· Charlotte Will · webscraping · 5 min read
Web Scraping with AsyncIO and Aiohttp in Python
Learn how to perform asynchronous web scraping using AsyncIO and Aiohttp in Python. This comprehensive guide covers practical tips, performance optimization techniques, and real-world examples to help you build efficient and robust web scraping applications.
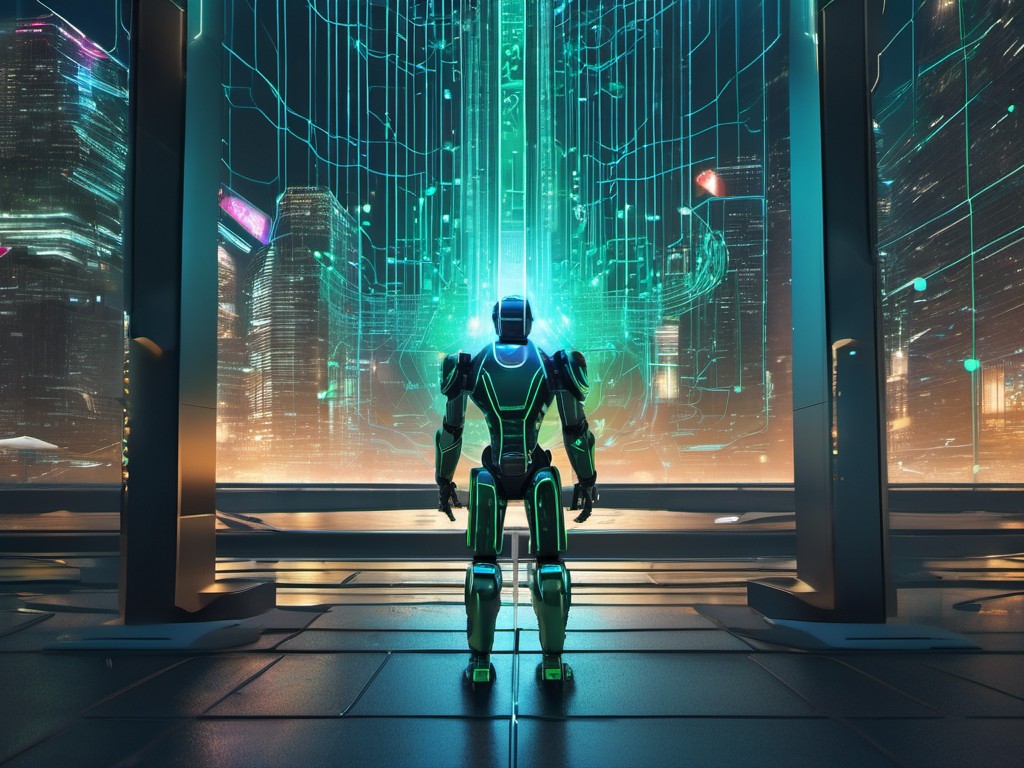
Welcome to the world of web scraping! If you’re looking to extract data from websites at lightning speeds, then asynchronous web scraping using AsyncIO and Aiohttp is your ticket. In this comprehensive guide, we’ll dive deep into how to use these powerful tools to optimize your Python web scraping projects for performance and efficiency.
Why Use AsyncIO and Aiohttp?
Traditional web scraping methods can be slow and inefficient, especially when dealing with multiple requests at once. Synchronous requests are sequential, meaning each request has to wait for the previous one to complete before it starts. This can lead to significant delays, particularly on websites with slower response times or large datasets.
AsyncIO and Aiohttp change the game by allowing you to make multiple requests simultaneously. Instead of waiting for one request to finish before moving onto the next, these tools enable you to send multiple requests at once, significantly speeding up your web scraping process.
Getting Started with AsyncIO
AsyncIO is Python’s built-in library for writing concurrent code using the async/await syntax. Before diving into aiohttp, it’s essential to understand how AsyncIO works.
Installing AsyncIO
First things first, you need to install AsyncIO:
pip install asyncio
Basic AsyncIO Example
Here’s a simple example to illustrate how AsyncIO works:
import asyncio
async def say_hello():
print("Hello")
await asyncio.sleep(1) # Simulating a delay
print("World!")
async def main():
task = asyncio.create_task(say_hello())
await task
# Run the main function until it's complete
asyncio.run(main())
Aiohttp: The Asynchronous HTTP Client
Aiohttp is an asynchronous HTTP client/server framework for Python that works seamlessly with AsyncIO. It’s designed to be fast, reliable, and easy to use.
Installing Aiohttp
Install aiohttp using pip:
pip install aiohttp
Basic Aiohttp Example
Let’s look at a simple example of how to make an asynchronous HTTP request with aiohttp:
import aiohttp
import asyncio
async def fetch(session, url):
async with session.get(url) as response:
return await response.text()
async def main():
url = 'https://example.com'
async with aiohttp.ClientSession() as session:
html = await fetch(session, url)
print(html[:100]) # Print the first 100 characters of the response
# Run the main function until it's complete
asyncio.run(main())
Performing Asynchronous Web Scraping
Now that you have a basic understanding of AsyncIO and Aiohttp, let’s put them together to perform asynchronous web scraping.
Asynchronous Web Scraping Example
Here’s an example where we scrape data from multiple URLs concurrently:
import aiohttp
import asyncio
from bs4 import BeautifulSoup
async def fetch(session, url):
async with session.get(url) as response:
return await response.text()
async def parse_html(html):
soup = BeautifulSoup(html, 'html.parser')
title = soup.title.string
return title
async def main():
urls = [
'https://example.com',
'https://www.python.org',
'https://docs.python.org/3/'
]
async with aiohttp.ClientSession() as session:
tasks = []
for url in urls:
task = asyncio.create_task(fetch(session, url))
tasks.append(task)
htmls = await asyncio.gather(*tasks)
for html in htmls:
title = await parse_html(html)
print(title)
# Run the main function until it's complete
asyncio.run(main())
Optimizing Performance with AsyncIO and Aiohttp
While the above example is a good start, there are several ways to optimize your asynchronous web scraping for better performance.
Using Semaphores to Control Concurrency
To prevent overloading the target server (and possibly getting blocked), you can control the number of concurrent requests using semaphores:
import aiohttp
import asyncio
from bs4 import BeautifulSoup
sem = asyncio.Semaphore(10) # Limit to 10 simultaneous connections
async def fetch(session, url):
async with sem:
async with session.get(url) as response:
return await response.text()
# Rest of the code remains the same...
Using Sessions Efficiently
Aiohttp sessions can be reused for multiple requests, which is more efficient than creating a new session for each request. Make sure to create and manage your sessions properly:
async def fetch(session, url):
async with session.get(url) as response:
return await response.text()
async def main():
urls = [
'https://example.com',
'https://www.python.org',
'https://docs.python.org/3/'
]
async with aiohttp.ClientSession() as session:
tasks = []
for url in urls:
task = asyncio.create_task(fetch(session, url))
tasks.append(task)
htmls = await asyncio.gather(*tasks)
# Rest of the code remains the same...
For more advanced techniques on optimizing performance with AsyncIO and Aiohttp, refer to our guide on Mastering Web Scraping with AsyncIO and Aiohttp for Performance Optimization.
Handling Exceptions
It’s crucial to handle exceptions in your web scraping code to ensure robustness and reliability. You can use try-except blocks to catch and manage errors gracefully:
async def fetch(session, url):
try:
async with session.get(url) as response:
return await response.text()
except aiohttp.ClientError as e:
print(f"Error fetching {url}: {e}")
return None
# Rest of the code remains the same...
Saving Data to a File
Once you’ve fetched and parsed your data, you might want to save it to a file for later use. Here’s how you can do that:
async def main():
# ... (fetching and parsing code remains the same)
with open('titles.txt', 'w') as f:
for title in titles:
f.write(title + '\n')
# Rest of the code remains the same...
Automating Your Web Scraping Tasks
If you’re looking to automate your web scraping tasks, check out our tutorial on How to Automate Web Scraping with Python and AsyncIO.
Conclusion
AsyncIO and Aiohttp are powerful tools for optimizing your web scraping projects in Python. By utilizing asynchronous programming, you can significantly speed up your data extraction process and handle multiple requests concurrently. With proper performance optimization techniques and exception handling, you can build robust and efficient web scraping applications.
Happy scraping! 🚀🐍
FAQs
Why should I use asynchronous web scraping? Asynchronous web scraping allows you to make multiple requests simultaneously, significantly speeding up the data extraction process compared to synchronous methods.
What is Aiohttp? Aiohttp is an asynchronous HTTP client/server framework for Python that works seamlessly with AsyncIO, designed to be fast and easy to use.
How do I install AsyncIO and Aiohttp? You can install them using pip:
pip install asyncio aiohttp
.Can I control the number of concurrent requests in my web scraping code? Yes, you can use semaphores to limit the number of simultaneous connections and prevent overloading the target server.
How do I handle exceptions when fetching data? You can use try-except blocks to catch and manage errors gracefully in your web scraping code.