· Charlotte Will · Amazon API · 6 min read
Mastering Web Scraping with AsyncIO and Aiohttp for Performance Optimization
Master web scraping with AsyncIO and Aiohttp to enhance performance and efficiency. Learn practical tips, best practices, and advanced strategies for error handling and rate limiting. Optimize your web scraper's performance when dealing with large datasets and paginated APIs.
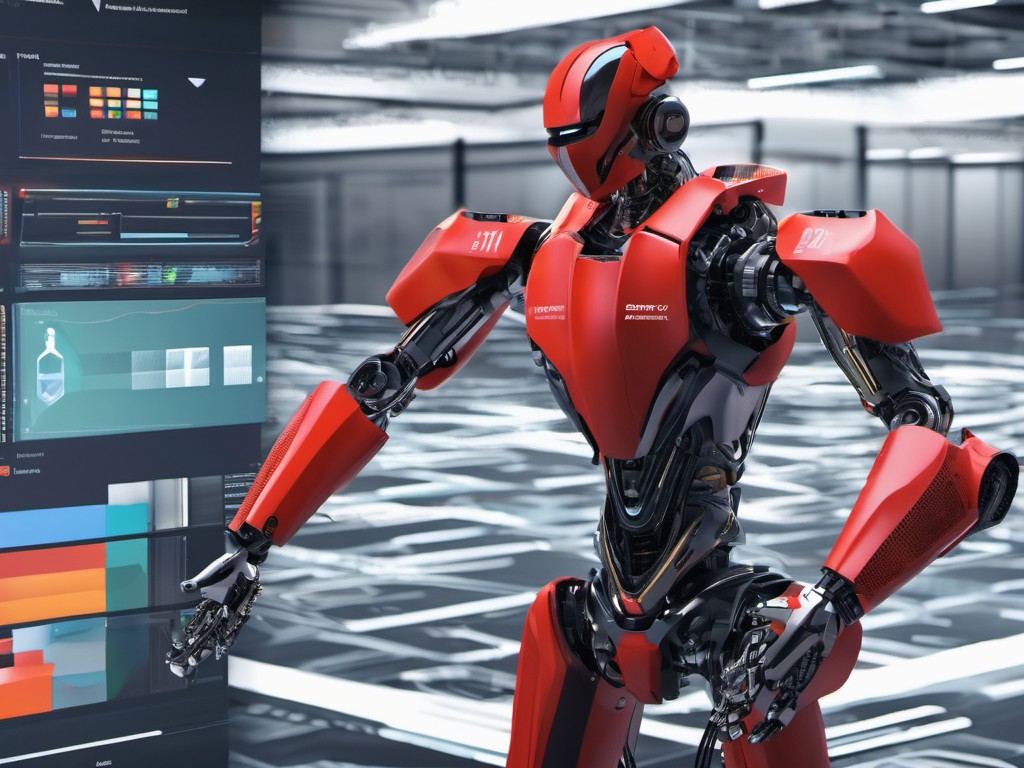
Web scraping is an essential technique for extracting valuable data from websites. However, traditional web scraping methods using synchronous requests can be slow and inefficient, especially when dealing with large-scale projects. This is where asynchronous programming comes into play, allowing you to perform multiple tasks concurrently and significantly improve performance. In this article, we will dive deep into mastering web scraping with AsyncIO and Aiohttp for performance optimization.
Introduction to Web Scraping and Performance Optimization
Web scraping involves extracting data from websites using automated scripts or software. While it can be incredibly useful, traditional synchronous methods can often lead to bottlenecks, especially when dealing with a large number of requests. This is where asynchronous programming shines, enabling you to handle multiple requests simultaneously without blocking the execution flow.
What is AsyncIO and Aiohttp?
AsyncIO is a Python library that provides support for asynchronous I/O operations. It allows you to write single-threaded concurrent code using the async
and await
keywords. Aiohttp, on the other hand, is an HTTP client/server framework that works seamlessly with AsyncIO, making it ideal for performing asynchronous web requests.
Benefits of Using AsyncIO and Aiohttp for Web Scraping
- Improved Performance: By handling multiple requests concurrently, you can drastically reduce the time required to scrape data from websites.
- Resource Efficiency: Asynchronous programming allows you to make better use of system resources, as it does not require creating new threads for each request.
- Scalability: AsyncIO and Aiohttp are well-suited for large-scale web scraping projects, enabling you to handle a high volume of requests efficiently.
- Ease of Use: Both libraries provide intuitive APIs that make it easy to write asynchronous code without sacrificing readability.
Setting Up Your Environment
Before we dive into the practical aspects of web scraping with AsyncIO and Aiohttp, let’s set up our environment:
pip install aiohttp
This will install the necessary libraries for performing asynchronous HTTP requests.
Writing Your First Asynchronous Web Scraper
Let’s start with a simple example to illustrate how you can use AsyncIO and Aiohttp to perform web scraping efficiently. We’ll create a script that fetches data from a hypothetical website asynchronously:
import asyncio
import aiohttp
async def fetch(session, url):
async with session.get(url) as response:
return await response.text()
async def main():
urls = [
'https://example.com/page1',
'https://example.com/page2',
'https://example.com/page3',
]
async with aiohttp.ClientSession() as session:
tasks = [fetch(session, url) for url in urls]
results = await asyncio.gather(*tasks)
for result in results:
print(result[:100]) # Print the first 100 characters of each response
if __name__ == '__main__':
asyncio.run(main())
In this example, we define an async
function called fetch
that makes an HTTP GET request to a given URL using Aiohttp’s ClientSession
. The main function then creates tasks for each URL and uses asyncio.gather
to run them concurrently. This allows us to fetch data from multiple URLs simultaneously, significantly improving performance compared to synchronous requests.
Handling Pagination and Large Datasets
When dealing with large datasets or paginated APIs, it’s important to handle the flow of data efficiently. Let’s modify our example to handle a situation where we need to scrape multiple pages:
import asyncio
import aiohttp
async def fetch_page(session, url):
async with session.get(url) as response:
return await response.json() # Assuming the data is in JSON format
async def main():
base_url = 'https://example.com/api'
num_pages = 10 # Replace with actual number of pages
async with aiohttp.ClientSession() as session:
tasks = [fetch_page(session, f"{base_url}?page={page}") for page in range(1, num_pages + 1)]
results = await asyncio.gather(*tasks)
for result in results:
print(result[:20]) # Print the first 20 characters of each JSON response
if __name__ == '__main__':
asyncio.run(main())
In this example, we assume that the API returns data in JSON format and that there are multiple pages to scrape. We generate tasks for fetching each page asynchronously and then gather the results. This approach ensures that we efficiently handle large datasets without overwhelming the server or our system resources.
Advanced Topics: Error Handling and Rate Limiting
When performing web scraping at scale, it’s crucial to implement error handling and rate limiting strategies to avoid getting blocked by target websites. Here’s how you can enhance your asynchronous web scraper with these features:
Error Handling
import asyncio
import aiohttp
async def fetch(session, url):
try:
async with session.get(url) as response:
return await response.text()
except Exception as e:
print(f"Error fetching {url}: {e}")
return None
async def main():
urls = [
'https://example.com/page1',
'https://example.com/page2',
'https://example.com/page3',
]
async with aiohttp.ClientSession() as session:
tasks = [fetch(session, url) for url in urls]
results = await asyncio.gather(*tasks)
for result in results:
print(result[:100]) # Print the first 100 characters of each response
if __name__ == '__main__':
asyncio.run(main())
Rate Limiting
To avoid getting blocked, you can introduce delays between requests using asyncio.sleep
:
import asyncio
import aiohttp
async def fetch(session, url):
try:
async with session.get(url) as response:
await asyncio.sleep(1) # Introduce a delay between requests
return await response.text()
except Exception as e:
print(f"Error fetching {url}: {e}")
return None
async def main():
urls = [
'https://example.com/page1',
'https://example.com/page2',
'https://example.com/page3',
]
async with aiohttp.ClientSession() as session:
tasks = [fetch(session, url) for url in urls]
results = await asyncio.gather(*tasks)
for result in results:
print(result[:100]) # Print the first 100 characters of each response
if __name__ == '__main__':
asyncio.run(main())
FAQ Section
What is AsyncIO and how does it benefit web scraping?
AsyncIO is a Python library that supports asynchronous I/O operations, allowing you to write concurrent code using the async
and await
keywords. In web scraping, AsyncIO enables you to handle multiple requests simultaneously without blocking the execution flow, significantly improving performance and resource efficiency.
How does Aiohttp complement AsyncIO for web scraping?
Aiohttp is an HTTP client/server framework that works seamlessly with AsyncIO, making it ideal for performing asynchronous web requests. It provides a simple and intuitive API for making HTTP requests, which can be easily integrated into your AsyncIO-based web scraper.
What are some best practices for error handling in asynchronous web scraping?
Some best practices for error handling in asynchronous web scraping include:
- Using try-except blocks to catch and handle exceptions gracefully.
- Implementing retries with exponential backoff to handle transient errors.
- Logging errors with relevant information, such as the URL and error message.
- Introducing delays between requests to avoid overwhelming the target website or your system resources.
How can I optimize my web scraper’s performance when dealing with large datasets?
To optimize your web scraper’s performance when dealing with large datasets, you can:
- Use asynchronous programming (AsyncIO and Aiohttp) to handle multiple requests concurrently.
- Implement pagination handling to efficiently fetch data from multiple pages.
- Introduce delays between requests to comply with rate limits and avoid getting blocked.
- Utilize parallel processing or multiprocessing to further improve performance, especially when dealing with CPU-bound tasks.
- Consider using a distributed system (e.g., Apache Kafka) for large-scale web scraping projects.
Conclusion
Mastering web scraping with AsyncIO and Aiohttp can significantly enhance your web scraping projects’ performance and efficiency. By leveraging asynchronous programming, you can handle multiple requests concurrently, reduce execution time, and make better use of system resources. This article has provided practical advice and actionable content to help you get started with asynchronous web scraping using Python.
For more insights into web scraping and related topics, check out these relevant articles: Mastering Web Scraping with AsyncIO and Aiohttp for Performance Optimization, Web Scraping with AsyncIO and Aiohttp in Python, How to Automate Web Scraping with Python and AsyncIO.