· Charlotte Will · webscraping · 5 min read
Building a Custom Web Crawler with Python for Advanced Scraping Needs
Learn how to build a custom web crawler using Python for advanced web scraping needs. This guide covers prerequisites, basic structure, handling requests and responses, parsing HTML with BeautifulSoup, implementing crawling logic, and advanced techniques like handling JavaScript-rendered content, rate limiting, error handling, and optimizing performance with asynchronous web scraping.
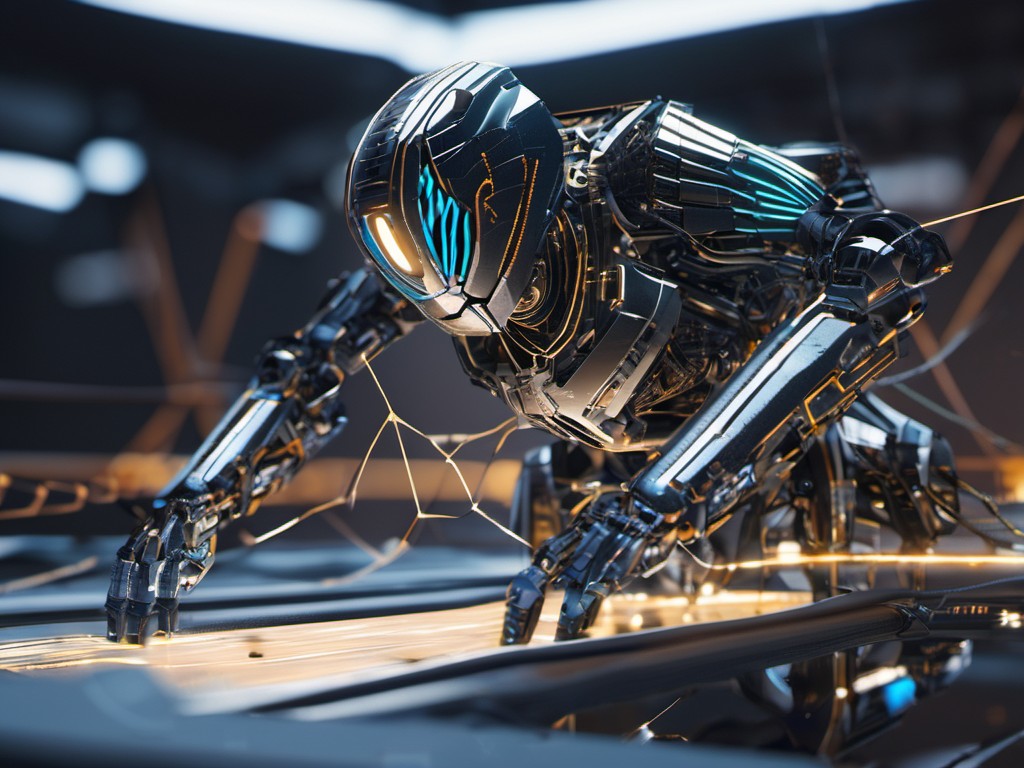
Introduction
In today’s data-driven world, web scraping has become an essential tool for extracting valuable information from websites. While there are numerous pre-built web crawlers and APIs available, sometimes these tools fall short of meeting specific needs. That’s where building a custom web crawler comes into play. Python, with its rich ecosystem of libraries, is a powerful tool for creating tailor-made web scraping solutions. This article will guide you through the process of building an advanced custom web crawler using Python.
Why Build a Custom Web Crawler?
Building a custom web crawler offers several advantages over pre-built solutions. Firstly, it allows for greater flexibility and control over the scraping process. You can fine-tune the crawler to suit your specific requirements, such as targeting particular types of data or handling complex website structures. Additionally, a custom web crawler can be more efficient and less resource-intensive than generic tools, which often come with unnecessary features.
Advanced scraping techniques require a deeper understanding of both the websites being scraped and the underlying technologies powering them. A custom web crawler enables you to implement sophisticated strategies for extracting data from dynamic content, managing rate limits, and ensuring data integrity. By leveraging Python’s robust libraries and frameworks, you can create a highly optimized and effective web scraper tailored to your advanced scraping needs.
Prerequisites and Setup
Before diving into the code, it is essential to set up the necessary libraries and tools. Here are the prerequisites for building a custom web crawler with Python:
Python: Ensure that you have Python installed on your machine. You can download the latest version from python.org.
Libraries: Install the following libraries using pip:
pip install requests beautifulsoup4 selenium asyncio aiohttp
Development Environment: Set up your preferred development environment, such as Visual Studio Code or Jupyter Notebook.
Building the Custom Web Crawler
Basic Structure
A basic web crawler consists of several key components:
- URL Fetcher: Responsible for sending HTTP requests to fetch web pages.
- HTML Parser: Extracts data from the fetched HTML content.
- Crawling Logic: Navigates through links and determines which pages to crawl next.
Handling Requests and Responses
To send HTTP requests and handle responses, we’ll use the requests
library:
import requests
def fetch_url(url):
response = requests.get(url)
if response.status_code == 200:
return response.text
else:
print(f"Failed to retrieve {url}")
return None
Parsing HTML with BeautifulSoup
For parsing HTML and extracting data, BeautifulSoup
from the bs4
library is an excellent tool:
from bs4 import BeautifulSoup
def parse_html(html):
soup = BeautifulSoup(html, 'html.parser')
# Example: Extract all links on the page
for link in soup.find_all('a'):
print(link.get('href'))
Implementing Crawling Logic
The crawling logic involves keeping track of visited URLs and exploring new ones:
import set
def crawl(start_url):
visited = set()
to_visit = [start_url]
while to_visit:
url = to_visit.pop()
if url in visited:
continue
html = fetch_url(url)
if not html:
continue
print(f"Visiting {url}")
visited.add(url)
# Extract new links from the current page
for link in parse_html(html):
to_visit.append(link)
Advanced Techniques
Handling JavaScript-Rendered Content
Modern websites often rely on JavaScript to render content dynamically. To handle such cases, we can use Selenium:
from selenium import webdriver
from selenium.webdriver.common.by import By
import time
def fetch_dynamic_content(url):
driver = webdriver.Chrome()
driver.get(url)
time.sleep(5) # Allow time for JavaScript to execute
html = driver.page_source
driver.quit()
return html
Rate Limiting and Politeness Policy
Respecting a website’s terms of service is crucial. Implement rate limiting to avoid overwhelming the server:
import time
def fetch_url(url):
response = requests.get(url)
if response.status_code == 200:
time.sleep(1) # Rate limit: sleep for 1 second before the next request
return response.text
else:
print(f"Failed to retrieve {url}")
return None
Error Handling and Retries
Robust error handling ensures data integrity during the scraping process:
import requests
from requests.exceptions import RequestException
def fetch_url(url, retries=3):
for attempt in range(retries):
try:
response = requests.get(url)
if response.status_code == 200:
return response.text
except RequestException as e:
print(f"Request failed: {e}")
time.sleep(1) # Wait before retrying
print(f"Failed to retrieve {url} after {retries} attempts")
return None
Optimizing Performance
Asynchronous Web Scraping with AsyncIO and Aiohttp
To improve performance, consider using asynchronous web scraping:
import aiohttp
import asyncio
async def fetch(session, url):
async with session.get(url) as response:
return await response.text()
async def main():
urls = ['https://example1.com', 'https://example2.com']
async with aiohttp.ClientSession() as session:
tasks = [fetch(session, url) for url in urls]
htmls = await asyncio.gather(*tasks)
for html in htmls:
print(html[:100]) # Print the first 100 characters of each page
asyncio.run(main())
Efficient Data Storage and Processing
Use efficient data storage solutions like databases or CSV files to handle large datasets:
import csv
def save_to_csv(data, filename):
with open(filename, 'w', newline='') as file:
writer = csv.writer(file)
writer.writerow(['URL', 'Title']) # Example header row
for url, title in data:
writer.writerow([url, title])
Internal Linking Section
For further insights into advanced techniques for web scraping, refer to our article Advanced Techniques for Python Web Scraping. Additionally, if you are interested in integrating APIs into your web scraping projects, check out our guide How to Integrate APIs into Your Web Scraping Project Using Python.
Conclusion
Building a custom web crawler with Python provides the flexibility and control needed for advanced scraping needs. By leveraging powerful libraries like requests
, BeautifulSoup
, and Selenium
, you can create tailored solutions that efficiently extract valuable data from websites. Implementing best practices such as rate limiting, error handling, and asynchronous processing enhances the performance and reliability of your web crawler.
FAQs
Why is it important to respect a website’s terms of service while scraping?
- Respecting a website’s terms of service ensures that you are not overloading their servers with requests, which could lead to legal issues or your IP being blocked.
How can I handle dynamic content rendered by JavaScript?
- You can use tools like Selenium to render JavaScript and extract dynamically generated content from web pages.
What is the purpose of rate limiting in web scraping?
- Rate limiting helps prevent overwhelming a website’s server with too many requests in a short period, ensuring that your scraper operates within acceptable usage limits.
Why use asynchronous web scraping?
- Asynchronous web scraping allows you to send multiple requests concurrently, significantly improving the speed and efficiency of your scraper compared to synchronous requests.
How can I ensure data integrity during the web scraping process?
- Implementing robust error handling and retry mechanisms helps in ensuring that your scraper gracefully handles failures and maintains data integrity throughout the scraping process.