· Charlotte Will · 5 min read
What You Need to Know About Using Python for Webscraping
Learn how to use Python for web scraping with our comprehensive guide. Discover step-by-step instructions, best practices, and advanced techniques to extract data from websites efficiently and ethically. Perfect for beginners and intermediate users looking to master web scraping with Python.
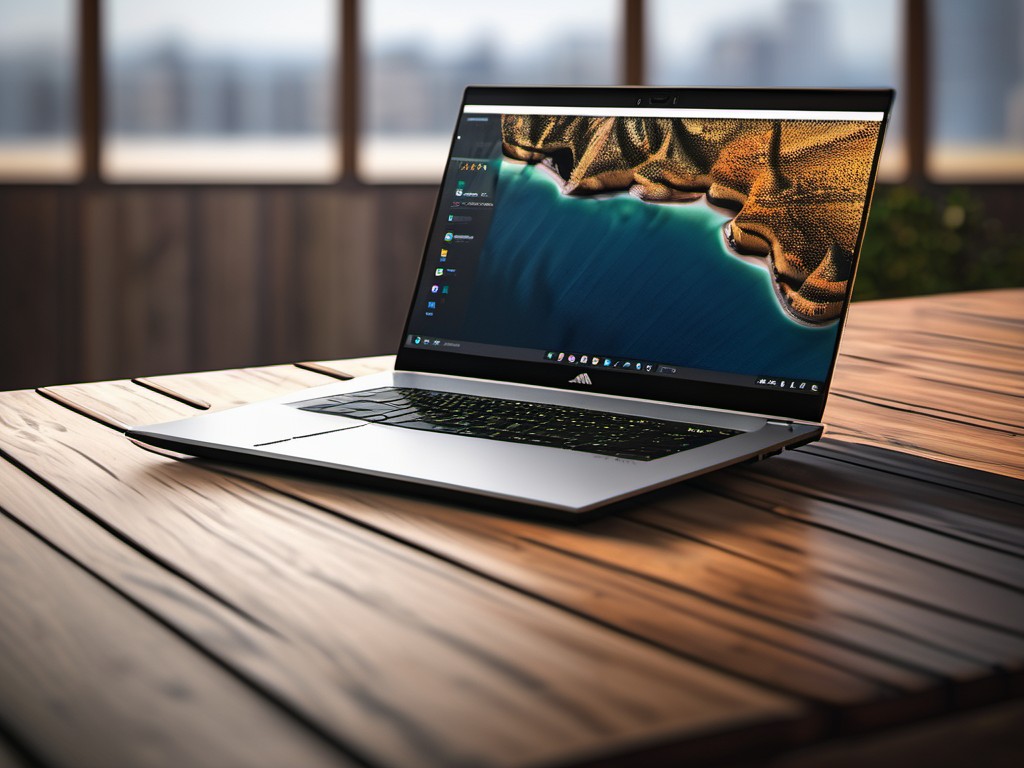
Introduction to Web Scraping with Python
Welcome to the exciting world of web scraping! If you’re reading this, chances are you’re interested in extracting data from websites using Python. But why would you want to do that? And what exactly is web scraping? Let’s dive right in.
Why Use Python for Web Scraping?
Python has become the go-to language for web scraping due to its simplicity, readability, and a plethora of libraries designed specifically for this task. Whether you’re looking to gather data for research, monitor prices on e-commerce sites, or build a database of information, Python makes it easy and efficient.
Basic Concepts of Web Scraping
At its core, web scraping involves sending HTTP requests to websites and parsing the HTML content returned by these requests. The key steps include:
- Sending Requests: Using libraries like
requests
, you can fetch the HTML content from a website. - Parsing HTML: Libraries such as Beautiful Soup or lxml help in extracting data from this HTML content.
- Storing Data: Finally, the extracted data is stored in a format of your choice, like CSV, JSON, or a database.
Setting Up Your Environment
Before you start scraping, it’s essential to set up your environment with all the necessary tools and libraries.
Installing Necessary Libraries
You can install the required Python packages using pip
. Here are some of the most commonly used ones:
- Requests: For sending HTTP requests.
pip install requests
- Beautiful Soup: For parsing HTML and XML documents.
pip install beautifulsoup4
- lxml: An alternative to Beautiful Soup for parsing HTML.
pip install lxml
- Pandas: For data manipulation and analysis.
pip install pandas
Step-by-Step Guide to Web Scraping with Python
Let’s walk through a basic example to get you started with web scraping using Python.
Writing Your First Web Scraper
Here’s a simple script that fetches and prints the title of a webpage:
import requests
from bs4 import BeautifulSoup
# Send a GET request to the webpage
response = requests.get('https://www.example.com')
webContent = response.content
# Parse the content using Beautiful Soup
soup = BeautifulSoup(webContent, 'html.parser')
# Extract and print the title
title = soup.title.string
print(title)
Handling Different Types of Data
Web scraping isn’t always about extracting text. You might need to handle different types of data like images, tables, or even JavaScript-rendered content.
For instance, if you want to scrape a table:
import requests
from bs4 import BeautifulSoup
import pandas as pd
# Send a GET request to the webpage
response = requests.get('https://www.example.com/table')
webContent = response.content
# Parse the content using Beautiful Soup
soup = BeautifulSoup(webContent, 'html.parser')
# Extract and print the table data
table = soup.find('table')
rows = table.find_all('tr')
data = []
for row in rows:
cols = row.find_all('td')
cols = [ele.text.strip() for ele in cols]
data.append([ele for ele in cols if ele]) # Get rid of empty values
# Convert the data to a pandas DataFrame
df = pd.DataFrame(data)
print(df)
Advanced Techniques and Best Practices
As you get more comfortable with web scraping, you’ll encounter more complex scenarios that require advanced techniques.
Dealing with Dynamic Websites
Many modern websites use JavaScript to render content dynamically. For such sites, requests
alone won’t suffice. You can use tools like Selenium or Playwright to interact with these pages as a user would in a browser.
Here’s an example using Selenium:
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.chrome.service import Service
from selenium.webdriver.chrome.options import Options
# Set up the WebDriver
chrome_options = Options()
chrome_options.add_argument("--headless") # Run in headless mode
service = Service('path/to/chromedriver')
driver = webdriver.Chrome(service=service, options=chrome_options)
# Open the website and find the element
driver.get('https://www.example.com')
element = driver.find_element(By.ID, 'dynamic-content')
print(element.text)
# Don't forget to close the WebDriver
driver.quit()
Ethical Considerations in Web Scraping
While web scraping can be incredibly useful, it’s crucial to consider ethical implications:
- Respect Robots.txt: Always check a website’s
robots.txt
file to ensure you’re not scraping restricted areas. - Avoid Overloading Servers: Implement rate limiting in your scripts to prevent overwhelming server resources.
- Data Privacy: Be mindful of personal data and comply with relevant regulations like GDPR.
Common Issues and Troubleshooting
No matter how prepared you are, issues can still arise. Here are some common problems and their solutions:
- HTTP Errors: Check your URLs and network connectivity. Sometimes websites block requests from certain IP addresses or geolocations.
- Parsing Issues: Ensure the HTML structure of the page hasn’t changed. Websites often update their layout, breaking existing scrapers.
- Rate Limiting and Blocking: Use delays between requests (e.g.,
time.sleep(2)
) and consider rotating proxies to avoid getting blocked.
Conclusion
Web scraping with Python is a powerful skill that can open up countless opportunities for data extraction and analysis. Whether you’re a beginner or looking to refine your skills, understanding the basics and best practices will serve you well.
So go ahead, dive into the world of web scraping, and unleash the power of Python!
FAQs
What are some popular libraries for web scraping with Python?
Some popular libraries include requests
for HTTP requests, Beautiful Soup or lxml for parsing HTML, Selenium or Playwright for handling JavaScript-rendered content, and Pandas for data manipulation.
How do I handle websites that require login for scraping?
You can use libraries like Selenium to automate the login process. After logging in via Selenium, you can capture cookies or session information and use them with requests
to make authenticated requests.
Is it legal to scrape any website?
Legality depends on the website’s terms of service and local laws. Always check a site’s robots.txt
file and terms of service before scraping. It’s also important to respect data privacy regulations like GDPR.
What are some alternatives to Python for web scraping?
Other popular languages for web scraping include JavaScript (with tools like Puppeteer), Ruby (with libraries like Nokogiri), and Java (with tools like Jsoup). However, Python remains a favorite due to its simplicity and extensive library support.
How can I handle websites that use CAPTCHA?
CAPTCHAs are designed to prevent automated access. While some services offer solutions for bypassing CAPTCHAs, they are often against the terms of service of many websites. It’s generally better to look for alternative data sources or consider manual data entry.