· Charlotte Will · webscraping · 7 min read
What is Web Scraping with BeautifulSoup?
Discover how to use BeautifulSoup for web scraping in Python! This comprehensive guide covers everything from basic setup to advanced techniques, helping you extract valuable data efficiently and effectively. Ideal for beginners and intermediate users looking to master web scraping skills.
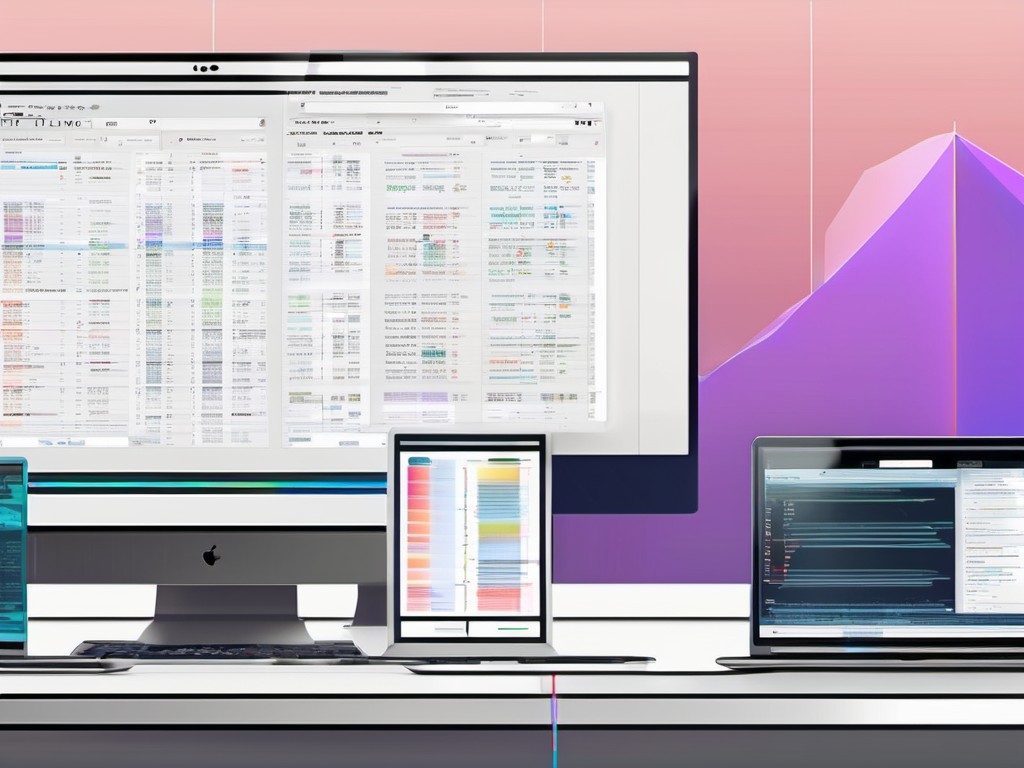
Web scraping has become an essential skill in today’s data-driven world, enabling users to extract valuable information from websites efficiently. Among various web scraping tools available, BeautifulSoup stands out due to its simplicity and effectiveness. This comprehensive guide will delve into what web scraping with BeautifulSoup entails, providing practical advice and actionable content for beginners to intermediate users.
Introduction to Web Scraping
Web scraping is the process of extracting data from websites automatically. It’s like sending a robot to read the web pages you are interested in and pulling out specific pieces of information. This could be anything from product prices, reviews, contact information, or even entire articles. The possibilities are endless.
Why Web Scraping Matters
- Data Collection: Automate data extraction for research, analytics, or market intelligence.
- Competitor Analysis: Keep tabs on your competitors’ strategies and pricing.
- Content Aggregation: Collect news articles, blog posts, or social media updates.
- Lead Generation: Gather contact information from websites to build a marketing database.
What is BeautifulSoup?
BeautifulSoup is a Python library used for parsing HTML and XML documents. It creates parse trees that are helpful to extract the data easily, which makes it perfect for web scraping tasks. BeautifulSoup transforms complex HTML structures into readable formats that can be navigated using familiar Python idioms.
Why Use BeautifulSoup?
- Ease of Use: Simple and intuitive API.
- Flexibility: Supports multiple parsers like lxml, html5lib, etc.
- Community Support: Widely used with extensive documentation and community support.
- Integration: Works seamlessly with other Python libraries like requests for HTTP requests.
Getting Started with BeautifulSoup
Before diving into the details, let’s set up your environment. You need to have Python installed on your computer. If you don’t already have it, download and install from python.org.
Installing BeautifulSoup
You can install BeautifulSoup using pip:
pip install beautifulsoup4
Additionally, you will need a parser like lxml or html5lib. Install one of them as well:
pip install lxml
or
pip install html5lib
Basic Concepts of BeautifulSoup
Parsing HTML with BeautifulSoup
To begin, you need to parse the HTML content. Here’s a basic example:
from bs4 import BeautifulSoup
import requests
# Make a request to the website
url = 'https://example.com'
response = requests.get(url)
html_content = response.content
# Parse the HTML content with BeautifulSoup
soup = BeautifulSoup(html_content, 'lxml') # You can also use 'html5lib' or 'html.parser'
Navigating the Parse Tree
Once you have your soup object, you can navigate through it to find the data you need. Here are some common methods:
soup.title
: Extracts the title of the webpage.soup.find('tag_name')
: Finds the first occurrence of a tag.soup.find_all('tag_name')
: Finds all occurrences of a tag.soup.select('css_selector')
: Uses CSS selectors to find elements.
Extracting Text and Attributes
You can extract text content and attributes from the tags easily:
# Extracting text
title = soup.title.text
# Extracting an attribute (e.g., href from a link)
link = soup.find('a')['href']
Tutorial on Python Web Scraping with BeautifulSoup
Let’s walk through a practical example of how to use BeautifulSoup for web scraping.
Example: Scraping Product Information from an E-commerce Site
Suppose you want to scrape product names and prices from an e-commerce website. Here’s how you can do it:
import requests
from bs4 import BeautifulSoup
# URL of the webpage
url = 'https://example-ecommerce-site.com/products'
# Send a GET request to the webpage
response = requests.get(url)
html_content = response.text
# Parse HTML content with BeautifulSoup
soup = BeautifulSoup(html_content, 'lxml')
# Find all product containers (assuming each product is in a <div> with class 'product-container')
products = soup.find_all('div', class_='product-container')
# Loop through products and extract data
for product in products:
name = product.find('h2').text # Assuming the product name is in an <h2> tag
price = product.find('span', class_='price').text # Assuming the price is in a <span> with class 'price'
print(f"Product: {name}, Price: {price}")
Handling Pagination
Many websites display data across multiple pages. You need to handle pagination to scrape all the data. Here’s an example of how to do it:
import requests
from bs4 import BeautifulSoup
base_url = 'https://example-ecommerce-site.com/products?page='
# Function to extract product info from a single page
def scrape_page(page_url):
response = requests.get(page_url)
soup = BeautifulSoup(response.text, 'lxml')
products = soup.find_all('div', class_='product-container')
for product in products:
name = product.find('h2').text
price = product.find('span', class_='price').text
print(f"Product: {name}, Price: {price}")
# Loop through pages (assuming there are 5 pages)
for page in range(1, 6):
url = base_url + str(page)
scrape_page(url)
Benefits of BeautifulSoup
Ease of Use
BeautifulSoup is designed to be user-friendly. Its API is intuitive, making it accessible even for beginners. You don’t need an in-depth understanding of HTML parsing; the library does most of the heavy lifting for you.
Flexibility and Compatibility
BeautifulSoup supports multiple parsers like lxml, html5lib, and html.parser. This flexibility allows you to choose the parser that best suits your needs. Additionally, it integrates well with other Python libraries such as requests for making HTTP requests.
Community Support
BeautifulSoup is widely used in the web scraping community. It has extensive documentation and numerous tutorials available online. If you encounter issues, chances are someone else has faced them before, and solutions are readily available.
Best Practices for Web Scraping with BeautifulSoup
Respect Robots.txt
Always check the robots.txt
file of a website to see if web scraping is allowed. This file specifies which parts of the site are off-limits to bots. Respect these rules to avoid getting blocked.
import requests
url = 'https://example.com/robots.txt'
response = requests.get(url)
print(response.text)
Be Polite
Don’t overload the server with too many requests at once. Implement delays between your requests to avoid being a nuisance.
import time
# Example of adding a delay between requests
time.sleep(1) # Sleeps for 1 second
Handle Exceptions
Web scraping can be unpredictable due to changes in website structure or server issues. Always handle exceptions gracefully.
import requests
from bs4 import BeautifulSoup
url = 'https://example.com'
try:
response = requests.get(url)
soup = BeautifulSoup(response.text, 'lxml')
# Your scraping code here
except Exception as e:
print(f"An error occurred: {e}")
Use Rotating Proxies
If you are making many requests to a single website, consider using rotating proxies. This helps distribute your requests across different IP addresses, reducing the risk of getting blocked.
Advanced Techniques with BeautifulSoup
Scraping Dynamic Content
Some websites load content dynamically using JavaScript. In such cases, you may need to use a library like Selenium in conjunction with BeautifulSoup.
from selenium import webdriver
from bs4 import BeautifulSoup
import time
# Initialize the WebDriver (assuming Chrome)
driver = webdriver.Chrome()
# Navigate to the URL
url = 'https://example-dynamic-site.com'
driver.get(url)
# Wait for JavaScript to load content
time.sleep(3)
# Get page source and parse with BeautifulSoup
html_content = driver.page_source
soup = BeautifulSoup(html_content, 'lxml')
# Your scraping code here
Storing Scraped Data
You can store the scraped data in various formats like CSV, JSON, or even a database. Here’s an example of saving data to a CSV file:
import csv
# Open a CSV file for writing
with open('products.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerow(['Name', 'Price']) # Write header row
# Assuming products is a list of dictionaries with keys 'name' and 'price'
for product in products:
writer.writerow([product['name'], product['price']])
Conclusion
Web scraping with BeautifulSoup is a powerful way to extract valuable data from websites. Whether you are gathering information for research, keeping tabs on competitors, or aggregating content, BeautifulSoup provides the tools and flexibility you need. By following best practices and leveraging advanced techniques, you can efficiently collect and analyze web data.
FAQs
What is Web Scraping?
Web scraping is an automated process of extracting information from websites. It involves sending HTTP requests to a server and parsing the HTML response to find specific data points.
Why Use BeautifulSoup for Web Scraping?
BeautifulSoup is user-friendly, flexible, and well-supported. It simplifies the process of parsing HTML and extracting data, making it ideal for both beginners and advanced users.
How Do I Install BeautifulSoup?
You can install BeautifulSoup using pip: pip install beautifulsoup4
. Additionally, you may need to install a parser like lxml or html5lib.
Can I Scrape Any Website with BeautifulSoup?
While technically possible, it’s essential to respect the website’s terms of service and check its robots.txt file. Some websites do not allow web scraping and may block your IP if you violate their policies.
What Are Some Best Practices for Web Scraping with BeautifulSoup?
Best practices include respecting robots.txt, implementing delays between requests, handling exceptions gracefully, using rotating proxies, and being polite to the server by not overloading it with too many requests at once.