· Charlotte Will · 5 min read
Understanding Python Webscraping Techniques for Data Extraction
Discover essential Python web scraping techniques to extract valuable data from websites efficiently. Learn about popular libraries like BeautifulSoup and Scrapy, best practices, real-world applications, and troubleshooting common issues in this comprehensive guide.
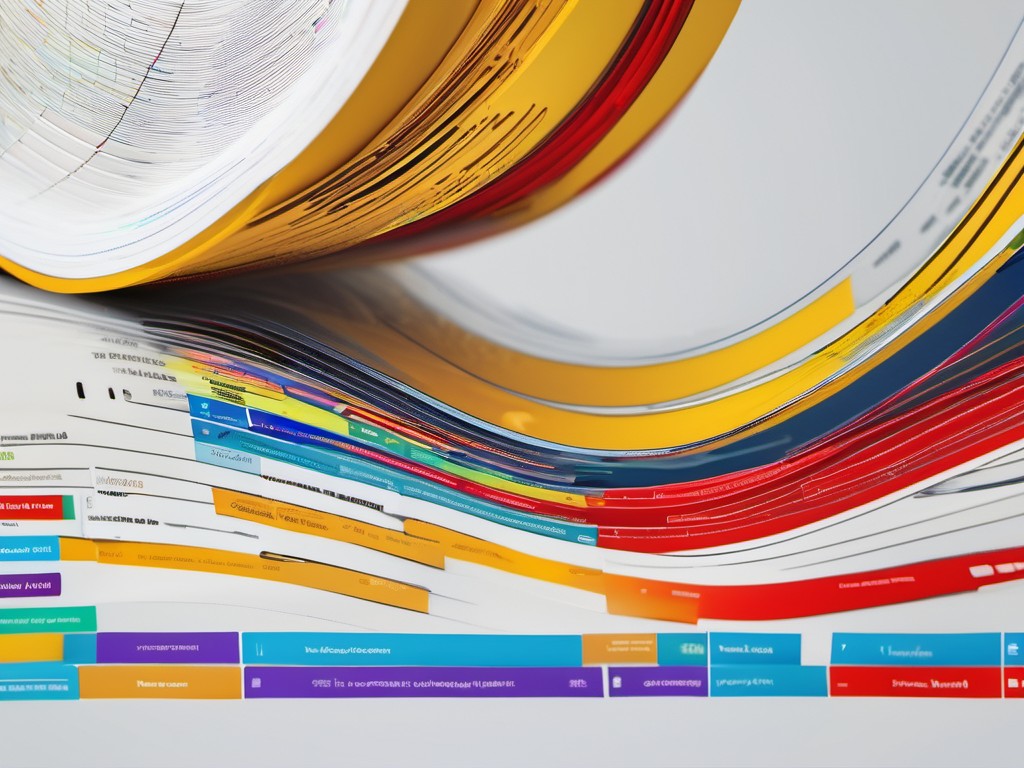
Web scraping has become an essential skill in today’s data-driven world. It enables developers and analysts to extract valuable data from websites, which can be used for various purposes such as market research, price comparison, and even machine learning projects. Python is one of the most popular languages for web scraping due to its simplicity and powerful libraries. In this article, we’ll explore the basics of web scraping in Python, delve into advanced techniques, and discuss best practices for effective data extraction.
Getting Started with Web Scraping in Python
Before diving into the technical aspects, let’s set up your environment. You will need Python installed on your machine. It’s also a good idea to use a virtual environment to manage dependencies. You can create one using venv
:
python -m venv env
source env/bin/activate # On Windows, use `env\Scripts\activate`
Understanding Basic Libraries for Web Scraping
BeautifulSoup
BeautifulSoup is a popular library used for parsing HTML and XML documents. It creates parse trees from page source code that can be used to extract data in a hierarchical, readable manner. Here’s how you can use it:
import requests
from bs4 import BeautifulSoup
url = 'http://example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
# Example of extracting a title tag
title = soup.find('title').text
print(title)
Requests
The requests
library is used for sending HTTP requests. It’s simple and powerful, making it an excellent choice for web scraping. Here’s how you can use it to fetch the content of a webpage:
import requests
url = 'http://example.com'
response = requests.get(url)
print(response.text)
Advanced Techniques Using Scrapy
Scrapy is a powerful, open-source web crawling framework for Python that can be used to build web spiders. It provides more advanced features compared to requests
and BeautifulSoup
.
Installation and Setup
First, install Scrapy using pip:
pip install scrapy
Create a new Scrapy project:
scrapy startproject my_scraper
cd my_scraper
Writing a Basic Scraper
Let’s create a simple spider to scrape data from a website. Create a new file example_spider.py
:
import scrapy
class ExampleSpider(scrapy.Spider):
name = 'example'
start_urls = ['http://example.com']
def parse(self, response):
title = response.xpath('//title/text()').get()
yield {'title': title}
Run the spider using:
scrapy crawl example -o output.json
This will save the scraped data into output.json
.
Best Practices for Effective Web Scraping
Respecting robots.txt
Before scraping any website, check its robots.txt
file to understand which parts of the site are off-limits. This is a simple text file located at http://www.example.com/robots.txt
.
User-agent: *
Disallow: /private/
Handling Dynamic Content with Selenium
Some websites use JavaScript to load content dynamically, which can be challenging for traditional scrapers. Selenium is a tool that automates web browsers and can handle such cases. Here’s an example:
from selenium import webdriver
from bs4 import BeautifulSoup
driver = webdriver.Chrome() # Make sure chromedriver is in your PATH
driver.get('http://example.com')
# Wait for JavaScript to load content
import time
time.sleep(5)
page_source = driver.page_source
soup = BeautifulSoup(page_source, 'html.parser')
title = soup.find('title').text
print(title)
driver.quit()
Case Studies: Real-world Applications of Python Web Scraping
Price Monitoring
Many businesses use web scraping to monitor competitors’ prices and adjust their own pricing strategies accordingly. For example, a retailer might scrape prices from Amazon and eBay daily to ensure they remain competitive.
Market Research
Web scraping can be used to gather data for market research. For instance, a company looking to enter a new market could scrape product reviews and customer feedback from relevant websites to understand the needs and preferences of potential customers.
Troubleshooting Common Issues in Web Scraping
Blocked by the Website
If you get blocked while scraping, it might be due to excessive requests in a short period or ignoring robots.txt
. Use proxies and respect rate limits to avoid this issue.
Malformed HTML
Sometimes websites have malformed HTML, which can make parsing difficult. Libraries like BeautifulSoup handle most cases well, but you might need to preprocess the HTML in some scenarios.
Conclusion
Python web scraping is a powerful tool for extracting data from websites. With libraries like BeautifulSoup and Scrapy, you can build robust scrapers that meet various needs. Always remember to respect the website’s terms of service and use ethical practices while scraping data.
FAQ Section
Q1: What is web scraping?
A1: Web scraping is a technique used to extract data from websites. It involves sending HTTP requests to a server, parsing the response HTML, and then extracting the desired information.
Q2: Is web scraping legal?
A2: The legality of web scraping depends on how it’s done and what data is being extracted. Always respect the website’s robots.txt
file and terms of service. It’s also a good idea to contact the website owner if you plan to scrape large amounts of data.
Q3: What are some common use cases for web scraping?
A3: Web scraping is used in various industries for tasks such as price monitoring, market research, lead generation, and even academic research. It can help businesses stay competitive by providing valuable insights into their markets and customers.
Q4: How can I handle dynamic content with JavaScript?
A4: For handling dynamic content that requires JavaScript execution, tools like Selenium or Playwright are used. These tools automate web browsers and allow you to interact with pages as a human would.
Q5: What are some best practices for web scraping?
A5: Some best practices include respecting robots.txt
, implementing rate limiting, using proxies to avoid IP blocking, handling errors gracefully, and ensuring your code is maintainable and well-documented.