· Charlotte Will · 4 min read
Webscraping with Python: How to Extract Useful Information
Learn how to extract valuable information from websites using Python's powerful web scraping techniques. This comprehensive guide covers basic and advanced methods, best practices, and ethical considerations for successful data extraction.
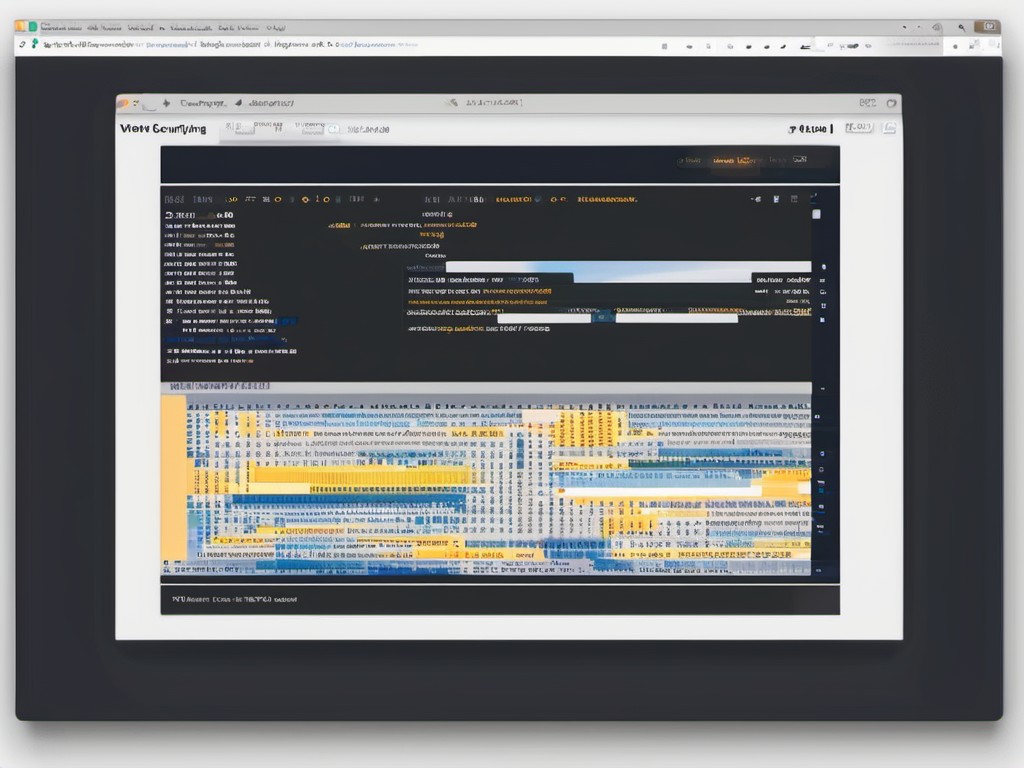
Introduction to Web Scraping with Python
Web scraping is an essential skill for data analysts, researchers, and developers alike. It allows you to extract valuable information from websites automatically. With Python, web scraping becomes a breeze thanks to its powerful libraries like BeautifulSoup and Selenium. Whether you’re looking to gather data for analysis or automate repetitive tasks, this guide will help you get started with web scraping using Python.
Setting Up Your Environment
Before diving into the code, let’s set up our environment. You’ll need Python installed on your system along with a few key libraries.
Installing Required Libraries
First, make sure you have Python installed. You can download it from python.org. Once that’s done, open your terminal or command prompt and create a new virtual environment:
python -m venv webscraping-env
Activate the virtual environment:
# On Windows
webscraping-env\Scripts\activate
# On macOS/Linux
source webscraping-env/bin/activate
Now, install the necessary libraries using pip
:
pip install requests beautifulsoup4 selenium
You’ll also need a WebDriver for Selenium. You can download it from Selenium Downloads.
Basic Web Scraping Techniques
Let’s start with some basic techniques using BeautifulSoup and Selenium for dynamic content.
Using BeautifulSoup
BeautifulSoup is a great library for parsing HTML and XML documents. Here’s how you can use it to scrape data from a static website:
import requests
from bs4 import BeautifulSoup
url = 'https://example.com'
response = requests.get(url)
soup = BeautifulSoup(response.content, 'html.parser')
# Extracting all the headings
headings = soup.find_all('h1')
for heading in headings:
print(heading.text)
Using Selenium for Dynamic Content
For websites that load content dynamically using JavaScript, you’ll need Selenium. Here’s an example of how to scrape such a site:
from selenium import webdriver
from selenium.webdriver.common.by import By
import time
# Path to your WebDriver executable (make sure it's in your PATH)
driver = webdriver.Chrome()
url = 'https://example.com/dynamic-content'
driver.get(url)
time.sleep(5) # Wait for the content to load
# Extracting data after JavaScript has loaded it
elements = driver.find_elements(By.TAG_NAME, 'h1')
for element in elements:
print(element.text)
driver.quit()
Advanced Topics in Python Web Scraping
Now that we have the basics covered, let’s dive into some advanced topics.
Handling Pagination and Infinite Scroll
Many websites use pagination or infinite scroll to load more content. You can handle this by simulating user interactions like clicking “Next” buttons or scrolling down:
from selenium.webdriver.common.keys import Keys
# For infinite scroll
last_height = driver.execute_script("return document.body.scrollHeight")
while True:
driver.find_element(By.TAG_NAME, "body").send_keys(Keys.END)
time.sleep(2) # Wait for new content to load
new_height = driver.execute_script("return document.body.scrollHeight")
if new_height == last_height:
break
last_height = new_height
# For pagination
next_button = driver.find_element(By.LINK_TEXT, "Next")
while next_button.is_displayed():
next_button.click()
time.sleep(2) # Wait for the new page to load
next_button = driver.find_element(By.LINK_TEXT, "Next")
Dealing with Captchas and Anti-Scraping Measures
Some websites employ captchas or other anti-scraping measures. In such cases, you might need to use a service like 2Captcha or solve the captchas manually. Be mindful of the website’s terms of service to ensure you’re not violating any rules.
Best Practices for Ethical Web Scraping
Respect Robots.txt
Always check the robots.txt
file of a website before scraping it. This file specifies which parts of the site can be crawled and indexed by automated agents.
https://example.com/robots.txt
Be Polite to Servers
Avoid making too many requests in a short period. Implement delays between your requests using time.sleep()
.
import time
time.sleep(2) # Sleep for 2 seconds
Use Headers and User Agents
Simulate a real browser by setting appropriate headers:
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36'
}
response = requests.get(url, headers=headers)
Frequently Asked Questions (FAQ)
Is web scraping legal?
Web scraping can be legal as long as you respect the website’s terms of service and robots.txt rules. It’s also important to use the data responsibly and ethically.
How do I handle JavaScript-heavy websites?
For sites with heavy JavaScript, Selenium is a powerful tool since it can render the page as a real browser would.
What are some ethical considerations in web scraping?
Ethical considerations include respecting the website’s robots.txt file, not overwhelming servers with too many requests, and using the data responsibly without violating privacy laws.
How can I avoid getting blocked by a website?
To minimize the risk of being blocked, use delays between requests (time.sleep()
), rotate IP addresses or use proxies, and respect the site’s rules and terms of service.
Can I scrape data from any website?
While technically possible, it’s not ethical or legal to scrape data from websites without permission. Always check the site’s terms of service and robots.txt file before proceeding.