· Charlotte Will · webscraping · 6 min read
What is Scrapy Web Scraping Tutorial?
Learn how to master web scraping with Scrapy! This comprehensive tutorial covers everything from installation to advanced techniques like handling JavaScript rendering and rotating proxies. Ideal for both beginners and intermediate users looking to extract data efficiently using Python.
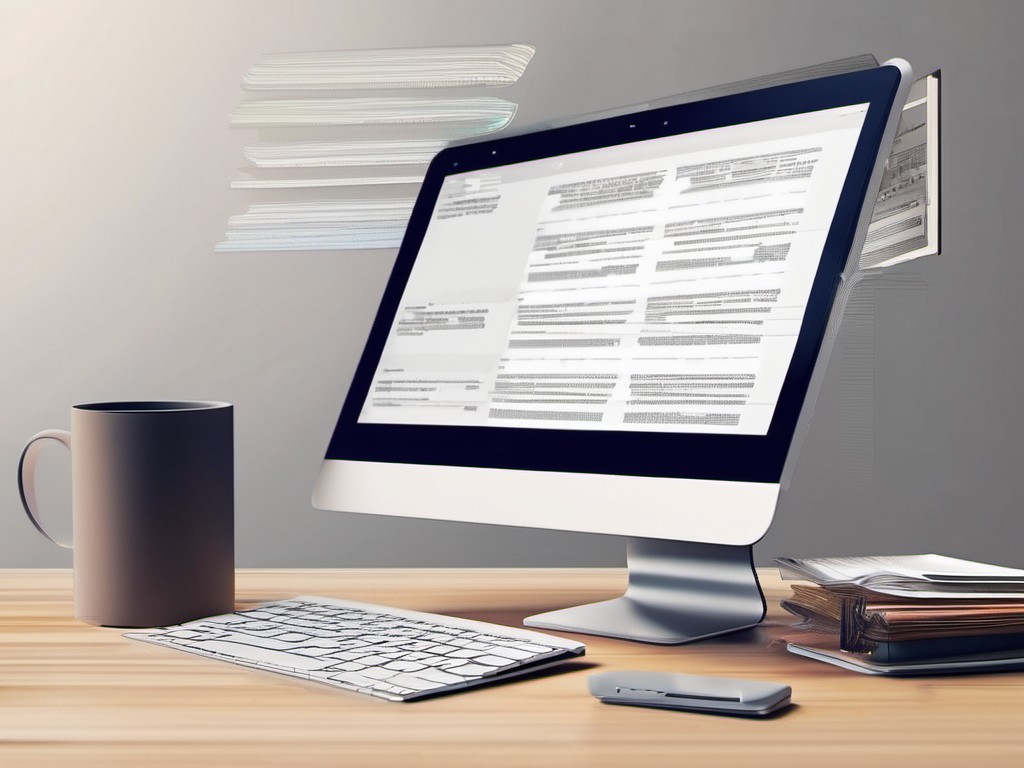
Are you looking to extract data from websites efficiently using Python? Look no further than Scrapy, an open-source web scraping framework designed for this very purpose. This comprehensive tutorial will guide you through everything you need to know about web scraping with Scrapy. Whether you’re a beginner or an intermediate user, we’ll cover the basics and advanced topics to help you master data extraction with Scrapy.
Introduction to Scrapy
Scrapy is not just any web scraping tool; it’s a powerful framework that allows you to write Python web scraping scripts effortlessly. With its flexibility and robustness, Scrapy can handle large-scale data extraction tasks efficiently. Let’s dive into why Scrapy stands out in the world of web scraping.
Why Choose Scrapy?
- Efficiency: Scrapy is built for speed and efficiency, capable of handling millions of requests per hour.
- Scalability: It can scale from small to large projects seamlessly.
- Extensibility: With a vast ecosystem of plugins and extensions, you can customize Scrapy to fit your needs.
- Community Support: A large community ensures continuous updates and support.
Installation and Setup of Scrapy
Before we dive into the practical aspects of web scraping with Scrapy, let’s set up our environment.
Prerequisites
To get started, you need to have Python installed on your system. Scrapy supports Python 3.5 and above. Ensure you also have pip
, the Python package installer.
Installing Scrapy
Open your command line interface (CLI) and run:
pip install scrapy
This will download and install Scrapy along with its dependencies. To verify the installation, you can type:
scrapy version
If everything is set up correctly, it should display the installed version of Scrapy.
Basic Concepts of Scrapy
Understanding some basic concepts will help you get a grasp of how Scrapy works.
Spiders
Spiders are classes in Scrapy that define how to follow links and extract structured data from web pages. They are the heart of any Scrapy project.
Items
Items are containers for the extracted data. Think of them as Python dictionaries with a predefined structure.
Selectors
Selectors are used to parse HTML or XML documents and extract the desired information. Scrapy uses lxml
for parsing, which is both fast and efficient.
Step-by-Step Guide to Web Scraping with Scrapy
Now that we have our environment set up, let’s dive into creating a basic web scraping project using Scrapy.
Creating a New Scrapy Project
Open your CLI and navigate to the directory where you want to create your project. Run:
scrapy startproject my_project
This will create a new directory called my_project
with the following structure:
my_project/
scrapy.cfg
my_project/
__init__.py
items.py
middlewares.py
pipelines.py
settings.py
spiders/
__init__.py
Writing Your First Spider
Navigate to the spiders
directory and create a new Python file, for example, my_spider.py
. Here’s a simple spider that scrapes data from a website:
import scrapy
class MySpider(scrapy.Spider):
name = "my_spider"
start_urls = [
'http://example.com',
]
def parse(self, response):
title = response.css('title::text').get()
yield {
'title': title,
}
This spider will fetch the HTML content from http://example.com
, extract the title using a CSS selector, and yield it as an item.
Running Your Spider
To run your spider, navigate back to your project’s root directory and use:
scrapy crawl my_spider -o output.json
This command will execute the my_spider
spider and save the extracted data in a JSON file named output.json
.
Handling Different Data Types
Scrapy is versatile when it comes to handling various types of data. Here’s how you can handle common data types:
Extracting Text
To extract text from HTML elements, use the get()
method with CSS selectors or XPath expressions.
text = response.css('p::text').get()
Handling Images and Files
For downloading images and files, you can use Scrapy’s built-in media pipelines. Add the following lines to your settings.py
:
ITEM_PIPELINES = {
'scrapy.pipelines.images.ImagesPipeline': 1,
}
IMAGES_STORE = '/path/to/image/directory'
Then, in your spider, extract the image URLs and yield them as items:
image_urls = response.css('img::attr(src)').getall()
yield {
'image_urls': image_urls,
}
Scraping JSON Data
Sometimes, websites provide data in JSON format. You can directly parse and extract this data using Python’s json
module:
import json
response_data = json.loads(response.body)
Advanced Topics in Scrapy
Now that you have a solid foundation, let’s explore some advanced topics to enhance your web scraping skills.
Rotating Proxies and User Agents
To avoid getting blocked while scraping, it’s crucial to rotate proxies and user agents. You can achieve this using middlewares:
from scrapy import signals
import random
class RotateUserAgentMiddleware:
def process_request(self, request, spider):
ua = random.choice(spider.settings.get('USER_AGENTS'))
if ua:
request.headers['User-Agent'] = ua
# In settings.py
USER_AGENTS = [
'Mozilla/5.0...',
'Opera/9.80...',
]
Handling Pagination
Many websites use pagination to display content across multiple pages. You can handle this by following links to subsequent pages:
def parse(self, response):
for item in response.css('.item'):
yield {
'title': item.css('.title::text').get(),
}
next_page = response.css('a.next::attr(href)').get()
if next_page is not None:
yield response.follow(next_page, self.parse)
Using Scrapy with Splash for JavaScript Rendering
Some websites rely heavily on JavaScript to render content. To handle such cases, you can use Splash, a JavaScript rendering service compatible with Scrapy:
- Install Splash and start the server:
docker run -p 8050:8050 scrapinghub/splash
- Configure your spider to use Splash for requests:
from scrapy_splash import SplashRequest
class MySpider(scrapy.Spider):
name = "my_spider"
start_urls = [
'http://example.com',
]
def start_requests(self):
for url in self.start_urls:
yield SplashRequest(url, self.parse, args={'wait': 0.5})
Storing Data in Databases
You can store the extracted data directly into databases like MySQL or MongoDB using Scrapy pipelines. Here’s an example of a pipeline for storing items in MongoDB:
import pymongo
class MongoPipeline:
def open_spider(self, spider):
self.client = pymongo.MongoClient('localhost', 27017)
self.db = self.client['scrapy_items']
def process_item(self, item, spider):
self.db[spider.name].insert(dict(item))
return item
Conclusion
Scrapy is a powerful tool for web scraping and data extraction in Python. With its robust features and extensive community support, it stands out as one of the best frameworks for automating your web scraping tasks. From basic setups to advanced techniques like handling JavaScript rendering and rotating proxies, Scrapy offers everything you need to efficiently scrape websites and extract valuable data.
FAQs
1. What is the difference between Scrapy and BeautifulSoup?
Scrapy is a full-fledged web scraping framework that includes features like request scheduling, item pipelines, and middlewares. BeautifulSoup, on the other hand, is a library specifically designed for parsing HTML and XML documents. While you can use both together, Scrapy offers more comprehensive functionality for large-scale web scraping projects.
2. Can I use Scrapy to scrape dynamic websites?
Yes, you can use Scrapy to scrape dynamic websites by integrating it with tools like Splash or Selenium that handle JavaScript rendering. This allows Scrapy to interact with web pages as a real user would and extract data from dynamically loaded content.
3. How do I handle CAPTCHAs while using Scrapy?
Handling CAPTCHAs can be challenging, but there are several strategies you can employ:
- Use a service like 2Captcha to solve CAPTCHAs programmatically.
- Implement machine learning models to recognize and solve CAPTCHAs automatically.
- Rotate proxies and user agents to avoid triggering CAPTCHA challenges frequently.
4. What is the best way to store extracted data using Scrapy?
Scrapy provides several options for storing extracted data:
- Save data in JSON, CSV, or XML files using built-in feed exporters.
- Use item pipelines to store data directly into databases like MySQL, PostgreSQL, or MongoDB.
- Export data to cloud storage solutions like Amazon S3 or Google Cloud Storage for scalable and distributed access.
5. How can I optimize Scrapy for faster scraping?
To optimize Scrapy for faster scraping:
- Use concurrent requests by adjusting the
CONCURRENT_REQUESTS
setting insettings.py
. - Implement middlewares to handle retries and rotate proxies efficiently.
- Optimize your spiders to minimize unnecessary requests and extract only relevant data.
- Utilize distributed scraping with Scrapy Cluster or other distributed crawling frameworks for large-scale projects.