· Charlotte Will · webscraping · 4 min read
Handling AJAX Requests in Python Web Scraping
Discover how to handle AJAX requests in Python web scraping effectively. Learn practical methods using Selenium and other tools to extract dynamic content seamlessly. Perfect for beginners and experienced developers aiming to capture real-time data from modern websites.
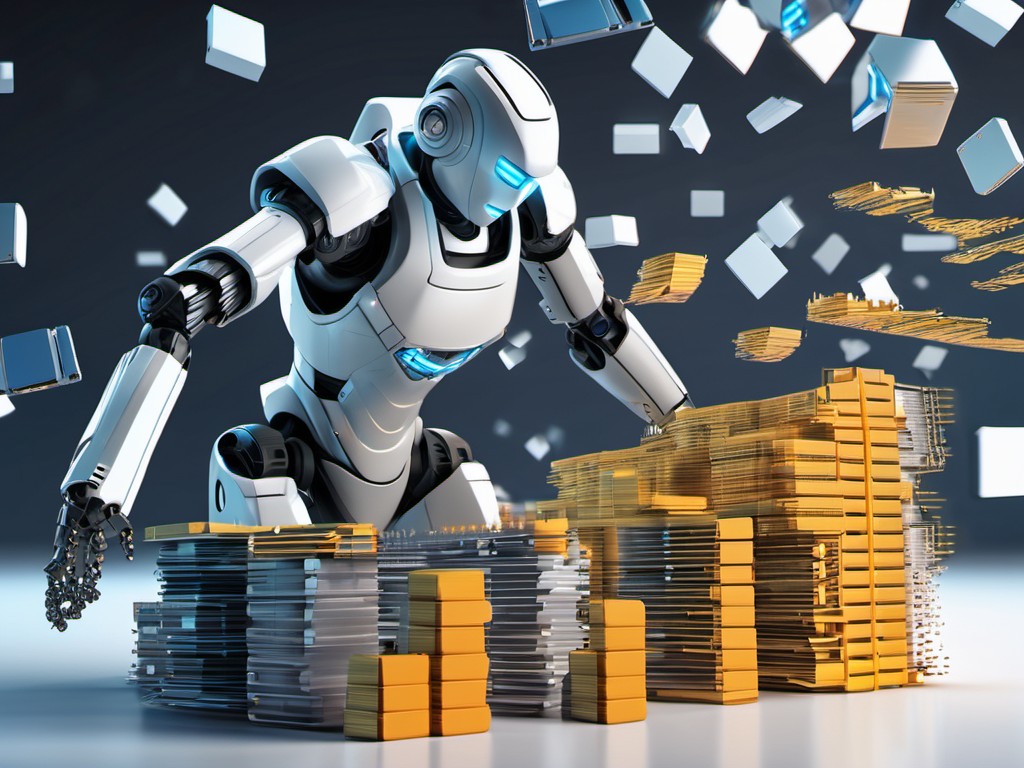
Web scraping is an essential skill in data extraction, allowing you to gather information from websites efficiently. However, many modern sites use dynamic content loading through AJAX (Asynchronous JavaScript and XML) requests, making traditional scraping methods ineffective. This guide will walk you through handling AJAX requests in Python web scraping, ensuring you can extract dynamic data seamlessly.
Understanding AJAX Requests
AJAX allows web pages to be updated asynchronously by exchanging small amounts of data with the server behind the scenes. This means content is loaded without refreshing the entire page, enhancing user experience. For scrapers, this introduces a challenge: static web scraping tools often fail to capture dynamically loaded content.
Why Handle AJAX Requests?
Handling AJAX requests is crucial for accessing real-time data, such as live updates on stock prices or social media feeds. Ignoring these requests can leave you with incomplete or outdated information.
Tools for Handling AJAX Requests
Several tools and libraries help Python developers handle AJAX requests effectively:
Selenium
Selenium is a popular choice for handling AJAX because it can automate browser interactions. By simulating real user behavior, Selenium ensures that all dynamically loaded content is captured.
Setting Up Selenium
First, install the Selenium package:
pip install selenium
Next, download a WebDriver for your browser (e.g., ChromeDriver for Google Chrome).
BeautifulSoup and Requests
For simpler cases where AJAX requests are not critical, BeautifulSoup combined with Requests can be used to parse HTML content. However, this method may miss dynamic data.
Handling AJAX Requests with Selenium
Here’s a step-by-step guide on using Selenium to handle AJAX requests:
1. Initialize the WebDriver
from selenium import webdriver
# Example for Chrome
driver = webdriver.Chrome(executable_path='/path/to/chromedriver')
2. Navigate to the Target Website
driver.get('http://example.com')
3. Wait for AJAX Content to Load
Use explicit waits to ensure all content is loaded before scraping:
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
WebDriverWait(driver, 10).until(EC.presence_of_element_located((By.ID, 'targetElement')))
4. Extract the Data
Once the content is loaded, you can extract data using BeautifulSoup or directly through Selenium:
from bs4 import BeautifulSoup
soup = BeautifulSoup(driver.page_source, 'html.parser')
data = soup.find_all('div', class_='targetDataClass')
for item in data:
print(item.text)
5. Close the WebDriver
Always close the WebDriver to free up resources:
driver.quit()
Advanced Techniques
Handling Infinite Scroll
Websites with infinite scroll load content as you scroll down. Selenium can automate this process:
last_height = driver.execute_script("return document.body.scrollHeight")
while True:
# Scroll down to bottom
driver.execute_script("window.scrollTo(0, document.body.scrollHeight);")
# Wait for new content to load
WebDriverWait(driver, 10).until(lambda driver: driver.execute_script("return document.readyState") == "complete")
# Calculate new scroll height and compare with last scroll height
new_height = driver.execute_script("return document.body.scrollHeight")
if new_height == last_height:
try:
driver.execute_script("window.stop();")
except:
break
last_height = new_height
Extracting Data from API Calls
Sometimes, AJAX requests fetch data via API calls. Intercept these using browser developer tools and mimic them in Python with requests
:
import requests
response = requests.get('http://example.com/api/endpoint')
data = response.json()
print(data)
Best Practices
Respect Robots.txt
Always check the website’s robots.txt
file to ensure you are allowed to scrape its content.
Handle Rate Limits
Avoid overwhelming servers with too many requests. Implement rate limiting using libraries like tenacity
.
Use Headless Browsers
For production-level scraping, use headless browsers (headless mode
) to speed up operations and reduce resource usage.
Conclusion
Handling AJAX requests in Python web scraping requires a combination of tools and techniques. Selenium stands out for its ability to interact with dynamic content, while BeautifulSoup and Requests can handle simpler tasks. By following best practices and understanding the intricacies of AJAX, you can effectively extract real-time data from modern websites.
FAQs
1. Can I use Selenium for large-scale web scraping?
While Selenium is powerful, it may not be the best choice for large-scale scraping due to its resource intensity. Consider using headless browsers and optimizing your code for efficiency.
2. How do I handle CAPTCHAs while scraping with Selenium?
Handling CAPTCHAs can be challenging. Services like 2Captcha or Anti-Captcha offer solutions, but using them may violate terms of service. Always prioritize ethical scraping practices.
3. What is the difference between explicit and implicit waits in Selenium?
Explicit waits are more flexible and reliable as they wait for a specific condition to be met. Implicit waits, on the other hand, apply a general timeout to all WebDriver commands.
4. How can I handle AJAX requests without using a browser automation tool?
For API-driven AJAX requests, you can directly interact with the API endpoints using libraries like requests
. However, this approach may not capture all dynamically loaded content.
5. Is it legal to scrape websites?
The legality of web scraping varies by country and website terms of service. Always review a site’s robots.txt file and terms of service before starting any scraping project. Ethical considerations are crucial in maintaining responsible data practices.