· Charlotte Will · webscraping · 5 min read
Advanced Image Extraction Techniques Using Web Scraping
Discover advanced image extraction techniques using web scraping, including handling dynamic content and large datasets. Learn practical tips and actionable advice suitable for both beginners and experienced users in Python and Scrapy.
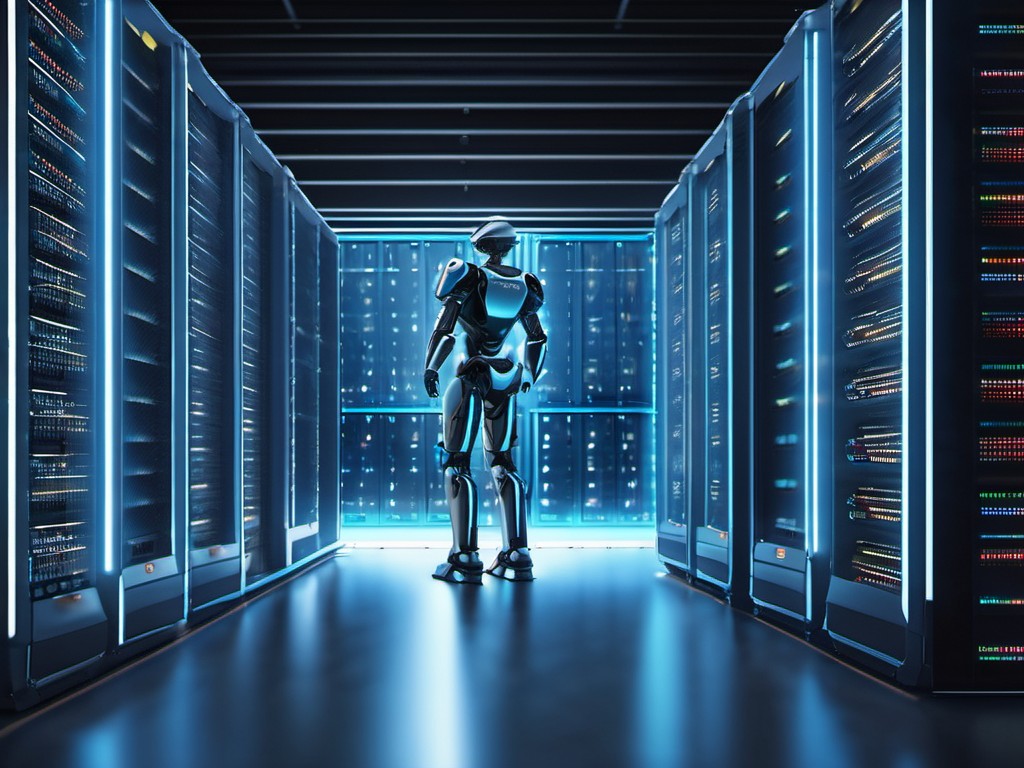
Web scraping has evolved from being a niche technique to an essential tool for data extraction, especially when it comes to images. With the rise of visual content on websites, extracting images programmatically has become increasingly important. This article delves into advanced image extraction techniques using web scraping, providing practical tips and actionable advice suitable for both beginners and experienced users.
Understanding Web Scraping for Images
Web scraping involves automating the process of data extraction from websites. When it comes to images, this involves not just downloading them but also organizing and processing them in a structured manner. Advanced techniques allow for more sophisticated handling, including dealing with dynamic content and large datasets.
Basic Techniques: Getting Started
Before diving into advanced methods, it’s essential to understand the basics of web scraping for images. Python is one of the most popular languages for this purpose due to its powerful libraries like BeautifulSoup and Selenium.
Extracting Images Using BeautifulSoup
BeautifulSoup is a widely-used library for parsing HTML and XML documents. Here’s a basic example of how you can extract images using BeautifulSoup:
import requests
from bs4 import BeautifulSoup
url = 'https://example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
images = soup.find_all('img')
for img in images:
print(img['src'])
This code fetches the HTML content of a webpage and extracts all image source URLs.
Advanced Python Web Scraping for Images
Handling Dynamic Content with Selenium
Sites that load content dynamically (e.g., through JavaScript) require more advanced techniques. Selenium, combined with ChromeDriver, can handle such cases by rendering the page as a user would in a browser.
from selenium import webdriver
driver = webdriver.Chrome(executable_path='/path/to/chromedriver')
driver.get('https://example.com')
images = driver.find_elements_by_tag_name('img')
for img in images:
print(img.get_attribute('src'))
driver.quit()
Extracting Metadata
Extracting image metadata (e.g., alt text, dimensions) can be useful for organizing and processing images. You can use BeautifulSoup to extract this information alongside the image URLs.
for img in images:
src = img['src']
alt = img.get('alt', '')
print(f"Image Source: {src}, Alt Text: {alt}")
Advanced Techniques for Large Datasets
Asynchronous Scraping with Scrapy and Image Pipelines
Scrapy is a powerful framework designed specifically for web scraping. It supports asynchronous requests, making it ideal for handling large datasets efficiently.
First, install Scrapy:
pip install scrapy
Create a new Scrapy project and spider:
scrapy startproject image_scraper
cd image_scraper
scrapy genspider example example.com
Modify the spider to extract images and use an Image Pipeline to download them:
import scrapy
from scrapy.pipelines.images import ImagesPipeline
class ExampleSpider(scrapy.Spider):
name = 'example'
start_urls = ['https://example.com']
def parse(self, response):
for img in response.css('img'):
yield {
'image_url': img.css('::attr(src)').get(),
'image_name': img.css('::attr(alt)').get() or 'no_name',
}
Configure the Image Pipeline in settings.py
:
ITEM_PIPELINES = {
'scrapy.pipelines.images.ImagesPipeline': 1,
}
IMAGES_STORE = '/path/to/save/images'
Handling Rate Limits and Proxies
To avoid getting blocked by websites, it’s crucial to respect rate limits and use proxies. Scrapy has built-in support for both:
# settings.py
DOWNLOADER_MIDDLEWARES = {
'scrapy.downloadermiddlewares.retry.RetryMiddleware': 90,
}
RETRY_TIMES = 5
RETRY_HTTP_CODES = [429, 500, 502, 503, 504]
PROXY_POOL_ENABLED = True
Practical Applications of Image Extraction
Competitive Intelligence and Market Analysis
Extracting images from competitor websites can provide insights into their product offerings and marketing strategies. This data can be used to inform your own strategies and identify gaps in the market.
Building Datasets for Machine Learning
Large datasets of images are essential for training machine learning models, particularly in computer vision tasks such as object recognition or image classification. Automated web scraping can help gather these datasets efficiently.
Best Practices and Ethical Considerations
Respect Robots.txt and Terms of Service
Always check a website’s robots.txt
file and terms of service before scraping to ensure you comply with their policies. Unauthorized scraping can lead to legal issues and damage your reputation.
Optimize and Maintain Your Code
Regularly update your scraping scripts to handle changes in the website’s structure. Use version control systems like Git to manage your codebase effectively.
Internal Linking for Further Learning
For more advanced techniques, consider exploring ‘Extracting Data from Web Forms Using Advanced Web Scraping Techniques’ which delves into handling complex web forms. Additionally, ‘Advanced Techniques for Competitive Intelligence Web Scraping’ offers insights into leveraging web scraping to gain a competitive edge in your industry.
Frequently Asked Questions (FAQs)
1. How can I handle JavaScript-rendered images?
To extract images rendered by JavaScript, use Selenium or Scrapy with Splash, which allows you to render the page before scraping it.
2. What are some common issues faced while scraping images?
Common issues include rate limiting, blocking by websites, and changes in website structure. Implementing retries, using proxies, and monitoring for structural changes can help mitigate these problems.
3. How do I handle large volumes of images?
For large datasets, consider asynchronous scraping with Scrapy and use an Image Pipeline to download and store the images efficiently.
4. What legal considerations should I be aware of when scraping images?
Always check the website’s terms of service and robots.txt
file. Be mindful of copyright laws, and ensure that you are not infringing upon any intellectual property rights.
5. How can I process extracted images for further use?
Extracted images can be processed using image processing libraries like Pillow in Python. This includes resizing, converting formats, and adding metadata to the images.