· Charlotte Will · 4 min read
What is Python Webscraping: A Beginner's Guide
Learn how to scrape web data using Python with this beginner-friendly guide. Discover essential libraries like BeautifulSoup and Scrapy, step-by-step tutorials, best practices, and common mistakes in web scraping. Start your data extraction journey today!
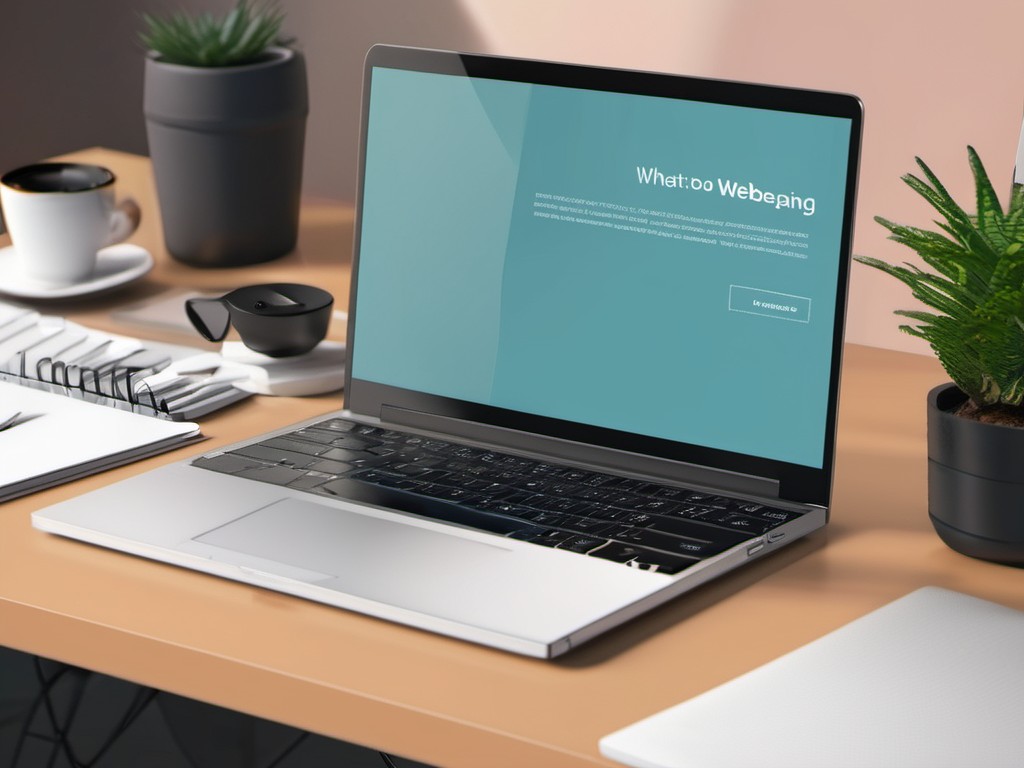
Welcome to our comprehensive guide on Python web scraping! If you’re new to the world of data extraction and eager to learn how to pull valuable information from websites, you’ve come to the right place. In this article, we will explore the basics of web scraping, essential Python libraries, and step-by-step instructions for creating your first web scraper. Let’s dive in!
Understanding the Basics of Web Scraping
Web scraping is the process of extracting data from websites programmatically. This technique is incredibly useful for gathering information that isn’t readily available through APIs or other means. Whether you’re looking to compile a list of products, monitor news articles, or gather data for analysis, web scraping can be a game-changer.
Why Use Python for Web Scraping?
Python is a popular choice for web scraping due to its simplicity and the wide range of libraries that support this task. Here are a few reasons why Python stands out:
- Easy to Learn: Python’s syntax is straightforward, making it an excellent choice for beginners.
- Powerful Libraries: Libraries like BeautifulSoup and Scrapy make web scraping a breeze.
- Flexible and Extensible: Python can handle various types of data extraction tasks with ease.
Essential Python Libraries for Web Scraping
BeautifulSoup Overview
BeautifulSoup is one of the most popular libraries for web scraping in Python. It allows you to pull data out of HTML and XML files by providing simple methods to navigate, search, and modify the parse tree.
- Installation: You can install BeautifulSoup using pip:
pip install beautifulsoup4
Using Scrapy for Advanced Scraping
Scrapy is a powerful and open-source web crawling framework. It’s particularly useful for larger-scale scraping projects and handling complex scraping tasks.
- Installation: Install Scrapy via pip:
pip install scrapy
Step-by-Step Guide to Your First Python Web Scraper
Setting Up the Environment
Before you start coding, ensure that you have the necessary libraries installed. You’ll need requests
for making HTTP requests and beautifulsoup4
for parsing HTML.
pip install requests beautifulsoup4
Writing Your First Script
Let’s create a simple web scraper to extract the titles of articles from a news website.
Import Libraries: Start by importing the required libraries.
import requests from bs4 import BeautifulSoup
Make an HTTP Request: Use
requests
to fetch the webpage content.url = 'https://example-news-site.com' response = requests.get(url)
Parse HTML Content: Use BeautifulSoup to parse the HTML.
soup = BeautifulSoup(response.content, 'html.parser')
Extract Data: Locate and extract the desired data (e.g., article titles).
for title in soup.find_all('h2', class_='title'): print(title.get_text())
Complete Script Example
Here is a complete script that combines all the steps:
import requests
from bs4 import BeautifulSoup
# Fetch the webpage content
url = 'https://example-news-site.com'
response = requests.get(url)
# Parse the HTML content with BeautifulSoup
soup = BeautifulSoup(response.content, 'html.parser')
# Extract and print article titles
for title in soup.find_all('h2', class_='title'):
print(title.get_text())
Best Practices and Common Mistakes
Respect Website Policies
Always check the website’s robots.txt
file to understand its scraping policies. Respect these rules to avoid legal issues.
Use Headers
Include appropriate headers in your requests to mimic a real browser and reduce the chance of being blocked.
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36'
}
response = requests.get(url, headers=headers)
Handle Pagination
Many websites use pagination to split content across multiple pages. Implement logic to handle this and scrape all required data.
Avoid Overloading the Server
Add delays between requests to avoid overwhelming the server. This is especially important when scraping large sites.
import time
time.sleep(2) # Pause for 2 seconds before the next request
Conclusion
Congratulations! You now have a solid foundation in Python web scraping. Web scraping is a powerful skill that can be used in a variety of applications, from data analysis to competitive intelligence. As you progress, explore more advanced techniques and libraries like Scrapy to handle larger and more complex projects. Happy scraping!
FAQs
What are the legal considerations of web scraping?
Web scraping can raise legal concerns if not done properly. Always check the website’s terms of service and robots.txt
file to ensure compliance. Some websites explicitly prohibit scraping, while others may allow it under specific conditions.
How do I handle dynamic content in web scraping?
Dynamic content (loaded via JavaScript) can be challenging to scrape because it’s not present in the initial HTML response. Tools like Selenium or using a headless browser with Scrapy can help render and extract this data.
What are some advanced techniques for web scraping?
Advanced techniques include handling CAPTCHAs, rotating proxies to avoid IP bans, using machine learning to extract data from complex layouts, and employing parallel processing for faster scraping.
Can I use Python for real-time web scraping?
Yes, Python can be used for real-time web scraping by implementing scheduled tasks or continuous monitoring. Tools like Scrapy with a built-in scheduler (Scrapyd) or using cron jobs can help achieve this.
How do I store and analyze the data extracted from web scraping?
Stored data can be analyzed using various tools such as Pandas, NumPy, or even databases like SQLite or PostgreSQL. Visualization libraries like Matplotlib or Seaborn can help you create insights from your data.