· Charlotte Will · webscraping · 4 min read
Automating Data Cleaning Post-Scrape with Pandas
Discover how to automate data cleaning post-scrape using Pandas. This comprehensive guide provides step-by-step instructions, best practices, and practical tips to enhance your web scraping workflow. Boost efficiency and accuracy in your data processing tasks today!
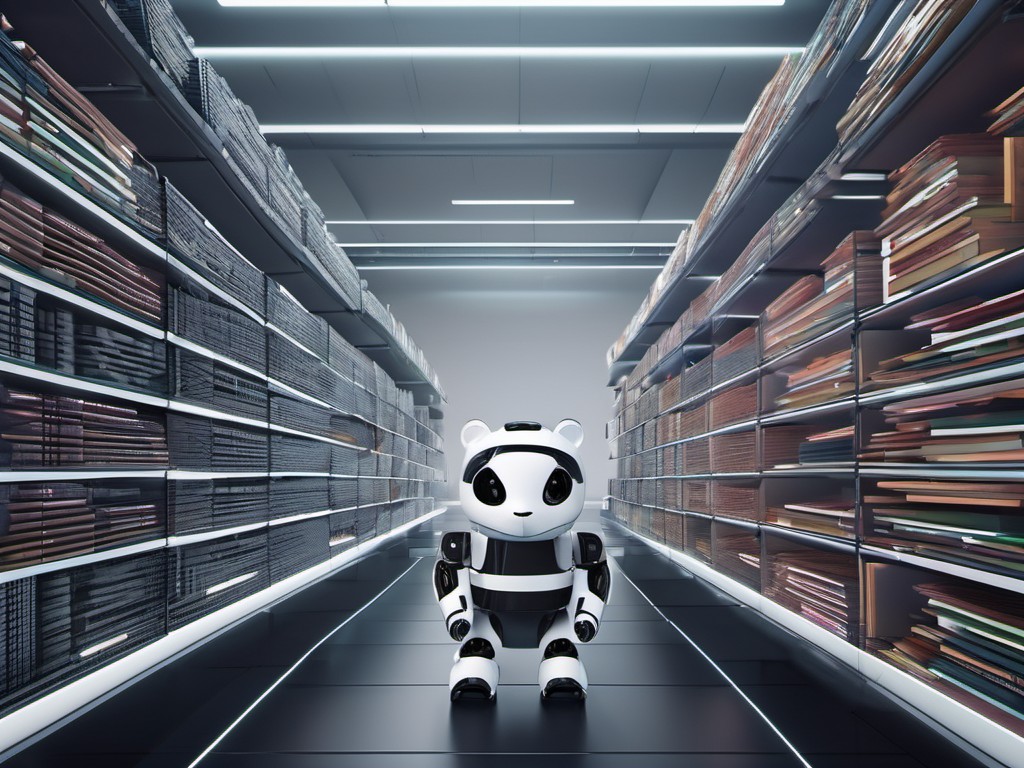
Automate data cleaning, streamline your workflow, and improve data quality post-scrape with Pandas.
Introduction to Automated Data Cleaning
Web scraping can be a powerful tool for gathering valuable data from the web, but the data collected is often messy and incomplete. Manual data cleaning is time-consuming and error-prone. Automating data cleaning post-scrape with Python libraries like Pandas can significantly enhance your efficiency and accuracy.
Why Use Pandas for Post-Scrape Data Cleaning?
Pandas is a robust library in the Python ecosystem that provides high-level data structures and manipulation tools. It’s particularly well-suited for post-scrape data cleaning due to its versatile functionality, ease of use, and performance. Here are some key reasons why Pandas stands out:
- DataFrame Structure: Pandas uses a DataFrame, which is analogous to a spreadsheet or SQL table, making it intuitive for those familiar with tabular data.
- Comprehensive Functions: Pandas offers an extensive range of functions for cleaning, manipulating, and analyzing data.
- Performance: It’s built on top of NumPy, ensuring fast computations even with large datasets.
Step-by-Step Guide to Automate Data Cleaning
1. Import Necessary Libraries
First, ensure you have the necessary libraries installed:
pip install pandas requests beautifulsoup4
Next, import them in your Python script:
import pandas as pd
import requests
from bs4 import BeautifulSoup
2. Web Scraping Data
For this guide, let’s assume you have already scraped data and stored it in a list of dictionaries or a CSV file. Here’s an example of how to start:
# Example list of dictionaries
data = [
{'name': 'Alice', 'age': '25', 'city': 'New York'},
{'name': 'Bob', 'age': '', 'city': 'Los Angeles'},
{'name': 'Charlie', 'age': None, 'city': ''}
]
3. Load Data into a Pandas DataFrame
Convert your data to a DataFrame for easy manipulation:
df = pd.DataFrame(data)
print(df)
4. Handling Missing Values
Missing values are common in scraped data. Use dropna()
, fillna()
, and interpolate()
to handle them:
# Drop rows with missing values
clean_df = df.dropna()
# Fill missing values (e.g., 'age' column with a default value)
df['age'] = df['age'].fillna(20)
# Interpolate missing values (useful for numerical data)
df['age'] = df['age'].interpolate()
5. Data Type Conversion
Ensure columns have the correct data types:
df['age'] = pd.to_numeric(df['age'], errors='coerce')
df['city'] = df['city'].astype('string')
6. Removing Duplicates
Remove duplicate rows to maintain data integrity:
clean_df = clean_df.drop_duplicates()
7. Handling Outliers
Outliers can distort your analysis. Use statistical methods or domain knowledge to detect and handle them:
Q1 = df['age'].quantile(0.25)
Q3 = df['age'].quantile(0.75)
IQR = Q3 - Q1
df = df[~((df['age'] < (Q1 - 1.5 * IQR)) | (df['age'] > (Q3 + 1.5 * IQR)))]
8. String Manipulation
Clean and standardize text data:
# Remove leading/trailing whitespace
df['city'] = df['city'].str.strip()
# Convert to lowercase
df['name'] = df['name'].str.lower()
9. Advanced Data Cleaning Techniques
For more complex cleaning tasks, consider using regular expressions (re
library) and custom functions:
import re
# Remove special characters from 'city' column
df['city'] = df['city'].str.replace('[^A-Za-z\s]', '', regex=True)
Best Practices for Efficient Data Cleaning
1. Understand Your Data
Before cleaning, thoroughly understand the structure and nature of your data. This helps in identifying the appropriate cleaning steps.
2. Use Descriptive Statistics
Descriptive statistics (mean, median, mode) can provide insights into your data’s distribution and help identify outliers or anomalies.
3. Automate Repetitive Tasks
Leverage Python’s capability to automate repetitive tasks by writing functions or using loops. This saves time and reduces errors.
4. Document Your Processes
Maintain clear documentation of your data cleaning processes. This is crucial for reproducibility and future reference.
5. Validate Cleaning Steps
Regularly validate the results of your cleaning steps to ensure they are producing the desired outcomes.
FAQs
Can I use Pandas for real-time data cleaning? Yes, Pandas is well-suited for both batch and real-time data cleaning tasks due to its performance and flexibility.
How do I handle large datasets with Pandas? For very large datasets, consider using Dask, which integrates seamlessly with Pandas and allows out-of-core computations.
Can I automate data cleaning with other libraries besides Pandas? Yes, libraries like NumPy, PySpark, and even SQL can be used for automated data cleaning, depending on your specific needs and dataset size.
What are some common challenges in data cleaning post-scrape? Common challenges include handling missing values, inconsistent data formats, and removing duplicates. Proper planning and use of tools like Pandas can mitigate these issues.
How can I ensure the quality of my cleaned data? Regularly validate your cleaning processes with statistical checks and domain-specific validation rules to maintain high data quality.
Conclusion
Automating data cleaning post-scrape with Pandas is a powerful way to enhance your data processing workflow. By leveraging Pandas’ robust features, you can efficiently handle missing values, clean text data, manage outliers, and ensure high data quality. Following best practices will further improve the reliability and effectiveness of your data cleaning processes.
If you’re new to web scraping, you might want to check out our guide on how to scrape JSON data using Python. Additionally, for more advanced techniques involving machine learning models, see our article on automating data cleaning post-scrape with Pandas and Machine Learning Models.