· Charlotte Will · 5 min read
What Does Python Webscraping Involve in Web Development
Learn how Python web scraping can enhance your web development projects. Discover practical tips, tools like BeautifulSoup and Scrapy, and best practices to extract data efficiently.
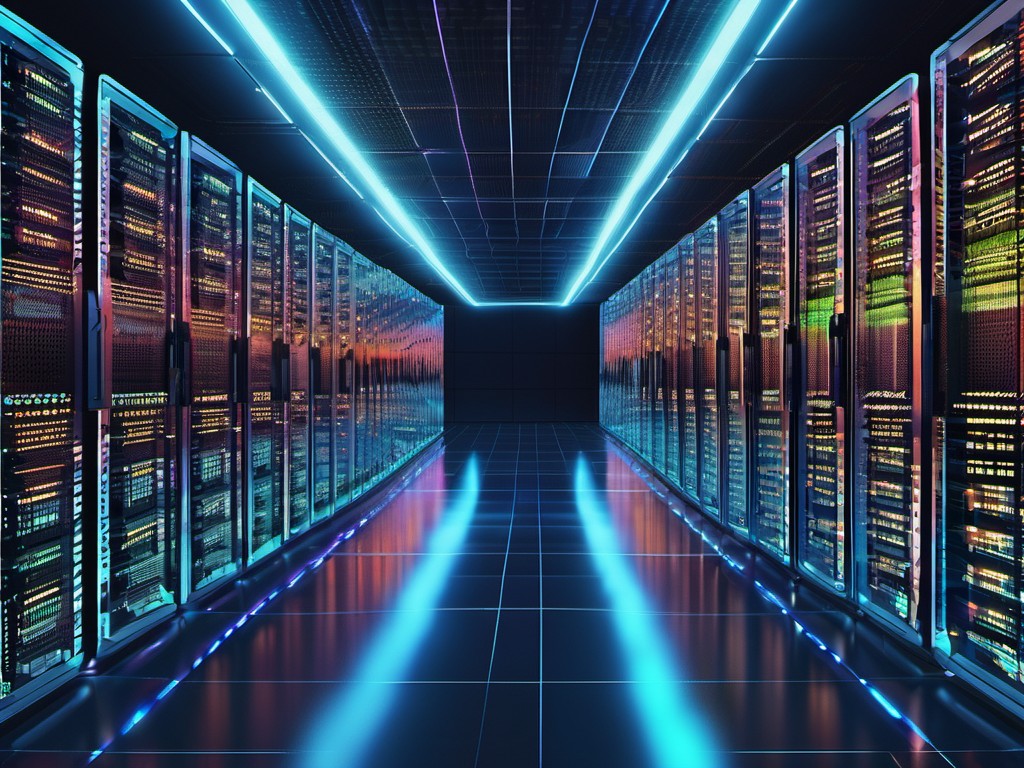
In the rapidly evolving world of web development, data extraction plays a pivotal role. One of the most effective ways to gather data from websites is through web scraping. With Python being one of the most popular programming languages, Python web scraping has become an essential skill for web developers. Let’s delve into what Python web scraping involves and how it can be integrated into web development projects.
Introduction to Python Web Scraping
Web scraping is a technique used to extract data from websites by automating the process of visiting a webpage and parsing its HTML content. This data can then be stored, analyzed, or visualized depending on your needs. Python, with its simplicity and powerful libraries, has become the go-to language for web scraping.
Why Use Python for Web Scraping?
Python’s popularity in web scraping stems from several factors:
- Ease of Use: Python’s syntax is straightforward and easy to learn, making it accessible even to beginners.
- Rich Ecosystem: Libraries like BeautifulSoup, Scrapy, and Selenium simplify the process of web scraping.
- Flexibility: Python can handle both simple and complex scraping tasks with ease.
- Community Support: A large community ensures that you can find help and resources for any issue you might encounter.
Setting Up Your Environment
Before diving into the code, it’s essential to set up your environment properly. Here are the steps:
Installing Python
First, ensure that Python is installed on your system. You can download it from python.org.
Essential Libraries
You will need several libraries for web scraping:
- Requests: To send HTTP requests and handle responses.
pip install requests
- BeautifulSoup: For parsing HTML and XML documents.
pip install beautifulsoup4
- Scrapy: A powerful web scraping framework.
pip install scrapy
- Selenium: To automate browser interactions, useful for handling JavaScript-rendered content.
pip install selenium
Basic Web Scraping with Python
Let’s start with a simple example of how to extract data from a website using the requests
and BeautifulSoup
libraries.
Step-by-Step Guide
Import Libraries
import requests from bs4 import BeautifulSoup
Send an HTTP Request
url = 'https://example.com' response = requests.get(url)
Parse the HTML Content
soup = BeautifulSoup(response.content, 'html.parser')
Extract Data
title = soup.title.string print(f'Title of the page: {title}')
This basic script fetches the HTML content of a webpage and extracts its title. You can expand this to scrape more complex data, such as lists of items or specific elements within the page.
Using BeautifulSoup for HTML Parsing
BeautifulSoup is a powerful library that allows you to navigate and search through the parse tree of an HTML document. It provides Pythonic idioms for iterating, searching, and modifying the parse tree.
Example: Extracting Links from a Webpage
import requests
from bs4 import BeautifulSoup
url = 'https://example.com'
response = requests.get(url)
soup = BeautifulSoup(response.content, 'html.parser')
for link in soup.find_all('a'):
print(link.get('href'))
This script will print all the href attributes of anchor tags on the page.
Advanced Scraping with Scrapy
Scrapy is a more robust framework designed for large-scale scraping projects. It handles many complexities, such as concurrency, download handling, and item processing.
Setting Up a Scrapy Project
Install Scrapy
pip install scrapy
Create a New Project
scrapy startproject myproject
Define an Item and Spider
In
myproject/items.py
:import scrapy class MyItem(scrapy.Item): title = scrapy.Field() link = scrapy.Field()
In
myproject/spiders/example_spider.py
:import scrapy class ExampleSpider(scrapy.Spider): name = 'example' start_urls = ['https://example.com'] def parse(self, response): for item in response.css('div.item'): title = item.css('span.title::text').get() link = item.css('a::attr(href)').get() yield {'title': title, 'link': link}
Running the Spider
scrapy crawl example -o output.json
This will save the scraped data to output.json
.
Handling Dynamic Content with Selenium
Some websites use JavaScript to load content dynamically, making it difficult to scrape using traditional methods like requests
. Selenium can handle such cases by interacting with the webpage as a real user would.
Example: Scraping a JavaScript-Rendered Page
from selenium import webdriver
from bs4 import BeautifulSoup
import time
# Set up the Selenium WebDriver
driver = webdriver.Chrome()
url = 'https://example.com'
driver.get(url)
time.sleep(5) # Allow JavaScript to load content
# Extract the page source and parse it with BeautifulSoup
soup = BeautifulSoup(driver.page_source, 'html.parser')
for link in soup.find_all('a'):
print(link.get('href'))
driver.quit()
Best Practices for Python Web Scraping
- Respect Robots.txt: Always check the
robots.txt
file of a website to understand its scraping policies. - Use Headers: Mimic a real browser by including user-agent headers in your requests.
- Throttle Requests: Avoid overwhelming the server with too many requests at once. Use libraries like
time.sleep()
orscrapy
’s built-in features. - Handle IP Bans: Rotate proxies and user agents to avoid getting banned.
- Error Handling: Implement robust error handling to manage network errors and unexpected content.
- Data Storage: Choose an appropriate storage solution, such as databases or file systems, based on your project requirements.
Conclusion
Python web scraping is a powerful tool in the arsenal of any web developer. Whether you are extracting simple data using requests
and BeautifulSoup
, building complex scrapers with Scrapy
, or handling dynamic content with Selenium
, Python offers a wealth of options to suit your needs. By following best practices and respecting the legal considerations, you can effectively harness the power of web scraping in your development projects.
FAQs
What are the legal considerations for web scraping?
- Always check a website’s
robots.txt
file and terms of service before scraping data. Respect intellectual property rights and avoid scraping sensitive or personal information without permission.
- Always check a website’s
How do I handle dynamic content with JavaScript?
- Use tools like Selenium that can interact with JavaScript-rendered content. These tools mimic user behavior, allowing you to scrape dynamically loaded data.
What are some best practices for avoiding IP bans while scraping?
- Implement request throttling, rotate proxies and user agents, and use headers that mimic real browser requests. Also, consider using a VPN or proxy services to hide your IP address.
Can I scrape data from any website?
- No, you should not scrape data from any website without permission. Always check the website’s policies and obtain necessary permissions if required.
How do I store the scraped data for further analysis?
- You can store the scraped data in various formats such as JSON, CSV, or databases like SQLite, PostgreSQL, or MongoDB depending on your project requirements and the volume of data involved.