· Charlotte Will · webscraping · 6 min read
How to Extract Data from JSON APIs for Web Scraping
Learn how to extract data from JSON APIs for web scraping with our comprehensive guide. Discover practical steps, best practices, and troubleshooting tips to master this technique and improve your data extraction efficiency. Perfect for both beginners and intermediate users.
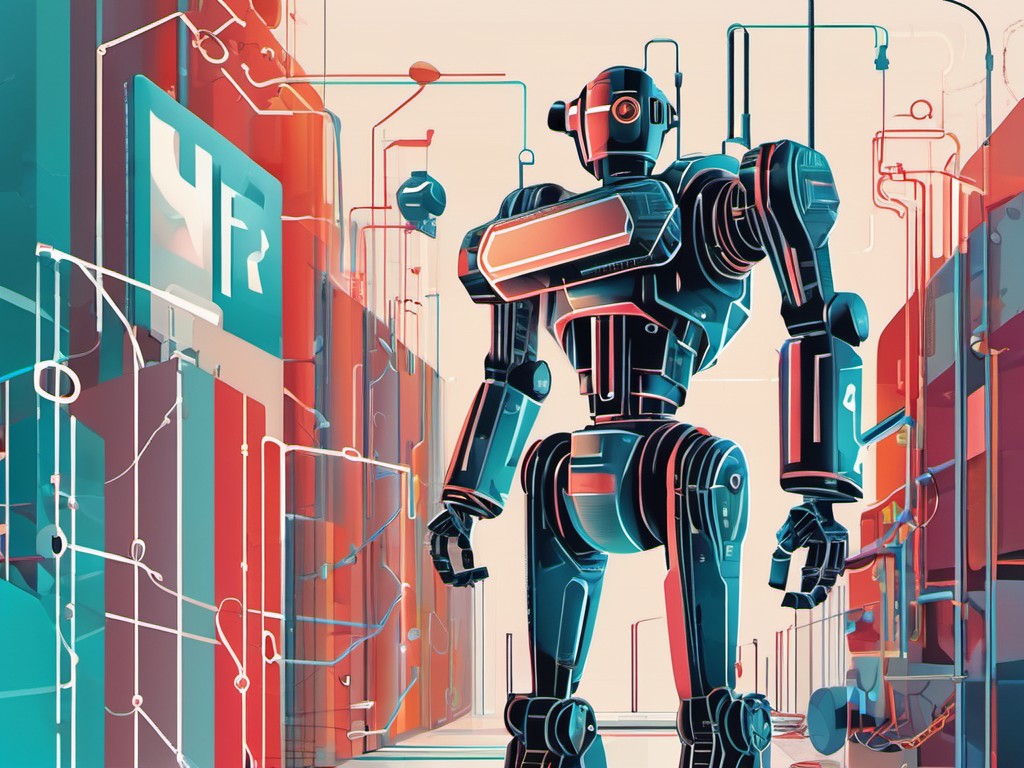
Web scraping has become an essential skill for developers, data scientists, and analysts who need to extract information from websites. One efficient method to achieve this is by leveraging JSON APIs. In this comprehensive guide, we will explore how to extract data from JSON APIs for web scraping, providing practical steps and best practices to help both beginners and intermediate users master this technique.
Understanding JSON APIs
Before diving into the extraction process, it’s essential to understand what JSON APIs are. JSON (JavaScript Object Notation) is a lightweight data interchange format that is easy for humans to read and write and easy for machines to parse and generate. An API (Application Programming Interface) allows different software applications to communicate with each other. A JSON API thus provides data in JSON format, making it easier to handle compared to traditional HTML scraping.
Why Use JSON APIs for Web Scraping?
Using JSON APIs for web scraping offers several advantages:
- Efficiency: APIs provide structured data that is much easier and faster to parse than unstructured HTML content.
- Reliability: APIs are less likely to change their structure compared to the constantly evolving HTML of a website.
- Legal Compliance: Using APIs can sometimes be more legally compliant, as they often provide terms of service that outline acceptable usage.
Step-by-Step Guide to Extract Data from JSON APIs
Prerequisites
Before you begin, ensure you have the following tools installed:
- A code editor (such as Visual Studio Code)
- Python (preferably version 3.x)
requests
library for making HTTP requests in Pythonjson
library to handle JSON data
You can install the required libraries using pip:
pip install requests
Step 1: Identify the JSON API Endpoint
The first step is to identify the JSON API endpoint you want to scrape. This information is usually available in the website’s documentation or can be discovered through browser developer tools (F12).
Step 2: Make an HTTP Request to the API
Using Python, you can make an HTTP request to the API using the requests
library. Here’s a basic example of how to do this:
import requests
url = "https://api.example.com/data"
response = requests.get(url)
# Check if the request was successful
if response.status_code == 200:
data = response.json()
print(data)
else:
print("Error:", response.status_code)
Step 3: Parse the JSON Data
Once you have the JSON response, you can parse it using Python’s built-in json
library. The data is typically a dictionary or list of dictionaries, which makes it easy to access specific information.
import requests
import json
url = "https://api.example.com/data"
response = requests.get(url)
if response.status_code == 200:
data = response.json()
print("Data:", json.dumps(data, indent=4)) # Pretty-print the JSON data
else:
print("Error:", response.status_code)
Step 4: Extract Relevant Data
Now that you have parsed the JSON data, you can extract relevant information based on your needs. For example, if you’re scraping a weather API, you might be interested in temperature and humidity levels.
import requests
url = "https://api.example.com/weather"
response = requests.get(url)
if response.status_code == 200:
data = response.json()
for item in data['items']:
print("Temperature:", item['temperature'])
print("Humidity:", item['humidity'])
else:
print("Error:", response.status_code)
Step 5: Handle Pagination (if necessary)
Some APIs return data in paginated form, meaning you’ll need to make multiple requests to gather all the data. Check the API documentation for details on handling pagination. Here’s an example of how to handle it programmatically:
import requests
base_url = "https://api.example.com/data"
params = {'page': 1, 'limit': 50}
all_data = []
while True:
response = requests.get(base_url, params=params)
if response.status_code != 200:
break
data = response.json()
all_data.extend(data['items'])
# Check if there's a next page
if 'next' in response.links:
params['page'] += 1
else:
break
# Process the collected data
print("Collected Data:", json.dumps(all_data, indent=4))
Best Practices for JSON API Web Scraping
1. Respect Rate Limits and Terms of Service
Always check the API’s rate limits and terms of service to ensure you are compliant with their usage policies. Exceeding rate limits can lead to your IP being blocked.
2. Implement Error Handling
Handle HTTP errors gracefully by implementing try-except blocks. This will prevent your script from crashing unexpectedly.
import requests
try:
response = requests.get(url)
data = response.json()
# Process the data
except requests.exceptions.RequestException as e:
print("Error:", e)
3. Use Headers for Authentication
Many APIs require authentication headers to access their data. Ensure you include any necessary headers in your request.
headers = {'Authorization': 'Bearer YOUR_API_KEY'}
response = requests.get(url, headers=headers)
4. Store Data Efficiently
If you are extracting large amounts of data, consider storing it efficiently in a database or using data serialization formats like CSV or Parquet for further analysis.
Common Issues and Troubleshooting
Handling API Rate Limits
API providers often impose rate limits to prevent abuse. If you hit the limit, you might receive a 429 Too Many Requests
status code. Implement exponential backoff to retry requests with increasing delays between attempts.
import time
def fetch_data(url):
while True:
response = requests.get(url)
if response.status_code == 200:
return response.json()
elif response.status_code == 429:
retry_after = int(response.headers['Retry-After'])
time.sleep(retry_after)
else:
print("Error:", response.status_code)
break
Dealing with API Changes
API structures can change over time, which might break your scraping script. Regularly check the API documentation and handle versioning in your requests if supported by the API.
url = "https://api.example.com/v1/data"
response = requests.get(url)
Conclusion
Extracting data from JSON APIs for web scraping is a powerful and efficient method to gather information from the internet. By following the steps outlined above and adhering to best practices, you can create robust scripts that handle various API scenarios. Remember to always respect the API’s terms of service and rate limits to ensure sustainable and legal data extraction.
FAQs
1. How do I find the endpoint for a JSON API?
You can usually find the endpoint in the website’s documentation or by inspecting network traffic using browser developer tools (F12).
2. What should I do if the API returns data in paginated form?
Handle pagination programmatically by making multiple requests, typically by iterating through pages and appending results. Check the API documentation for specific details on pagination.
3. How can I respect rate limits when scraping data from APIs?
Implement exponential backoff to retry requests with increasing delays between attempts if you receive a 429 Too Many Requests
status code.
4. What should I do if the API structure changes?
Regularly check the API documentation for any updates or changes in structure. Implement versioning in your requests if supported by the API to ensure compatibility with different versions.
5. How can I handle authentication when making API requests?
Include the necessary headers, such as an API key, in your request to authenticate and access protected data. Refer to the API documentation for specific authentication methods.
In addition to extracting data from JSON APIs, it’s important to understand how to create custom APIs for more tailored web scraping projects. You can find more information on this topic in our guide How to Create Custom APIs for Data Integration with Web Scraping. If you’re looking to integrate APIs into your web scraping project using Python, check out our comprehensive guide How to Integrate APIs into Your Web Scraping Project Using Python. For advanced users, discover how to build custom web scraping APIs for data integration in our detailed article Building Custom Web Scraping APIs for Data Integration.