· Charlotte Will · webscraping · 4 min read
Using WebSockets for Real-Time Web Scraping Applications
Discover how WebSockets enhance real-time web scraping applications with improved performance and scalability. Learn practical tips, best practices, and code examples to implement effective real-time data extraction using WebSockets in Python.
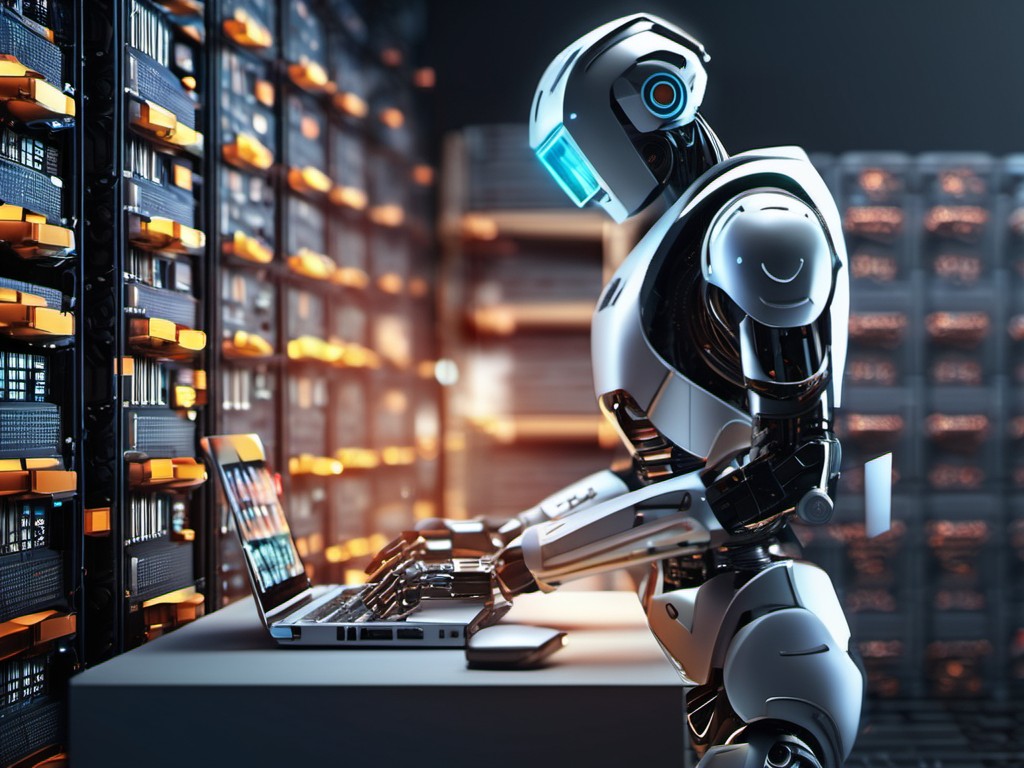
Using WebSockets for Real-Time Web Scraping Applications
Real-time web scraping has become increasingly essential in today’s data-driven world, where businesses need instant access to live data feeds. Traditional HTTP-based web scraping methods can be slow and inefficient, especially when dealing with dynamic content that updates frequently. This is where WebSockets come into play, offering a powerful solution for real-time data extraction.
What are WebSockets? WebSockets provide full-duplex communication channels over a single TCP connection. Unlike HTTP requests, which involve opening and closing connections repeatedly, WebSockets maintain a persistent connection between the client and server. This makes them ideal for applications requiring real-time data updates, such as live sports scores, stock market tickers, or social media feeds.
Benefits of Using WebSockets in Real-Time Web Scraping
Improved Performance
- With a persistent connection, WebSockets reduce latency and improve the speed of data transmission. This is crucial for real-time applications where delays can be costly.
Efficient Resource Usage
- By eliminating the overhead associated with multiple HTTP requests, WebSockets make more efficient use of network resources. This translates to lower bandwidth usage and reduced server load.
Scalability
- WebSockets can handle a large number of simultaneous connections, making them highly scalable for applications that need to process real-time data from numerous sources.
Instant Updates
- Real-time web scraping applications using WebSockets receive instant updates as soon as the data changes on the server side. This ensures that your application always displays the latest information.
Getting Started with WebSocket-Based Real-Time Web Scraping
To implement real-time web scraping using WebSockets, you need to follow a few key steps:
Establish a WebSocket Connection
- Begin by establishing a connection between your client and the server that provides the data feeds. Here’s an example in Python using the
websocket-client
library:from websocket import create_connection ws = create_connection("wss://example.com/socket") print("Connection established!")
- Begin by establishing a connection between your client and the server that provides the data feeds. Here’s an example in Python using the
Handle Real-Time Data
- Once the connection is established, you can start receiving real-time data updates. Here’s how to handle incoming messages:
while True: result = ws.recv() print(result)
- Once the connection is established, you can start receiving real-time data updates. Here’s how to handle incoming messages:
Optimize Performance
- To ensure optimal performance, consider the following best practices:
- Compression: Use data compression techniques to reduce the amount of data transmitted over the network.
- Message Batching: Combine multiple updates into a single message to minimize the number of transmissions.
- Efficient Parsing: Use efficient parsing libraries to quickly process incoming data and extract relevant information.
- To ensure optimal performance, consider the following best practices:
Implementing WebSocket-Based Real-Time Web Scraping in Python
Here’s a more comprehensive example demonstrating how to use WebSockets for real-time web scraping with Python:
Install Required Libraries
pip install websocket-client
Create the WebSocket Client
from websocket import create_connection import json def on_message(ws, message): data = json.loads(message) print("Received data:", data) # Process and extract relevant information here def on_error(ws, error): print("Error occurred:", error) def on_close(ws): print("Connection closed") def on_open(ws): ws.send("Hello Server!") print("Sent message to server") if __name__ == "__main__": websocket_url = "wss://example.com/socket" ws = create_connection(websocket_url) ws.on_message = on_message ws.on_error = on_error ws.on_close = on_close ws.on_open = on_open print("Connecting to {}".format(websocket_url)) try: import time while True: time.sleep(1) except KeyboardInterrupt: ws.close()
Troubleshooting Common Issues
- Connection Errors: Ensure that the WebSocket server URL is correct and accessible. Check your network connection and firewall settings.
- Data Parsing Issues: Make sure the data format received from the server matches what you expect. Use appropriate parsing libraries to handle different data types (e.g., JSON, XML).
- Performance Bottlenecks: Profile your application to identify any performance bottlenecks. Optimize network transmission, message handling, and data processing steps as needed.
Conclusion
WebSockets provide a powerful solution for real-time web scraping applications, offering improved performance, efficient resource usage, scalability, and instant updates. By following best practices and optimizing your implementation, you can build robust and effective real-time data extraction systems.
FAQs
What is the difference between WebSockets and traditional HTTP requests?
- WebSockets maintain a persistent connection between the client and server, whereas traditional HTTP requests involve opening and closing connections repeatedly for each request/response cycle.
How can I ensure that my WebSocket-based web scraping application is scalable?
- Optimize your server and client code to handle multiple simultaneous connections efficiently. Use load balancing techniques and consider horizontally scaling your infrastructure as needed.
Can WebSockets be used for both real-time data extraction and sending updates to clients?
- Yes, WebSockets enable full-duplex communication, allowing both the client and server to send and receive messages in real time. This makes them suitable for a wide range of applications requiring live data feeds.
What are some common issues I might encounter when using WebSockets for web scraping?
- Common issues include connection errors, data parsing problems, and performance bottlenecks. Troubleshoot these by ensuring correct server URLs, matching expected data formats, and optimizing your code for better performance.
How can I secure my WebSocket connections?
- Use WebSocket Secure (WSS) to encrypt communication between the client and server. Implement authentication mechanisms and consider using token-based authorization to ensure that only authorized clients can connect to your WebSocket server.