· Charlotte Will · webscraping · 5 min read
How to Make an API Call for Web Scraping
Learn how to make an API call for web scraping efficiently and legally. This comprehensive guide covers everything from setting up your environment to handling rate limits and advanced techniques like pagination and throttling. Ideal for beginners and intermediate developers looking to master web scraping using APIs.
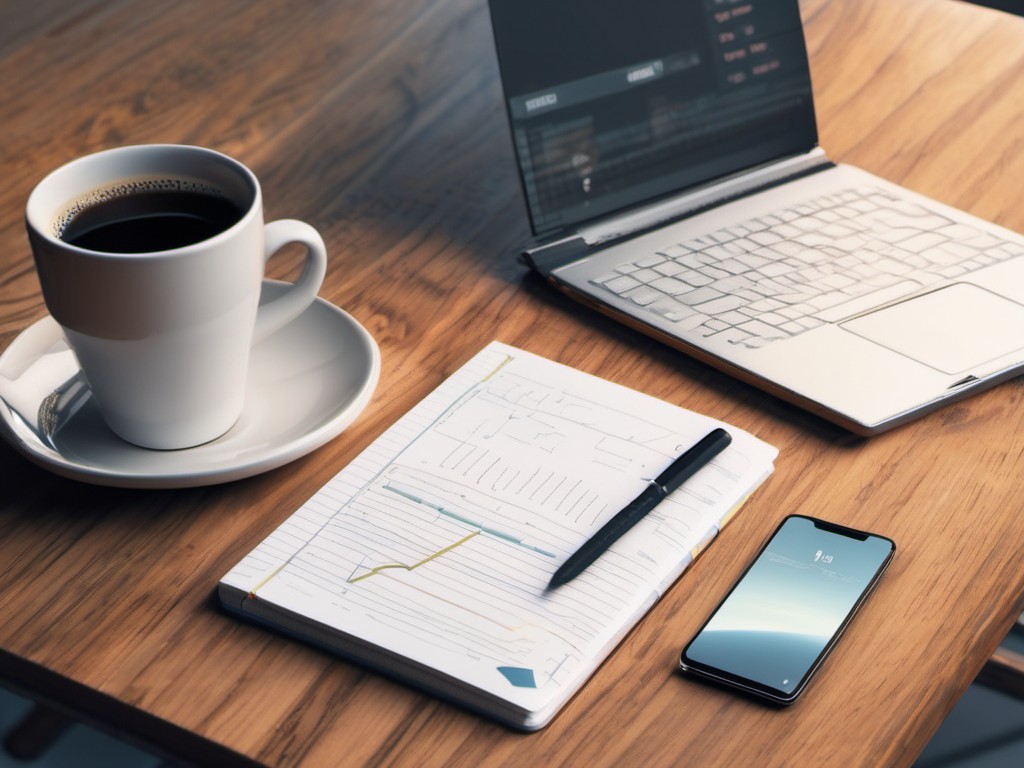
Web scraping is an essential skill for anyone looking to extract data from websites efficiently and accurately. While traditional methods involve parsing HTML, using APIs can often be a more reliable and straightforward approach. In this article, we’ll guide you through making an API call for web scraping, offering practical advice suitable for beginners and intermediate developers alike.
Why Use an API for Web Scraping?
Using an API for web scraping has several advantages:
- Efficiency: APIs often provide data in a structured format like JSON or XML, making it easier to parse.
- Reliability: APIs are less likely to change compared to the HTML structure of a website.
- Legality: Many websites offer public APIs that allow you to access their data legally and within their terms of service.
Understanding API Basics
Before diving into making an API call, let’s cover some basic concepts:
What is an API?
An Application Programming Interface (API) allows different software applications to communicate with each other. In the context of web scraping, APIs provide a way to retrieve data from a server in a structured format.
Types of APIs
- RESTful APIs: These use HTTP requests to perform CRUD operations (Create, Read, Update, Delete). Most web scraping tasks will involve RESTful APIs.
- GraphQL APIs: Unlike REST, GraphQL allows you to request exactly the data you need in a single query.
Getting Started with API Calls
1. Find the Right API
The first step is to identify an API that provides the data you need. Many websites offer public APIs; some popular examples include Twitter, GitHub, and OpenWeatherMap. You can usually find documentation on a website’s developer portal or by searching for “API documentation” followed by the site name.
2. Get Your API Key
Most APIs require an API key to authenticate your requests. This is typically provided when you sign up for the service. Some APIs offer free tiers with limited usage, which can be perfect for small-scale projects.
3. Set Up Your Development Environment
For this tutorial, we’ll use Python, a popular language for web scraping due to its simplicity and extensive libraries. You’ll need:
- Python installed on your computer (download here)
- A text editor or IDE like Visual Studio Code, PyCharm, or Sublime Text
4. Install Required Libraries
You’ll need the requests
library to make HTTP requests in Python. You can install it using pip:
pip install requests
Making Your First API Call
1. Import the Requests Library
Start by importing the requests
library into your Python script:
import requests
2. Define the Endpoint and Headers
The endpoint is the URL you’ll be making a request to. Headers often include your API key for authentication. Here’s an example using the OpenWeatherMap API:
endpoint = "http://api.openweathermap.org/data/2.5/weather"
headers = {
'x-rapidapi-key': 'YOUR_API_KEY',
'x-rapidapi-host': 'community-open-weather-map.p.rapidapi.com'
}
3. Make the Request
Use the requests.get()
function to make a GET request:
response = requests.get(endpoint, headers=headers)
4. Handle the Response
Check if the request was successful (status code 200), and then parse the JSON response:
if response.status_code == 200:
data = response.json()
print(data)
else:
print("Error:", response.status_code, response.text)
Parsing and Using the Data
Once you have the JSON data, you can parse it to extract the information you need. For example, if you want to get the current temperature from the OpenWeatherMap API:
temperature = data['main']['temp']
print(f"Current temperature is {temperature} K")
Handling Rate Limits and Errors
Rate Limiting
Most APIs have rate limits to prevent abuse. These are usually specified in the documentation. To handle rate limits, you can:
- Implement exponential backoff strategies.
- Use a library like
ratelimit
to manage your requests automatically.
Error Handling
Always include error handling to ensure your script doesn’t break unexpectedly. Common errors include invalid API keys, exceeding rate limits, and network issues. Here’s an example:
try:
response = requests.get(endpoint, headers=headers)
response.raise_for_status() # Raise exception for HTTP errors
except requests.exceptions.HTTPError as errh:
print ("Http Error:",errh)
except requests.exceptions.ConnectionError as errc:
print ("Error Connecting:",errc)
except requests.exceptions.Timeout as errt:
print ("Timeout Error:",errt)
except requests.exceptions.RequestException as err:
print ("OOps: Something Else",err)
Advanced Techniques for Web Scraping with APIs
Pagination
Some APIs return data in paginated format, meaning you get a limited number of results per request. To scrape all the data, you’ll need to handle pagination:
page = 1
while True:
params = {'page': page}
response = requests.get(endpoint, headers=headers, params=params)
if response.status_code != 200 or not response.json():
break
data = response.json()
# Process the data here
page += 1
Throttling Requests
To avoid hitting rate limits, you can throttle your requests using the time
library:
import time
for i in range(10):
response = requests.get(endpoint, headers=headers)
if response.status_code == 200:
data = response.json()
# Process the data here
time.sleep(60) # Wait for 60 seconds before making another request
Using Proxies
If you need to make a large number of requests, using proxies can help distribute your requests and avoid rate limits:
proxies = {
'http': 'http://10.10.1.10:3128',
'https': 'http://10.10.1.10:443',
}
response = requests.get(endpoint, headers=headers, proxies=proxies)
Conclusion
Making an API call for web scraping is a powerful and efficient way to extract data from websites. By understanding the basics of APIs, setting up your environment, making requests, and handling responses, you can automate the process of gathering information. Advanced techniques like pagination, throttling, and using proxies can help you scale your scraping projects effectively.
FAQs
1. What is an API key?
An API key is a unique identifier used to authenticate requests to an API. It ensures that only authorized users can access the data.
2. How do I find out if a website has a public API?
Check the website’s developer portal or documentation section. Many websites have dedicated pages for their APIs, including usage guides and examples.
3. What is JSON and why is it used in APIs?
JSON (JavaScript Object Notation) is a lightweight data interchange format that is easy for humans to read and write, and easy for machines to parse and generate. It’s commonly used in APIs because of its simplicity and compatibility with various programming languages.
4. How can I handle rate limits when using an API?
You can implement exponential backoff strategies, use a library like ratelimit
, or simply throttle your requests by adding delays between them to stay within the allowed limit.
5. Can I scrape data without using an API?
Yes, you can scrape data directly from HTML pages using libraries like BeautifulSoup and Scrapy in Python. However, APIs offer a more structured and reliable way to access data.