· Charlotte Will · webscraping · 5 min read
Advanced Techniques for Extracting Embedded Media from Websites
Learn advanced techniques for extracting embedded media from websites using BeautifulSoup, lxml, Selenium, and Puppeteer. This comprehensive guide covers dynamic content handling, proxy management, real-time data extraction, and ethical considerations in web scraping.
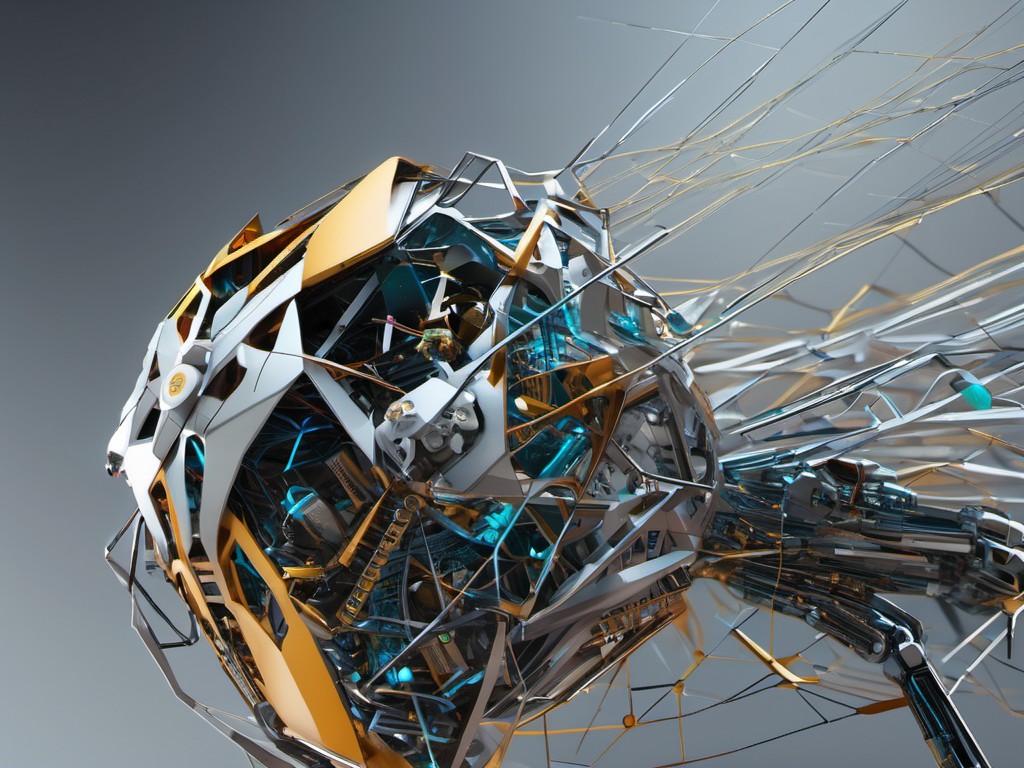
Welcome to the comprehensive guide on advanced techniques for extracting embedded media from websites. As the digital landscape evolves, the importance of web scraping and data extraction continues to grow. This article will delve into the intricate world of embedding media extraction, equipping you with practical tools and strategies to master this skill.
Introduction
Web scraping has become an essential tool for businesses and individuals looking to extract valuable data from websites. Among the various types of data, embedded media such as images, videos, and audio files are particularly sought after. This guide will walk you through advanced techniques for extracting embedded media from websites, focusing on efficiency, accuracy, and ethical considerations.
Understanding Embedded Media Extraction
Embedded media extraction involves fetching multimedia content directly embedded within web pages. This can include images, videos, audio files, and even interactive elements like maps or graphs. Understanding the structure of a website is crucial before you begin scraping. HTML elements such as <img>
, <video>
, and <audio>
tags are common targets for media extraction.
Advanced Techniques for Extracting Embedded Media
Using BeautifulSoup
BeautifulSoup is a popular Python library used for web scraping due to its simplicity and readability. It allows you to parse HTML and XML documents with ease. Here’s how you can use BeautifulSoup to extract embedded images:
import requests
from bs4 import BeautifulSoup
url = 'https://example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
images = soup.find_all('img')
for img in images:
print(img['src'])
Employing lxml for Media Extraction
lxml is another powerful library that offers faster parsing speeds compared to BeautifulSoup. It’s particularly useful for handling large datasets and complex XML structures.
from lxml import html
import requests
url = 'https://example.com'
response = requests.get(url)
tree = html.fromstring(response.text)
images = tree.xpath('//img/@src')
for img in images:
print(img)
Advanced Image Extraction with Selenium
Selenium is a tool used for automating web browsers, making it ideal for handling dynamic content that requires JavaScript execution. Here’s how you can use Selenium to extract embedded media:
from selenium import webdriver
from selenium.webdriver.common.by import By
driver = webdriver.Chrome()
driver.get('https://example.com')
images = driver.find_elements(By.TAG_NAME, 'img')
for img in images:
print(img.get_attribute('src'))
driver.quit()
Handling Dynamic Websites and JavaScript Content
Many modern websites use JavaScript to load content dynamically, making it challenging for traditional scraping methods. Tools like Selenium and Puppeteer can handle these scenarios by rendering the JavaScript before extracting data.
Puppeteer, in particular, is a headless browser that allows you to control a Chrome or Chromium browser instance programmatically. Here’s an example:
import asyncio
from pyppeteer import launch
async def main():
browser = await launch()
page = await browser.new_page()
await page.goto('https://example.com')
images = await page.evaluate('document.querySelectorAll("img")')
for img in images:
print(await (await img.get_attribute('src')))
await browser.close()
asyncio.run(main())
Dealing with Authentication and CAPTCHAs
Some websites require authentication to access their content. Libraries like requests-oauthlib
can help manage OAuth tokens for authenticated requests. Additionally, services like 2Captcha or Anti-Captcha can assist in solving CAPTCHAs programmatically.
Optimizing Proxy Management for Large-Scale Scraping
When performing large-scale scraping, using proxies is essential to avoid IP bans and rate limiting. Tools like Scrapy
or proxy management services can help rotate proxies effectively.
import requests
from scrapy import settings
proxies = [
{"http": "http://10.10.1.10:3128", "https": "https://10.10.1.10:1080"},
{"http": "http://10.10.1.11:3128", "https": "https://10.10.1.11:1080"},
]
url = 'https://example.com'
response = requests.get(url, proxies=proxies[0])
Real-Time Data Extraction Techniques
For real-time data extraction, consider using WebSocket connections to receive live updates from the server. Libraries like websockets
can help establish these connections.
import asyncio
import websockets
async def receive_data(uri):
async with websockets.connect(uri) as websocket:
while True:
data = await websocket.recv()
print(data)
asyncio.run(receive_data('ws://example.com/socket'))
Best Practices and Ethical Considerations
- Respect Robots.txt: Always check the
robots.txt
file of a website to understand their scraping policies. - Ethical Scraping: Ensure that your scraping activities comply with legal and ethical standards.
- Rate Limiting: Implement rate limiting to avoid overloading the server.
- Data Storage: Efficiently store extracted data using databases like MongoDB or SQLite.
- Error Handling: Incorporate robust error handling to manage network issues and unexpected content changes.
For more advanced techniques related to social media web scraping, refer to our guide on Advanced Techniques for Social Media Web Scraping. Additionally, understanding how to extract embedded metadata can be crucial; read more about it in our article on Extracting Embedded Metadata from Websites Using Web Scraping.
Conclusion
Extracting embedded media from websites can be both challenging and rewarding. By leveraging advanced techniques like BeautifulSoup, lxml, Selenium, and Puppeteer, you can efficiently gather valuable multimedia content. Always remember to adhere to best practices and ethical considerations to ensure your scraping activities are responsible and effective.
FAQ Section
Q: Can I use web scraping for commercial purposes? A: Yes, web scraping can be used for commercial purposes, but it’s essential to comply with the target website’s terms of service and legal regulations.
Q: How do I handle CAPTCHAs during web scraping? A: Services like 2Captcha or Anti-Captcha can help solve CAPTCHAs programmatically, allowing you to continue scraping even when faced with these challenges.
Q: What is the best way to handle dynamic content loaded via JavaScript? A: Tools like Selenium and Puppeteer are ideal for handling dynamic content that requires JavaScript execution. They render the JavaScript before extracting data, ensuring you capture all necessary information.
Q: How can I avoid getting my IP banned during large-scale scraping? A: Using proxies and rotating them effectively can help avoid IP bans. Tools like Scrapy or proxy management services can assist in this process.
Q: What is the most efficient way to store extracted data? A: Efficient data storage depends on your specific needs, but databases like MongoDB (for unstructured data) and SQLite (for structured data) are popular choices for storing web scraping results.