· Charlotte Will · webscraping · 5 min read
How to Scrape Data from Infinite Scroll Websites
Learn how to scrape data from infinite scroll websites using tools like Selenium and BeautifulSoup. This comprehensive guide provides step-by-step instructions, common challenges, and best practices for handling dynamic content loading, rate limiting, and CAPTCHA challenges. Enhance your web scraping skills today!
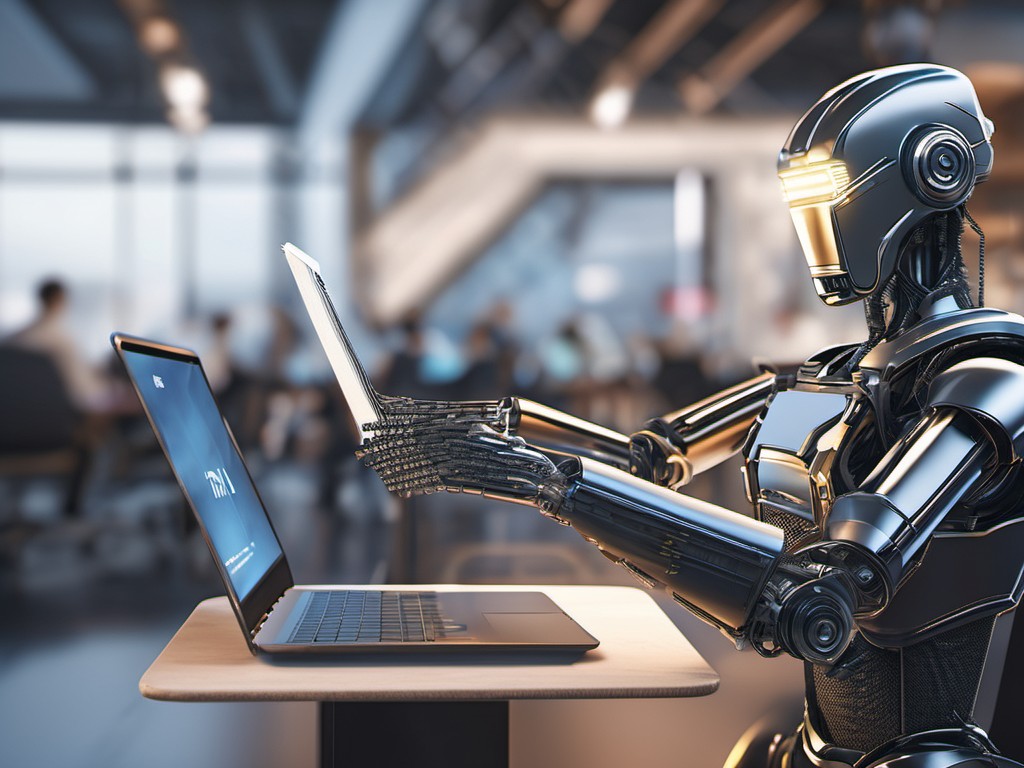
Introduction
Web scraping has become an essential tool for extracting valuable data from websites, but traditional methods often falter when encountering infinite scroll pages. These websites load content dynamically as you scroll down, presenting unique challenges for scrapers. However, with the right tools and techniques, you can efficiently scrape data from infinite scroll websites. This guide will walk you through the process step-by-step.
Understanding Infinite Scroll
What is Infinite Scroll?
Infinite scroll is a web design technique where content continues to load as the user scrolls down the page, instead of having to click through multiple pages. Popularized by social media platforms like Facebook and Twitter, infinite scroll improves user experience but complicates data extraction processes.
Why Scrape Infinite Scroll Websites?
Infinite scroll websites often contain a wealth of data that can be invaluable for market research, competitive analysis, or even personal projects. By scraping these sites effectively, you can gather large datasets quickly and efficiently.
Tools and Libraries for Infinite Scroll Scraping
Selenium
Selenium is a powerful tool that automates web browser interactions. It’s ideal for handling infinite scroll websites because it can simulate user actions like scrolling, clicking, and typing. By using Selenium, you can interact with dynamic content in the same way a human user would.
BeautifulSoup
BeautifulSoup is a popular Python library used for parsing HTML and XML documents. While it doesn’t handle JavaScript-rendered content natively, it works well in conjunction with Selenium to scrape data from infinite scroll websites once the dynamic content has been loaded.
Puppeteer
Puppeteer is a Node.js library that provides a high-level API to control headless Chrome or Chromium over the DevTools Protocol. It’s excellent for handling complex, JavaScript-heavy sites and can be used in conjunction with tools like BeautifulSoup or Scrapy for data extraction.
Step-by-Step Guide to Scrape Data from Infinite Scroll Websites
Setting Up Your Environment
Before you start scraping, ensure you have the necessary tools installed. For Python, you’ll need Selenium and BeautifulSoup:
pip install selenium beautifulsoup4
You’ll also need a web driver compatible with your browser (e.g., ChromeDriver for Google Chrome).
Identifying the Infinite Scroll Elements
The first step is to identify the elements that trigger the infinite scroll. Common elements include buttons, links, or specific HTML tags. Use browser developer tools to inspect the page and locate these elements.
Simulating User Actions
With Selenium, you can simulate user actions to load more content. Here’s an example in Python:
from selenium import webdriver
import time
# Set up the web driver
driver = webdriver.Chrome()
# Load the page with infinite scroll
driver.get('https://example.com/infinite-scroll-page')
# Wait for the initial content to load
time.sleep(5)
# Scroll down to trigger loading more content
driver.execute_script("window.scrollTo(0, document.body.scrollHeight);")
# Repeat scrolling until all content is loaded
while True:
time.sleep(3) # Wait for new content to load
driver.execute_script("window.scrollTo(0, document.body.scrollHeight);")
if not driver.find_elements_by_css_selector('.load-more-button'):
break
Extracting and Cleaning Data
Once all the content is loaded, you can use BeautifulSoup to parse the HTML and extract the desired data:
from bs4 import BeautifulSoup
import requests
# Get the page source after loading all content
html = driver.page_source
soup = BeautifulSoup(html, 'html.parser')
# Extract the data you need (e.g., titles and links)
titles = soup.find_all('h2', class_='title')
links = [a['href'] for a in soup.find_all('a', href=True)]
# Close the web driver
driver.quit()
Handling Common Challenges
Rate Limiting
Many websites impose rate limits to prevent abuse and ensure fair usage. To handle rate limiting, you can add delays between requests or use rotating proxies to distribute your traffic across multiple IP addresses.
CAPTCHA Challenges
CAPTCHAs are designed to block automated scraping. While there’s no foolproof solution, using headless browsers like Puppeteer or Selenium can help mimic human behavior more effectively. Additionally, some services offer CAPTCHA-solving solutions for a fee.
Dynamic Content Loading
Infinite scroll websites often load content dynamically using JavaScript. Tools like Selenium and Puppeteer can handle this by rendering the page as a human user would, allowing you to scrape data from the fully loaded content.
FAQ Section
1. Can I scrape infinite scroll websites without using browser automation tools? While it’s technically possible to scrape infinite scroll websites using only HTTP requests, this approach is often impractical and prone to errors. Browser automation tools like Selenium and Puppeteer provide a more reliable solution by simulating user interactions.
2. How can I avoid getting my IP banned while scraping? To minimize the risk of getting your IP banned, use rotating proxies, respect rate limits, and add delays between requests. Additionally, consider using a VPN service to mask your IP address.
3. What is the best programming language for web scraping infinite scroll websites? Python is widely regarded as the best programming language for web scraping due to its extensive libraries and community support. Libraries like Selenium, BeautifulSoup, and Scrapy make it easy to automate browser interactions and extract data from web pages.
4. How can I handle JavaScript-rendered content in infinite scroll websites? To handle JavaScript-rendered content, use headless browsers like Puppeteer or Selenium that can render the page as a human user would. These tools allow you to interact with dynamic content and scrape data from fully loaded pages.
5. What are some ethical considerations when scraping infinite scroll websites? When web scraping, it’s essential to respect the target website’s terms of service and robots.txt file. Avoid overloading the server with too many requests simultaneously, and always consider the potential legal implications of your actions. Additionally, it’s crucial to use the extracted data responsibly and ethically.