· Charlotte Will · webscraping · 5 min read
Implementing Geospatial Data Extraction with Python and Web Scraping
Discover how to implement geospatial data extraction using Python and web scraping techniques. This comprehensive guide covers practical methods, libraries like BeautifulSoup, Geopy, Folium, and Geopandas, as well as real-time data extraction and advanced analysis techniques.
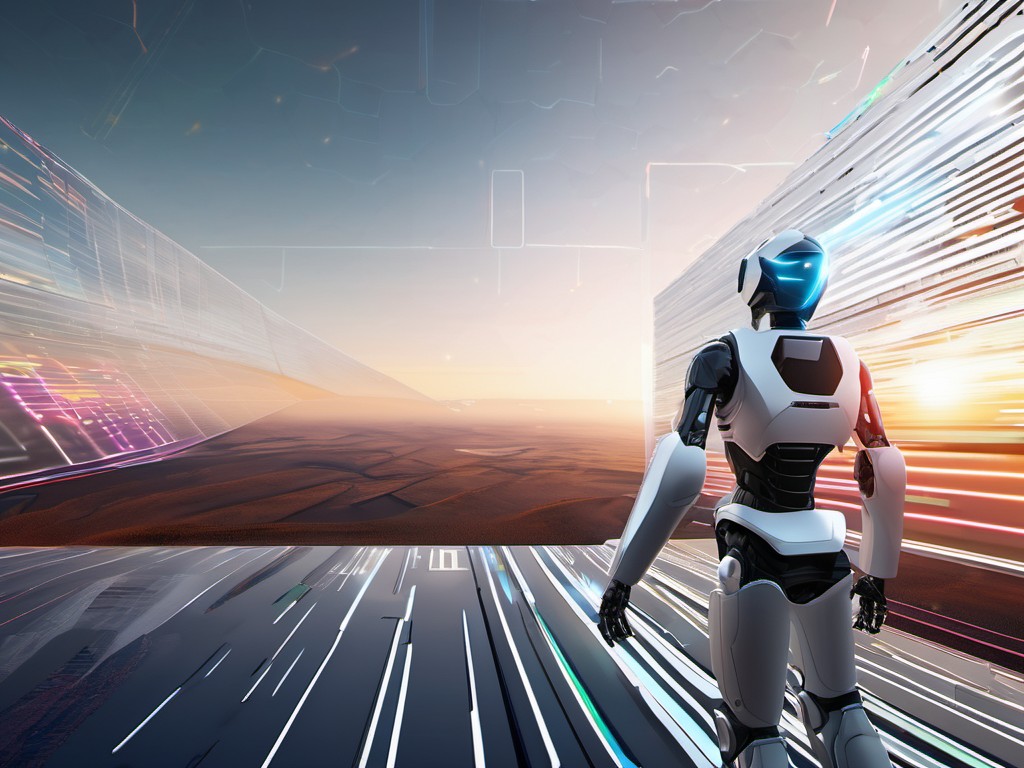
Geospatial data extraction has become increasingly crucial in various industries, including urban planning, environmental science, and logistics. By combining the power of web scraping and Python, you can effectively extract, analyze, and visualize geographic information. This guide will walk you through the process of implementing geospatial data extraction using Python and web scraping techniques.
Introduction to Geospatial Data Extraction
Geospatial data encompasses any information that has a location component, such as addresses, latitude-longitude coordinates, or even spatial boundaries. Extracting this data can involve gathering it from various sources like websites, APIs, and databases. Web scraping is an effective method to collect such data programmatically, while Python offers robust libraries for processing and analyzing geospatial information.
Why Use Python for Geospatial Data Extraction?
Python is a popular choice for geospatial data extraction due to its versatile libraries and ease of use. Libraries like BeautifulSoup
, Scrapy
, and requests
make web scraping straightforward, while GIS-specific libraries such as geopandas
and folium
facilitate spatial data analysis and visualization.
Setting Up Your Environment
Before diving into the code, you need to set up your Python environment. Install necessary libraries using pip:
pip install requests beautifulsoup4 geopandas folium
Web Scraping Basics for Geospatial Data
Extracting Addresses with BeautifulSoup
Let’s start by scraping addresses from a website using BeautifulSoup
.
import requests
from bs4 import BeautifulSoup
url = 'https://example.com/locations'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
addresses = []
for item in soup.find_all('div', class_='location'):
addr = item.find('p').text
addresses.append(addr)
print(addresses)
This code snippet fetches and parses the HTML content of a webpage, extracting addresses from specific elements.
Geocoding with Geopy
Once you have the addresses, you can convert them into geographic coordinates using libraries like geopy
.
from geopy.geocoders import Nominatim
geolocator = Nominatim(user_agent="geoapiExercises")
def geocode_address(address):
location = geolocator.geocode(address)
return (location.latitude, location.longitude) if location else None
coordinates = [geocode_address(addr) for addr in addresses]
print(coordinates)
This function takes an address and returns its latitude and longitude coordinates.
Data Visualization with Folium
Visualizing geospatial data is essential for understanding spatial patterns. Folium
makes it easy to create interactive maps.
import folium
m = folium.Map(location=[37.0, -95.0], zoom_start=6)
for coord in coordinates:
if coord:
folium.Marker([coord[0], coord[1]], popup=f'{coord}').add_to(m)
m.save('map.html')
This code creates a map centered around the United States, marking each location with its coordinates.
Spatial Data Analysis with Geopandas
For more advanced spatial data analysis, geopandas
provides powerful tools.
import geopandas as gpd
from shapely.geometry import Point
# Assume 'addresses' and 'coordinates' are already defined
df = pd.DataFrame({'Address': addresses, 'Coordinates': coordinates})
gdf = gpd.GeoDataFrame(df, geometry=[Point(xy) for xy in df['Coordinates']], crs="EPSG:4326")
# Example analysis: Calculate the centroid of all points
centroid = gdf.geometry.centroid
print(centroid)
This code converts addresses and coordinates into a geospatial dataframe, enabling complex spatial analyses like calculating centroids or performing spatial joins.
Integrating Geospatial APIs
APIs offer another way to extract geospatial data. For instance, the OpenStreetMap (OSM) API allows you to query for specific points of interest.
import requests
url = 'https://nominatim.openstreetmap.org/search'
params = {'q': 'restaurant', 'format': 'json', 'limit': 5}
response = requests.get(url, params=params)
data = response.json()
for place in data:
print(place['display_name'])
This code snippet queries the OSM API for restaurants and prints their names.
Real-Time Geospatial Data Extraction
Extracting real-time geospatial data can be achieved by setting up a web scraper to run at regular intervals or using streaming APIs that push updates. For example, you could use Scrapy
with a scheduler to periodically extract data from websites.
import scrapy
from scrapy.crawler import CrawlerProcess
class GeoSpider(scrapy.Spider):
name = "geospatial_data"
start_urls = ['https://example.com/live-locations']
def parse(self, response):
for item in response.css('div.location'):
addr = item.css('p::text').get()
yield {'Address': addr}
process = CrawlerProcess({
'USER_AGENT': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36'
})
process.crawl(GeoSpider)
process.start()
This code defines a Scrapy
spider to extract real-time data and schedules it to run periodically.
Advanced Geospatial Data Analysis Techniques
Buffer Analysis
Create buffers around points to analyze spatial relationships.
buffer = gdf.geometry.buffer(5) # Buffer of 5 km
gdf['Buffer'] = buffer
print(gdf)
Spatial Joins
Combine geospatial data with other datasets based on location.
other_gdf = gpd.read_file('other_data.shp')
joined_gdf = gpd.sjoin(gdf, other_gdf, how="inner", op='intersects')
print(joined_gdf)
Heatmaps
Visualize density using heatmaps.
import folium
m = folium.Map(location=[37.0, -95.0], zoom_start=6)
folium.GeoJson(gdf).add_to(m)
folium.plugins.HeatMap(gdf).add_to(m)
m.save('heatmap.html')
Conclusion
Implementing geospatial data extraction with Python and web scraping opens up a world of possibilities for spatial analysis and visualization. By combining powerful libraries like BeautifulSoup
, Geopy
, Folium
, and Geopandas
, you can efficiently extract, process, and analyze geospatial data. Whether you’re performing simple address extraction or advanced spatial joins, Python provides the tools needed to succeed in geospatial data extraction.
FAQs
What is geospatial data extraction? Geospatial data extraction involves collecting location-based information from various sources like websites and APIs.
Why use Python for geospatial data analysis? Python offers a rich ecosystem of libraries tailored for geospatial data analysis, making it an ideal choice for processing and visualizing spatial data.
How can web scraping be used to extract geographic information? Web scraping allows you to programmatically fetch geographic information from websites, enabling automated data collection at scale.
What libraries are essential for geospatial data analysis in Python? Libraries like
BeautifulSoup
for web scraping,Geopy
for geocoding, andFolium
&Geopandas
for data visualization and spatial analysis are crucial.How can I perform real-time geospatial data extraction? Real-time extraction can be achieved by using schedulers with web scraping tools like
Scrapy
, or leveraging streaming APIs that push updates.