· Charlotte Will · webscraping · 4 min read
Extracting Embedded Metadata from Websites Using Web Scraping
Learn practical techniques to extract embedded metadata from websites using web scraping. Optimize SEO, analyze content, and gain competitive insights with tools like BeautifulSoup and Scrapy. Master best practices for efficient data extraction and avoid common pitfalls.
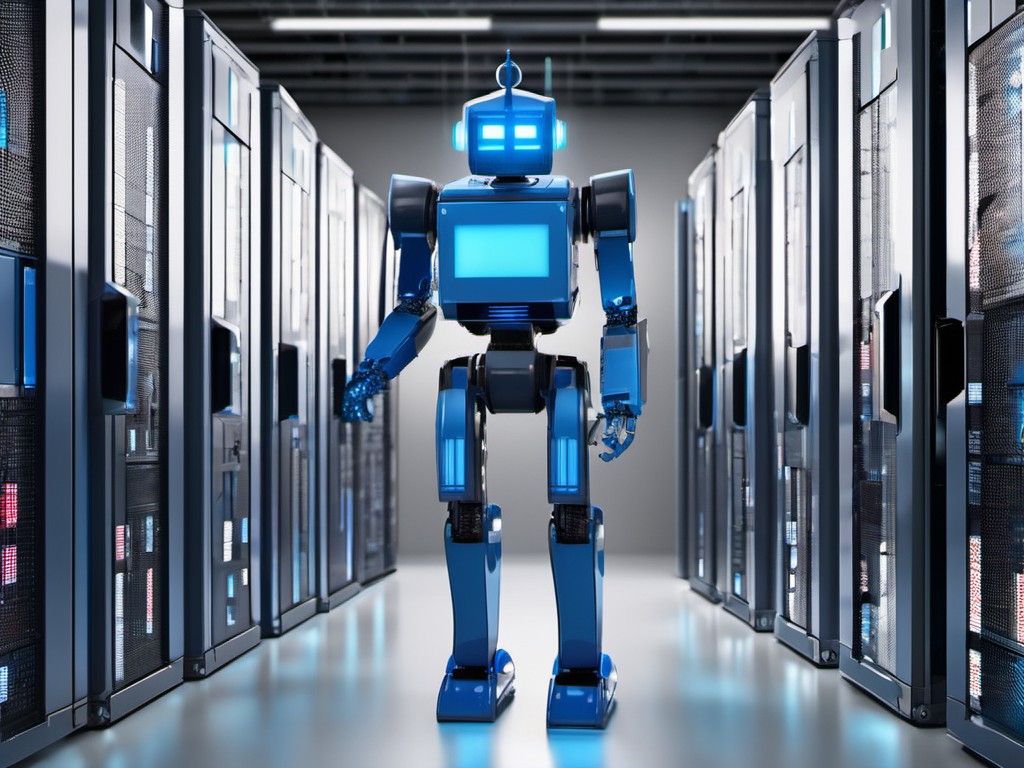
Web scraping is a powerful technique for extracting valuable data from websites. One of the most useful applications of web scraping is extracting embedded metadata. Metadata, often overlooked, provides crucial information about the content, such as its creation date, author, and keywords. In this article, we will delve into how to extract embedded metadata from websites using web scraping techniques.
Understanding Web Scraping
Before diving into the specifics of metadata extraction, let’s quickly review what web scraping is all about. Web scraping involves using automated scripts or software to systematically extract data from websites. This technique can be used to gather information that is not readily available in structured formats like APIs or databases.
What is Embedded Metadata?
Embedded metadata refers to information embedded within the content of a webpage, such as HTML tags. Examples include meta descriptions, title tags, and Open Graph data. This metadata is essential for search engines and social media platforms to understand and index your content effectively.
Why Extract Embedded Metadata?
Extracting embedded metadata offers several benefits:
- SEO Optimization: Understand how your competitors are using metadata to optimize their search engine rankings.
- Content Analysis: Gain insights into the types of keywords and topics that a website focuses on.
- Competitive Intelligence: Stay informed about changes in your competitor’s marketing strategies by monitoring their metadata.
Techniques for Extracting Embedded Metadata
Here are some practical techniques for extracting embedded metadata from websites using web scraping:
1. HTML Parsing with BeautifulSoup
BeautifulSoup is a popular Python library for parsing HTML and XML documents. It allows you to navigate the parse tree and extract data easily.
from bs4 import BeautifulSoup
import requests
url = 'https://example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
title_tag = soup.title.string
meta_description = soup.find('meta', attrs={'name': 'description'})['content']
print(f"Title: {title_tag}\nDescription: {meta_description}")
2. Using Scrapy Framework
Scrapy is an open-source web crawling framework that makes it easy to extract data from websites and process it concurrently.
import scrapy
class MetadataSpider(scrapy.Spider):
name = "metadata"
start_urls = ['https://example.com']
def parse(self, response):
title = response.css('title::text').get()
description = response.css('meta[name=description]::attr(content)').get()
yield {
'url': response.url,
'title': title,
'description': description
}
3. Handling Dynamic Websites with Selenium
For websites that rely heavily on JavaScript to load content, tools like Selenium can be used in conjunction with web scraping techniques. Read more about handling dynamic websites with Selenium here.
4. Leveraging APIs for Metadata Extraction
Some services provide APIs to extract metadata more efficiently. Tools like SerpApi or Google Custom Search API can be very useful.
Best Practices for Metadata Extraction Using Web Scraping
- Respect Robots.txt: Always check a website’s
robots.txt
file to ensure you are not scraping disallowed sections. - Use Proxies and Rotating IPs: To avoid getting blocked, use proxies or rotating IP addresses.
- Implement Rate Limiting: Avoid overwhelming the server by introducing delays between requests.
- Clean and Normalize Data: Ensure extracted metadata is clean and normalized for further analysis.
Tools for Extracting Website Metadata via Web Scraping
Several tools can help simplify the process of extracting embedded metadata:
- Octoparse: A user-friendly web scraping tool with a point-and-click interface.
- ParseHub: Another powerful tool that supports JavaScript rendering.
- Import.io: Known for its robust data extraction capabilities and ease of use.
How to Handle Dynamic Websites with Selenium for Web Scraping
Handling dynamic websites is a common challenge in web scraping. Tools like Selenium can help by simulating browser interactions to load JavaScript-driven content. Learn more about advanced techniques here.
FAQs
What is the difference between web scraping and API usage? Web scraping involves extracting data directly from a website’s HTML, whereas APIs provide structured data in formats like JSON or XML.
Can I get into legal trouble for web scraping? It depends on the terms of service of the website you are scraping and local laws. Always check the
robots.txt
file and terms of service.How do I handle websites with CAPTCHA? Handling CAPTCHAs can be complex, often requiring human intervention or advanced techniques like CAPTCHA solving services.
What is the best language for web scraping? Python is widely regarded as the best language due to its simplicity and powerful libraries such as BeautifulSoup and Scrapy.
How do I ensure my web scraper doesn’t get blocked? Use techniques like rate limiting, rotating proxies, and user-agent rotation to mimic human behavior and avoid detection.
Conclusion
Extracting embedded metadata from websites using web scraping can be a game-changer for SEO optimization, content analysis, and competitive intelligence. By following the best practices and leveraging the right tools, you can efficiently gather valuable data to make informed decisions. Whether you prefer Python libraries like BeautifulSoup or frameworks like Scrapy, the key is to start small and iterate based on your specific needs. Happy scraping!