· Charlotte Will · webscraping · 5 min read
How to Scrape JSON Data Using Python
Discover how to effectively scrape JSON data using Python with this comprehensive guide. Learn step-by-step methods, best practices, and troubleshooting tips to extract valuable information from web pages efficiently. Perfect for beginners to intermediate Python developers looking to enhance their data scraping skills.
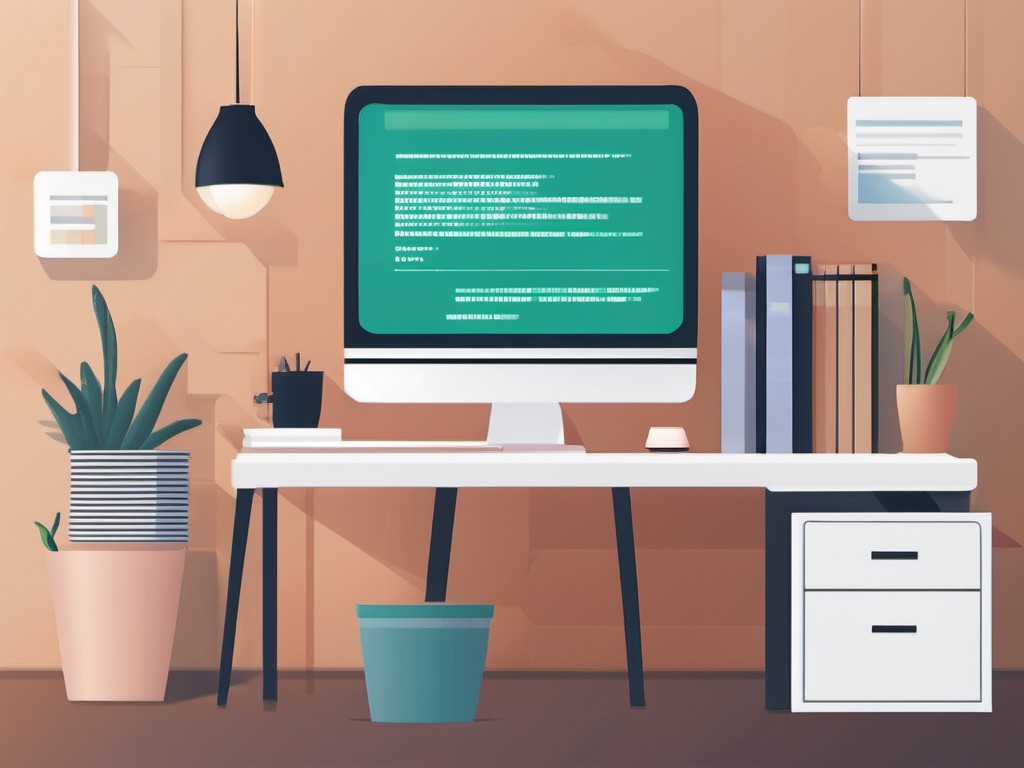
How to Scrape JSON Data Using Python
JSON (JavaScript Object Notation) is a lightweight data interchange format that’s easy for humans to read and write, and easy for machines to parse and generate. It has become the standard for transmitting data between web applications and servers. This article will guide you through the process of scraping JSON data using Python, providing practical advice and code examples to help you get started.
Introduction
In today’s data-driven world, being able to extract and manipulate JSON data is a crucial skill. Whether you’re gathering data for analysis or integrating different services, knowing how to scrape JSON data can save you time and effort. Python, with its powerful libraries like requests
and BeautifulSoup
, is an excellent choice for this task.
Setting Up Your Environment
Before diving into the code, let’s set up your environment by installing the necessary libraries. You’ll need requests
to make HTTP requests and json
to handle JSON data. Optionally, you can use BeautifulSoup
for parsing HTML if needed.
Installing Necessary Libraries
You can install these libraries using pip:
pip install requests beautifulsoup4
Understanding JSON Data Structure
JSON data is structured in key-value pairs, similar to Python dictionaries. It supports various data types like strings, numbers, arrays (lists), and objects (dictionaries). Here’s a simple example of a JSON file:
{
"name": "John Doe",
"age": 30,
"isStudent": false,
"courses": ["Math", "Science"],
"address": {
"street": "123 Main St",
"city": "Anytown"
}
}
Scraping JSON from Web Pages
Scraping JSON data involves making HTTP requests to web pages that serve JSON and then parsing the response. Let’s go through the steps:
Step 1: Make an HTTP Request
Use the requests
library to send a GET request to the URL serving JSON data.
import requests
url = 'https://api.example.com/data'
response = requests.get(url)
Step 2: Parse the JSON Response
Once you have the response, you can parse it using the json()
method provided by the requests
library.
json_data = response.json()
print(json_data)
Complete Example
Here’s a complete example that demonstrates these steps:
import requests
url = 'https://api.example.com/data'
response = requests.get(url)
json_data = response.json()
print(json_data)
Handling Different Types of JSON Data
JSON data can be nested, containing arrays and objects within other arrays or objects. Understanding these structures is essential for effective scraping.
Nested JSON Structures
Here’s an example of a nested JSON structure:
{
"user": {
"name": "John Doe",
"age": 30,
"addresses": [
{"street": "123 Main St", "city": "Anytown"},
{"street": "456 Elm St", "city": "Othertown"}
]
}
}
To access nested data, you can use Python’s dictionary and list indexing:
name = json_data['user']['name']
first_address = json_data['user']['addresses'][0]
print(f"Name: {name}")
print(f"First Address: {first_address}")
Arrays vs Objects in JSON
JSON arrays are equivalent to Python lists, while objects are equivalent to dictionaries. Here’s how you can handle both:
Arrays (Lists)
{
"fruits": ["apple", "banana", "cherry"]
}
Accessing array elements in Python:
first_fruit = json_data['fruits'][0]
print(first_fruit)
Objects (Dictionaries)
{
"person": {
"name": "John",
"age": 30
}
}
Accessing object elements in Python:
person_name = json_data['person']['name']
print(person_name)
Best Practices for Scraping JSON Data
While scraping data, it’s important to follow ethical guidelines and best practices. Here are a few tips:
Ethical Considerations and Legalities
- Respect Website Terms of Service: Ensure you have permission to scrape the data.
- Check Robots.txt: Some websites specify rules for web crawlers in their
robots.txt
file. Respect these rules.
Rate Limiting and Respecting robots.txt
To avoid overwhelming servers, implement rate limiting:
import time
url = 'https://api.example.com/data'
while True:
response = requests.get(url)
json_data = response.json()
print(json_data)
time.sleep(5) # Wait for 5 seconds before making the next request
Troubleshooting Common Issues
Web scraping can sometimes be tricky due to various challenges like CAPTCHAs, rate limits, and IP blocks. Here’s how you can handle some common issues:
Handling CAPTCHAs
CAPTCHAs are designed to prevent automated access. If a website uses CAPTCHAs, you might need human intervention or use CAPTCHA-solving services (though these come with ethical considerations).
Dealing with Rate Limits and IP Blocks
If your requests get blocked due to rate limits, try implementing exponential backoff:
import time
from random import randint
url = 'https://api.example.com/data'
while True:
response = requests.get(url)
if response.status_code == 200:
json_data = response.json()
print(json_data)
else:
backoff_time = randint(1, 3) # Random backoff time between 1 and 3 seconds
print(f"Rate limited. Retrying after {backoff_time} seconds.")
time.sleep(backoff_time)
FAQ Section
What is JSON data?
JSON (JavaScript Object Notation) is a lightweight format for storing and transporting data. It is easy to read and write, making it ideal for data interchange between different systems.
How do I install the required Python libraries?
You can install the necessary libraries using pip:
pip install requests beautifulsoup4
Is it legal to scrape JSON data from websites?
The legality of web scraping depends on various factors, including the website’s terms of service and local laws. Always check the website’s robots.txt
file and seek permission when necessary.
How can I handle nested JSON structures?
You can access nested JSON structures using Python’s dictionary and list indexing. For example:
name = json_data['user']['name']
print(name)
What should I do if my scraper gets blocked?
If your scraper gets blocked, consider implementing rate limiting, respecting the website’s robots.txt
file, and using exponential backoff strategies to avoid overwhelming the server.
In conclusion, scraping JSON data using Python is a powerful skill that can be applied in various scenarios. By following best practices and understanding the structure of JSON data, you can efficiently extract and manipulate the information you need. Happy scraping!