· Charlotte Will · webscraping · 4 min read
How to Scrape Data from Websites with Complex Structures
Learn how to scrape data from complex websites using Python, BeautifulSoup, lxml, and Selenium. This comprehensive guide covers practical techniques and advanced strategies for effective web scraping.
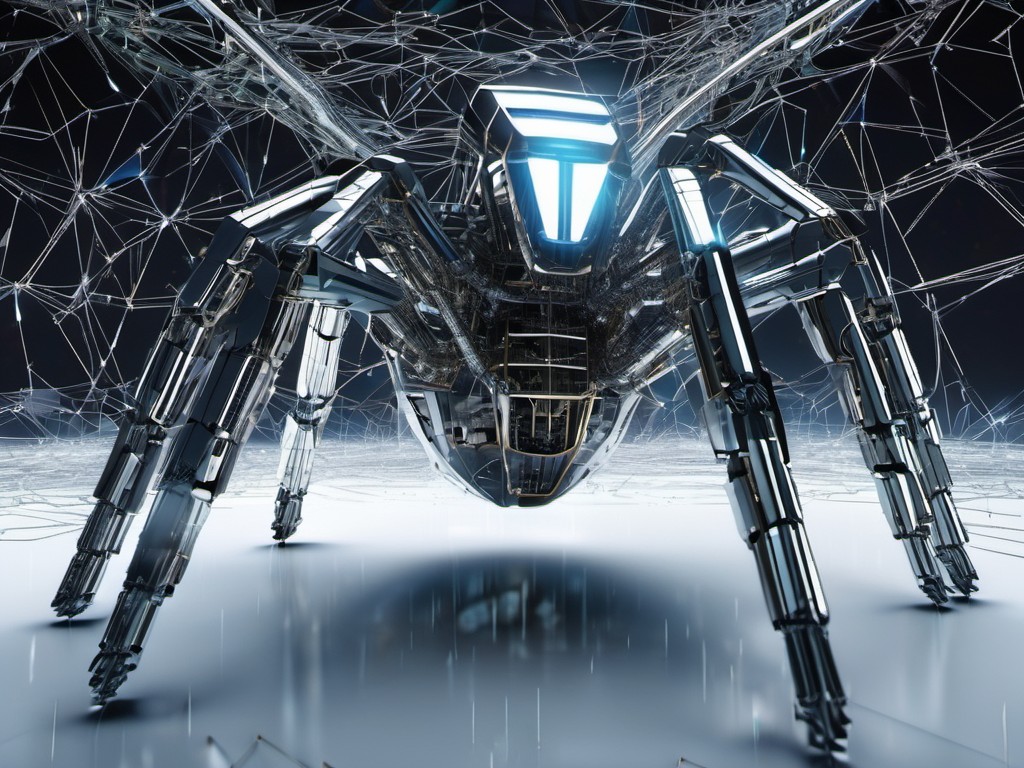
Web scraping has become an indispensable tool for extracting data from websites, especially when dealing with complex structures. Whether you’re a beginner looking to dive into web scraping or an advanced user seeking to optimize your data extraction techniques, this comprehensive guide will provide you with practical and actionable advice.
Understanding Web Scraping
Web scraping is the process of extracting data from websites. This data can be used for various purposes such as market analysis, price monitoring, or content aggregation. However, not all websites are created equal. Some have simple structures that make data extraction straightforward, while others have complex structures that require more advanced techniques.
Challenges of Scraping Complex Websites
Complex websites pose several challenges for web scrapers. These challenges include:
- Dynamic content loading using JavaScript.
- Intricate HTML structures with nested elements.
- Anti-scraping measures like CAPTCHAs and bot detection.
To overcome these challenges, you need a robust set of tools and techniques. This article will focus on using Python along with libraries such as BeautifulSoup, lxml, and Selenium to scrape data from complex structures effectively.
Getting Started with Python
Python is one of the most popular languages for web scraping due to its simplicity and extensive library support. Before diving into the code, ensure you have Python installed on your machine along with the required libraries:
pip install requests beautifulsoup4 lxml selenium pandas
Basic Web Scraping with BeautifulSoup
BeautifulSoup is a powerful library for parsing HTML and XML documents. It creates a parse tree from the page’s source code, which can be used to extract data in a hierarchical manner.
Example: Basic Data Extraction
import requests
from bs4 import BeautifulSoup
url = "https://example.com"
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
# Extracting the title
title = soup.title.string
print("Title:", title)
While BeautifulSoup is excellent for simple structures, complex websites require more sophisticated techniques.
Advanced Scraping with lxml
lxml is another powerful library that supports both HTML and XML parsing. It offers faster parsing compared to BeautifulSoup but has a steeper learning curve.
Example: Advanced Data Extraction with lxml
from lxml import html
import requests
url = "https://example.com"
response = requests.get(url)
tree = html.fromstring(response.text)
# Extracting data using XPath
titles = tree.xpath('//title/text()')
print("Titles:", titles)
Handling Dynamic Content with Selenium
Selenium is a powerful tool for automating web browsers. It’s particularly useful when dealing with dynamic content loaded using JavaScript.
Example: Scraping Dynamic Content
from selenium import webdriver
from bs4 import BeautifulSoup
import time
driver = webdriver.Chrome() # Ensure you have the ChromeDriver installed
url = "https://example.com"
driver.get(url)
time.sleep(5) # Allow time for JavaScript to load content
page_source = driver.page_source
soup = BeautifulSoup(page_source, 'html.parser')
# Extract data after JavaScript has loaded the content
dynamic_content = soup.find('div', {'id': 'dynamic-content'}).text
print("Dynamic Content:", dynamic_content)
driver.quit()
Combining Techniques
In many cases, you’ll need to combine different techniques to effectively scrape complex websites. For instance, you might use Selenium to handle dynamic content and then parse the resulting HTML with BeautifulSoup or lxml.
Data Cleaning Post-Scrape
After scraping data, it’s crucial to clean it before using it for analysis. Libraries like Pandas can automate this process, ensuring your data is accurate and ready for use.
To learn more about automating data cleaning, refer to our article: How to Automate Data Cleaning Post-Scrape with Pandas.
Advanced Techniques for Data Extraction
For more advanced data extraction techniques, consider exploring the capabilities of BeautifulSoup and lxml in depth. Our article: Advanced Techniques for Data Extraction Using BeautifulSoup and lxml provides a deep dive into these libraries.
Conclusion
Scraping data from websites with complex structures requires a combination of tools and techniques. By understanding the challenges and leveraging powerful libraries like BeautifulSoup, lxml, and Selenium, you can effectively extract the data you need. Whether you’re just getting started or looking to optimize your existing workflows, this guide provides practical advice to help you succeed in web scraping.
FAQs
1. What is web scraping?
Web scraping is the process of extracting data from websites by automatically fetching and parsing content.
2. Why is it important to handle dynamic content?
Dynamic content loaded using JavaScript can change the structure of a website significantly, making it crucial to handle such content accurately during web scraping.
3. How do I choose between BeautifulSoup and lxml for parsing HTML?
BeautifulSoup is easier to use and more forgiving with malformed HTML, while lxml is faster but has a steeper learning curve. Choose based on your specific needs and comfort level.
4. What is Selenium used for in web scraping?
Selenium is used to automate web browsers and handle dynamic content that requires JavaScript execution.
5. How can I clean data after scraping?
You can use libraries like Pandas to automate the cleaning of scraped data, ensuring it’s accurate and ready for analysis.