· Charlotte Will · webscraping · 4 min read
Scraping Single Page Applications (SPA) with Headless Browsers
Learn how to scrape Single Page Applications (SPA) using headless browsers like Puppeteer, Selenium, and Playwright. Discover best practices, common challenges, and effective tools for extracting dynamic content from SPAs.
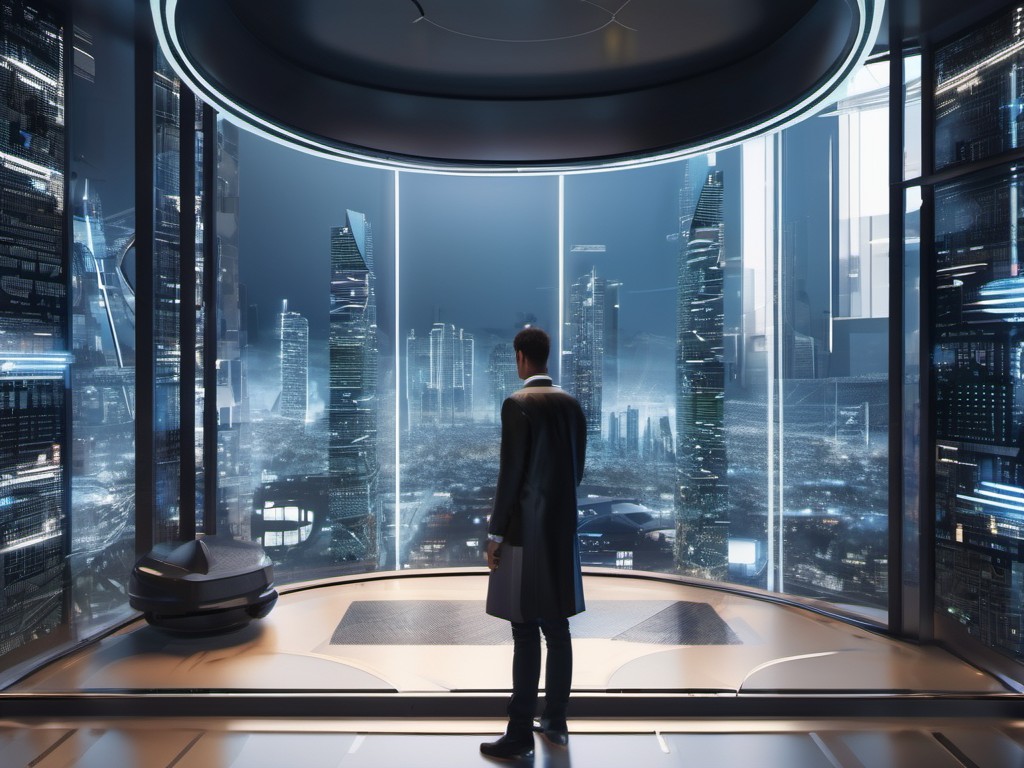
Introduction to Scraping Single Page Applications (SPA)
In today’s dynamic web landscape, Single Page Applications (SPAs) have become increasingly popular. SPAs provide a seamless user experience by dynamically updating content without requiring page reloads. However, this also makes traditional web scraping methods ineffective, as the data is loaded via JavaScript after the initial page load.
Enter headless browsers—these tools automate web interactions and render pages just like a regular browser would, making them ideal for scraping SPAs. This guide will walk you through everything you need to know about using headless browsers to scrape Single Page Applications effectively.
What are Headless Browsers?
Headless browsers are web automation tools that operate without a graphical user interface (GUI). They can perform all the tasks of a regular browser, including rendering JavaScript and interacting with dynamic content, but they do so in a server environment rather than on your desktop. Popular headless browsers include Puppeteer, Selenium, and Playwright.
Why Use Headless Browsers for SPA Scraping?
Scraping SPAs with traditional methods like curl
or requests
is inadequate because these tools can’t execute JavaScript. Headless browsers solve this problem by:
- Rendering JavaScript: They can execute and wait for JavaScript to load content dynamically.
- Handling Dynamic Content: Interacting with elements like dropdowns, buttons, and forms that traditional scrapers cannot handle.
- Simulating Real User Behavior: Mimicking human interactions, making it harder for websites to detect and block your scraper.
Step-by-Step Guide to Scraping SPAs with Headless Browsers
Setting Up Your Environment
Before you start scraping, ensure your environment is set up correctly:
- Install Node.js: Most headless browsers require Node.js.
- Create a Project Directory: Use
npm init
to set up a new project. - Install Dependencies: Install the headless browser library of your choice (e.g., Puppeteer).
Choosing the Right Headless Browser
Several headless browsers are available, each with its own strengths:
- Puppeteer: Developed by Google, it’s widely used and well-documented.
- Selenium: Supports multiple languages and has a large community.
- Playwright: Offers multi-browser support (Chromium, Firefox, WebKit).
Writing the Scraper Script
Here’s an example using Puppeteer to scrape data from an SPA:
const puppeteer = require('puppeteer');
(async () => {
const browser = await puppeteer.launch();
const page = await browser.newPage();
await page.goto('https://example.com/spa');
// Wait for the necessary data to load
await page.waitForSelector('#dynamic-content');
// Extract data
const data = await page.evaluate(() => {
return Array.from(document.querySelectorAll('.data-item')).map(item => item.textContent);
});
console.log(data);
await browser.close();
})();
Best Practices for Effective SPA Scraping
- Use Proper Waits: Ensure your script waits for elements to load before extracting data.
- Handle CAPTCHAs and Bot Detection: Implement strategies like rotating IP addresses or using proxy services.
- Optimize Performance: Minimize browser actions and use efficient selectors to improve speed.
- Respect Robots.txt: Always check the
robots.txt
file of the website you’re scraping and adhere to its rules. - Log Errors Gracefully: Implement error handling and logging to make debugging easier.
Common Challenges and Solutions in SPA Scraping
- Infinite Scrolling: Use
page.evaluateHandle
to check for more content and scroll accordingly. - Anti-Bot Mechanisms: Rotate user agents, use proxies, and simulate human behavior.
- Dynamic URLs: Extract data from the browser’s history API or use regex to handle dynamic routes.
Tools and Libraries for SPA Scraping with Headless Browsers
- Puppeteer: Excellent for Node.js projects, with robust APIs.
- Selenium: Versatile and supports multiple languages and browsers.
- Playwright: Offers advanced features like multi-browser support.
- Cheerio and Axios: Useful for scraping static content after headless browser extraction.
For a more comprehensive guide, refer to our detailed article on Scraping Single Page Applications (SPA) with Headless Browsers. If you’re new to headless browsers and want a broader understanding, check out our guide on How to Use Headless Browsers for Web Scraping.
Conclusion
Scraping SPAs with headless browsers is a powerful technique that allows you to extract dynamic content effectively. By following best practices and leveraging the right tools, you can build robust scrapers that handle even the most complex JavaScript-heavy sites. Whether you’re new to web scraping or an experienced developer, headless browsers provide the flexibility and power needed to stay ahead in today’s fast-paced web environment.
FAQ Section
Can I use headless browsers for other tasks besides scraping? Yes, headless browsers are also used for automated testing, generating screenshots, and more.
Which is the best headless browser for SPA scraping? The “best” browser depends on your specific needs. Puppeteer is great for Node.js projects, while Playwright offers multi-browser support.
How can I handle CAPTCHAs when scraping with a headless browser? Implement strategies like rotating IP addresses or using proxy services to reduce the likelihood of encountering CAPTCHAs.
Is it legal to scrape websites? The legality of web scraping depends on your use case and the website’s terms of service. Always check
robots.txt
and respect the site’s rules.What are some common mistakes to avoid when scraping SPAs with headless browsers? Avoid hardcoding selectors, neglecting error handling, and ignoring website policies. Always test your script thoroughly to ensure it works as expected.