· Charlotte Will · webscraping · 5 min read
Advanced Data Extraction Techniques Using BeautifulSoup and lxml
Discover advanced data extraction techniques using BeautifulSoup and lxml for efficient web scraping. Learn best practices, real-world examples, and how to combine these powerful Python libraries to handle complex HTML structures and large datasets. Enhance your data extraction capabilities today!
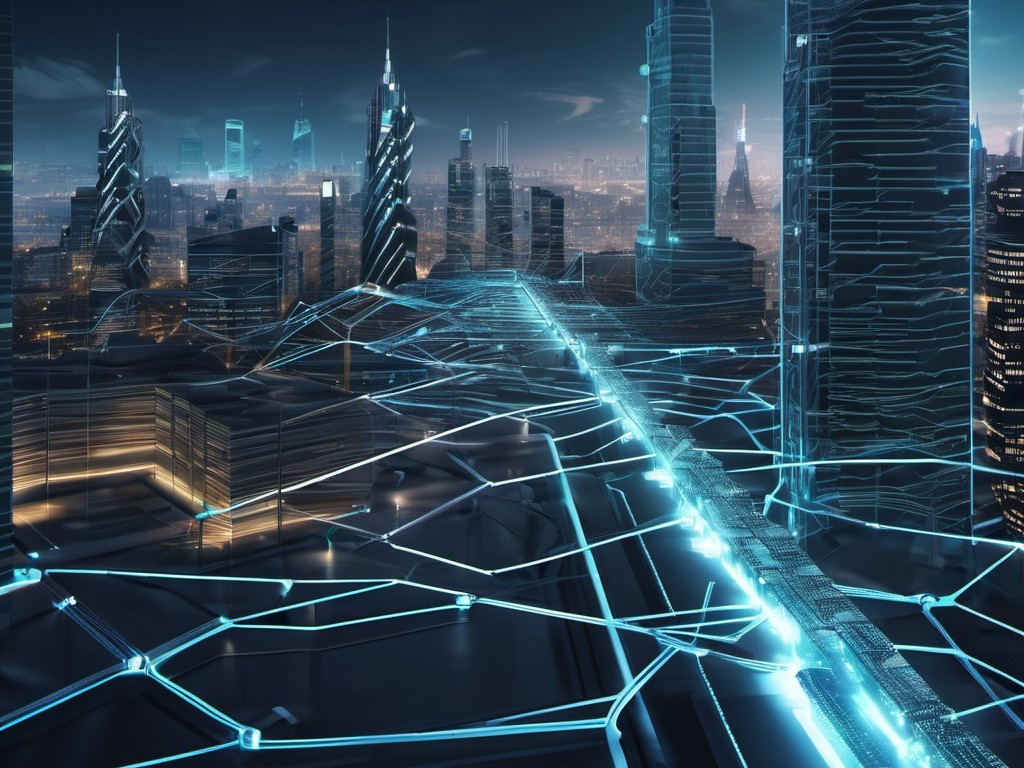
Advanced Data Extraction Techniques Using BeautifulSoup and lxml
In today’s data-driven world, the ability to extract valuable information from web pages has become increasingly essential. Web scraping allows us to gather data from HTML documents efficiently, but it can be challenging due to complex HTML structures. Two of the most powerful Python libraries for this task are BeautifulSoup and lxml. This comprehensive guide will explore advanced data extraction techniques using these tools, providing practical examples and best practices.
Introduction to Advanced Data Extraction Techniques
Data extraction involves parsing HTML documents to retrieve specific information. While basic extraction can be accomplished with simple libraries like requests
or urllib
, more complex tasks require robust tools. BeautifulSoup is renowned for its ease of use and readability, while lxml offers high performance and flexibility. Combining these libraries can unlock even greater capabilities.
Why Use BeautifulSoup for Web Scraping?
BeautifulSoup stands out due to its user-friendly API and strong support community. It excels at navigating and searching through HTML documents with ease, making it an excellent choice for developers who prioritize simplicity and readability. The library’s ability to handle malformed HTML gracefully is another significant advantage.
Key Features of BeautifulSoup:
- Intuitive syntax for parsing HTML.
- Robust error handling for malformed HTML.
- Supports multiple parsers, including the built-in Python HTML parser and external libraries like lxml.
Why Use lxml for Web Scraping?
lxml is known for its high performance and compliance with XML and HTML standards. It provides a fast and efficient way to parse documents and supports XPath and XSLT, making it ideal for complex parsing tasks. Additionally, lxml’s support for namespaces and custom DTDs makes it suitable for scraping modern web pages that use advanced HTML features.
Key Features of lxml:
- High performance with C-based parsing.
- Support for XPath and XSLT.
- Comprehensive error handling and validation.
Combining BeautifulSoup and lxml for Optimal Results
By combining BeautifulSoup and lxml, you can leverage the strengths of both libraries. Use lxml’s fast parsing capabilities to handle complex HTML structures quickly and then switch to BeautifulSoup for more straightforward navigation and manipulation.
Steps to Combine BeautifulSoup and lxml:
- Parse the HTML document with lxml.
- Convert the parsed tree to a BeautifulSoup object.
- Use BeautifulSoup’s intuitive API for data extraction.
Here’s an example of how to combine these libraries:
from lxml import html
from bs4 import BeautifulSoup
import requests
# Fetch the HTML content
response = requests.get('http://example.com')
html_content = response.text
# Parse with lxml and convert to BeautifulSoup
lxml_tree = html.fromstring(html_content)
soup = BeautifulSoup(lxml_tree, 'html.parser')
# Extract data using BeautifulSoup
data = soup.find_all('div', class_='example-class')
for item in data:
print(item.text)
Practical Examples of Using BeautifulSoup and lxml Together
Handling Complex HTML Structures
Some websites use complex nested structures that can be difficult to parse with a single library. By using lxml for the initial parsing and then switching to BeautifulSoup, you can handle these tasks more efficiently.
from lxml import html
from bs4 import BeautifulSoup
import requests
# Fetch the HTML content
response = requests.get('http://example.com/complex')
html_content = response.text
# Parse with lxml and convert to BeautifulSoup
lxml_tree = html.fromstring(html_content)
soup = BeautifulSoup(lxml_tree, 'html.parser')
# Extract data from complex structures
complex_data = soup.find('div', {'id': 'complex-id'})
for nested in complex_data.find_all('div', class_='nested'):
print(nested.text)
Efficient Data Extraction Methods
When dealing with large datasets, performance becomes crucial. Using lxml for initial parsing and then leveraging BeautifulSoup for more straightforward tasks can significantly improve efficiency.
from lxml import html
from bs4 import BeautifulSoup
import requests
# Fetch the HTML content
response = requests.get('http://example.com/large-dataset')
html_content = response.text
# Parse with lxml and convert to BeautifulSoup
lxml_tree = html.fromstring(html_content)
soup = BeautifulSoup(lxml_tree, 'html.parser')
# Efficiently extract large datasets
large_dataset = soup.find_all('tr', class_='data-row')
for row in large_dataset:
data = row.find_all('td')
print([item.text for item in data])
Best Practices for Efficient Data Extraction
- Use Headers and Cookies: Respect the website’s robots.txt file and include appropriate headers to avoid getting blocked.
- Rate Limiting: Implement rate limiting to prevent overwhelming the server with too many requests in a short period.
- Error Handling: Always include robust error handling to manage network issues, parse errors, and changes in HTML structure.
- Data Storage: Efficiently store extracted data using databases like SQLite or MongoDB for easy retrieval and analysis.
- Regular Updates: Periodically update your scraping scripts to adapt to changes in website layouts and structures.
Linking to Relevant Articles: For more insights into Python webscraping techniques, check out our article on “Understanding Python Webscraping Techniques for Data Extraction.” If you’re interested in image extraction, read our guide on “Advanced Image Extraction Techniques Using Web Scraping.”
Conclusion: Enhancing Your Web Scraping Capabilities
By mastering the advanced data extraction techniques using BeautifulSoup and lxml, you can significantly enhance your web scraping capabilities. Combining these powerful libraries allows for efficient handling of complex HTML structures and large datasets. Whether you’re a beginner or an experienced developer, understanding how to use BeautifulSoup and lxml together will open up new opportunities for data extraction and analysis.
FAQ Section
1. Which library is better for parsing complex HTML structures?
- For complex structures, using lxml for initial parsing and then switching to BeautifulSoup can provide the best of both worlds in terms of performance and ease of use.
2. How can I handle malformed HTML gracefully?
- BeautifulSoup excels at handling malformed HTML due to its robust error handling capabilities. It allows you to parse and extract data even from poorly structured documents.
3. Can I use XPath with BeautifulSoup?
- BeautifulSoup does not natively support XPath. However, you can combine it with lxml, which does support XPath, to leverage the strengths of both libraries.
4. What is the best way to store extracted data?
- Efficient data storage depends on your use case. For small datasets, CSV files might suffice. For larger and more complex data, databases like SQLite or MongoDB are recommended.
5. How can I ensure my web scraping is compliant with legal requirements?
- Always check the website’s
robots.txt
file to understand its scraping policies. Respect rate limits and include proper headers and cookies in your requests. Additionally, review any terms of service to ensure compliance with legal requirements.