· Charlotte Will · webscraping · 7 min read
Deep Dive into JavaScript Rendering for Web Scraping
Discover how to effectively handle JavaScript rendering in web scraping using tools like Selenium, Puppeteer, and headless browsers. Learn advanced techniques and strategies to extract dynamic content from JavaScript-heavy websites.
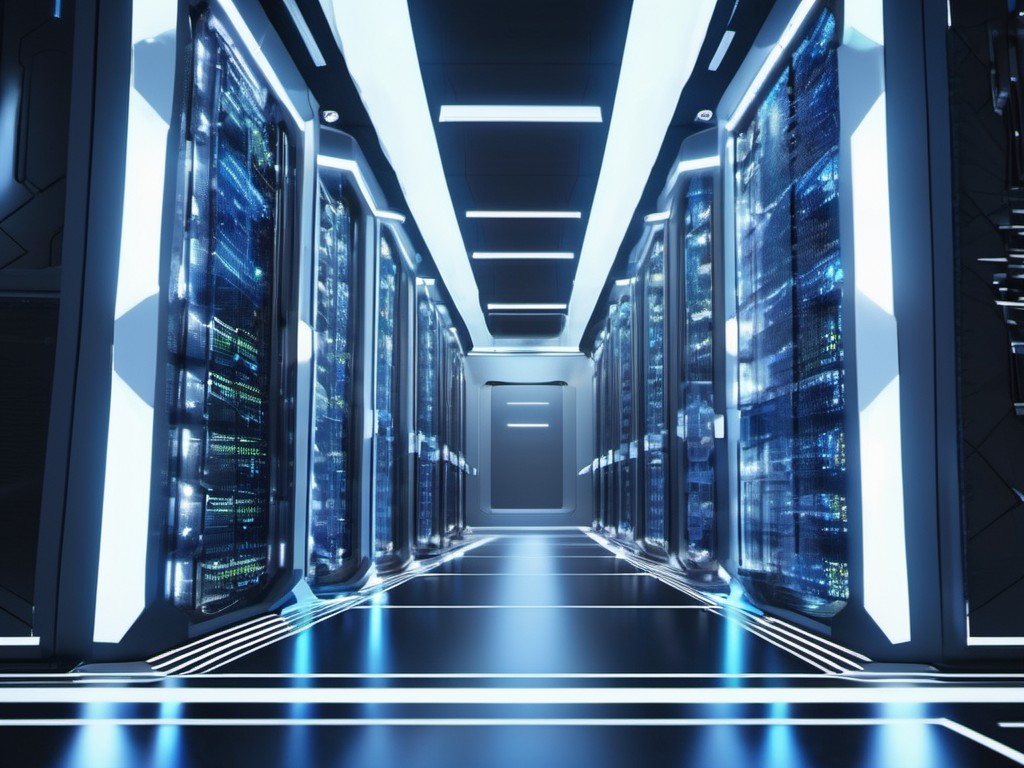
JavaScript has become an integral part of modern web development, allowing developers to create dynamic and interactive websites. However, for web scrapers, JavaScript-rendered content can be a significant challenge. Traditional web scraping tools often fail to handle content generated by JavaScript, leading to incomplete or incorrect data extraction. In this comprehensive guide, we’ll explore various techniques and strategies to effectively handle JavaScript rendering when web scraping.
Understanding JavaScript Rendering
Before diving into the techniques, it’s crucial to understand what JavaScript rendering is. Traditional web pages are static, meaning their content is pre-rendered on the server before being sent to the client’s browser. In contrast, JavaScript-rendered content is dynamically generated in the browser after the initial page load. This dynamic nature poses a challenge for traditional scraping tools that only parse the initial HTML response.
Types of JavaScript Rendering
- Client-Side Rendering (CSR): In CSR, the entire web page is rendered on the client side using JavaScript frameworks like React or Vue.js. This approach offers high interactivity but can be problematic for scrapers since the content isn’t available in the initial HTML response.
- Server-Side Rendering (SSR): In SSR, the web page is pre-rendered on the server and sent to the client as a fully rendered HTML page. However, some parts of the page may still be dynamically loaded with JavaScript after the initial load.
- Static Site Generation (SSG): SSG involves generating static HTML pages at build time using JavaScript frameworks like Next.js or Gatsby. These pages are then served to clients, offering fast load times but minimal interactivity.
Techniques for Handling JavaScript Rendering in Web Scraping
1. Using Headless Browsers with Selenium
One of the most effective ways to handle JavaScript rendering is by using headless browsers. A headless browser runs without a graphical user interface, allowing you to control it programmatically. Selenium is a popular tool for automating web browsers and can be used to scrape JavaScript-rendered content.
Example Code Snippet (Python with Selenium):
from selenium import webdriver
from selenium.webdriver.chrome.options import Options
# Set up the headless Chrome browser
options = Options()
options.headless = True
browser = webdriver.Chrome(options=options)
# Navigate to the target website
browser.get('https://example.com')
# Wait for JavaScript rendering to complete (use WebDriverWait for more complex scenarios)
import time
time.sleep(5)
# Extract the rendered content
content = browser.page_source
print(content)
# Close the browser
browser.quit()
2. Scrapy with Splash or Playwright
Scrapy is a powerful and flexible web scraping framework for Python, but it doesn’t handle JavaScript rendering out of the box. To overcome this limitation, you can use Splash or Playwright middleware to render JavaScript content within your Scrapy spider.
Example Code Snippet (Scrapy with Splash):
import scrapy
from scrapy_splash import SplashRequest
class MySpider(scrapy.Spider):
name = 'my_spider'
start_urls = ['https://example.com']
def start_requests(self):
for url in self.start_urls:
yield SplashRequest(url, self.parse, args={'wait': 5})
def parse(self, response):
# Extract the rendered content
content = response.body
print(content)
3. Using Puppeteer for Headless Chrome
Puppeteer is a Node.js library that provides a high-level API to control headless Chrome or Chromium browsers. It’s an excellent choice for JavaScript rendering in web scraping, especially when you prefer using JavaScript rather than Python.
Example Code Snippet (JavaScript with Puppeteer):
const puppeteer = require('puppeteer');
(async () => {
const browser = await puppeteer.launch({ headless: true });
const page = await browser.newPage();
// Navigate to the target website
await page.goto('https://example.com', { waitUntil: 'networkidle2' });
// Extract the rendered content
const content = await page.content();
console.log(content);
await browser.close();
})();
4. Handling AJAX Requests
Many websites use AJAX requests to load dynamic content asynchronously. To handle these cases, you need to intercept and process the AJAX requests along with the initial page load. Tools like Selenium and Puppeteer can help you achieve this by allowing you to wait for specific elements or network conditions before extracting data.
Advanced Strategies for JavaScript Rendering in Web Scraping
1. Handling Infinite Scroll Pages
Infinite scroll pages load additional content as the user scrolls down. To scrape these pages, you need to simulate scroll actions and wait for new content to render before extracting data.
Example Code Snippet (Python with Selenium):
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
import time
# Set up the headless Chrome browser
browser = webdriver.Chrome()
# Navigate to the target website
browser.get('https://example.com/infinite-scroll')
# Simulate scroll actions and wait for new content
for _ in range(10): # Adjust the number of scrolls as needed
browser.find_element_by_tag_name('body').send_keys(Keys.END)
time.sleep(2) # Wait for new content to load
# Extract the rendered content
content = browser.page_source
print(content)
# Close the browser
browser.quit()
2. Dealing with JavaScript Frameworks and Libraries
Different JavaScript frameworks and libraries may require specific handling techniques. For example, React and Vue.js use virtual DOMs that need to be rendered before extracting data. Familiarize yourself with the target website’s technology stack to optimize your scraping approach accordingly.
3. Optimizing Performance
Headless browsers can be resource-intensive, especially when scraping large websites or multiple pages simultaneously. To improve performance, consider the following tips:
- Reuse browser instances: Instead of launching a new browser instance for each request, reuse existing ones to save resources and reduce startup time.
- Limit browser tabs: Opening too many browser tabs can lead to excessive memory usage. Monitor your resource consumption and adjust the number of concurrent requests accordingly.
- Use efficient selectors: Optimize your CSS or XPath selectors to quickly locate and extract data from rendered pages.
- Parallel processing: Leverage parallel processing techniques to scrape multiple pages simultaneously while avoiding bottlenecks caused by JavaScript rendering.
FAQs About JavaScript Rendering for Web Scraping
1. Which tools are best for handling JavaScript rendering in web scraping?
Selenium, Puppeteer, and headless browser middleware like Splash or Playwright are popular choices for handling JavaScript rendering in web scraping. The best tool depends on your specific use case, programming language preference, and project requirements.
2. Can I use traditional web scraping tools to handle JavaScript-rendered content?
Traditional web scraping tools like BeautifulSoup or Scrapy may not be sufficient for handling JavaScript-rendered content, as they primarily parse static HTML. However, you can combine these tools with headless browsers or middleware to extract dynamic data effectively.
3. How do I know if a website uses JavaScript rendering?
To determine if a website uses JavaScript rendering, inspect the page’s source code and network requests. Look for signs of dynamic content loading, such as AJAX requests, JavaScript frameworks, or inline scripts that manipulate the DOM after the initial load.
4. What are the main challenges of web scraping with JavaScript rendering?
The primary challenges of web scraping with JavaScript rendering include:
- Dynamic content loading: Waiting for JavaScript-rendered content to load before extracting data.
- Resource consumption: Headless browsers can be resource-intensive, requiring careful management of browser instances and concurrent requests.
- Technology stack variance: Different websites may use various JavaScript frameworks and libraries, requiring custom handling techniques.
- Legal and ethical considerations: Ensure your web scraping activities comply with the target website’s terms of service and relevant laws.
5. How can I optimize my web scraping performance when dealing with JavaScript rendering?
To optimize web scraping performance with JavaScript rendering, focus on:
- Reusing browser instances: Minimize the overhead of launching new browsers for each request.
- Limiting browser tabs: Monitor and control resource consumption by adjusting the number of concurrent requests.
- Using efficient selectors: Optimize your data extraction selectors to quickly locate and process rendered content.
- Parallel processing: Leverage parallel processing techniques to scrape multiple pages simultaneously while managing JavaScript rendering efficiently.
For a deeper understanding of handling JavaScript rendered content, you might want to check out our guide on How to Handle JavaScript Rendered Content in Python Web Scraping. If you’re interested in scraping dynamic websites, consider exploring Scraping Dynamic Content Loaded by JavaScript Frameworks and Handling AJAX Requests in Python Web Scraping.
Conclusion
Handling JavaScript rendering is an essential skill for modern web scrapers. By leveraging headless browsers, middleware, and advanced strategies, you can effectively extract data from dynamic websites. Keep in mind the challenges and optimization techniques discussed in this guide to enhance your web scraping performance while staying compliant with legal and ethical considerations.
With practice and experimentation, you’ll become proficient in tackling JavaScript rendering and unlock new possibilities for web scraping projects. Happy scraping! 🕷️💻