· Charlotte Will · webscraping · 6 min read
Building a Custom Proxy Rotator for Enhanced Web Scraping
Discover how to build a custom proxy rotator to enhance your web scraping capabilities. Learn advanced techniques like proxy health checks and load balancing. Boost your scraping efficiency and stealth with our comprehensive guide on Python-based proxy management.
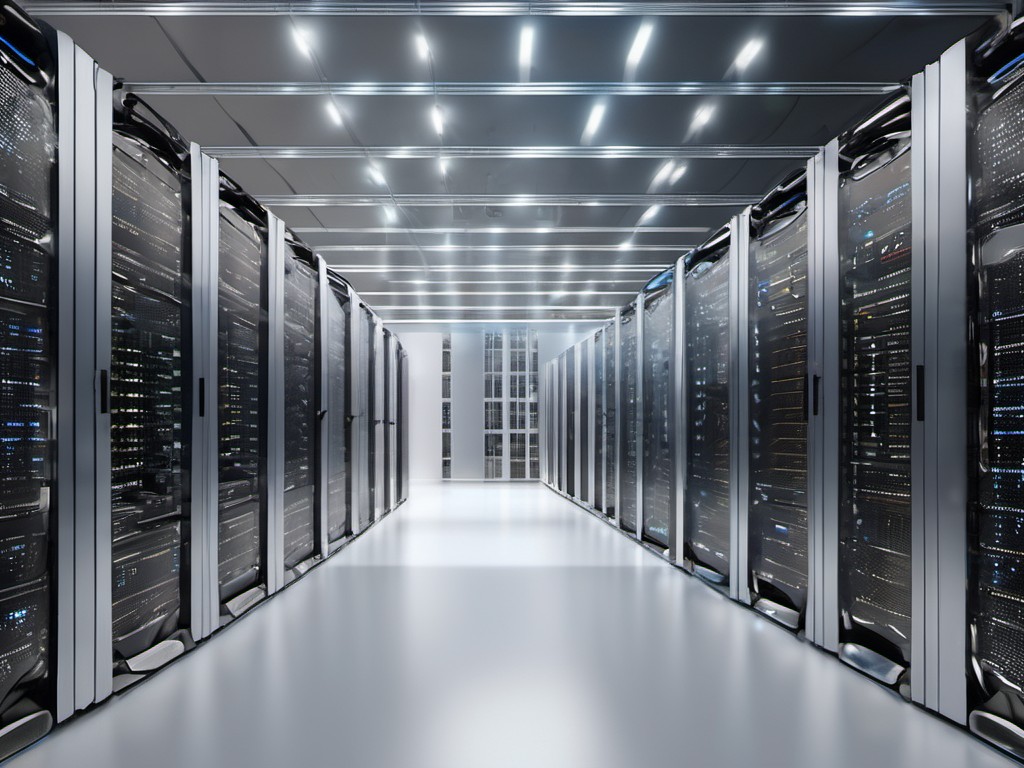
Web scraping is an essential technique in today’s data-driven world, allowing businesses and developers to extract valuable information from websites. However, as web administrators become more adept at detecting and blocking scraping activities, the use of proxies has emerged as a crucial strategy for maintaining stealth and ensuring the continuity of scraping operations.
In this article, we’ll delve into the art of building a custom proxy rotator to enhance your web scraping capabilities. We’ll cover the fundamentals of proxy management, explore advanced techniques for optimizing proxy rotation in Python, and provide practical tips to ensure robust and efficient data extraction.
Understanding Proxy Rotation
Proxy rotation involves using multiple proxies during a scraping session to distribute requests across various IP addresses. This practice helps evade detection and prevents your IP from being banned by the target website. By rotating proxies, you can maintain a lower profile and increase the longevity of your scraping efforts.
Why Use Proxy Rotation?
- Avoid Detection: Websites often implement measures to detect and block suspicious activity from a single IP address.
- Maintain Stealth: Rotating proxies helps in avoiding detection mechanisms that flag repeated requests from the same source.
- Enhance Scraping Speed: By distributing requests across multiple proxies, you can parallelize your scraping tasks and speed up data collection.
Building a Custom Proxy Rotator
To build an effective custom proxy rotator, we’ll use Python as our programming language. Python’s rich ecosystem of libraries makes it an ideal choice for web scraping and proxy management.
Requirements
Before diving into the code, ensure you have the following libraries installed:
requests
: For making HTTP requests.BeautifulSoup
: For parsing HTML content.concurrent.futures
: For managing concurrent tasks.
You can install these using pip:
pip install requests beautifulsoup4
Setting Up Your Proxy List
Maintain a list of proxies in a text file, with each line containing an IP address and port number, separated by a colon. Here’s an example of what your proxies.txt
might look like:
192.168.0.1:8080
10.0.0.1:3128
172.16.0.1:8080
Reading Proxies from a File
First, let’s write a function to read proxies from the file and store them in a list.
def read_proxies(filepath):
with open(filepath, 'r') as f:
proxies = [line.strip() for line in f]
return proxies
Proxy Rotation Function
Next, we’ll create a function to rotate through the list of proxies. This function will use a simple round-robin technique to distribute requests evenly among the available proxies.
def get_next_proxy(proxies):
proxy_pool = itertools.cycle(proxies)
return next(proxy_pool)
Implementing the Scraper
Now, let’s implement a basic scraper that uses our proxy rotator function to fetch data from a website. We’ll use requests
to make HTTP requests and BeautifulSoup
to parse the HTML content.
import requests
from bs4 import BeautifulSoup
def scrape_website(url, proxies):
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/89.0.4389.82 Safari/537.36'
}
while True:
proxy = get_next_proxy(proxies)
try:
response = requests.get(url, headers=headers, proxies={"http": proxy, "https": proxy})
if response.status_code == 200:
soup = BeautifulSoup(response.content, 'html.parser')
# Extract the desired data here
print(soup.prettify())
except requests.exceptions.RequestException as e:
print(f"Error using proxy {proxy}: {e}")
Putting It All Together
Finally, let’s put everything together in a script that reads proxies from a file and uses them to scrape data from a website.
import itertools
proxies = read_proxies('proxies.txt')
url = 'https://example.com' # Replace with the target URL
scrape_website(url, proxies)
Advanced Proxy Management Techniques
To further enhance your web scraping capabilities, consider implementing the following advanced techniques:
Proxy Health Checks
Periodically check the health of your proxies to ensure they are still active and functional. This can be done by making a simple HTTP request to a known endpoint and verifying the response.
def is_proxy_healthy(proxy):
try:
response = requests.get('https://www.google.com', proxies={"http": proxy, "https": proxy}, timeout=5)
return response.status_code == 200
except requests.exceptions.RequestException:
return False
Backoff and Retry Logic
Implement a backoff and retry mechanism to handle temporary failures or bans. This involves retrying failed requests with increasing delays between attempts.
import time
from random import expovariate
def exponential_backoff(retries=5, base_delay=0.1):
for i in range(retries):
delay = expovariate(base_delay)
time.sleep(delay)
Load Balancing with Concurrent Requests
Leverage concurrency to make multiple requests simultaneously, improving scraping speed and efficiency. Use Python’s concurrent.futures
module for this purpose.
from concurrent.futures import ThreadPoolExecutor
def concurrent_scrape(url, proxies):
with ThreadPoolExecutor(max_workers=5) as executor:
futures = [executor.submit(scrape_single_page, url, proxy) for proxy in proxies]
for future in futures:
try:
print(future.result())
except Exception as e:
print(f"Error: {e}")
Best Practices for Proxy Management
- Maintain a Diverse Proxy Pool: Use proxies from different providers and locations to avoid detection.
- Rotate Proxies Regularly: Implement random or round-robin rotation to distribute requests evenly.
- Monitor Proxy Performance: Keep track of proxy health and performance metrics to ensure optimal usage.
- Respect Robots.txt: Always follow the rules specified in a website’s
robots.txt
file to avoid legal issues. - Implement Rate Limiting: Limit the number of requests per IP address to avoid triggering anti-scraping measures.
Conclusion
Building a custom proxy rotator is an essential skill for any web scraper looking to enhance their capabilities and maintain stealth. By implementing advanced techniques such as proxy health checks, backoff and retry logic, and concurrent requests, you can significantly improve the efficiency and reliability of your scraping operations.
For further reading on advanced web scraping techniques, see our guide on Building Custom Web Scraping APIs for Data Integration. Additionally, if you’re interested in building a robust web crawler, check out our detailed article on Building a Custom Web Crawler with Python for Advanced Scraping Needs.
FAQs
What is the difference between HTTP and HTTPS proxies?
- HTTP proxies only support unencrypted traffic, while HTTPS proxies can handle encrypted traffic as well. It’s recommended to use HTTPS proxies for web scraping to ensure data security.
How often should I rotate my proxies?
- The optimal proxy rotation frequency depends on the target website and its anti-scraping measures. A common practice is to rotate proxies every 10-15 requests or after a certain period (e.g., every minute).
Can I use free proxies for web scraping?
- While it’s possible to use free proxies, they are often unreliable and can lead to IP bans. It’s recommended to invest in high-quality proxy services that provide stable and secure connections.
How do I handle CAPTCHA challenges during web scraping?
- CAPTCHA challenges can be difficult to bypass programmatically. Some options include using third-party CAPTCHA solving services, employing human solvers, or implementing machine learning techniques to automate the process.
What should I do if my IP gets banned during web scraping?
- If your IP gets banned, you can try using a different proxy, wait for some time before retrying, or implement a backoff and retry mechanism with increasing delays between attempts. Additionally, consider rotating user agents and adjusting request headers to mimic human-like behavior.