· Charlotte Will · webscraping · 6 min read
Building Custom Web Scraping APIs for Data Integration
Discover how to build custom web scraping APIs for seamless data integration, automation tools, and real-time extraction. Enhance business intelligence with tailored solutions in Python.
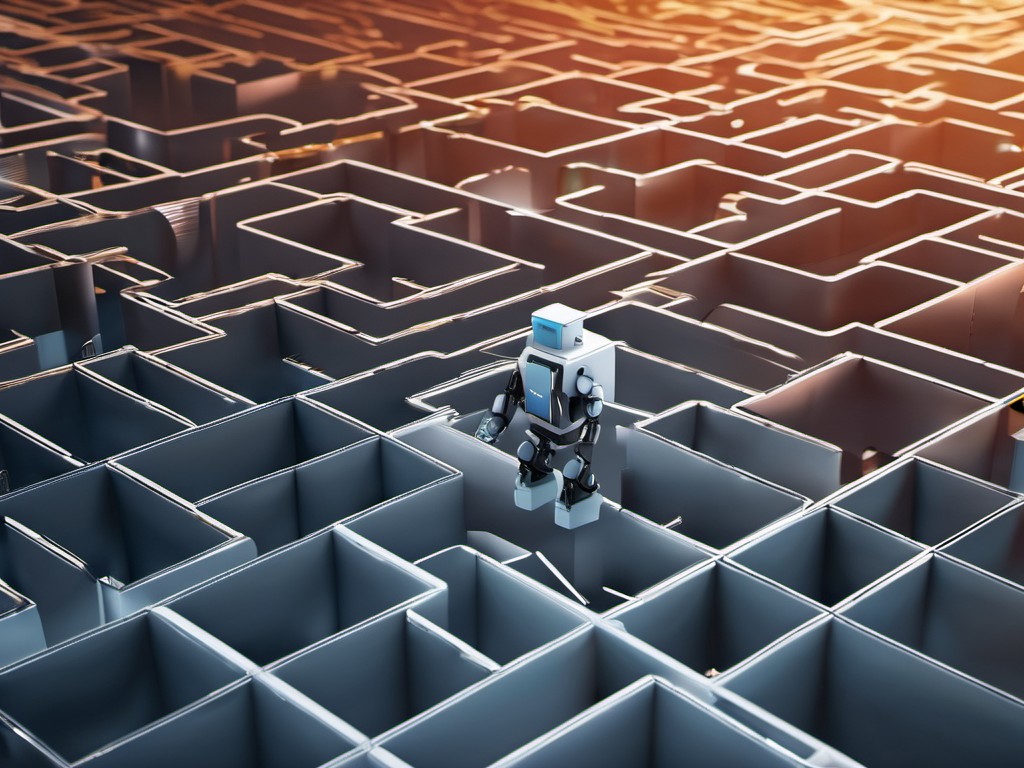
In today’s data-driven world, businesses rely on timely and accurate information to make informed decisions. One of the most effective ways to gather this data is through web scraping—extracting structured data from websites. However, manually scraping websites can be time-consuming and error-prone. This is where custom web scraping APIs come into play. By automating the process, these APIs can streamline data integration, making it a critical tool for business intelligence.
Understanding Web Scraping APIs
Web scraping APIs are tools that allow you to extract data from websites in a programmatic way. Unlike traditional web scraping methods, which often involve manually copying and pasting data, APIs provide a structured and automated approach to data extraction. This automation can significantly increase the efficiency and accuracy of your data collection processes.
Why Build Custom Web Scraping APIs?
While there are many off-the-shelf web scraping tools available, building custom APIs offers several advantages:
Tailored to Your Needs
Custom APIs can be designed specifically to meet your unique business requirements. This ensures that you get the exact data you need in the format that works best for your systems.
Enhanced Security and Privacy
With custom APIs, you have full control over how data is handled. You can implement security measures tailored to your needs, ensuring that sensitive information remains protected.
Real-Time Data Extraction
Custom web scraping APIs can be configured to provide real-time data extraction, allowing you to stay up-to-date with the latest information without delays.
Steps in Developing a Custom Web Scraping API
1. Define Your Requirements
The first step is to clearly define what data you need and how it will be used. This includes identifying specific websites, determining the frequency of data collection, and deciding on the format for the extracted data (e.g., JSON, XML).
2. Choose the Right Tools and Technologies
Selecting the right tools is crucial. Python is a popular choice for web scraping due to its simplicity and powerful libraries like BeautifulSoup and Scrapy. For API development, frameworks such as Flask or Django can be very helpful.
3. Write the Scraper Code
This involves writing the actual code to extract data from websites. You’ll need to handle various challenges, including dealing with different website structures, handling JavaScript-rendered content, and respecting robots.txt rules.
4. Develop the API Endpoints
Once you have your scraper code ready, the next step is to develop the API endpoints. These endpoints will allow other applications to request data from your web scraper. You’ll need to decide on the HTTP methods (GET, POST, etc.) and design the request/response structure.
5. Testing and Debugging
Thoroughly test your API to ensure it is returning the correct data. This includes testing for edge cases, handling errors gracefully, and optimizing performance.
6. Deployment and Maintenance
Deploy your API to a reliable server or cloud platform. Regular maintenance is essential to keep your API up-to-date with changes in website structures and to ensure continued accuracy and reliability.
Best Practices for Maintaining Custom Web Scraping APIs
1. Monitor Changes in Website Structure
Websites frequently update their layout and structure, which can break your scraper. Regularly monitor the websites you scrape to identify and address any changes promptly.
2. Handle Errors Gracefully
Implement error handling mechanisms to manage situations where data extraction fails. This could involve retries, fallback mechanisms, or alerting systems to notify you of issues.
3. Optimize Performance
Efficiently scrape and process data to minimize load times. Use techniques like caching to store frequently requested data and reduce the need for repeated scrapes.
Integrating Custom Web Scraping APIs into Existing Systems
Once your API is built, you’ll want to integrate it into your existing systems to make use of the collected data. This could involve:
1. Data Synchronization
Ensure that the data extracted by your API is synchronized with your databases and other systems in real-time or at scheduled intervals.
2. Automation Tools
Use automation tools to trigger data extraction based on specific events or schedules. This can help maintain up-to-date information without manual intervention.
3. Business Intelligence
Integrate the extracted data into your business intelligence systems to gain insights and make data-driven decisions.
Practical Example: Building a Custom Web Scraping API with Python
Let’s walk through a simple example of building a custom web scraping API using Python, Flask, and BeautifulSoup.
Step 1: Install Dependencies
First, install the necessary libraries:
pip install flask beautifulsoup4 requests
Step 2: Write the Scraper Code
Create a script to extract data from a website using BeautifulSoup.
import requests
from bs4 import BeautifulSoup
def scrape_website(url):
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
# Extract specific data from the website
title = soup.title.string
return {'title': title}
Step 3: Develop the API Endpoints with Flask
Create a simple Flask application to handle HTTP requests.
from flask import Flask, jsonify, request
import scraper_module # Assume the above script is saved in scraper_module.py
app = Flask(__name__)
@app.route('/scrape', methods=['GET'])
def scrape():
url = request.args.get('url')
data = scrape_website(url)
return jsonify(data)
if __name__ == '__main__':
app.run(debug=True)
Step 4: Test Your API
Run the Flask application and test your endpoint by sending a GET request with a URL parameter. For example, using curl
:
curl "http://127.0.0.1:5000/scrape?url=https://example.com"
This should return the extracted data in JSON format.
Conclusion
Building custom web scraping APIs offers numerous benefits for data integration, including tailored solutions, enhanced security, and real-time data extraction. By following best practices and leveraging powerful tools like Python, you can create efficient and effective APIs that meet your business needs. Whether you’re extracting competitor pricing, monitoring social media trends, or gathering market insights, custom web scraping APIs are a powerful tool in the modern data landscape.
FAQs
Q1: What is the difference between a custom web scraping API and an off-the-shelf solution?
A: Custom web scraping APIs are tailored to your specific requirements, offering greater flexibility, security, and control over how data is extracted and used. Off-the-shelf solutions provide quick setup but may lack the customization needed for complex use cases.
Q2: How do I handle changes in website structure that break my scraper?
A: Regularly monitor the websites you scrape and implement error handling mechanisms to detect when data extraction fails. You can also use tools like ChangeTower to get notified of structural changes on target websites.
Q3: Can custom web scraping APIs provide real-time data?
A: Yes, custom web scraping APIs can be configured to provide real-time data extraction. This involves setting up the API to continually monitor and extract data as it becomes available on the source website.
Q4: What are some common challenges in building custom web scraping APIs?
A: Common challenges include handling JavaScript-rendered content, respecting robots.txt rules, dealing with dynamic website structures, and ensuring the accuracy and reliability of extracted data over time.
Q5: How do I maintain and update my custom web scraping API?
A: Regular maintenance involves monitoring changes in target websites, optimizing performance, handling errors gracefully, and updating your code to adapt to new challenges or requirements. Scheduled checks and automated tests can help ensure continued reliability.