· Charlotte Will · 5 min read
Using Python Webscraping to Make API Calls for Real-Time Data Collection
Learn how to use Python for web scraping and making API calls to collect real-time data effectively. This comprehensive guide covers practical examples, best practices, and ethical considerations for both techniques. Improve your data collection skills with Python today!
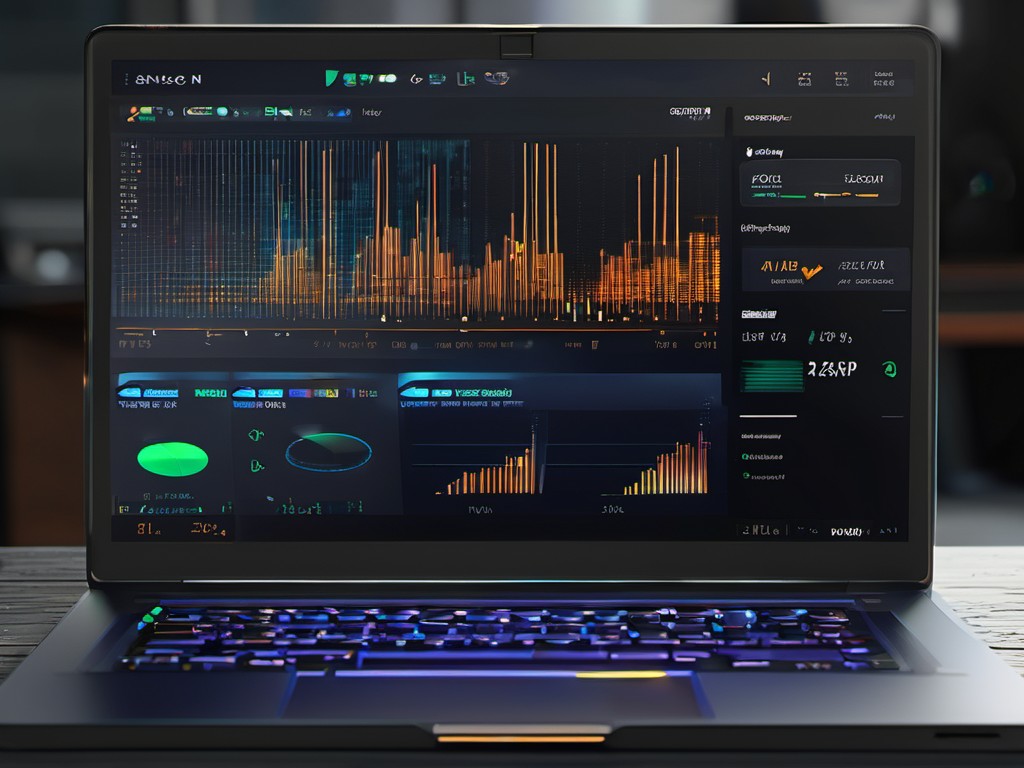
In today’s data-driven world, collecting real-time information is crucial for businesses, researchers, and developers alike. While APIs provide structured access to data, sometimes the data you need isn’t available through an API. This is where web scraping comes in—it allows you to extract data directly from websites. Python, with its rich ecosystem of libraries, makes this task easier than ever. Let’s dive into how you can use Python for both web scraping and making API calls to collect real-time data effectively.
Introduction to Web Scraping with Python
Web scraping involves extracting data from websites programmatically. This could be anything from product prices, news articles, social media posts, or even weather updates. Python is a popular choice for web scraping due to its simplicity and powerful libraries like BeautifulSoup
and Scrapy
.
Why Use Python for Web Scraping?
Python’s ease of use, along with its vast library support, makes it an ideal language for web scraping. Libraries such as requests
, BeautifulSoup
, and Scrapy
streamline the process of fetching and parsing HTML content. Additionally, Python’s readability ensures that your code is maintainable and easy to understand.
Setting Up Your Environment
Before you start scraping or making API calls, ensure you have a proper environment set up. Here’s how:
- Install Python: If you haven’t already, download and install the latest version of Python from python.org.
- Create a Virtual Environment: This helps in managing dependencies.
python -m venv myenv source myenv/bin/activate # On Windows use `myenv\Scripts\activate`
- Install Required Libraries:
pip install requests beautifulsoup4 scrapy
Making API Calls for Real-Time Data Collection
Making API calls in Python is straightforward with the requests
library. APIs provide a structured way to fetch data, which can be more reliable and faster than web scraping. Here’s how you can get started:
Basic API Requests
The requests
library allows you to send HTTP requests easily.
import requests
response = requests.get('https://api.example.com/data')
data = response.json() # Parse JSON response
print(data)
This simple snippet fetches data from an API endpoint and prints it out.
Handling Authentication
Many APIs require authentication, typically using an API key.
headers = {
'Authorization': 'Bearer YOUR_API_KEY'
}
response = requests.get('https://api.example.com/data', headers=headers)
print(response.json())
Replace YOUR_API_KEY
with your actual API key.
Practical Examples of Web Scraping
Let’s look at some practical examples using BeautifulSoup
and Scrapy
.
Example 1: Using BeautifulSoup
Here’s a simple web scraper to extract headlines from a news website.
import requests
from bs4 import BeautifulSoup
url = 'https://news.example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
headlines = soup.find_all('h2', class_='headline')
for headline in headlines:
print(headline.get_text())
This script fetches the webpage and extracts all headlines with the class headline
.
Example 2: Using Scrapy for Complex Scraping
For more complex scraping tasks, Scrapy
is a powerful framework. First, you need to set up a new Scrapy project.
scrapy startproject news_scraper
cd news_scraper
Create a spider in the spiders
directory:
import scrapy
class NewsSpider(scrapy.Spider):
name = 'news'
start_urls = ['https://news.example.com']
def parse(self, response):
headlines = response.css('h2.headline::text').getall()
for headline in headlines:
yield {'headline': headline}
Run the spider:
scrapy crawl news -o news.json
This will save the extracted data into a news.json
file.
Combining Web Scraping and API Calls
Sometimes, you might need to combine both web scraping and API calls for comprehensive data collection. For instance, you could scrape a website for initial data and then make API calls to fetch additional details.
Example: Fetching Weather Data
Let’s say you want to extract weather information from a website that doesn’t provide an API. You can scrape the base data and use the location to make an API call for detailed weather forecasts.
import requests
from bs4 import BeautifulSoup
# Step 1: Web Scraping to get location data
url = 'https://weather.example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
location = soup.find('span', class_='location').get_text()
# Step 2: Making API call to get detailed weather data
api_url = f'https://api.weatherapi.com/v1/current.json?key=YOUR_API_KEY&q={location}'
api_response = requests.get(api_url)
weather_data = api_response.json()
print(weather_data)
This script first scrapes the location from a website and then uses that location to fetch detailed weather data via an API.
Best Practices for Web Scraping and API Calls
- Respect Robots.txt: Always check a site’s
robots.txt
file to understand its scraping policies. - Rate Limiting: Be mindful of the rate at which you send requests to avoid overwhelming servers.
- Error Handling: Implement robust error handling to manage network issues and API errors gracefully.
- Data Storage: Decide where and how to store your scraped data (e.g., databases, files).
- Ethical Considerations: Ensure that your web scraping activities comply with legal and ethical guidelines.
Conclusion
Web scraping and making API calls are powerful techniques for collecting real-time data in Python. By combining these methods, you can gather comprehensive data tailored to your needs. Whether you’re using requests
for simple API calls or leveraging BeautifulSoup
and Scrapy
for complex web scraping tasks, Python offers the flexibility and tools required for effective data collection.
FAQs
Q: What are some common use cases of web scraping?
- A: Common use cases include price monitoring, market research, news aggregation, lead generation, and social media analysis.
Q: How do I handle paginated content while web scraping?
- A: You can handle paginated content by iterating through the pages’ URLs or using Scrapy’s
LinkExtractor
to follow “next” links automatically.
- A: You can handle paginated content by iterating through the pages’ URLs or using Scrapy’s
Q: Can I use web scraping for commercial purposes?
- A: While it is technically possible, always ensure you comply with the website’s terms of service and legal regulations. It’s often safer to use official APIs for commercial data needs.
Q: How can I avoid getting blocked while web scraping?
- A: Use techniques like rotating IP addresses, setting reasonable delays between requests, and respecting the website’s
robots.txt
.
- A: Use techniques like rotating IP addresses, setting reasonable delays between requests, and respecting the website’s
Q: What should I do if an API requires authentication with OAuth?
- A: Libraries such as
requests-oauthlib
can help you handle OAuth authentication easily by managing tokens and authorization flows.
- A: Libraries such as