· Charlotte Will · webscraping · 5 min read
How to Automate Web Scraping with Selenium
Discover how to automate web scraping with Selenium in this comprehensive guide. Learn step-by-step instructions, best practices, and advanced techniques for efficient data extraction from dynamic websites. Perfect for both beginners and experienced developers.
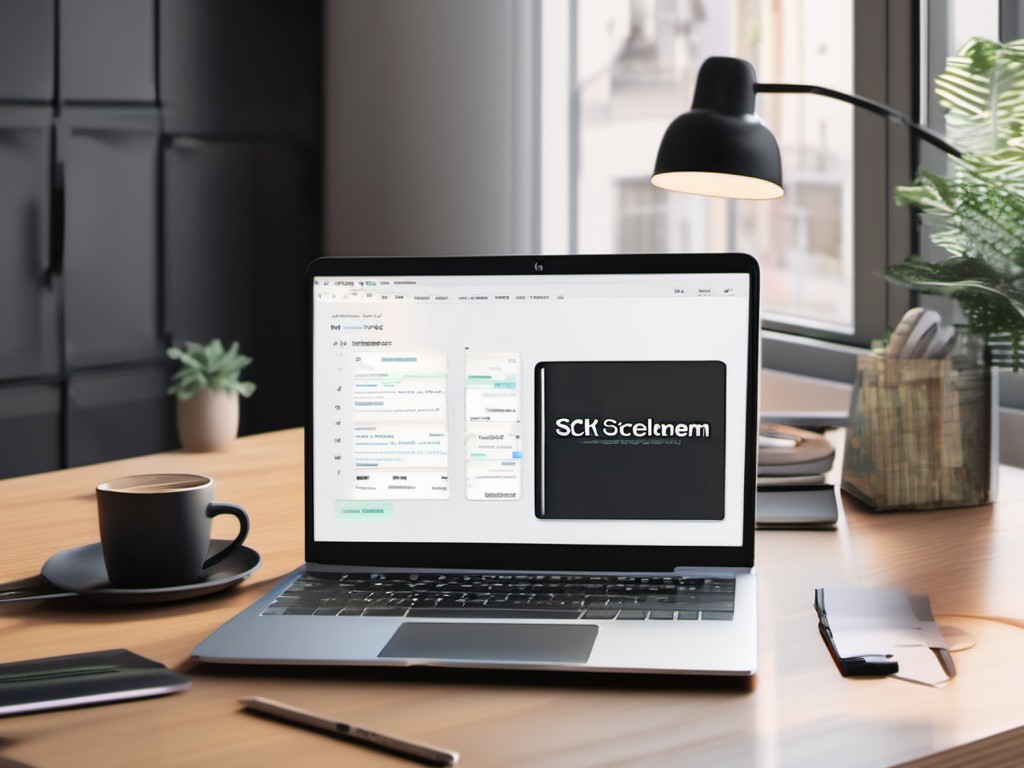
Web scraping has become an essential tool for data extraction and analysis in today’s digital age. Whether you are a data scientist, marketer, or developer, knowing how to automate web scraping can save you time and provide valuable insights. In this comprehensive guide, we will explore how to automate web scraping with Selenium. We’ll cover everything from setting up your environment to writing advanced scripts that handle dynamic content. Let’s dive in!
What is Selenium?
Selenium is an open-source tool that allows you to control web browsers through programs and perform browser automation. While it is primarily used for testing, its versatility makes it a powerful choice for web scraping as well. With Selenium, you can interact with web elements, fill out forms, click buttons, and extract data just like a human user would.
Setting Up Your Environment
Before you start automating web scraping with Selenium, you need to set up your development environment. Here’s how:
Installation of Selenium
First, ensure that Python is installed on your machine. Then, install the Selenium library via pip:
pip install selenium
Configuring Browsers
Selenium supports multiple browsers like Chrome, Firefox, and Edge. You’ll need to download the respective WebDriver for your browser of choice. For example, if you are using Google Chrome, download the ChromeDriver and place it in a directory that is included in your system’s PATH.
Writing Your First Script
Now that your environment is set up, let’s write our first web scraping script using Selenium.
Basic Web Scraping Example
We’ll start with a simple example: extracting the title of a webpage.
Import the necessary libraries:
from selenium import webdriver
Initialize the WebDriver:
driver = webdriver.Chrome()
Navigate to a website and extract data:
driver.get('https://www.example.com') title = driver.title print(title) driver.quit()
This basic script initializes the Chrome browser, navigates to example.com
, retrieves the page title, prints it, and then closes the browser.
Handling Dynamic Content
Websites often use JavaScript to load content dynamically. Selenium can wait for these elements to appear before interacting with them. Here’s how you can handle dynamic content:
Import WebDriverWait and expected_conditions:
from selenium.webdriver.common.by import By from selenium.webdriver.support.ui import WebDriverWait from selenium.webdriver.support import expected_conditions as EC
Use WebDriverWait to wait for elements:
driver = webdriver.Chrome() driver.get('https://www.example.com') try: element = WebDriverWait(driver, 10).until( EC.presence_of_element_located((By.ID, "dynamic-content")) ) print(element.text) finally: driver.quit()
In this example, the script waits up to 10 seconds for an element with the ID dynamic-content
to appear before extracting its text.
Advanced Selenium Techniques
To make your web scraping more robust and efficient, let’s explore some advanced techniques.
Handling Pagination
Many websites split data across multiple pages. You can automate the process of navigating through these pages with Selenium.
driver = webdriver.Chrome()
driver.get('https://www.example.com/page1')
while True:
# Extract data from the current page
elements = driver.find_elements(By.CLASS_NAME, 'data-item')
for element in elements:
print(element.text)
try:
next_button = driver.find_element(By.LINK_TEXT, 'Next')
next_button.click()
WebDriverWait(driver, 10).until(EC.staleness_of(next_button)) # Wait for the new page to load
except Exception as e:
print("No more pages")
break
driver.quit()
Using Proxies and Headless Mode
To avoid detection and speed up your scraping, you can use proxies and run Selenium in headless mode (without opening a browser window).
Add proxy configuration:
from selenium.webdriver.common.proxy import Proxy, ProxyType proxy = Proxy({ 'proxyType': ProxyType.MANUAL, 'httpProxy': 'your_proxy:port', 'ftpProxy': 'your_proxy:port', 'sslProxy': 'your_proxy:port', 'noProxy': '' # set this value as desired }) options = webdriver.ChromeOptions() options.proxy = proxy driver = webdriver.Chrome(options=options)
Run in headless mode:
options = webdriver.ChromeOptions() options.add_argument('--headless') driver = webdriver.Chrome(options=options)
Handling CAPTCHAs
CAPTCHAs are designed to prevent automated access, but there are ways around them:
- Third-party Services: Use services like 2Captcha or DeathByCaptcha to solve CAPTCHAs.
- Machine Learning Models: Train your own model to recognize and bypass CAPTCHAs (advanced users only).
Best Practices for Selenium Web Scraping
Respect Robots.txt
Always check the robots.txt
file of a website to see if they allow web scraping. Ignoring this can get you banned from accessing the site.
Rate Limiting and Retries
Implement rate limiting to avoid overloading servers, and use retries for handling temporary failures:
import time
from random import randint
driver = webdriver.Chrome()
retries = 3
for _ in range(retries):
try:
driver.get('https://www.example.com')
# Extract data here
break
except Exception as e:
time.sleep(randint(1, 5)) # Wait before retrying
Error Handling
Use try-except blocks to handle common errors gracefully and ensure your script continues running:
try:
driver.get('https://www.example.com')
element = driver.find_element(By.ID, 'some-id')
print(element.text)
except NoSuchElementException as e:
print("Element not found:", e)
finally:
driver.quit()
Conclusion
Automating web scraping with Selenium is a powerful way to extract data from the internet efficiently. By following this guide, you’ve learned how to set up your environment, write basic and advanced scripts, handle dynamic content, use proxies, and implement best practices. With these tools in hand, you are well-equipped to tackle even the most complex web scraping projects. Happy scraping!
FAQs
What are the legal considerations of web scraping?
Web scraping can have legal implications depending on the website’s terms of service and local laws. Always check the robots.txt
file and consult with a legal professional if you are unsure about the legality of your scraping activities.
How do I handle CAPTCHAs with Selenium?
CAPTCHAs can be handled using third-party services like 2Captcha or DeathByCaptcha, which offer APIs to solve CAPTCHAs programmatically. Alternatively, advanced users can train machine learning models to recognize and bypass CAPTCHAs.
Can Selenium run in headless mode?
Yes, Selenium supports running in headless mode, which means it runs without opening a browser window. This is useful for faster execution and avoiding detection. You can enable headless mode using ChromeOptions or FirefoxOptions.
What are the best practices for web scraping with Selenium?
Some best practices include respecting robots.txt
, implementing rate limiting, handling errors gracefully, and rotating proxies to avoid detection. Always aim to minimize the load on the target server and follow ethical guidelines.
How do I extract data from dynamically loaded content with Selenium?
To handle dynamic content, you can use WebDriverWait along with expected_conditions in Selenium. This allows your script to wait for specific elements to appear before interacting with them, ensuring that the content is fully loaded before extraction.