· Charlotte Will · webscraping · 5 min read
Optimizing Web Scraper Performance with Parallel Processing Techniques
Discover how to optimize web scraper performance with advanced parallel processing techniques. Learn about multithreading, multiprocessing, asyncio, and aiohttp libraries to enhance your web scraping speed and efficiency. Boost your data extraction capabilities with practical tips and best practices for Python-based web scraping.
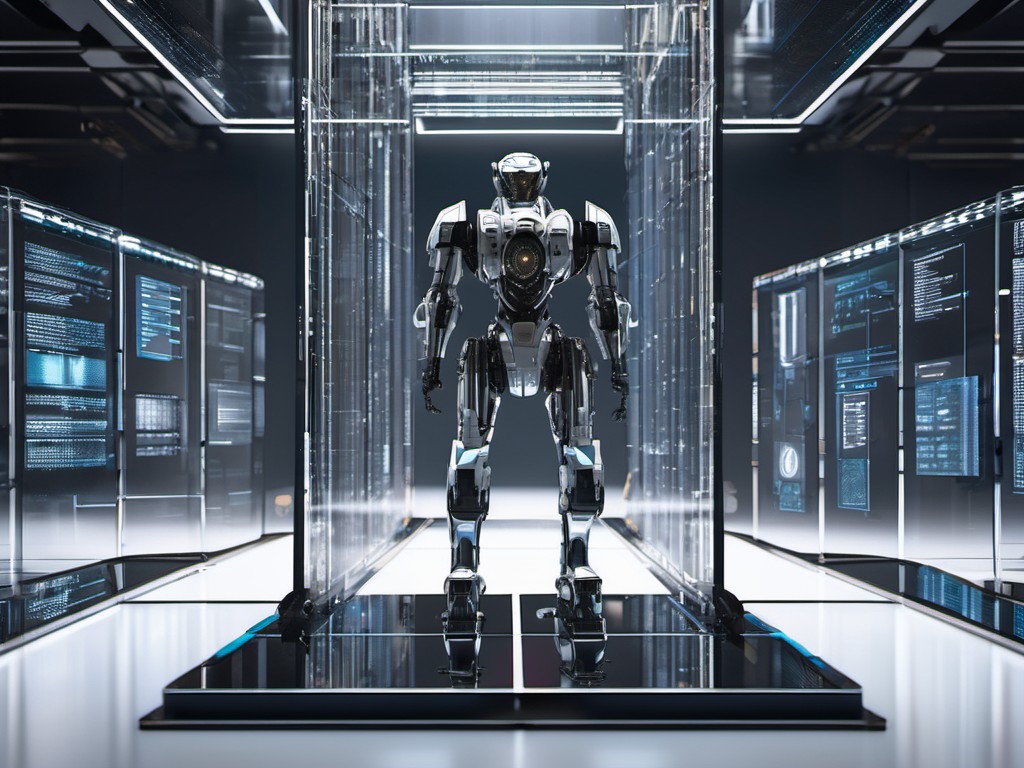
Web scraping has become an essential tool for data extraction and analysis, enabling businesses to gather competitive intelligence, monitor market trends, and extract valuable insights from the web. However, as websites grow more complex and data-intensive, traditional sequential web scrapers often struggle to keep up with the demands of modern data requirements. This is where parallel processing techniques come into play, offering a powerful way to optimize web scraper performance and efficiency.
Understanding Web Scraper Performance
Factors Affecting Web Scraper Speed
The performance of a web scraper can be influenced by several factors:
- Network Latency: The time it takes for data to travel between your server and the target website.
- Server Response Time: How quickly the target server responds to your requests.
- Website Complexity: The complexity of the HTML structure on the target site.
- Concurrency Limitations: Restrictions imposed by the target website on the number of simultaneous connections.
Why Optimize Web Scraper Performance?
Optimizing web scraper performance can lead to significant improvements in:
- Data Extraction Speed: Faster data extraction allows for more efficient analysis.
- Resource Utilization: Efficient use of CPU and memory resources.
- Scalability: The ability to handle larger datasets and complex websites.
Introduction to Parallel Processing
Parallel processing involves executing multiple tasks simultaneously, utilizing the full potential of modern multi-core processors. This can be achieved through various techniques such as multithreading, multiprocessing, and asynchronous programming.
Multithreading vs. Multiprocessing
- Multithreading: Uses multiple threads within a single process to execute tasks concurrently. It is efficient for I/O-bound tasks due to shared memory space but can face issues with CPU-bound tasks due to the Global Interpreter Lock (GIL) in Python.
- Multiprocessing: Involves using multiple processes, each with its own memory space. This avoids GIL issues and is suitable for CPU-bound tasks. However, it involves higher overhead due to interprocess communication.
Optimizing Web Scraper Performance with Python Libraries
1. Requests Library
The requests
library is popular for its simplicity but can be slow for large-scale scraping due to its synchronous nature. To optimize performance:
- Session Reuse: Reusing sessions can reduce overhead and speed up requests.
- Concurrent Requests: Use libraries like
concurrent.futures
orasyncio
withaiohttp
for asynchronous requests.
Example: Asynchronous Web Scraping with aiohttp
import aiohttp
import asyncio
async def fetch(session, url):
async with session.get(url) as response:
return await response.text()
async def main(urls):
async with aiohttp.ClientSession() as session:
tasks = [fetch(session, url) for url in urls]
results = await asyncio.gather(*tasks)
return results
urls = ["https://example.com/page1", "https://example.com/page2"]
results = asyncio.run(main(urls))
print(results)
2. BeautifulSoup and lxml
- BeautifulSoup: A popular library for parsing HTML and XML documents but can be slow with large datasets due to its synchronous nature.
- lxml: Faster than BeautifulSoup for parsing, especially with large datasets.
Example: Parallel Processing with BeautifulSoup and lxml
from bs4 import BeautifulSoup
import lxml
import concurrent.futures
def parse_page(url):
response = requests.get(url)
soup = BeautifulSoup(response.content, 'lxml')
# Extract data here
return extracted_data
urls = ["https://example.com/page1", "https://example.com/page2"]
with concurrent.futures.ThreadPoolExecutor() as executor:
results = list(executor.map(parse_page, urls))
3. Scrapy
Scrapy is a powerful and flexible web scraping framework that supports parallel processing out of the box.
- Twisted: The underlying asynchronous networking library used by Scrapy.
- Concurrent Requests: Scrapy uses a thread pool for concurrent requests, which can be configured to optimize performance.
Example: Parallel Processing with Scrapy
import scrapy
from scrapy.crawler import CrawlerProcess
class MySpider(scrapy.Spider):
name = "myspider"
start_urls = ["https://example.com/page1", "https://example.com/page2"]
def parse(self, response):
# Extract data here
yield {'data': extracted_data}
process = CrawlerProcess()
process.crawl(MySpider)
process.start()
Best Practices for Optimizing Web Scraper Performance
1. Use Efficient Libraries and Tools
Choose libraries that are optimized for speed and parallel processing, such as aiohttp
, asyncio
, and Scrapy.
2. Implement Rate Limiting
Respect the target website’s rate limits to avoid getting blocked. This can be done using libraries like tenacity
or built-in features in Scrapy.
3. Leverage Caching Mechanisms
Use caching mechanisms such as Redis or Memcached to store frequently accessed data, reducing the need for repeated requests.
4. Optimize Network Requests
Reduce network latency by using CDNs and optimizing DNS resolution.
5. Monitor and Adjust Resources
Continuously monitor CPU and memory usage, and adjust resources as needed to prevent bottlenecks.
Common Questions about Optimizing Web Scraper Performance
FAQs
What is the difference between multithreading and multiprocessing in web scraping?
- Multithreading involves using multiple threads within a single process, which is efficient for I/O-bound tasks but can face issues with CPU-bound tasks due to the GIL. Multiprocessing uses multiple processes with separate memory spaces, avoiding GIL issues and suitable for CPU-bound tasks but with higher overhead.
How does asyncio improve web scraper performance?
asyncio
allows for asynchronous programming, enabling you to perform multiple I/O-bound operations concurrently without creating threads or processes. This leads to more efficient resource utilization and faster data extraction.
What is the role of aiohttp in web scraping?
aiohttp
is an asynchronous HTTP client library for Python that integrates well withasyncio
. It allows you to make concurrent HTTP requests, improving the performance and efficiency of your web scraper.
Can Scrapy handle parallel processing?
- Yes, Scrapy supports parallel processing out of the box using its built-in thread pool for concurrent requests. You can configure settings such as
CONCURRENT_REQUESTS
to optimize performance.
- Yes, Scrapy supports parallel processing out of the box using its built-in thread pool for concurrent requests. You can configure settings such as
How do I optimize BeautifulSoup for faster parsing?
- Use a more efficient parser like lxml, and consider implementing parallel processing using libraries like
concurrent.futures
. Additionally, pre-fetching data in chunks can help reduce parse time.
- Use a more efficient parser like lxml, and consider implementing parallel processing using libraries like
Conclusion
Optimizing web scraper performance with parallel processing techniques is crucial for handling large datasets and complex websites efficiently. By leveraging Python libraries such as aiohttp
, asyncio
, BeautifulSoup, lxml, and Scrapy, you can significantly enhance your web scraper’s speed and efficiency. Always remember to monitor resource usage, respect rate limits, and continuously optimize your code for the best results.
Discuss how parallel processing can significantly enhance web scraper performance by referencing techniques mentioned in “Optimizing Web Scraper Performance with Parallel Processing Techniques”.
Explore the benefits of multithreading and multiprocessing for web scraping by delving into more details in “How to Optimize Web Scraping Performance with Parallel Processing”.
Highlight the importance of choosing the right Python libraries for web scraping efficiency, as discussed in “Advanced Techniques for Python Web Scraping” and how they can be optimized using parallel processing techniques.