· Charlotte Will · webscraping · 5 min read
Advanced Error Handling Techniques in Web Scraping
Learn advanced error handling techniques to enhance your web scraping projects. Discover practical methods for managing 404 errors, implementing retry mechanisms, and more. Optimize your scrapers with Selenium and effective logging strategies.
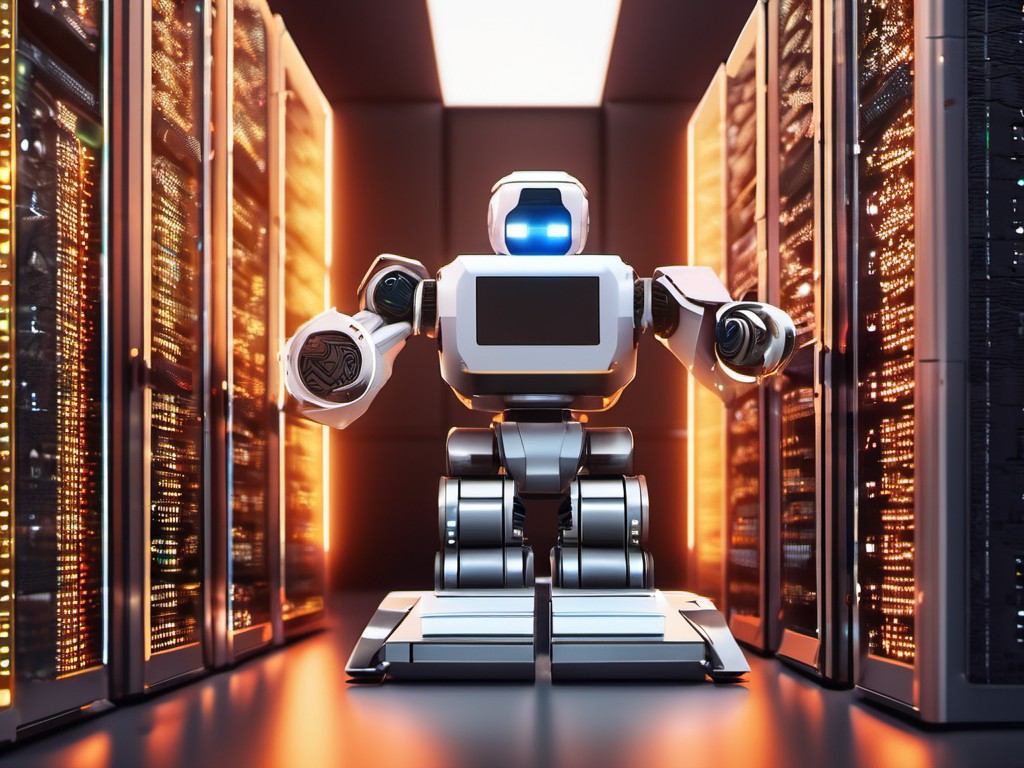
Advanced Error Handling Techniques in Web Scraping
Web scraping is an essential technique for extracting data from websites, but it comes with its own set of challenges, particularly when dealing with errors. Advanced error handling techniques can significantly enhance the robustness and efficiency of your web scraping projects. In this article, we will explore various strategies to manage common errors and improve the overall reliability of your scraping scripts.
Understanding Error Handling in Web Scraping
Error handling is crucial for maintaining the stability and effectiveness of a web scraper. Errors can occur due to various reasons such as network issues, changes in website structure, or server-side protections against automated access. By implementing advanced error handling techniques, you can ensure that your scraper continues to function even when errors arise.
Common Errors in Web Scraping
Before diving into the techniques, let’s identify some common errors encountered during web scraping:
- 404 Errors: Occur when a requested resource could not be found on the server.
- 5XX Errors: Indicate server-side issues that prevent the successful retrieval of data.
- Timeouts: Happen when the server takes too long to respond or there are network delays.
- Captchas and Bot Detection Mechanisms: Websites often use these to block automated access.
- JavaScript-rendered Content: Some websites load content dynamically using JavaScript, which can be challenging to scrape.
Advanced Error Handling Techniques
1. Retry Mechanisms
Implementing a retry mechanism allows your scraper to attempt fetching a resource multiple times before giving up. This approach is particularly useful for handling transient errors like timeouts or temporary server unavailability.
import requests
from requests.exceptions import RequestException
def fetch_with_retries(url, retries=3):
attempts = 0
while attempts < retries:
try:
response = requests.get(url)
if response.status_code == 200:
return response.content
except RequestException as e:
print(f"Error occurred: {e}. Retrying...")
attempts += 1
return None
2. Exception Handling
Catching exceptions is a fundamental part of error handling in web scraping. By using try-except blocks, you can manage errors gracefully and ensure that your script continues to run.
import requests
from requests.exceptions import HTTPError
def fetch_data(url):
try:
response = requests.get(url)
response.raise_for_status() # Raises an HTTPError for bad responses (4xx or 5xx)
except HTTPError as http_err:
print(f"HTTP error occurred: {http_err}")
except Exception as err:
print(f"An error occurred: {err}")
3. Handling 404 Errors
A 404 error indicates that the requested resource is not available on the server. Implementing a check for these errors can help you handle them appropriately, such as skipping the URL or marking it for later inspection.
def check_404(url):
response = requests.head(url)
if response.status_code == 404:
print(f"Resource not found: {url}")
return False
return True
4. Error Logging
Logging errors is essential for diagnosing issues and improving the reliability of your scraper over time. By keeping a record of errors, you can identify patterns and address underlying problems more effectively.
import logging
from requests.exceptions import RequestException
logging.basicConfig(filename='scraping_errors.log', level=logging.ERROR)
def fetch_data_with_logging(url):
try:
response = requests.get(url)
response.raise_for_status()
except RequestException as e:
logging.error(f"Error fetching {url}: {e}")
5. Web Scraping with Selenium Error Handling
When dealing with JavaScript-rendered content, tools like Selenium can be invaluable. However, errors specific to browser automation must also be handled effectively.
from selenium import webdriver
from selenium.common.exceptions import NoSuchElementException, TimeoutException
import time
def fetch_data_with_selenium(url):
driver = webdriver.Chrome()
driver.get(url)
try:
element = driver.find_element_by_id('target-element')
return element.text
except NoSuchElementException:
print("Element not found")
except TimeoutException:
print("Timed out waiting for the element to appear")
finally:
driver.quit()
Practical Tips for Effective Error Handling
- Use Exponential Backoff: When implementing retry mechanisms, consider using exponential backoff to avoid overwhelming the server with rapid retries.
- Monitor Server Load: Be mindful of the load you place on the target server to prevent your IP from being blocked.
- Rotate Proxies and User-Agents: Using different proxies and user-agent strings can help you bypass some common blocking mechanisms.
- Implement Circuit Breakers: Prevent a cascade of failures by using circuit breakers that temporarily halt retries when too many consecutive errors occur.
Real-world Applications
Advanced error handling techniques are critical for various real-world applications, from competitive intelligence to social media monitoring. By incorporating robust error handling, you can significantly enhance the reliability of your scraping projects. For more insights into advanced web scraping techniques, refer to our articles on Advanced Techniques for Social Media Web Scraping and Advanced Techniques for Competitive Intelligence Web Scraping.
Conclusion
Implementing advanced error handling techniques is crucial for building reliable web scrapers. By managing common errors like 404s, timeouts, and server issues effectively, you can ensure that your scraper continues to function smoothly even in the face of adversity. Incorporate retry mechanisms, exception handling, logging, and browser automation tools like Selenium to enhance the robustness of your web scraping projects.
FAQs
What are some common errors encountered in web scraping? Common errors include 404 errors, timeouts, server-side issues (5XX errors), captchas, and JavaScript-rendered content that cannot be easily parsed.
How can I handle 404 errors effectively? Implement a check to detect 404 errors and either skip the URL or mark it for later inspection. You can use the
requests
library’shead()
method to perform a non-destructive request.What is a retry mechanism, and why is it important? A retry mechanism allows your scraper to attempt fetching a resource multiple times before giving up. This approach is crucial for handling transient errors like network delays or temporary server unavailability.
How can I log errors effectively during web scraping? Use the
logging
module in Python to record errors. Logging helps diagnose issues and improve the reliability of your scraper over time. Ensure that you capture both HTTP errors and exceptions raised by your code.What is exponential backoff, and why should I use it? Exponential backoff involves increasing the delay between retries exponentially to prevent overwhelming the server with rapid requests. This technique helps in managing server load and reduces the likelihood of IP blocking.