· Charlotte Will · webscraping · 7 min read
Mastering Scrapy Extensions and Plugins for Enhanced Functionality
Master Scrapy extensions and plugins to enhance your web scraping projects. Learn how to install Scrapy extensions, create custom middleware, and leverage advanced techniques for improved performance and functionality.
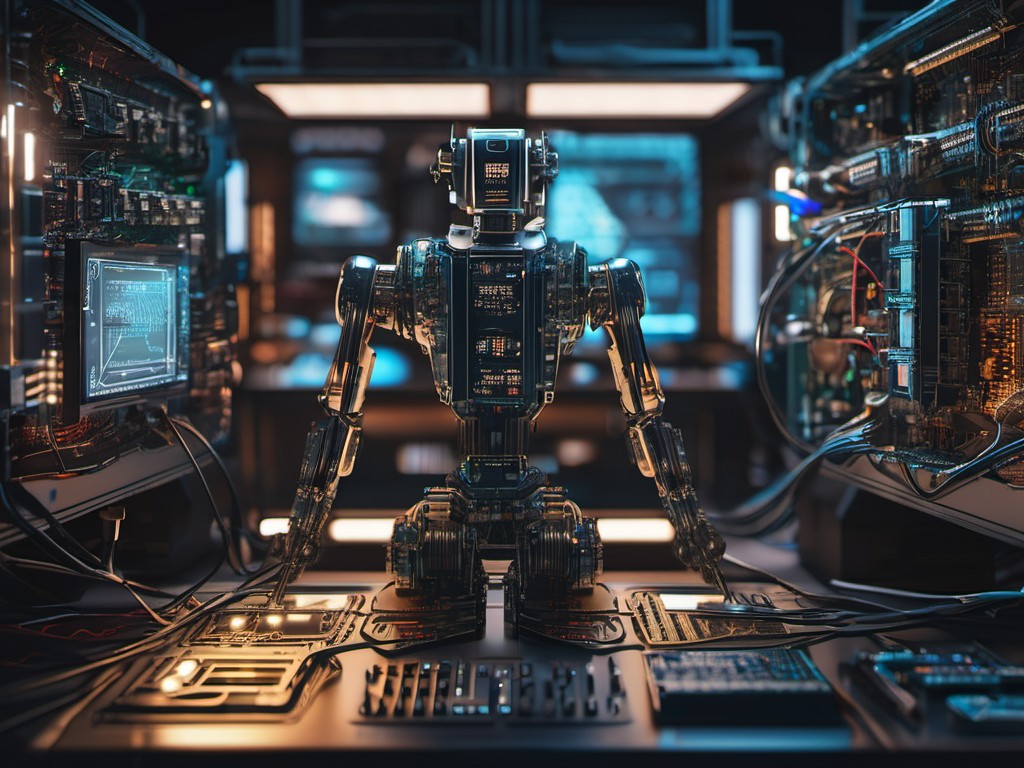
Introduction
Web scraping has become an essential tool in the modern data landscape. It allows businesses to extract valuable information from websites, enabling informed decision-making and competitive advantage. One of the most powerful tools available for web scraping is Scrapy, a popular open-source framework written in Python. While Scrapy itself offers robust functionalities out of the box, extending its capabilities with extensions and plugins can significantly enhance your web scraping projects.
This comprehensive guide will walk you through mastering Scrapy extensions and plugins, providing practical advice on how to install them, create custom middleware, and leverage advanced techniques for improved performance and functionality. Whether you’re a beginner or an experienced developer, this tutorial will equip you with the knowledge needed to maximize your web scraping efforts.
Understanding Scrapy Extensions and Plugins
Before diving into how to use Scrapy extensions and plugins, it’s essential to understand what they are and why they are crucial for enhancing functionality.
What Are Scrapy Extensions?
Scrapy extensions are packages or modules that provide additional features or functionalities to the base Scrapy framework. They can be used to simplify common tasks, integrate with other tools or services, and improve overall performance. Examples include extensions for handling proxies, managing cookies, and interacting with databases.
What Are Scrapy Plugins?
Scrapy plugins are similar to extensions but often refer to more specific components like middleware, pipelines, or custom settings. Middleware, for instance, allows you to intercept requests and responses, enabling tasks such as logging, data transformation, or rate limiting. Pipelines handle the processing of extracted data, allowing for tasks such as cleaning, storing, or exporting the data.
How to Install Scrapy Extensions
Installing Scrapy extensions is a straightforward process, thanks to Python’s package management system, pip
. Here’s how you can add extensions to your Scrapy project:
Step-by-Step Guide to Installing Extensions
- Create a Virtual Environment:
python -m venv myenv source myenv/bin/activate # On Windows use `myenv\Scripts\activate`
- Install Scrapy and Required Extensions:
pip install scrapy scrapy-splash
- Enable the Extension in Your Scrapy Project: Create or modify your
settings.py
file to include the extension:# settings.py DOWNLOADER_MIDDLEWARES = { 'scrapy_splash.SplashCookiesMiddleware': 723, 'scrapy_splash.SplashMiddleware': 725, 'scrapy.downloadermiddlewares.httpcompression.HttpCompressionMiddleware': 810, } SPIDER_MIDDLEWARES = { 'scrapy_splash.SplashDeduplicateArgsMiddleware': 100, }
- Use the Extension in Your Spider:
# my_spider.py import scrapy from scrapy_splash import SplashRequest class MySpider(scrapy.Spider): name = 'myspider' start_urls = ['http://example.com'] def start_requests(self): for url in self.start_urls: yield SplashRequest(url, self.parse, args={'wait': 2}) def parse(self, response): print(response.body)
By following these steps, you can easily integrate extensions into your Scrapy projects, enhancing their capabilities and simplifying complex tasks.
Best Scrapy Plugins for Data Extraction
While the base Scrapy framework is powerful, certain plugins can significantly enhance data extraction capabilities. Here are some of the best Scrapy plugins for data extraction:
1. Scrapy-Splash
Scrapy-Splash integrates Splash, a lightweight browser with JavaScript support, into your Scrapy projects. This allows you to scrape dynamic content generated by JavaScript.
pip install scrapy-splash
Usage Example:
from scrapy_splash import SplashRequest
response = SplashRequest(url, self.parse_js, args={'wait': 2})
yield response
2. Scrapy-Redis
Scrapy-Redis is an extension that allows you to use Redis as a message queue broker for Scrapy. This can significantly boost your project’s performance by enabling distributed scraping and load balancing.
pip install scrapy-redis
Usage Example:
# settings.py
SCHEDULER = "scrapy_redis.scheduler.Scheduler"
DUPEFILTER_CLASS = "scrapy_redis.dupefilter.RFPDupeFilter"
3. Scrapy-Playwright
Scrapy-Playwright integrates Playwright, a framework for browser automation, into your Scrapy projects. This allows you to handle complex interactions and extract data from modern JavaScript-heavy websites.
pip install scrapy-playwright
Usage Example:
from scrapy_playwright.page import PageMethod
@playwright.run_in_executor
def run(page: Page, request) -> None:
page.on("requestfinished", lambda r: print(r.url))
page.on("response", lambda r: print(r.status))
page.goto(request.url)
4. Scrapy-Rotating-Proxies
Scrapy-Rotating-Proxies allows you to rotate proxies automatically, helping you to avoid IP bans and improve scraping efficiency.
pip install scrapy-rotating-proxies
Usage Example:
# settings.py
PROXY_POOL_ENABLED = True
PROXY_POOL_PAGE_RETRY_TIMES = 10
DOWNLOADER_MIDDLEWARES = {
'scrapy.downloadermiddlewares.retry.RetryMiddleware': None,
'scrapy_proxies.RandomProxy': 100,
'scrapy.downloadermiddlewares.httpproxy.HttpProxyMiddleware': 110,
}
Boosting Scrapy Performance with Middleware
Scrapy middleware allows you to intercept and process requests and responses, enhancing performance and functionality in your web scraping projects. Here’s how you can leverage custom middleware to boost Scrapy’s performance:
Creating Custom Scrapy Middleware Components
- Define Your Custom Middleware:
from scrapy import signals from scrapy.http import HtmlResponse class CustomMiddleware: @classmethod def from_crawler(cls, crawler): s = cls() crawler.signals.connect(s.spider_opened, signal=signals.spider_opened) return s def process_response(self, request, response): if isinstance(response, HtmlResponse): response.body = response.body.replace(b'<div>', b'<!--<div>-->\n') return response
- Enable Your Middleware in
settings.py
:# settings.py DOWNLOADER_MIDDLEWARES = { 'myproject.middlewares.CustomMiddleware': 543, }
Advanced Web Scraping Techniques with Custom Middleware
- Rate Limiting: Control the rate of requests to avoid overwhelming servers and getting banned.
- Data Transformation: Modify data on-the-fly as it’s being processed, such as cleaning HTML tags or transforming data formats.
- Logging and Monitoring: Log detailed information about requests and responses for debugging and performance monitoring.
For a deeper understanding of how Scrapy can be utilized in large-scale web scraping projects, refer to our guide on Mastering Scrapy for Large-Scale Web Scraping Projects.
Creating Custom Scrapy Pipeline Components
Scrapy pipelines handle the processing of extracted data, including cleaning, storing, and exporting. Here’s how you can create custom pipeline components to enhance your web scraping projects:
Step-by-Step Guide to Creating a Custom Pipeline
- Define Your Custom Pipeline:
class CustomPipeline: def process_item(self, item, spider): # Perform data processing tasks here item['cleaned_data'] = self.clean_data(item['raw_data']) return item def clean_data(self, data): # Implement your data cleaning logic cleaned_data = data.strip().lower() return cleaned_data
- Enable Your Pipeline in
settings.py
:# settings.py ITEM_PIPELINES = { 'myproject.pipelines.CustomPipeline': 300, }
Enhancing Data Processing with Custom Pipelines
- Data Cleaning: Remove unwanted characters, normalize data formats, and ensure data quality.
- Data Storage: Store extracted data in databases, file systems, or cloud services for further analysis or integration.
- Data Export: Export processed data to CSV, JSON, or other formats for reporting or sharing with stakeholders.
To learn more about custom plugins and middleware for enhancing your web scraping projects, see our article on Scrapy Middleware: Enhancing Your Web Scraping Projects with Custom Plugins.
FAQs
What are some common use cases for Scrapy extensions?
Scrapy extensions can be used for various tasks, including handling proxies, managing cookies, integrating with databases, and enhancing data extraction capabilities.
How do I install custom middleware in my Scrapy project?
To install custom middleware in your Scrapy project, define your middleware class and enable it in the DOWNLOADER_MIDDLEWARES
or SPIDER_MIDDLEWARES
settings in your settings.py
file.
Can I use multiple extensions in my Scrapy project?
Yes, you can use multiple extensions in your Scrapy project. Simply install the desired extensions and enable them in your settings.py
file. Make sure to configure any necessary settings for each extension.
How do I create a custom pipeline in Scrapy?
To create a custom pipeline in Scrapy, define your pipeline class with a process_item
method and enable it in the ITEM_PIPELINES
setting in your settings.py
file.
What is the best way to handle dynamic content in Scrapy?
The best way to handle dynamic content in Scrapy is to use extensions that support JavaScript rendering, such as Scrapy-Splash or Scrapy-Playwright. These extensions allow you to scrape content generated by JavaScript.
Conclusion
Mastering Scrapy extensions and plugins can significantly enhance your web scraping functionality, enabling you to extract more data, improve performance, and handle complex tasks with ease. By understanding how to install and configure extensions, create custom middleware and pipelines, and leverage advanced techniques, you can unlock the full potential of Scrapy for your web scraping projects.
Stay up-to-date with the latest trends and best practices in SEO writing by following industry experts and regularly updating your knowledge and skills. With dedication and practice, you’ll become a master of Scrapy extensions and plugins, capable of tackling even the most challenging web scraping tasks. Happy scraping!
For insights into building custom data warehouses and enhancing business intelligence using Amazon SP-API, check out our comprehensive guide on Building a Custom Data Warehouse for Enhanced Business Insights Using Amazon SP-API.