· Charlotte Will · webscraping · 5 min read
What is Python Webscraping?
Discover what Python web scraping is and how to get started with practical examples using BeautifulSoup, Scrapy, and more. Learn benefits, best practices, and real-world applications in this comprehensive guide.
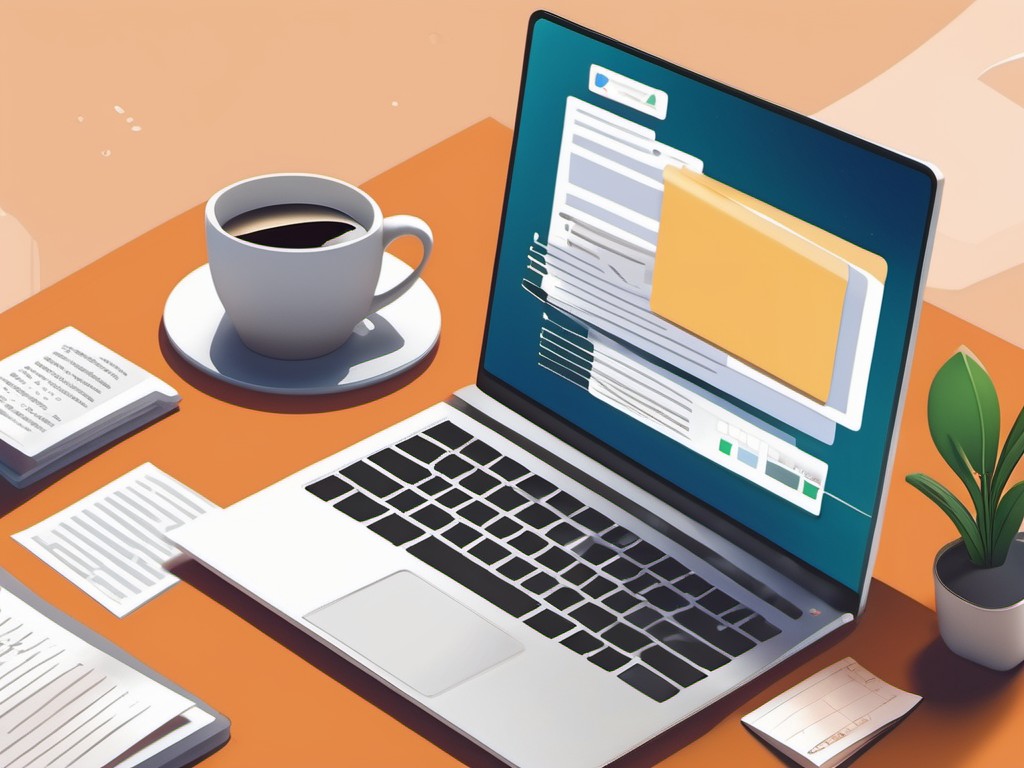
Are you curious about how to extract data from websites efficiently? Welcome to the world of Python web scraping! Whether you’re a beginner or an advanced user looking to brush up on your skills, this comprehensive guide will walk you through everything you need to know. We’ll cover what Python web scraping is, its benefits, and how to get started with practical examples using popular libraries like BeautifulSoup and Scrapy.
Understanding Web Scraping
Web scraping involves extracting data from websites. Imagine a website as a vast library of information—web scraping allows you to sift through this library and collect the specific pieces of information you need. Whether it’s gathering product prices, monitoring social media trends, or compiling research data, web scraping is an invaluable tool for automating these tasks.
What Makes Python Ideal for Web Scraping?
Python stands out as a top choice for web scraping due to its simplicity and powerful libraries. It’s easy to learn, write, and read, making it accessible even for beginners. Additionally, Python boasts robust libraries like BeautifulSoup, Scrapy, and Selenium that are specifically designed to handle web scraping tasks with ease.
Benefits of Python Web Scraping
Automation
Web scraping automates the process of data collection, saving time and reducing manual effort. Instead of copy-pasting information from hundreds of pages, you can write a script to do it for you.
Scalability
Python web scraping is highly scalable. You can start with small scripts and gradually build them into large-scale data collection systems as your needs grow.
Data Analysis
Once you’ve collected the data, Python’s powerful data analysis libraries like Pandas and NumPy allow you to process and analyze it efficiently. This makes Python a one-stop solution for both scraping and analyzing web data.
Getting Started with Python Web Scraping
Setting Up Your Environment
Before diving into coding, make sure your environment is set up properly:
- Install Python: Download and install the latest version of Python from python.org.
- Install Libraries: Use pip to install necessary libraries like BeautifulSoup, Scrapy, and requests.
pip install beautifulsoup4 scrapy requests
Basic Web Scraping with BeautifulSoup
BeautifulSoup is an easy-to-use library for parsing HTML and XML documents. Let’s go through a simple example of web scraping using BeautifulSoup.
Installing BeautifulSoup
pip install beautifulsoup4
Example: Scraping Data from a Webpage
Here’s a step-by-step guide to scraping data from a webpage using BeautifulSoup:
- Import Libraries:
import requests from bs4 import BeautifulSoup
- Send a Request to the Webpage:
url = 'https://example.com' response = requests.get(url)
- Parse HTML Content:
soup = BeautifulSoup(response.content, 'html.parser')
- Extract Data:
title_tag = soup.find('title') print(title_tag.string)
Advanced Web Scraping with Scrapy
Scrapy is a powerful framework designed for large-scale web scraping projects. It’s more complex than BeautifulSoup but offers advanced features like built-in support for asynchronous requests and middleware.
Installing Scrapy
pip install scrapy
Creating a Simple Scrapy Project
- Create a New Scrapy Project:
scrapy startproject myproject
- Define Your Spider: Navigate to the
spiders
directory and create a new Python file, e.g.,example_spider.py
.import scrapy class ExampleSpider(scrapy.Spider): name = "example" start_urls = ['https://example.com'] def parse(self, response): title = response.css('title::text').get() yield {'title': title}
- Run Your Spider:
scrapy crawl example -o output.json
Best Practices for Python Web Scraping
Respect Robots.txt
Always check a website’s robots.txt
file to see if it allows web scraping. This file outlines the rules and permissions for crawling the site.
Throttle Your Requests
Avoid overwhelming servers by limiting the number of requests you make per second. You can achieve this using libraries like time
in Python:
import time
time.sleep(1) # Wait for 1 second between requests
Handle CAPTCHAs and Anti-Scraping Measures
Websites often employ measures to prevent scraping, like CAPTCHAs. For these cases, consider using libraries such as Selenium or third-party services that can solve CAPTCHAs automatically.
Python Libraries for Web Scraping
BeautifulSoup
Ideal for small to medium-scale projects, BeautifulSoup is easy to use and great for beginners.
Scrapy
Perfect for large-scale scraping tasks, Scrapy offers advanced features like middleware and asynchronous requests.
Selenium
Useful for interacting with websites that rely heavily on JavaScript, Selenium automates browser actions to mimic human behavior.
Web Scraping Techniques
CSS Selectors
Use CSS selectors to precisely target the elements you want to scrape. BeautifulSoup and Scrapy both support CSS selectors for easy data extraction.
XPath
Another powerful way to navigate HTML documents, XPath allows you to query specific parts of a webpage effectively.
Real-World Applications of Python Web Scraping
Price Monitoring
Scrape product prices from various e-commerce sites to monitor price changes and trends.
Market Research
Collect data on competitors, market trends, and customer sentiments for in-depth analysis.
SEO Analysis
Gather SEO metrics like backlinks, keywords, and traffic data to optimize your website’s ranking.
Conclusion
Python web scraping is a powerful tool that can save you time, automate tedious tasks, and provide valuable insights from web data. Whether you’re a beginner or an experienced developer, libraries like BeautifulSoup, Scrapy, and Selenium offer flexible solutions for various scraping needs. Always remember to respect website policies and use ethical practices when scraping the web.
FAQs
Is Web Scraping Legal?
Web scraping legality varies by jurisdiction and depends on how you use the data. Always check a website’s terms of service and consult legal advice if unsure.
Can I Scrape Data from Any Website?
Not all websites allow web scraping. Check the robots.txt
file for permissions, and respect any restrictions outlined by the site.
What Are Some Common Challenges in Web Scraping?
Challenges include dealing with CAPTCHAs, anti-scraping measures, handling dynamic content, and ensuring data integrity.
How Can I Handle Dynamic Content on Websites?
For websites that rely heavily on JavaScript, use Selenium to automate browser actions and extract the rendered HTML.
What Is the Best Way to Store Scraped Data?
You can store scraped data in various formats like CSV, JSON, or databases (SQLite, MySQL) depending on your project’s requirements.