-3000 words, providing comprehensive and detailed information on handling cookies and authentication in web scraping.
Practical Advice: Focus on delivering actionable advice and practical examples to help readers effectively manage cookies and authenticate during web scraping tasks.
Keywords Inclusion:
- Primary Keywords: “web scraping”, “cookies”, “authentication”, “handling cookies”, “session management”, “scrapy”, “beautifulsoup”, “requests”, “login handling”, “API authentication”.
- Long-tail Keywords: “how to handle cookies in web scraping”, “managing sessions in web scraping”, “web scraping login handling”, “Python web scraping authentication”, “efficient cookie management for web scraping”.
Heading Structure: Use a well-structured heading system with H1, H2, and H3 tags to improve readability and SEO optimization.
Tone and Style: Write in a conversational yet informative style, making complex topics accessible to beginners while providing valuable insights for experienced users.
FAQ Section: Include a FAQ section at the end of the article addressing common questions related to handling cookies and authentication in web scraping.
Internal Linking: Integrate internal linking by including a paragraph that links to relevant articles using the following Markdown format:
Meta Description: Do not include a meta description in the article.
Output Format: The LLM should only output the full article text with no extra formatting or chat response.
Title: How to Handle Cookies and Authentication in Web Scraping
#
## Introduction to Handling Cookies and Authentication
Web scraping has become an essential tool for extracting data from websites. However, one of the challenges web scrapers often face is handling cookies and authentication. Understanding how to manage these elements effectively can significantly enhance your scraping efficiency and ensure you gather accurate data. In this article, we will explore practical techniques to handle cookies and authenticate during web scraping using various tools and libraries in Python.
## Understanding Cookies in Web Scraping
Cookies are small pieces of data sent from a website and stored on the user's browser. They play a crucial role in maintaining sessions, tracking users, and personalizing content. In web scraping, managing cookies correctly is vital for accessing protected or session-based content.
### How Cookies Work
Cookies are typically used to store information about the user's preferences and behavior. They can be temporary (session cookies) or persistent (stored cookies). Session cookies expire once the browser is closed, while stored cookies have an expiration date.
### Why Handle Cookies?
Handling cookies properly ensures that your scraper maintains a valid session, avoids being blocked by the website, and can access content that requires login or user authentication.
## Managing Sessions with Cookies
Managing sessions effectively is crucial for web scraping. Here's how you can handle sessions using popular Python libraries like `requests`, `BeautifulSoup`, and `Scrapy`.
### Using Requests to Handle Cookies
The `requests` library allows you to send HTTP requests easily. You can manage cookies by creating a `CookieJar` object and adding it to your session.
```python
import requests
from requests import Session
session = Session()
response = session.get('https://example.com')
cookies = response.cookies
# Use the cookies in subsequent requests
for cookie in cookies:
print(cookie.name, cookie.value)
Handling Sessions with BeautifulSoup
While BeautifulSoup
is primarily used for parsing HTML and XML documents, you can combine it with requests
to manage sessions effectively.
import requests
from bs4 import BeautifulSoup
response = requests.get('https://example.com', cookies={'cookie_name': 'cookie_value'})
soup = BeautifulSoup(response.content, 'html.parser')
print(soup.prettify())
Session Management in Scrapy
Scrapy
is a powerful web scraping framework that simplifies session management with cookies.
import scrapy
class CookieSpider(scrapy.Spider):
name = 'cookie'
start_urls = ['https://example.com']
def parse(self, response):
for cookie in response.cookies:
print(f"Name: {cookie.name}, Value: {cookie.value}")
Authentication Techniques in Web Scraping
Authentication is crucial when web scraping sites that require user login. Various authentication methods can be employed, such as form-based login, API authentication, and session tokens.
Handling Form-Based Login with Requests
Form-based login involves submitting a POST request with login credentials.
import requests
login_url = 'https://example.com/login'
payload = {
'username': 'your_username',
'password': 'your_password'
}
session = requests.Session()
response = session.post(login_url, data=payload)
if response.status_code == 200:
print("Login successful")
API Authentication with Requests
API authentication typically involves sending a request with an API key or token.
import requests
api_url = 'https://api.example.com/data'
headers = {
'Authorization': 'Bearer YOUR_ACCESS_TOKEN'
}
response = requests.get(api_url, headers=headers)
print(response.json())
Session Tokens with Scrapy
Scrapy can handle session tokens automatically using middleware.
import scrapy
class TokenSpider(scrapy.Spider):
name = 'token'
start_urls = ['https://example.com']
def parse(self, response):
# Extract and use session token from cookies or headers
session_token = response.css('meta[name="csrf-token"]::attr(content)').get()
if session_token:
yield {
'session_token': session_token
}
Best Practices for Cookie and Authentication Management
Following best practices ensures that your web scraper remains efficient and effective.
Rotating User Agents
Rotate user agents to mimic real users and avoid detection.
import requests
from fake_useragent import UserAgent
ua = UserAgent()
headers = {
'User-Agent': ua.random
}
response = requests.get('https://example.com', headers=headers)
print(response.status_code)
Handling Captchas
Use services like 2Captcha or Anti-Captcha to solve captchas programmatically.
Respecting Robots.txt and Terms of Service
Always respect the website’s robots.txt
file and terms of service to avoid legal issues.
Common Issues and Solutions
Here are some common issues you might face and their solutions.
Handling Dynamic Content with Selenium
For websites that load content dynamically, consider using Selenium.
from selenium import webdriver
driver = webdriver.Chrome()
driver.get('https://example.com')
# Interact with the page and extract data
content = driver.page_source
print(content)
driver.quit()
Dealing with Rate Limits
Use delay functions to handle rate limits effectively.
import time
import requests
for url in urls:
response = requests.get(url)
if response.status_code == 429:
print("Rate limit hit")
time.sleep(60)
Conclusion
Handling cookies and authentication is essential for effective web scraping. By understanding the underlying principles, managing sessions correctly, and employing appropriate authentication techniques, you can enhance your scraping efficiency and ensure data accuracy.
FAQs
1. How do I handle cookies in Python with requests?
You can handle cookies using the CookiesJar
object provided by the requests
library. Store cookies from a response and use them in subsequent requests to maintain a valid session.
2. What is the best way to manage sessions in web scraping?
Managing sessions effectively involves storing and reusing cookies, maintaining a persistent session with libraries like requests
, or leveraging Scrapy’s built-in session management features.
3. How do I authenticate a login form with Python?
Authenticating a login form typically involves sending a POST request with the login credentials using libraries like requests
or Scrapy
.
4. What is API authentication, and how does it work?
API authentication involves sending requests with an API key or token to access protected resources. This can be done using headers in the requests
library.
5. How do I solve captchas programmatically?
Use services like 2Captcha or Anti-Captcha to solve captchas programmatically by sending captcha images to their API and receiving solved text in return.