· Charlotte Will · webscraping · 4 min read
How to Scrape Data from Password-Protected Websites
Discover how to scrape data from password-protected websites using Python, Selenium, and other tools. Learn best practices for handling authentication, cookies, sessions, and ethical considerations in web scraping.
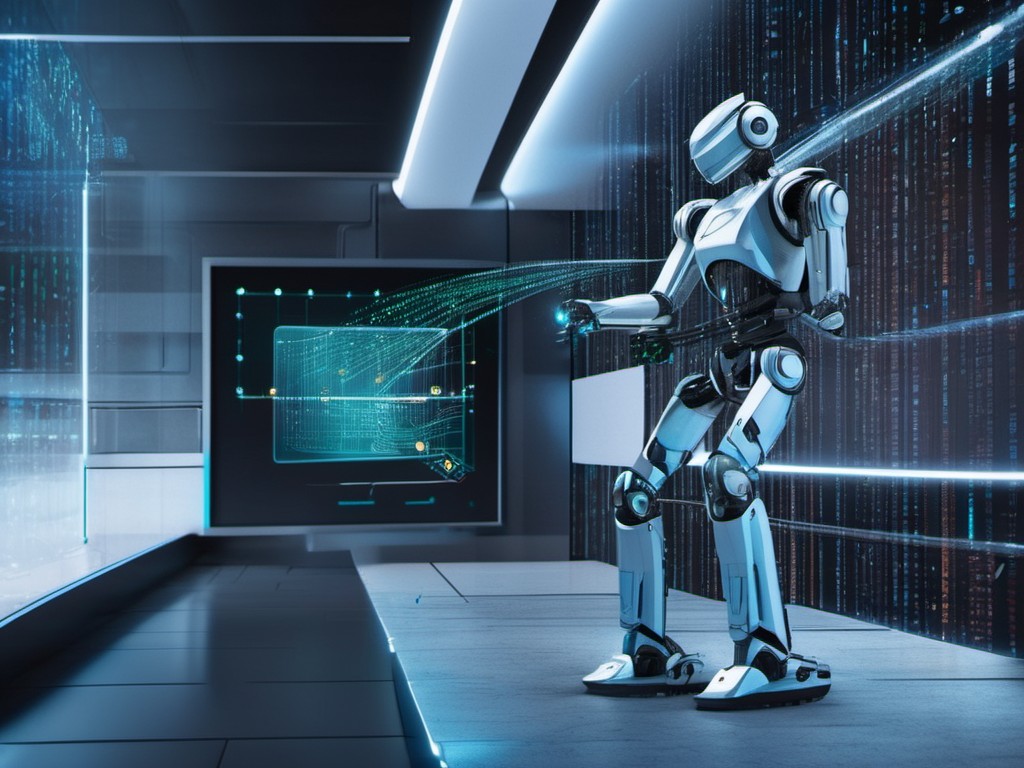
Introduction
Web scraping has become an essential skill in data extraction, especially when dealing with password-protected websites. While these sites offer valuable information, they pose a challenge due to their protected nature. In this guide, we’ll explore the best practices and tools for scraping data from password-protected websites securely and efficiently.
Understanding Password-Protected Websites
Password-protected websites require users to log in before accessing their content. This added layer of security makes it more challenging but not impossible to scrape data. The key lies in mimicking a human login process programmatically, which involves handling authentication mechanisms like cookies and sessions.
Tools and Libraries for Web Scraping
Several tools and libraries can help you scrape password-protected websites effectively. Some popular choices include:
Python Libraries
- Beautiful Soup: Great for parsing HTML and XML documents.
- Requests: For handling HTTP requests.
- Selenium: A powerful tool for automating web browsers.
- Scrapy: An open-source web crawling framework.
Specialized Tools
- Octoparse: A user-friendly web scraping tool with a point-and-click interface.
- ParseHub: Offers advanced features and handles complex scraping tasks.
Setting Up Authentication for Scraping
To scrape password-protected websites, you need to automate the login process. Here’s how you can do it using Python and Selenium:
Step-by-Step Instructions
- Install Selenium: Use
pip install selenium
. - Download WebDriver: Get the appropriate driver for your browser (e.g., ChromeDriver).
- Login Script: Write a script to automate the login process.
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
import time
# Initialize WebDriver
driver = webdriver.Chrome(executable_path='/path/to/chromedriver')
# Navigate to the login page
driver.get('https://example.com/login')
# Locate username and password fields, then input credentials
username = driver.find_element_by_id('username')
password = driver.find_element_by_id('password')
username.send_keys('your_username')
password.send_keys('your_password')
# Submit the login form
password.send_keys(Keys.RETURN)
# Wait for the page to load after logging in
time.sleep(5)
Handling Cookies and Sessions
Cookies and sessions are crucial for maintaining a logged-in state while scraping. Most web frameworks, such as Requests and Scrapy, support managing cookies directly.
Example with Requests
import requests
from bs4 import BeautifulSoup
# Login and save session cookies
session = requests.Session()
login_data = {'username': 'your_username', 'password': 'your_password'}
response = session.post('https://example.com/login', data=login_data)
# Now you can use the session to make authenticated requests
authenticated_response = session.get('https://example.com/protected-page')
soup = BeautifulSoup(authenticated_response.content, 'html.parser')
Best Practices for Scraping Password-Protected Sites
- Respect Robots.txt: Always check the
robots.txt
file to ensure you’re not scraping disallowed pages. - Rate Limiting: Implement rate limiting to avoid overloading the server.
- Error Handling: Include error handling for network issues and authentication failures.
- Logging: Use logging to keep track of your scraping activities and any errors that occur.
- Data Storage: Store extracted data securely, preferably in encrypted databases or files.
Legal and Ethical Considerations
While web scraping can be highly beneficial, it’s essential to consider the legal and ethical implications:
- Terms of Service (ToS): Ensure your scraping activities comply with the website’s ToS.
- Copyright Laws: Be aware of copyright laws and respect intellectual property rights.
- Privacy Concerns: Handle personal data responsibly and adhere to privacy regulations like GDPR.
Conclusion
Scraping password-protected websites requires a combination of technical skills and ethical considerations. By using the right tools and following best practices, you can extract valuable data efficiently while respecting legal boundaries.
For more advanced techniques on web scraping, refer to our guides on How to Scrape Data from Websites Using Puppeteer and How to Scrape Data from Infinite Scroll Websites. These resources provide deeper insights into automating data extraction using different tools and methods.
FAQ Section
What is the difference between web scraping and crawling? Web scraping involves extracting specific data from a website, while crawling refers to systematically browsing and indexing websites for search engines.
Can I scrape data without breaking the law? Yes, as long as you respect the website’s ToS, comply with copyright laws, and handle personal data responsibly.
How often should I update my scraped data? The frequency depends on how frequently the data updates on the source website. Regular checks can help maintain data relevance.
What is the best tool for web scraping password-protected sites? Selenium is a powerful and versatile tool for automating login processes and scraping password-protected sites.
How do I handle CAPTCHAs during web scraping? CAPTCHA handling can be complex and sometimes requires third-party services or manual intervention. It’s best to avoid websites with heavy CAPTCHA protection if possible.